What to Expect in a Mobile Development Interview
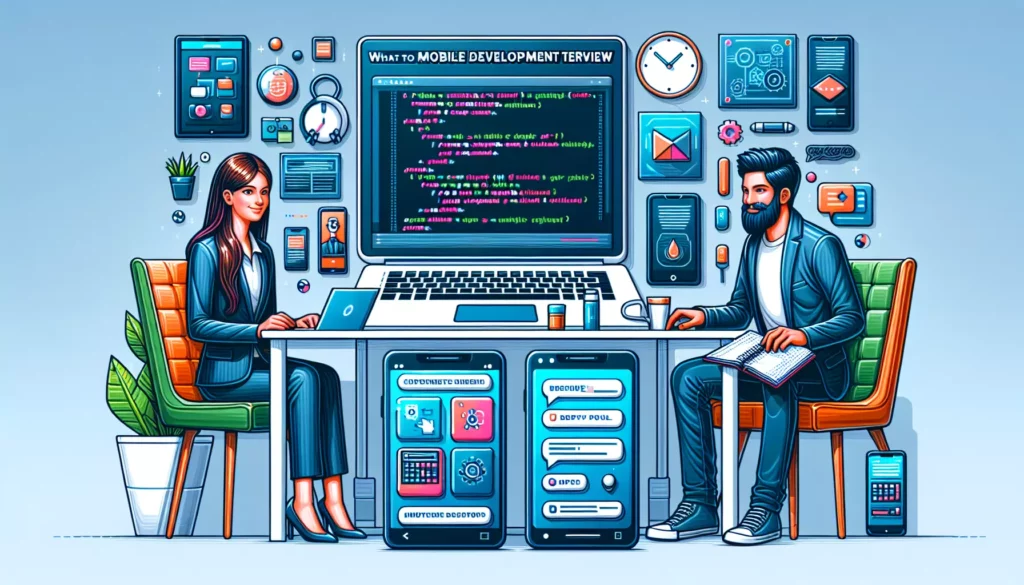
As the mobile app industry continues to flourish, the demand for skilled mobile developers is at an all-time high. Whether you’re a seasoned developer or a fresh graduate looking to break into the field, preparing for a mobile development interview can be a daunting task. This comprehensive guide will walk you through what to expect in a mobile development interview, covering everything from technical questions to soft skills assessments.
1. Understanding the Mobile Development Landscape
Before diving into the specifics of interview preparation, it’s crucial to understand the current mobile development landscape. Mobile development primarily focuses on two major platforms:
- iOS (Apple devices)
- Android (Google’s operating system)
Some companies also develop cross-platform applications using frameworks like React Native, Flutter, or Xamarin. Knowing which platform or framework the company you’re interviewing with uses is essential for tailoring your preparation.
2. Technical Knowledge
The bulk of your mobile development interview will likely focus on your technical knowledge. Here are some key areas you should be prepared to discuss:
2.1. Programming Languages
Depending on the platform, you should be well-versed in the following languages:
- iOS: Swift and Objective-C
- Android: Java and Kotlin
- Cross-platform: JavaScript (for React Native), Dart (for Flutter), or C# (for Xamarin)
Be prepared to answer questions about language-specific features, syntax, and best practices.
2.2. Mobile Development Frameworks and SDKs
Familiarity with platform-specific development tools is crucial:
- iOS: Xcode, Cocoa Touch
- Android: Android Studio, Android SDK
- Cross-platform: React Native CLI, Flutter SDK, Xamarin SDK
Interviewers may ask about your experience with these tools and how you’ve used them in previous projects.
2.3. UI/UX Design Principles
Mobile developers often work closely with designers, so understanding UI/UX principles is important. Be prepared to discuss:
- Platform-specific design guidelines (Material Design for Android, Human Interface Guidelines for iOS)
- Responsive design and adapting to different screen sizes
- Accessibility features and their implementation
2.4. Data Management and Persistence
Knowledge of data storage and management is crucial for mobile development. Be ready to talk about:
- Local storage options (SQLite, Core Data, Room)
- Remote data fetching and API integration
- State management (Redux, MobX, Provider)
2.5. Performance Optimization
Mobile apps need to be efficient and responsive. Expect questions about:
- Memory management and avoiding leaks
- Optimizing app launch time
- Reducing battery consumption
- Efficient use of network resources
2.6. Testing and Debugging
Quality assurance is a critical part of mobile development. Be prepared to discuss:
- Unit testing frameworks (XCTest, JUnit)
- UI testing and automation
- Debugging tools and techniques
- Continuous Integration/Continuous Deployment (CI/CD) practices
3. Coding Challenges
Many mobile development interviews include coding challenges. These can range from whiteboard coding exercises to take-home projects. Here are some types of challenges you might encounter:
3.1. Algorithm and Data Structure Problems
While not always directly related to mobile development, these questions test your problem-solving skills and coding ability. Common topics include:
- Array manipulation
- String processing
- Tree and graph traversal
- Dynamic programming
Here’s an example of a simple array manipulation problem you might encounter:
// Given an array of integers, return indices of the two numbers
// such that they add up to a specific target.
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
// Example usage:
console.log(twoSum([2, 7, 11, 15], 9)); // Output: [0, 1]
3.2. Mobile-Specific Coding Tasks
These challenges are more directly related to mobile development. Examples include:
- Implementing a custom UI component
- Creating a simple app that fetches and displays data from an API
- Optimizing a given piece of code for better performance
Here’s a simple example of fetching data from an API in Swift:
import Foundation
func fetchData(from url: String, completion: @escaping (Result<Data, Error>) -> Void) {
guard let url = URL(string: url) else {
completion(.failure(NSError(domain: "Invalid URL", code: 0, userInfo: nil)))
return
}
URLSession.shared.dataTask(with: url) { (data, response, error) in
if let error = error {
completion(.failure(error))
return
}
guard let data = data else {
completion(.failure(NSError(domain: "No data received", code: 0, userInfo: nil)))
return
}
completion(.success(data))
}.resume()
}
// Usage:
fetchData(from: "https://api.example.com/data") { result in
switch result {
case .success(let data):
print("Data received: \(data)")
case .failure(let error):
print("Error: \(error.localizedDescription)")
}
}
3.3. System Design Questions
For more senior positions, you might be asked to design a mobile app or a specific feature. This tests your ability to think about architecture, scalability, and user experience. Topics might include:
- Designing a chat application
- Creating a photo sharing app
- Implementing a caching system for offline use
4. Soft Skills and Behavioral Questions
Technical skills are crucial, but soft skills are equally important in a team-based development environment. Be prepared to answer questions about:
4.1. Collaboration and Communication
- How do you work with designers and back-end developers?
- Can you describe a time when you had to explain a technical concept to a non-technical stakeholder?
- How do you handle code reviews and feedback?
4.2. Problem-Solving and Adaptability
- Describe a challenging bug you encountered and how you solved it.
- How do you stay updated with the latest mobile development trends and technologies?
- How do you approach learning a new programming language or framework?
4.3. Project Management
- How do you prioritize tasks in a project?
- Can you describe your experience with Agile development methodologies?
- How do you handle tight deadlines or changing requirements?
5. Portfolio and Past Projects
Be prepared to discuss your previous work in detail. Interviewers may ask about:
- Specific apps you’ve developed
- Challenges you faced during development and how you overcame them
- Your role in team projects
- Any open-source contributions or personal projects
Having a well-maintained GitHub profile or a personal website showcasing your projects can be a significant advantage.
6. Industry Knowledge
Demonstrating awareness of the mobile industry and its trends can set you apart. Be prepared to discuss:
- Recent updates to iOS or Android
- Emerging technologies in mobile development (e.g., AR, 5G)
- App Store and Google Play policies and best practices
- Mobile app monetization strategies
7. Interview Process and Tips
The mobile development interview process typically involves several stages:
- Initial phone or video screening
- Technical interview(s)
- Coding challenge or take-home project
- On-site interview (may include multiple rounds with different team members)
Here are some tips to help you succeed:
- Practice coding on a whiteboard or shared document, as many interviews are conducted this way.
- Be prepared to explain your thought process while solving problems.
- Review the company’s products or apps before the interview.
- Prepare questions to ask your interviewers about the role, team, and company.
- Be honest about what you know and don’t know. If you’re unsure about something, it’s okay to say so and explain how you would find the answer.
8. Conclusion
Preparing for a mobile development interview requires a combination of technical knowledge, practical skills, and soft skills. By focusing on the areas outlined in this guide, you’ll be well-equipped to tackle the challenges of the interview process.
Remember, the key to success is not just knowing the answers, but demonstrating your problem-solving skills, your ability to learn and adapt, and your passion for mobile development. With thorough preparation and the right mindset, you’ll be well on your way to landing your dream job in mobile development.
Good luck with your interview preparation, and don’t forget to stay curious and keep learning. The mobile development field is constantly evolving, and the best developers are those who embrace this change and continually strive to improve their skills.