Technical Interviews: What to Expect and How to Prepare
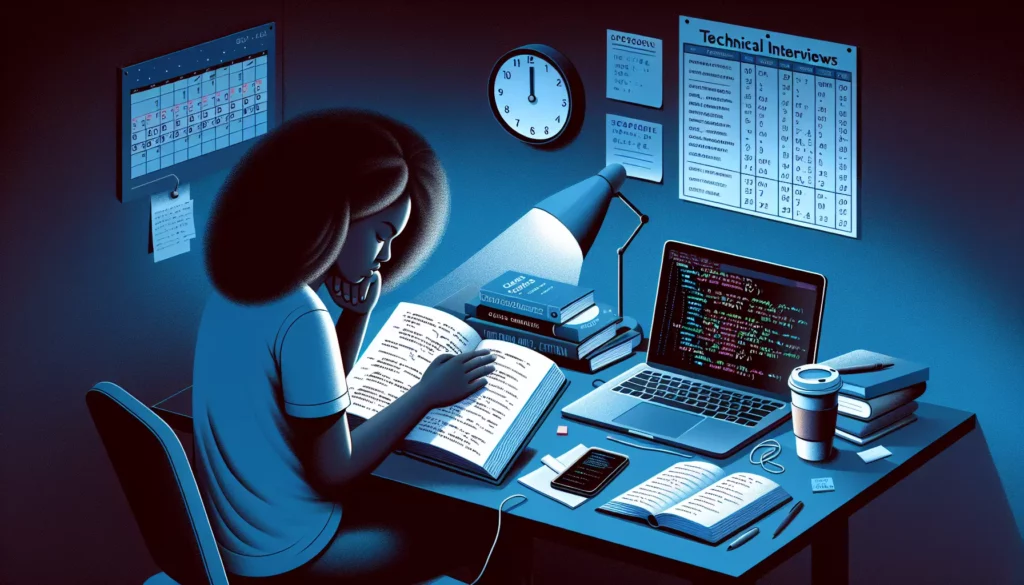
Technical interviews are a crucial step in the hiring process for software engineering and other technology-related positions. These interviews are designed to assess a candidate’s technical skills, problem-solving abilities, and cultural fit within the company. For many aspiring developers, especially those aiming for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), preparing for technical interviews can be a daunting task. In this comprehensive guide, we’ll explore what to expect during technical interviews and provide strategies to help you prepare effectively.
Understanding the Technical Interview Process
Before diving into preparation strategies, it’s essential to understand the typical structure of technical interviews. While the exact format may vary depending on the company and position, most technical interviews follow a similar pattern:
- Initial Screening: This may involve a phone or video call with a recruiter or hiring manager to discuss your background and interest in the position.
- Technical Phone Screen: A brief technical assessment, often involving coding or problem-solving questions.
- Take-home Assignment: Some companies may provide a coding project to complete within a specified timeframe.
- On-site Interviews: A series of face-to-face interviews (or virtual equivalents) with team members and potential colleagues.
- Final Decision: The company evaluates your performance and makes a hiring decision.
What to Expect in Technical Interviews
Technical interviews can cover a wide range of topics and formats. Here are some common elements you might encounter:
1. Coding Challenges
Expect to solve coding problems on a whiteboard, in a shared online coding environment, or on paper. These challenges typically focus on data structures, algorithms, and problem-solving skills. You may be asked to:
- Implement a specific algorithm or data structure
- Solve a problem using optimal time and space complexity
- Debug or optimize existing code
- Explain your thought process and approach to problem-solving
2. System Design Questions
For more senior positions or roles at larger companies, you may be asked to design a system or architecture. This could involve:
- Designing a scalable web service
- Creating a database schema for a given scenario
- Explaining how you would approach building a complex feature or product
3. Behavioral Questions
While technical skills are crucial, companies also want to assess your soft skills and cultural fit. Expect questions about:
- Your past experiences and projects
- How you handle challenges and conflicts
- Your approach to teamwork and collaboration
- Your career goals and motivations
4. Technical Concepts and Knowledge
Interviewers may ask questions to gauge your understanding of fundamental computer science concepts and industry best practices. Topics might include:
- Object-oriented programming principles
- Database design and SQL
- Web technologies and protocols
- Version control systems (e.g., Git)
- Software development methodologies
How to Prepare for Technical Interviews
Now that you know what to expect, let’s explore strategies to help you prepare effectively for technical interviews:
1. Master Data Structures and Algorithms
A strong foundation in data structures and algorithms is crucial for success in technical interviews. Focus on understanding and implementing the following:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Sorting and searching algorithms
- Dynamic programming
- Recursion
Practice implementing these data structures and algorithms from scratch in your preferred programming language. Platforms like AlgoCademy offer interactive coding tutorials and resources to help you master these fundamental concepts.
2. Solve Practice Problems
Regularly solving coding problems is one of the most effective ways to prepare for technical interviews. Here are some strategies:
- Use online platforms like LeetCode, HackerRank, or AlgoCademy to access a wide variety of coding challenges.
- Start with easier problems and gradually increase the difficulty as you improve.
- Time yourself while solving problems to simulate interview conditions.
- Review and learn from other people’s solutions after solving a problem.
- Focus on understanding the problem-solving process rather than memorizing solutions.
3. Study System Design
For more experienced developers or those applying to senior positions, system design knowledge is crucial. To prepare:
- Read books on system design and architecture (e.g., “Designing Data-Intensive Applications” by Martin Kleppmann).
- Study real-world architectures of popular services and platforms.
- Practice designing systems on a whiteboard or with diagrams.
- Understand scalability concepts, load balancing, caching, and database design.
4. Review Computer Science Fundamentals
Brush up on core computer science concepts that you may have learned in school or through self-study. Key areas to review include:
- Operating systems
- Computer networks
- Database management systems
- Object-oriented programming principles
- Design patterns
5. Practice Behavioral Questions
Prepare for behavioral questions by reflecting on your past experiences and projects. Use the STAR method (Situation, Task, Action, Result) to structure your responses. Some tips:
- Prepare specific examples of challenges you’ve faced and how you overcame them.
- Practice explaining technical concepts in simple terms.
- Be ready to discuss your strengths, weaknesses, and areas for improvement.
- Research the company’s culture and values to align your responses accordingly.
6. Mock Interviews
Conducting mock interviews is an excellent way to simulate the real interview experience and receive feedback. You can:
- Practice with friends or colleagues in the industry.
- Use online platforms that offer mock interview services with experienced interviewers.
- Record yourself answering questions to review your performance and body language.
7. Build Projects
Working on personal projects demonstrates your passion for coding and gives you practical experience to discuss during interviews. Consider:
- Building a web application or mobile app
- Contributing to open-source projects
- Implementing algorithms or data structures from scratch
- Creating a portfolio website to showcase your projects
Tips for Success During the Interview
When the day of your technical interview arrives, keep these tips in mind to maximize your chances of success:
1. Communicate Clearly
Clear communication is crucial during technical interviews. Make sure to:
- Ask clarifying questions about the problem before starting to code.
- Explain your thought process and approach as you work through the problem.
- Use proper technical terminology and explain concepts clearly.
- Be open to feedback and suggestions from the interviewer.
2. Think Aloud
Interviewers want to understand your problem-solving process. Verbalize your thoughts as you work through the problem, discussing:
- Your initial approach to solving the problem
- Any assumptions you’re making
- Trade-offs between different solutions
- Time and space complexity considerations
3. Start with a Simple Solution
When faced with a coding challenge:
- Begin with a brute-force or naive solution to demonstrate your understanding of the problem.
- Discuss the limitations of this approach and potential optimizations.
- Iteratively improve your solution, explaining your reasoning at each step.
4. Test Your Code
After implementing a solution:
- Walk through your code with a simple example to ensure it works as expected.
- Consider edge cases and how your solution handles them.
- Discuss potential improvements or alternative approaches.
5. Stay Calm and Positive
Technical interviews can be stressful, but maintaining a positive attitude is essential:
- Take deep breaths and stay focused if you feel overwhelmed.
- If you’re stuck, explain your thought process and ask for hints if needed.
- View the interview as a learning experience and an opportunity to showcase your skills.
Common Pitfalls to Avoid
Be aware of these common mistakes that candidates make during technical interviews:
- Jumping into coding without fully understanding the problem
- Failing to consider edge cases or test your solution
- Becoming defensive or argumentative when receiving feedback
- Giving up too quickly when faced with a challenging problem
- Neglecting to ask clarifying questions when needed
- Focusing solely on the solution without explaining your thought process
Leveraging Tools and Resources
To enhance your preparation for technical interviews, consider using the following tools and resources:
1. Online Coding Platforms
Platforms like AlgoCademy, LeetCode, and HackerRank offer a wealth of coding challenges and resources to help you practice:
- Solve problems across various difficulty levels and topics
- Participate in coding contests to test your skills under time pressure
- Review solutions and explanations from other users
- Track your progress and identify areas for improvement
2. Interview Preparation Books
Several books can provide valuable insights and strategies for technical interviews:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “System Design Interview” by Alex Xu
3. Online Courses and Video Tutorials
Supplement your learning with online courses and video tutorials:
- Coursera and edX offer computer science and algorithm courses from top universities
- YouTube channels like “Back To Back SWE” and “TechLead” provide algorithm explanations and interview tips
- Platforms like Udemy and Pluralsight offer comprehensive interview preparation courses
4. GitHub Repositories
Explore GitHub repositories that curate interview preparation resources:
- Algorithm implementations and explanations
- Coding challenge solutions
- System design resources and examples
- Interview experiences and tips from successful candidates
Conclusion
Preparing for technical interviews requires dedication, practice, and a structured approach. By understanding what to expect, focusing on core computer science concepts, and honing your problem-solving skills, you can significantly increase your chances of success. Remember that the interview process is not just about finding the perfect solution but also about demonstrating your thought process, communication skills, and ability to learn and adapt.
As you embark on your interview preparation journey, consider leveraging platforms like AlgoCademy that offer comprehensive resources, interactive coding tutorials, and AI-powered assistance to help you progress from beginner-level coding to mastering technical interview skills. With consistent practice and the right mindset, you’ll be well-equipped to tackle even the most challenging technical interviews at top tech companies.
Good luck with your preparation, and remember that each interview, regardless of the outcome, is an opportunity to learn and grow as a developer!