How to Interpret Your Dreams Using JavaScript Syntax
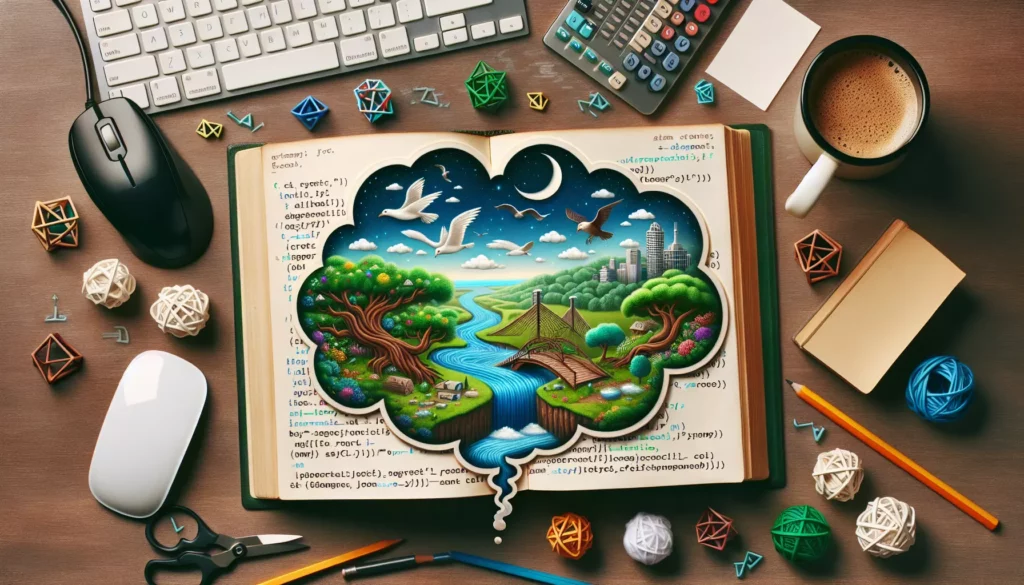
Have you ever woken up from a vivid dream, wondering what it all meant? What if I told you that you could use your JavaScript skills to decode the hidden messages in your subconscious? In this unique exploration, we’ll dive into the world of dream interpretation using the familiar syntax of JavaScript. Whether you’re a coding newbie or a seasoned developer, this guide will help you unlock the secrets of your dreams while honing your programming skills.
The Connection Between Dreams and Code
At first glance, dreams and JavaScript might seem like two entirely different realms. However, both involve complex systems of symbols and logic that, when understood, can reveal deeper meanings. Just as JavaScript uses syntax to create functionality in web applications, our dreams use symbols and scenarios to process our thoughts and emotions.
In the world of AlgoCademy, where we focus on developing coding skills and algorithmic thinking, this unconventional approach to dream interpretation can serve as a fun and insightful way to practice your JavaScript while gaining personal insights.
Setting Up Your Dream Interpretation Environment
Before we dive into interpreting dreams with JavaScript, let’s set up our coding environment. We’ll create a simple JavaScript object to store our dream symbols and their meanings:
const dreamDictionary = {
flying: "freedom, success, or overcoming obstacles",
falling: "insecurity, loss of control, or failure",
teeth: "anxiety, transition, or personal power",
water: "emotions, the unconscious mind, or cleansing",
naked: "vulnerability, authenticity, or fear of exposure"
};
This object will serve as our basic dream dictionary. Feel free to expand it with more symbols and meanings as you explore your dreams.
Creating a Dream Interpreter Function
Now, let’s create a function that will help us interpret our dreams using this dictionary:
function interpretDream(dream) {
const words = dream.toLowerCase().split(/\s+/);
const interpretation = [];
for (const word of words) {
if (dreamDictionary.hasOwnProperty(word)) {
interpretation.push(`${word}: ${dreamDictionary[word]}`);
}
}
return interpretation.length > 0
? interpretation.join("\n")
: "No known symbols found in your dream.";
}
This function takes a dream description as input, splits it into individual words, and checks each word against our dream dictionary. If a match is found, it adds the interpretation to an array, which is then joined into a string for output.
Using the Dream Interpreter
Let’s try out our dream interpreter with a sample dream:
const myDream = "I was flying over a lake, but suddenly my teeth started falling out.";
console.log(interpretDream(myDream));
This might output:
flying: freedom, success, or overcoming obstacles
falling: insecurity, loss of control, or failure
teeth: anxiety, transition, or personal power
water: emotions, the unconscious mind, or cleansing
Expanding the Dream Interpreter
Our basic interpreter is a good start, but dreams are often more complex. Let’s enhance our interpreter to handle more nuanced scenarios:
1. Adding Context Sensitivity
Dreams often have context that can change the meaning of symbols. Let’s update our dreamDictionary
to include context-sensitive interpretations:
const dreamDictionary = {
flying: {
default: "freedom, success, or overcoming obstacles",
context: {
"difficulty flying": "struggling with a challenge",
"flying too high": "unrealistic goals or fear of success"
}
},
falling: {
default: "insecurity, loss of control, or failure",
context: {
"falling into water": "emotional overwhelm",
"falling and landing safely": "overcoming fears"
}
}
// ... add more symbols with context
};
2. Updating the Interpreter Function
Now let’s update our interpretDream
function to handle this new structure:
function interpretDream(dream) {
const dreamLower = dream.toLowerCase();
const interpretation = [];
for (const [symbol, meanings] of Object.entries(dreamDictionary)) {
if (dreamLower.includes(symbol)) {
let meaning = meanings.default;
for (const [context, contextMeaning] of Object.entries(meanings.context || {})) {
if (dreamLower.includes(context)) {
meaning = contextMeaning;
break;
}
}
interpretation.push(`${symbol}: ${meaning}`);
}
}
return interpretation.length > 0
? interpretation.join("\n")
: "No known symbols found in your dream.";
}
3. Testing the Enhanced Interpreter
Let’s test our enhanced interpreter with a more detailed dream:
const detailedDream = "I was flying over a beautiful landscape, but I was having difficulty flying. Then I started falling, but I landed safely in a lake.";
console.log(interpretDream(detailedDream));
This might output:
flying: struggling with a challenge
falling: overcoming fears
water: emotions, the unconscious mind, or cleansing
Adding Emotional Analysis
Dreams often carry strong emotions. Let’s add an emotional analysis feature to our interpreter:
const emotions = {
positive: ["happy", "excited", "peaceful", "joyful"],
negative: ["scared", "anxious", "angry", "sad"],
neutral: ["confused", "surprised", "curious"]
};
function analyzeEmotion(dream) {
const dreamWords = dream.toLowerCase().split(/\s+/);
const emotionCounts = { positive: 0, negative: 0, neutral: 0 };
for (const word of dreamWords) {
for (const [emotion, words] of Object.entries(emotions)) {
if (words.includes(word)) {
emotionCounts[emotion]++;
}
}
}
const dominantEmotion = Object.entries(emotionCounts).reduce((a, b) => a[1] > b[1] ? a : b)[0];
return `The dominant emotion in your dream appears to be ${dominantEmotion}.`;
}
Now we can add emotional analysis to our dream interpretation:
function analyzeDream(dream) {
return `
Symbol Interpretation:
${interpretDream(dream)}
Emotional Analysis:
${analyzeEmotion(dream)}
`;
}
const emotionalDream = "I was flying happily over a peaceful meadow, but then I became scared when I saw a storm approaching.";
console.log(analyzeDream(emotionalDream));
Creating a Dream Journal Class
To take our dream interpretation to the next level, let’s create a DreamJournal
class to store and analyze multiple dreams over time:
class DreamJournal {
constructor() {
this.dreams = [];
}
addDream(date, description) {
this.dreams.push({ date, description });
}
interpretAllDreams() {
return this.dreams.map(dream => ({
date: dream.date,
description: dream.description,
interpretation: analyzeDream(dream.description)
}));
}
findCommonSymbols() {
const symbolCounts = {};
this.dreams.forEach(dream => {
const symbols = Object.keys(dreamDictionary).filter(symbol =>
dream.description.toLowerCase().includes(symbol)
);
symbols.forEach(symbol => {
symbolCounts[symbol] = (symbolCounts[symbol] || 0) + 1;
});
});
return Object.entries(symbolCounts)
.sort((a, b) => b[1] - a[1])
.slice(0, 5)
.map(([symbol, count]) => `${symbol}: ${count} times`);
}
}
Now we can use our DreamJournal
class to track and analyze multiple dreams:
const myDreamJournal = new DreamJournal();
myDreamJournal.addDream("2023-05-01", "I was flying over a city at night, feeling exhilarated.");
myDreamJournal.addDream("2023-05-03", "I was swimming in a clear lake, but then my teeth started falling out.");
myDreamJournal.addDream("2023-05-05", "I was naked in a crowded place, feeling very anxious.");
console.log("Dream Interpretations:");
console.log(JSON.stringify(myDreamJournal.interpretAllDreams(), null, 2));
console.log("\nMost Common Symbols:");
console.log(myDreamJournal.findCommonSymbols().join("\n"));
Leveraging AI for Advanced Dream Analysis
As we dive deeper into dream interpretation, we can leverage AI to enhance our analysis. While implementing a full AI model is beyond the scope of this article, we can simulate AI-assisted interpretation using more advanced JavaScript techniques:
const dreamAI = {
analyzeNarrative: function(dream) {
// Simulating AI analysis of dream narrative
const narrativePatterns = [
{ pattern: /chase|run.*away|escape/i, interpretation: "You may be avoiding a problem or responsibility in your waking life." },
{ pattern: /search.*|look.*for/i, interpretation: "You might be seeking answers or direction in your life." },
{ pattern: /transform|change.*shape/i, interpretation: "You could be going through personal growth or life transitions." }
];
for (const { pattern, interpretation } of narrativePatterns) {
if (pattern.test(dream)) {
return interpretation;
}
}
return "No specific narrative pattern detected.";
},
generateInsight: function(dream) {
// Simulating AI-generated insight
const insights = [
"Consider how this dream might reflect your current life situation.",
"Think about the emotions you experienced in the dream and how they relate to your waking life.",
"Reflect on any recurring themes in your dreams and what they might signify.",
"How might this dream be guiding you towards personal growth or change?"
];
return insights[Math.floor(Math.random() * insights.length)];
}
};
function aiEnhancedAnalysis(dream) {
return `
AI Narrative Analysis:
${dreamAI.analyzeNarrative(dream)}
AI-Generated Insight:
${dreamAI.generateInsight(dream)}
`;
}
Now we can incorporate this AI-enhanced analysis into our DreamJournal
class:
class DreamJournal {
// ... previous methods ...
aiAnalyzeDream(date) {
const dream = this.dreams.find(d => d.date === date);
if (!dream) return "Dream not found.";
return `
Dream Date: ${dream.date}
Dream Description: ${dream.description}
${analyzeDream(dream.description)}
${aiEnhancedAnalysis(dream.description)}
`;
}
}
// Example usage:
myDreamJournal.addDream("2023-05-07", "I was being chased through a maze, searching for a hidden door.");
console.log(myDreamJournal.aiAnalyzeDream("2023-05-07"));
Visualizing Dream Patterns
To gain even more insights from our dreams, we can create visualizations of our dream patterns. While we can’t create actual graphics in this text-based environment, we can simulate a text-based visualization:
class DreamJournal {
// ... previous methods ...
visualizeEmotionTrends() {
const emotionTrends = this.dreams.map(dream => {
const dreamWords = dream.description.toLowerCase().split(/\s+/);
const emotionCounts = { positive: 0, negative: 0, neutral: 0 };
for (const word of dreamWords) {
for (const [emotion, words] of Object.entries(emotions)) {
if (words.includes(word)) {
emotionCounts[emotion]++;
}
}
}
const total = Object.values(emotionCounts).reduce((a, b) => a + b, 0);
return {
date: dream.date,
positive: emotionCounts.positive / total,
negative: emotionCounts.negative / total,
neutral: emotionCounts.neutral / total
};
});
return emotionTrends.map(trend => {
const barLength = 20;
const positiveBar = '+'.repeat(Math.round(trend.positive * barLength));
const negativeBar = '-'.repeat(Math.round(trend.negative * barLength));
const neutralBar = '='.repeat(Math.round(trend.neutral * barLength));
return `${trend.date}: ${positiveBar}${negativeBar}${neutralBar}`;
}).join('\n');
}
}
// Example usage:
console.log("Emotion Trends Visualization:");
console.log(myDreamJournal.visualizeEmotionTrends());
Conclusion: Dreaming in Code
By interpreting our dreams using JavaScript syntax, we’ve not only gained insights into our subconscious but also practiced valuable coding skills. This unique approach demonstrates how programming concepts can be applied to various aspects of our lives, even something as abstract as dream interpretation.
As you continue your journey with AlgoCademy, remember that coding is not just about solving technical problems. It’s a versatile tool that can help you understand and interact with the world in new and exciting ways. Whether you’re preparing for technical interviews or exploring the depths of your subconscious, the logical thinking and problem-solving skills you develop through coding will serve you well.
So, the next time you wake up from a vivid dream, why not fire up your JavaScript editor and see what hidden messages your code can reveal? Happy dreaming and coding!
Further Exploration
To continue developing your JavaScript skills and dream interpretation abilities, consider these challenges:
- Expand the
dreamDictionary
with more symbols and context-sensitive interpretations. - Implement a more sophisticated emotion analysis algorithm using natural language processing techniques.
- Create a web interface for your dream journal, allowing users to input dreams and view interpretations.
- Integrate machine learning algorithms to improve dream interpretation accuracy over time.
- Develop a feature to compare dream patterns with external factors like daily activities or moon phases.
Remember, the key to both effective coding and insightful dream interpretation is practice and continuous learning. Keep exploring, keep coding, and keep dreaming!