The Best Way to Prepare for Competitive Programming
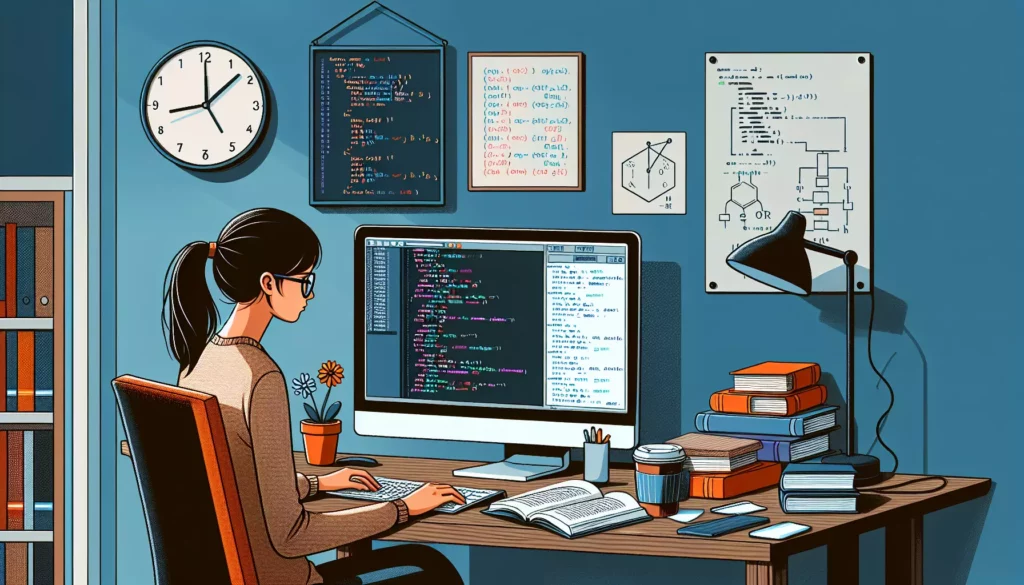
Competitive programming has become increasingly popular in recent years, attracting students, professionals, and coding enthusiasts alike. It’s not just a hobby; it’s a gateway to improving problem-solving skills, enhancing algorithmic thinking, and even landing coveted positions at top tech companies. In this comprehensive guide, we’ll explore the best strategies and resources to prepare for competitive programming, helping you excel in contests and boost your overall coding prowess.
Understanding Competitive Programming
Before diving into preparation strategies, it’s crucial to understand what competitive programming entails. Competitive programming is a mind sport where participants solve well-defined computational problems within a limited time frame. These contests test not only coding skills but also problem-solving abilities, algorithm knowledge, and the capacity to work under pressure.
Key Components of Competitive Programming
- Problem-solving skills
- Algorithmic knowledge
- Efficient coding practices
- Time management
- Ability to handle stress
Setting the Foundation: Essential Skills and Knowledge
To excel in competitive programming, you need a solid foundation in several key areas:
1. Master a Programming Language
Choose a language and become proficient in it. Popular choices include:
- C++
- Java
- Python
While C++ is often preferred due to its speed and extensive standard library, any of these languages can be suitable for competitive programming.
2. Understand Data Structures
Familiarize yourself with essential data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
3. Learn Fundamental Algorithms
Master these core algorithmic concepts:
- Sorting and Searching
- Recursion and Backtracking
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (BFS, DFS, Shortest Path)
- String Algorithms
4. Develop Problem-Solving Techniques
Learn and practice various problem-solving approaches:
- Divide and Conquer
- Sliding Window
- Two Pointers
- Binary Search
- Memoization
Creating a Structured Learning Plan
With the basics covered, it’s time to create a structured learning plan to systematically improve your skills.
1. Start with the Basics
Begin by solving easy problems to build confidence and reinforce fundamental concepts. Platforms like LeetCode, HackerRank, and Codeforces offer a wide range of beginner-friendly problems.
2. Gradually Increase Difficulty
As you become comfortable with easier problems, gradually move to medium and hard difficulty levels. This progression helps you build problem-solving stamina and exposes you to more complex algorithms and data structures.
3. Focus on Specific Topics
Dedicate time to master one topic at a time. For example, spend a week focusing solely on graph algorithms, then move on to dynamic programming the next week.
4. Participate in Online Contests
Regularly participate in online coding contests. Websites like Codeforces, AtCoder, and TopCoder host weekly or bi-weekly contests that simulate real competitive programming environments.
5. Analyze and Learn from Solutions
After each contest or problem-solving session, take time to analyze your solutions and compare them with more efficient ones. This practice helps you learn new techniques and optimize your code.
Leveraging Online Resources and Tools
The internet offers a wealth of resources for aspiring competitive programmers. Here are some top recommendations:
1. Online Judges and Practice Platforms
- LeetCode: Offers a vast collection of coding problems with difficulty ratings.
- HackerRank: Provides a mix of tutorials and coding challenges.
- Codeforces: Hosts regular coding contests and has an extensive problem archive.
- AtCoder: Known for high-quality problems and regular contests.
- SPOJ (Sphere Online Judge): Features a large problem set covering various algorithms.
2. Educational Websites and Courses
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
- Coursera: Provides courses on algorithms and competitive programming from top universities.
- edX: Offers free online courses on computer science and programming.
3. Books and References
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Competitive Programming 3” by Steven and Felix Halim
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
4. YouTube Channels and Blogs
- Errichto: Offers tutorials and live coding sessions.
- William Lin: Provides insights into competitive programming strategies.
- Algorithms Live!: Explains complex algorithms in an accessible manner.
Developing Effective Problem-Solving Strategies
To excel in competitive programming, you need to develop efficient problem-solving strategies. Here are some techniques to enhance your approach:
1. Read and Understand the Problem Thoroughly
Before diving into coding, make sure you fully understand the problem statement. Identify the input format, output requirements, and any constraints or edge cases.
2. Break Down Complex Problems
For difficult problems, break them down into smaller, manageable sub-problems. Solve each sub-problem independently and then combine the solutions.
3. Start with Brute Force
Begin with a simple, brute-force solution. This helps you understand the problem better and provides a baseline for optimization.
4. Optimize Incrementally
Once you have a working solution, look for ways to optimize it. Consider time and space complexity improvements.
5. Learn to Recognize Problem Patterns
As you solve more problems, you’ll start recognizing common patterns. This skill allows you to quickly identify the type of algorithm or data structure needed for a given problem.
6. Practice Implementing Algorithms from Scratch
While many programming languages offer built-in functions for common algorithms, it’s crucial to know how to implement them from scratch. This deep understanding can be invaluable during competitions.
Time Management and Contest Strategies
Effective time management is crucial in competitive programming contests. Here are some strategies to maximize your performance:
1. Read All Problems First
Quickly skim through all the problems at the beginning of the contest. This gives you an overview of what to expect and helps you prioritize.
2. Start with the Easiest Problem
Begin with the problem you find easiest. This builds confidence and ensures you score some points early on.
3. Don’t Get Stuck
If you’re stuck on a problem for too long, move on to the next one. You can always come back later if time permits.
4. Practice Time-Boxed Problem Solving
When practicing, set time limits for each problem to simulate contest conditions. This helps improve your speed and decision-making under pressure.
5. Learn to Estimate Time Complexity
Develop the ability to quickly estimate the time complexity of your solutions. This skill helps you avoid implementing solutions that will exceed the time limit.
Improving Code Efficiency and Debugging Skills
Writing efficient code and quickly identifying and fixing bugs are crucial skills in competitive programming.
1. Write Clean and Modular Code
Even under time pressure, strive to write clean, readable code. This makes debugging easier and reduces the chances of introducing errors.
2. Use Templates
Develop a personal code template with commonly used functions and macros. This can save precious time during contests.
3. Master Your IDE or Text Editor
Learn keyboard shortcuts and efficient coding practices in your chosen development environment.
4. Practice Debugging Techniques
Learn to use debugging tools effectively. Practice finding and fixing bugs in your code and others’ code.
5. Optimize Your Code
Learn techniques for writing more efficient code, such as:
- Using appropriate data structures
- Avoiding unnecessary computations
- Leveraging bitwise operations when applicable
Building a Competitive Programming Mindset
Success in competitive programming isn’t just about technical skills; it also requires the right mindset.
1. Embrace Challenges
View difficult problems as opportunities to learn and grow rather than insurmountable obstacles.
2. Learn from Failures
Don’t get discouraged by incorrect submissions or low contest rankings. Analyze your mistakes and use them as learning experiences.
3. Stay Persistent
Improvement in competitive programming takes time and consistent effort. Stay motivated and keep practicing regularly.
4. Collaborate and Learn from Others
Engage with the competitive programming community. Participate in forums, discuss problems with peers, and learn from top performers.
5. Balance Practice with Rest
While consistent practice is important, make sure to give yourself adequate rest to avoid burnout.
Advanced Topics for Competitive Programming
As you progress in your competitive programming journey, you’ll encounter more advanced topics. Here are some areas to explore:
1. Advanced Data Structures
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
- Disjoint Set Union (DSU)
- Trie
2. Advanced Algorithms
- Network Flow Algorithms
- String Matching Algorithms (KMP, Z-algorithm)
- Lowest Common Ancestor (LCA)
- Heavy-Light Decomposition
3. Mathematical Concepts
- Number Theory
- Combinatorics
- Probability
- Game Theory
4. Optimization Techniques
- Bit Manipulation
- Meet in the Middle
- Heavy-Light Decomposition
Real-world Applications of Competitive Programming Skills
The skills developed through competitive programming have numerous real-world applications:
1. Technical Interviews
Many top tech companies use coding interviews similar to competitive programming problems. Your practiced problem-solving skills give you a significant advantage.
2. Efficient Software Development
The ability to write optimized code and solve complex problems efficiently translates directly to real-world software development.
3. Algorithm Design
Your deep understanding of algorithms can be applied to designing efficient solutions for real-world computational problems.
4. Research and Innovation
The analytical and problem-solving skills honed in competitive programming are valuable in fields like computer science research and technological innovation.
Conclusion
Preparing for competitive programming is a challenging but rewarding journey. It requires dedication, consistent practice, and a strategic approach to learning. By following the strategies outlined in this guide, you can systematically improve your skills and excel in competitive programming contests.
Remember that success in competitive programming is not just about winning contests; it’s about developing a problem-solving mindset, improving your coding skills, and preparing yourself for a successful career in technology. Whether you aim to top the leaderboards in international competitions or simply want to enhance your coding abilities, the skills you gain through competitive programming will serve you well in your professional journey.
Start your competitive programming journey today, and embrace the challenges and growth opportunities it offers. Happy coding!