The Secret Connection Between Your Coding Style and Your Personality Type
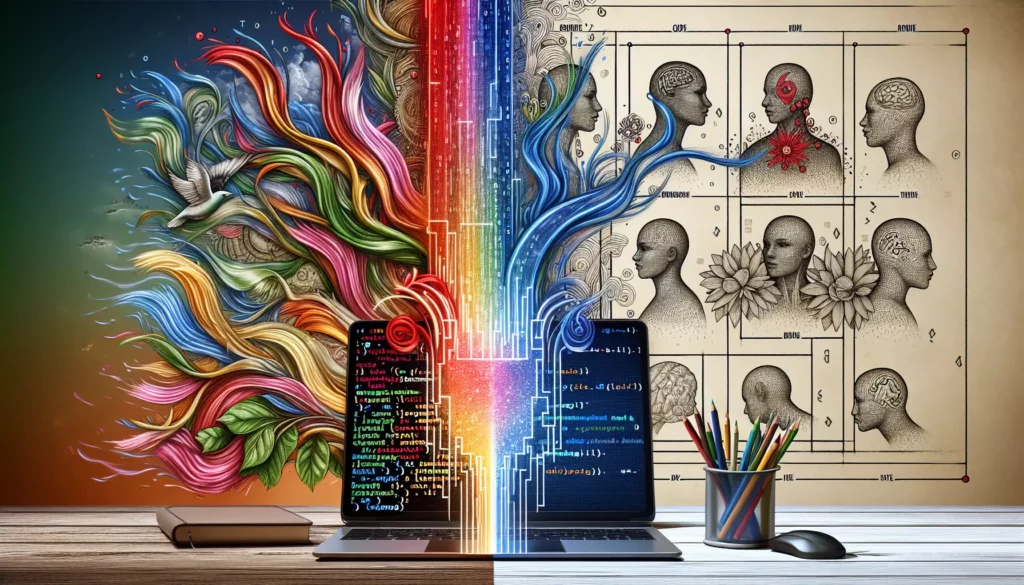
Have you ever wondered why you code the way you do? Or why your colleague’s code looks so different from yours, even though you’re both following the same style guide? The answer might lie deeper than you think – in your personality.
As software developers, we often focus on technical skills, algorithms, and best practices. But there’s an intriguing connection between our coding style and our personality type that’s worth exploring. This blog post will dive into the fascinating world where psychology meets programming, revealing how your personality traits might influence your coding habits and preferences.
Understanding Personality Types
Before we delve into the connection between personality and coding style, let’s briefly review some common personality frameworks:
Myers-Briggs Type Indicator (MBTI)
The MBTI is one of the most widely recognized personality assessments. It categorizes individuals into 16 personality types based on four dichotomies:
- Extraversion (E) vs. Introversion (I)
- Sensing (S) vs. Intuition (N)
- Thinking (T) vs. Feeling (F)
- Judging (J) vs. Perceiving (P)
Big Five (OCEAN)
The Big Five model, also known as OCEAN, identifies five broad personality traits:
- Openness to experience
- Conscientiousness
- Extraversion
- Agreeableness
- Neuroticism
Now that we have a basic understanding of personality types, let’s explore how they might influence coding styles.
Coding Styles and Personality Traits
1. Code Organization and Structure
Judging (J) vs. Perceiving (P) in MBTI
Developers with a strong preference for Judging (J) tend to approach coding with a structured and organized mindset. They often prefer:
- Clear and consistent naming conventions
- Well-defined function and class structures
- Comprehensive documentation
On the other hand, those leaning towards Perceiving (P) might have a more flexible approach:
- Adaptable coding structures
- Quick prototyping and iterative development
- More focus on functionality than structure initially
Consider these two code snippets for a simple calculator function:
Judging (J) style:
def add(a: float, b: float) -> float:
"""
Adds two numbers and returns the result.
Args:
a (float): The first number to add.
b (float): The second number to add.
Returns:
float: The sum of a and b.
"""
return a + b
def subtract(a: float, b: float) -> float:
"""
Subtracts the second number from the first and returns the result.
Args:
a (float): The number to subtract from.
b (float): The number to subtract.
Returns:
float: The difference between a and b.
"""
return a - b
# More functions for multiplication, division, etc.
Perceiving (P) style:
def calc(op, x, y):
if op == '+':
return x + y
elif op == '-':
return x - y
# More operations can be added easily
The Judging style is more structured and documented, while the Perceiving style is more flexible and concise.
2. Problem-Solving Approach
Sensing (S) vs. Intuition (N) in MBTI
Sensing (S) individuals tend to focus on concrete facts and details. In coding, this might translate to:
- Step-by-step problem-solving
- Preference for well-defined algorithms
- Detailed comments explaining each step
Intuition (N) types, on the other hand, might approach problems more holistically:
- Focus on the big picture and overall system design
- Preference for creative or novel solutions
- Comments that explain the overall strategy rather than individual steps
Let’s look at how these different approaches might tackle a sorting problem:
Sensing (S) style:
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Traverse the array from 0 to n-i-1
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Intuition (N) style:
def quick_sort(arr):
# Base case: arrays with 0 or 1 element are already sorted
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
The Sensing approach uses a straightforward, step-by-step bubble sort algorithm, while the Intuition approach opts for a more abstract and efficient quicksort implementation.
3. Code Commenting and Documentation
Thinking (T) vs. Feeling (F) in MBTI
Thinking (T) types tend to prioritize logic and consistency. In coding, this might manifest as:
- Concise, factual comments
- Focus on technical details and performance considerations
- Preference for code that speaks for itself with minimal explanation
Feeling (F) types, however, might approach documentation with more consideration for the human element:
- More extensive comments explaining the reasoning behind decisions
- Consideration of how the code affects users or team members
- Emphasis on making code accessible and understandable to others
Here’s how these different approaches might document a function:
Thinking (T) style:
def calculate_discount(price, discount_rate):
# Apply discount rate to price
return price * (1 - discount_rate)
Feeling (F) style:
def calculate_discount(price, discount_rate):
"""
Calculates the discounted price of an item.
This function helps us provide fair and transparent pricing to our customers.
It ensures that discounts are applied consistently across our product range.
Args:
price (float): The original price of the item.
discount_rate (float): The discount rate as a decimal (e.g., 0.1 for 10% off).
Returns:
float: The price after applying the discount.
Example:
>>> calculate_discount(100, 0.2)
80.0
"""
discounted_price = price * (1 - discount_rate)
return discounted_price
The Thinking style provides just enough information to understand what the function does, while the Feeling style offers more context and consideration for the user of the function.
4. Coding Environment and Tools
Extraversion (E) vs. Introversion (I) in MBTI
While this dichotomy might not directly affect coding style, it can influence a developer’s preferred working environment:
Extraverted (E) developers might prefer:
- Collaborative coding environments (e.g., pair programming)
- Tools that facilitate real-time code sharing and discussion
- Regular code reviews and team discussions
Introverted (I) developers might lean towards:
- Quiet, focused coding environments
- Tools that support deep work and minimize distractions
- Asynchronous communication for code reviews and discussions
5. Code Complexity and Abstraction
Openness to Experience in Big Five
Developers high in Openness to Experience might:
- Embrace new programming paradigms and languages
- Experiment with complex, abstract solutions
- Enjoy working with cutting-edge technologies
Those lower in Openness might prefer:
- Tried-and-true coding patterns
- Straightforward, concrete implementations
- Stable, well-established technologies
Consider these two approaches to implementing a simple data structure:
High Openness style:
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
if not self.head:
self.head = Node(value)
return
current = self.head
while current.next:
current = current.next
current.next = Node(value)
def __iter__(self):
current = self.head
while current:
yield current.value
current = current.next
# Usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
for value in ll:
print(value)
Low Openness style:
class SimpleList:
def __init__(self):
self.items = []
def append(self, value):
self.items.append(value)
def get_all(self):
return self.items
# Usage
sl = SimpleList()
sl.append(1)
sl.append(2)
sl.append(3)
for value in sl.get_all():
print(value)
The high Openness style implements a more complex linked list structure with custom iteration, while the low Openness style opts for a simpler wrapper around a built-in list.
6. Code Maintenance and Refactoring
Conscientiousness in Big Five
Highly conscientious developers tend to:
- Regularly refactor and optimize code
- Maintain consistent coding standards
- Pay attention to edge cases and error handling
Developers lower in conscientiousness might:
- Focus more on getting features working quickly
- Be more relaxed about following coding standards
- Address edge cases and optimization as needed rather than proactively
Here’s an example of how these approaches might differ in handling a simple user input scenario:
High Conscientiousness style:
def get_user_age():
while True:
try:
age = int(input("Please enter your age: "))
if 0 <= age <= 120:
return age
else:
print("Age must be between 0 and 120. Please try again.")
except ValueError:
print("Invalid input. Please enter a number.")
def main():
age = get_user_age()
print(f"Your age is: {age}")
Low Conscientiousness style:
def main():
age = input("Enter your age: ")
print(f"Your age is: {age}")
The high Conscientiousness style includes input validation, error handling, and a separate function for getting user input, while the low Conscientiousness style focuses on the basic functionality without these additional considerations.
The Impact of Personality on Team Dynamics
Understanding the connection between personality types and coding styles can have significant implications for team dynamics and project management in software development:
1. Team Composition
A diverse team with various personality types can bring different strengths to a project:
- Judging (J) types can ensure project structure and deadlines are met
- Perceiving (P) types can adapt quickly to changing requirements
- Sensing (S) types can focus on implementation details
- Intuition (N) types can provide innovative solutions and system-level thinking
2. Code Reviews
Awareness of personality differences can improve the code review process:
- Thinking (T) types might focus on logical consistency and performance
- Feeling (F) types might consider user experience and code readability
- Conscientious team members might pay extra attention to coding standards and potential edge cases
3. Project Planning
Different personality types may prefer different project management approaches:
- Judging (J) types might prefer structured methodologies like Waterfall
- Perceiving (P) types might thrive in more flexible Agile environments
- Extraverted (E) team members might prefer frequent stand-ups and pair programming
- Introverted (I) developers might prefer more independent work with asynchronous communication
4. Conflict Resolution
Understanding personality differences can help in resolving conflicts:
- A debate over code structure might be rooted in Judging vs. Perceiving preferences
- Disagreements about documentation might stem from Thinking vs. Feeling approaches
- Tensions over project pace could relate to differences in Conscientiousness
Leveraging Personality Insights for Better Coding
While it’s important to recognize the influence of personality on coding style, it’s equally crucial not to use these insights as a way to pigeonhole developers or excuse poor coding practices. Instead, this knowledge can be leveraged to improve both individual and team performance:
1. Personal Growth
Understanding your own personality type and its influence on your coding style can help you:
- Identify your strengths and leverage them
- Recognize areas for improvement and work on them
- Adapt your style when working on different types of projects or with different team members
2. Team Collaboration
Recognizing personality differences in your team can lead to:
- Better task allocation based on individual strengths
- Improved communication by understanding different perspectives
- More effective pair programming by matching complementary styles
3. Code Quality
A team with diverse personality types can contribute to better overall code quality:
- Structured thinkers can ensure robust architecture
- Creative problem-solvers can provide innovative solutions
- Detail-oriented members can catch potential bugs and edge cases
- Empathetic coders can improve user experience and code readability
4. Continuous Learning
Exposure to different coding styles can broaden your skills:
- Learn to appreciate approaches that differ from your natural style
- Experiment with new coding patterns and paradigms
- Develop a more well-rounded skill set by adopting practices from various personality types
Conclusion
The connection between personality types and coding styles is a fascinating area that merits further exploration. By understanding this relationship, we can gain valuable insights into our own coding habits, improve team dynamics, and ultimately write better code.
Remember, the goal isn’t to change who you are or to judge others based on their personality type. Instead, it’s about recognizing and appreciating the diverse strengths that different personalities bring to the table. By embracing this diversity and learning from each other, we can create more robust, efficient, and innovative software solutions.
As you continue your coding journey, take some time to reflect on your own personality traits and how they might influence your coding style. Are you a structured planner or a flexible adapter? Do you focus on the details or the big picture? By understanding yourself better, you can not only improve your own coding skills but also become a more effective team member in the diverse world of software development.
The next time you’re working on a coding project, whether it’s a personal endeavor or a team effort, consider how personality types might be influencing the process. You might just discover new ways to leverage your strengths, complement your teammates, and create even better software.
Happy coding, and may your unique personality shine through in every line of code you write!