How to Practice Coding Challenges Consistently: A Comprehensive Guide
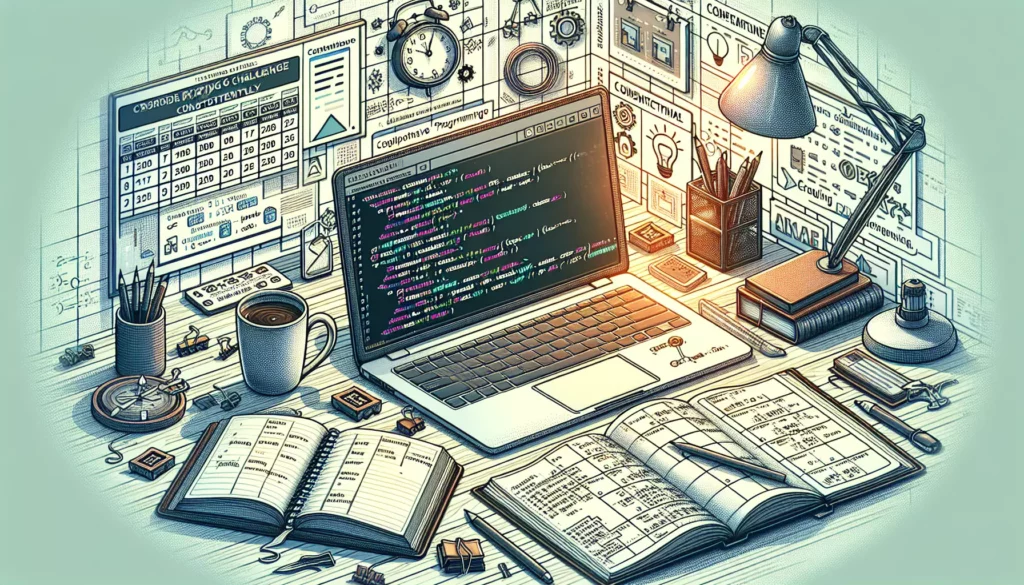
In the ever-evolving world of technology, coding skills have become increasingly valuable. Whether you’re a beginner looking to break into the tech industry or an experienced developer aiming to land a job at a top-tier tech company, consistent practice of coding challenges is crucial. This comprehensive guide will walk you through effective strategies to maintain a regular coding practice routine, helping you sharpen your skills and prepare for technical interviews.
Why Consistent Practice Matters
Before diving into the “how,” let’s briefly discuss why consistent practice is so important:
- Skill Retention: Regular practice helps reinforce concepts and prevents skill decay.
- Problem-Solving Improvement: Exposure to various challenges enhances your ability to approach problems creatively.
- Interview Readiness: Consistent practice mimics the pressure of technical interviews, making you more comfortable and confident.
- Algorithm Mastery: Repeated exposure to different algorithms helps you internalize their patterns and applications.
- Code Efficiency: Regular practice leads to writing cleaner, more efficient code.
1. Set Clear Goals
The first step in establishing a consistent coding practice routine is to set clear, achievable goals. These goals will serve as your roadmap and motivation throughout your journey.
Short-term Goals
- Solve X number of problems per week
- Master a specific algorithm or data structure
- Complete a coding course or tutorial series
Long-term Goals
- Prepare for technical interviews at FAANG companies
- Contribute to open-source projects
- Build a personal project showcasing your skills
Remember to make your goals SMART: Specific, Measurable, Achievable, Relevant, and Time-bound.
2. Create a Structured Learning Plan
With your goals in mind, create a structured learning plan that outlines what you’ll study and when. This plan should cover various aspects of coding and problem-solving:
Core Topics to Include
- Data Structures (Arrays, Linked Lists, Trees, Graphs, etc.)
- Algorithms (Sorting, Searching, Dynamic Programming, etc.)
- Time and Space Complexity Analysis
- Object-Oriented Programming Concepts
- System Design (for more advanced learners)
Platforms like AlgoCademy offer structured learning paths that can help you organize your study plan effectively. Their interactive coding tutorials and AI-powered assistance can guide you through progressively challenging topics, ensuring a well-rounded preparation.
3. Establish a Daily Routine
Consistency is key when it comes to improving your coding skills. Establish a daily routine that fits your schedule and stick to it.
Tips for Creating a Routine
- Set a specific time: Choose a time of day when you’re most alert and least likely to be interrupted.
- Start small: Begin with 30 minutes to an hour per day and gradually increase as you build the habit.
- Use time-blocking: Allocate specific time blocks for different activities (e.g., learning new concepts, solving problems, reviewing solutions).
- Create a dedicated space: Have a designated area for coding practice to minimize distractions.
Remember, consistency trumps intensity. It’s better to practice for 30 minutes every day than to cram for several hours once a week.
4. Utilize Coding Platforms and Resources
Take advantage of the numerous online platforms and resources available for coding practice. These tools offer a wide range of problems, interactive environments, and learning materials to support your journey.
Recommended Platforms
- AlgoCademy: Offers interactive coding tutorials, AI-powered assistance, and a focus on preparing for technical interviews at major tech companies.
- LeetCode: Provides a vast collection of coding challenges, often used by tech companies for interview preparation.
- HackerRank: Offers coding challenges and competitions across various domains.
- CodeSignal: Features a mix of practice problems and real company challenges.
- Project Euler: Focuses on mathematical-oriented programming problems.
Diversify your practice by using multiple platforms. This exposes you to different problem-solving approaches and question styles.
5. Follow the “Learn, Practice, Review” Cycle
To maximize the effectiveness of your coding practice, follow a structured learning cycle:
Learn
- Study new concepts, algorithms, or data structures
- Watch video tutorials or read articles explaining the topic
- Understand the theoretical foundations before implementation
Practice
- Implement the concept in code
- Solve related problems on coding platforms
- Try to solve problems without looking at solutions first
Review
- Analyze your solutions for efficiency and correctness
- Study alternative solutions and optimizations
- Reflect on what you’ve learned and how to apply it in the future
This cycle ensures that you’re not just memorizing solutions but truly understanding and internalizing concepts.
6. Implement Spaced Repetition
Spaced repetition is a learning technique that involves reviewing material at increasing intervals. This method helps reinforce your knowledge and improve long-term retention.
How to Implement Spaced Repetition
- After solving a problem, schedule reviews at increasing intervals (e.g., 1 day, 3 days, 1 week, 2 weeks)
- During reviews, try to solve the problem again without looking at your previous solution
- If you struggle with a problem during review, reset the interval and review it more frequently
- Use spaced repetition software like Anki to manage your review schedule
This technique is particularly effective for retaining complex algorithms and problem-solving patterns.
7. Participate in Coding Competitions
Coding competitions provide an excellent opportunity to test your skills under time pressure and compare your performance with others. Regular participation can significantly improve your problem-solving speed and accuracy.
Benefits of Coding Competitions
- Simulates the pressure of technical interviews
- Exposes you to a wide variety of problem types
- Helps you learn from other participants’ solutions
- Provides a benchmark for your progress
Popular Coding Competition Platforms
- Codeforces
- TopCoder
- AtCoder
- Google Code Jam
- Facebook Hacker Cup
Start with shorter, more frequent contests and gradually work your way up to longer, more challenging competitions.
8. Join a Study Group or Find a Coding Buddy
Collaborative learning can significantly enhance your coding practice. Joining a study group or finding a coding buddy provides motivation, accountability, and diverse perspectives on problem-solving.
Benefits of Collaborative Learning
- Accountability to maintain consistent practice
- Opportunity to explain concepts, reinforcing your own understanding
- Exposure to different problem-solving approaches
- Mock interview practice with peers
Where to Find Coding Partners
- Local coding meetups or hackathons
- Online communities like Reddit’s r/learnprogramming or Discord servers
- University or bootcamp alumni networks
- Platforms like CodeBuddy or Tandem that match coding partners
Regular sessions with your study group or coding buddy can help maintain motivation and provide a support system throughout your learning journey.
9. Implement Mock Interviews
As you progress in your coding skills, start incorporating mock interviews into your practice routine. This helps simulate the actual interview experience and prepares you for the pressure of real technical interviews.
How to Conduct Mock Interviews
- Use platforms like Pramp or interviewing.io for peer-to-peer mock interviews
- Ask a friend or coding buddy to act as the interviewer
- Practice explaining your thought process out loud as you solve problems
- Time yourself to mimic real interview conditions
- Seek feedback on both your technical skills and communication
Regular mock interviews will help you become more comfortable with the interview process and identify areas for improvement.
10. Track Your Progress
Keeping track of your progress is crucial for maintaining motivation and identifying areas that need more focus. Implement a system to monitor your growth over time.
Ways to Track Progress
- Maintain a log of problems solved, categorized by difficulty and topic
- Use GitHub to track your coding projects and contributions
- Keep a learning journal to reflect on challenges and breakthroughs
- Regularly assess your performance in mock interviews or coding competitions
- Use platforms like AlgoCademy that offer progress tracking features
Regularly reviewing your progress can help you adjust your learning plan and celebrate your achievements, keeping you motivated in the long run.
11. Focus on Understanding, Not Just Solving
While solving numerous problems is important, it’s equally crucial to deeply understand the underlying concepts and patterns. Focus on quality over quantity in your practice.
Tips for Deep Understanding
- After solving a problem, try to explain the solution to someone else (or even to yourself)
- Analyze multiple solutions to the same problem and understand the trade-offs
- Implement solutions from scratch without referring to previous code
- Try to derive general problem-solving patterns from specific problems
- Relate new problems to ones you’ve solved before
This approach ensures that you’re building a solid foundation of problem-solving skills, rather than just memorizing solutions.
12. Balance Breadth and Depth
While it’s important to cover a wide range of topics, it’s equally crucial to develop deep expertise in core areas. Strike a balance between breadth and depth in your learning.
Strategies for Balancing
- Rotate through different topics to maintain breadth
- Dedicate focused study periods to dive deep into specific areas
- Revisit fundamental topics regularly to reinforce your foundation
- Challenge yourself with increasingly difficult problems in your areas of expertise
- Use platforms like AlgoCademy that offer both broad coverage and in-depth learning paths
This balanced approach ensures that you have a well-rounded skill set while also developing areas of specialization.
13. Learn from Your Mistakes
Mistakes are an integral part of the learning process. Embrace them as opportunities for growth rather than viewing them as failures.
How to Learn from Mistakes
- Keep a “mistake log” to track common errors and misconceptions
- Analyze why you made a particular mistake and how to avoid it in the future
- Re-attempt problems where you made mistakes after a short break
- Look for patterns in your mistakes to identify areas needing more focus
- Share your mistakes with peers or mentors to get additional insights
By actively learning from your mistakes, you’ll accelerate your progress and develop a growth mindset.
14. Stay Updated with Industry Trends
The tech industry is constantly evolving, and staying updated with current trends can give you an edge in interviews and your career.
Ways to Stay Updated
- Follow tech blogs and news sites (e.g., TechCrunch, Hacker News)
- Participate in online coding communities and forums
- Attend tech conferences or webinars
- Follow influential developers and companies on social media
- Experiment with new technologies and frameworks in your projects
Staying informed about industry trends can help you focus your learning on relevant skills and technologies.
15. Take Care of Your Well-being
Consistent coding practice requires mental stamina and focus. Taking care of your physical and mental well-being is crucial for maintaining long-term consistency.
Tips for Maintaining Well-being
- Get adequate sleep to ensure your mind is sharp for problem-solving
- Take regular breaks during study sessions to avoid burnout
- Exercise regularly to boost cognitive function and reduce stress
- Practice mindfulness or meditation to improve focus and reduce anxiety
- Maintain a balanced diet to support brain function
Remember, a healthy mind and body are essential for effective learning and consistent practice.
Conclusion
Consistent practice of coding challenges is a journey that requires dedication, strategy, and patience. By implementing these strategies and leveraging resources like AlgoCademy, you can develop a robust practice routine that will significantly improve your coding skills and prepare you for technical interviews at top tech companies.
Remember that everyone’s learning journey is unique. Be patient with yourself, celebrate your progress, and stay committed to your goals. With consistent effort and the right approach, you’ll be well on your way to mastering coding challenges and advancing your career in tech.
Happy coding, and may your consistent practice lead you to success in your programming endeavors!