How to Prioritize Time and Space Efficiency in Coding Challenges
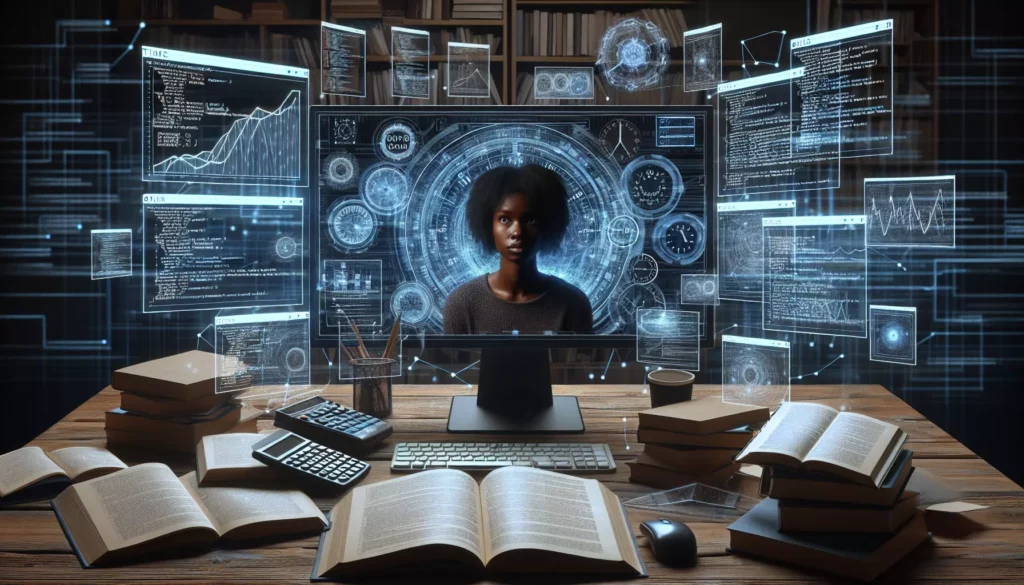
In the world of competitive programming and technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), the ability to write efficient code is paramount. This skill goes beyond just solving problems; it’s about solving them in the most optimal way possible, considering both time and space complexity. In this comprehensive guide, we’ll explore strategies to prioritize time and space efficiency in coding challenges, helping you elevate your problem-solving skills to the next level.
Understanding the Importance of Efficiency
Before diving into specific strategies, it’s crucial to understand why efficiency matters so much in coding challenges and technical interviews:
- Real-world applicability: Efficient algorithms translate to better performance in real-world applications, which is critical for large-scale systems.
- Demonstrating problem-solving skills: Optimizing solutions showcases your ability to think critically and approach problems from multiple angles.
- Interview success: Many tech companies, especially FAANG, place a high emphasis on algorithmic efficiency during their interview processes.
- Scalability: Efficient solutions are more likely to scale well with larger inputs, a crucial consideration in today’s data-driven world.
Time Efficiency: Strategies and Techniques
Time efficiency refers to how quickly an algorithm can execute as the input size grows. Here are some strategies to improve time efficiency:
1. Choose the Right Data Structures
Selecting appropriate data structures can significantly impact the time complexity of your solution. Consider these examples:
- Arrays vs. Linked Lists: Arrays offer O(1) access time but O(n) insertion/deletion at arbitrary positions. Linked lists have O(n) access time but O(1) insertion/deletion at known positions.
- Hash Tables: Provide O(1) average-case lookup, insertion, and deletion, making them excellent for quick access to elements.
- Binary Search Trees: Offer O(log n) operations for search, insertion, and deletion in balanced trees.
2. Utilize Efficient Algorithms
Familiarize yourself with common algorithmic techniques and their time complexities:
- Sorting: QuickSort (O(n log n) average case) vs. BubbleSort (O(n²))
- Searching: Binary Search (O(log n)) vs. Linear Search (O(n))
- Graph Traversal: BFS/DFS (O(V + E)) for most graph problems
3. Implement Memoization and Dynamic Programming
For problems with overlapping subproblems, consider these techniques:
- Memoization: Store results of expensive function calls and return the cached result when the same inputs occur again.
- Dynamic Programming: Build solutions to subproblems and use them to construct solutions for larger problems.
Here’s a simple example of memoization in Python for calculating Fibonacci numbers:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
print(fibonacci(100)) # Calculates 100th Fibonacci number efficiently
4. Optimize Loops and Conditionals
Careful structuring of loops and conditionals can lead to significant time savings:
- Minimize the number of nested loops when possible.
- Use break statements to exit loops early when conditions are met.
- Arrange conditional statements so that the most likely conditions are checked first.
5. Leverage Built-in Functions and Libraries
Many programming languages offer optimized built-in functions and libraries. Using these can often be more efficient than implementing algorithms from scratch:
// JavaScript example
// Less efficient:
function customSort(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] > arr[j]) {
[arr[i], arr[j]] = [arr[j], arr[i]];
}
}
}
return arr;
}
// More efficient:
function efficientSort(arr) {
return arr.sort((a, b) => a - b);
}
Space Efficiency: Strategies and Techniques
Space efficiency refers to how much memory an algorithm uses as the input size grows. Here are strategies to improve space efficiency:
1. In-place Algorithms
Whenever possible, modify the input data structure directly instead of creating new ones. This approach can significantly reduce space complexity:
// Java example: In-place array reversal
public static void reverseArray(int[] arr) {
int start = 0;
int end = arr.length - 1;
while (start < end) {
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
2. Reuse Variables
Instead of creating new variables for each operation, reuse existing ones when possible:
// Python example
# Less space-efficient
def sum_array(arr):
total = 0
for num in arr:
new_total = total + num
total = new_total
return total
# More space-efficient
def sum_array_efficient(arr):
total = 0
for num in arr:
total += num
return total
3. Use Generators or Iterators
In languages that support them, generators or iterators can help process large datasets without loading everything into memory at once:
// Python generator example
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
# Usage
fib = fibonacci_generator()
for _ in range(10):
print(next(fib))
4. Bit Manipulation
For certain problems, especially those involving sets or flags, bit manipulation can be both time and space efficient:
// C++ example: Check if a number is even
bool isEven(int n) {
return !(n & 1);
}
5. Careful Use of Recursion
While recursion can lead to elegant solutions, it can also consume excessive stack space. Consider tail recursion or iterative solutions for better space efficiency:
// JavaScript: Tail-recursive factorial
function factorial(n, accumulator = 1) {
if (n <= 1) return accumulator;
return factorial(n - 1, n * accumulator);
}
Balancing Time and Space Efficiency
Often, there’s a trade-off between time and space efficiency. Here are some guidelines for balancing the two:
1. Understand the Problem Constraints
Before optimizing, consider:
- What are the input size limits?
- Are there specific time or space constraints mentioned?
- Is the problem more sensitive to time or space complexity?
2. Analyze the Worst-Case Scenario
Always consider the worst-case time and space complexity of your solution. This is typically what interviewers and coding challenges focus on.
3. Start with a Working Solution
Begin by implementing a solution that correctly solves the problem, even if it’s not the most efficient. Then, optimize step by step:
- Identify bottlenecks in your current solution.
- Consider alternative algorithms or data structures.
- Implement optimizations and measure the impact.
- Repeat until you reach a satisfactory balance of time and space efficiency.
4. Use Profiling Tools
Many programming environments offer profiling tools that can help identify performance bottlenecks in your code. Familiarize yourself with these tools for your preferred language.
5. Consider the Scale
For small inputs, a less efficient algorithm might be acceptable if it’s simpler to implement and maintain. However, as the scale increases, efficiency becomes more critical.
Common Pitfalls to Avoid
When focusing on efficiency, be wary of these common mistakes:
1. Premature Optimization
Don’t optimize before you have a working solution or before you’ve identified actual bottlenecks. As Donald Knuth famously said, “Premature optimization is the root of all evil.”
2. Sacrificing Readability
While pursuing efficiency, don’t make your code overly complex or hard to read. Clear, maintainable code is often more valuable than marginal performance gains.
3. Ignoring Built-in Functions
Don’t reinvent the wheel. Many languages have highly optimized built-in functions for common operations. Use them unless you have a compelling reason not to.
4. Overcomplicating Simple Problems
Not every problem requires an complex algorithm. Sometimes, a straightforward approach is both efficient and sufficient.
5. Neglecting Edge Cases
In the pursuit of efficiency, don’t forget to handle edge cases and ensure your solution works correctly for all inputs.
Practical Examples
Let’s look at a few practical examples of optimizing for time and space efficiency:
Example 1: Two Sum Problem
Given an array of integers and a target sum, find two numbers in the array that add up to the target.
Naive approach (O(n²) time, O(1) space):
def two_sum_naive(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
Optimized approach (O(n) time, O(n) space):
def two_sum_optimized(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
Example 2: Fibonacci Sequence
Calculate the nth Fibonacci number.
Recursive approach (O(2â¿) time, O(n) space due to call stack):
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
Dynamic Programming approach (O(n) time, O(n) space):
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Space-optimized approach (O(n) time, O(1) space):
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
Conclusion
Prioritizing time and space efficiency in coding challenges is a crucial skill for any programmer, especially those aiming for positions at top tech companies. By understanding the importance of efficiency, mastering various optimization techniques, and practicing with real-world problems, you can significantly improve your problem-solving abilities and coding prowess.
Remember, the goal is not just to solve problems, but to solve them in the most optimal way possible. This often requires a delicate balance between time and space efficiency, as well as consideration for code readability and maintainability.
As you continue your journey in competitive programming and prepare for technical interviews, keep these strategies in mind. Practice regularly, analyze your solutions critically, and always strive for improvement. With time and dedication, you’ll develop the intuition to quickly identify efficient approaches to even the most challenging coding problems.
Happy coding, and may your algorithms always run in O(1) time!