The Role of Dynamic Programming in Solving Complex Problems
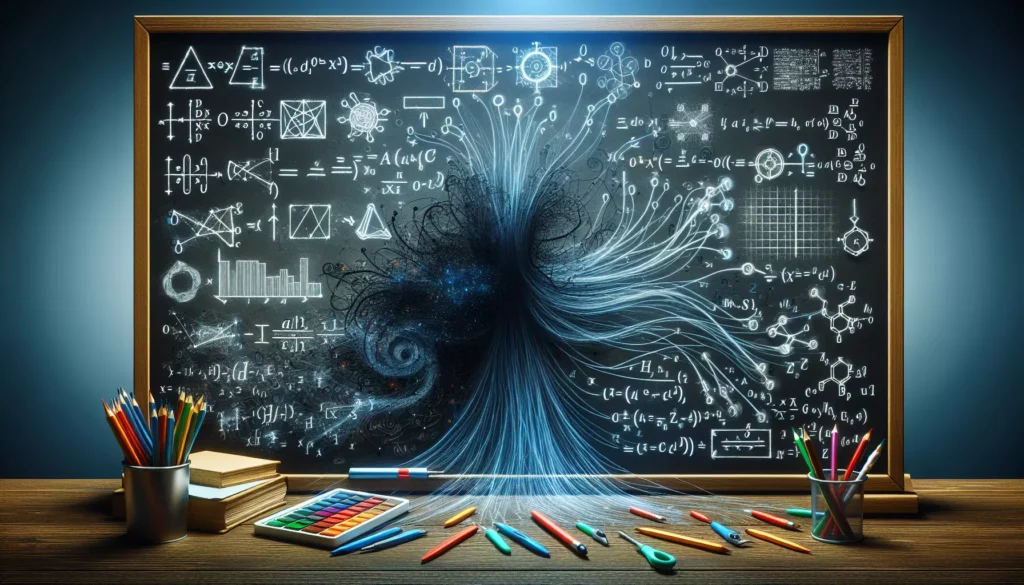
In the ever-evolving world of computer science and software engineering, the ability to solve complex problems efficiently is a highly sought-after skill. Among the various problem-solving techniques, dynamic programming stands out as a powerful method that has revolutionized the way we approach algorithmic challenges. This blog post will delve into the role of dynamic programming in solving complex problems, exploring its principles, applications, and significance in the field of computer science.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is a method for solving optimization problems by making a sequence of decisions. The term “dynamic programming” was coined by Richard Bellman in the 1950s, although the core ideas behind this technique have been around for much longer.
The key idea behind dynamic programming is to store the results of subproblems so that we do not have to re-compute them when needed later. This approach can significantly reduce the time complexity of an algorithm, often transforming an exponential-time solution into a polynomial-time solution.
Core Principles of Dynamic Programming
To understand dynamic programming better, let’s look at its core principles:
1. Optimal Substructure
A problem is said to have optimal substructure if an optimal solution to the problem contains optimal solutions to its subproblems. This property allows us to build the solution to the original problem from the solutions of its subproblems.
2. Overlapping Subproblems
In dynamic programming, the same subproblems are solved multiple times. By storing the results of these subproblems, we can avoid redundant computations and improve the efficiency of our algorithm.
3. Memoization
Memoization is the technique of storing the results of expensive function calls and returning the cached result when the same inputs occur again. This is typically implemented using a hash table or an array.
Approaches to Dynamic Programming
There are two main approaches to implementing dynamic programming:
1. Top-down Approach (Memoization)
In this approach, we start with the original problem and recursively break it down into subproblems. We use memoization to store the results of subproblems as they are computed. This approach is often easier to implement as it follows the natural recursive structure of the problem.
2. Bottom-up Approach (Tabulation)
In this approach, we start by solving the smallest subproblems and use their solutions to build up to larger problems. We typically use a table (array) to store the results of subproblems. This approach is often more efficient as it avoids the overhead of recursive function calls.
Examples of Dynamic Programming Problems
Let’s look at some classic problems that can be efficiently solved using dynamic programming:
1. Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. A naive recursive approach to calculate the nth Fibonacci number would have an exponential time complexity. However, using dynamic programming, we can reduce it to linear time complexity.
Here’s a Python implementation using the bottom-up approach:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(fibonacci(10)) # Output: 55
2. Longest Common Subsequence (LCS)
The Longest Common Subsequence problem is to find the longest subsequence present in given two sequences in the same order. This problem has applications in bioinformatics and version control systems.
Here’s a Python implementation using the bottom-up approach:
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
X = "ABCDGH"
Y = "AEDFHR"
print(f"Length of LCS is {lcs(X, Y)}") # Output: Length of LCS is 3
3. Knapsack Problem
The Knapsack problem is a problem in combinatorial optimization. Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
Here’s a Python implementation of the 0/1 Knapsack problem:
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(knapsack(W, wt, val, n)) # Output: 220
Applications of Dynamic Programming
Dynamic programming has a wide range of applications in various fields:
1. Computer Science and Software Engineering
- String matching algorithms (e.g., edit distance)
- Graph algorithms (e.g., shortest path problems)
- Resource allocation problems
- Optimization of recursive algorithms
2. Bioinformatics
- Sequence alignment
- RNA structure prediction
- Protein folding
3. Operations Research
- Inventory management
- Supply chain optimization
- Portfolio optimization
4. Economics and Finance
- Option pricing models
- Resource allocation in economics
- Dynamic asset allocation strategies
Advantages of Dynamic Programming
Dynamic programming offers several advantages in solving complex problems:
1. Efficiency
By avoiding redundant computations, dynamic programming can significantly reduce the time complexity of algorithms. This is particularly useful for problems with exponential time complexity when solved naively.
2. Optimal Solutions
Dynamic programming guarantees finding the optimal solution to problems with optimal substructure. This is crucial in many optimization problems where we need to find the best possible solution.
3. Handling Large Inputs
Due to its efficiency, dynamic programming allows us to solve problems with much larger inputs compared to brute-force approaches.
4. Versatility
Dynamic programming can be applied to a wide range of problems across various domains, making it a versatile problem-solving technique.
Challenges in Dynamic Programming
While dynamic programming is a powerful technique, it comes with its own set of challenges:
1. Problem Identification
Recognizing whether a problem can be solved using dynamic programming requires practice and experience. Not all problems are suitable for this approach.
2. Formulating the Recurrence Relation
Defining the correct recurrence relation is crucial for the success of a dynamic programming solution. This step often requires careful analysis of the problem structure.
3. Space Complexity
While dynamic programming often improves time complexity, it may increase space complexity due to the storage of subproblem solutions. In some cases, space optimization techniques may be necessary.
4. Debugging and Testing
Dynamic programming solutions can be complex to implement and debug, especially for large problem instances. Thorough testing is essential to ensure correctness.
Best Practices for Dynamic Programming
To effectively use dynamic programming in solving complex problems, consider the following best practices:
1. Identify the Subproblems
Carefully analyze the problem to identify the overlapping subproblems. This is the foundation of any dynamic programming solution.
2. Define the State
Clearly define the state that represents a subproblem. This typically involves identifying the parameters that uniquely define each subproblem.
3. Establish the Recurrence Relation
Formulate the recurrence relation that expresses the solution to a problem in terms of solutions to its subproblems.
4. Determine the Base Cases
Identify and handle the base cases correctly. These are typically the smallest subproblems that can be solved directly.
5. Choose the Right Implementation Approach
Decide between the top-down (memoization) and bottom-up (tabulation) approaches based on the problem characteristics and performance requirements.
6. Optimize Space Usage
If space is a concern, consider optimizing the space usage. In many cases, you can reduce the space complexity by only storing the necessary previous states.
7. Test with Small Inputs
Start by testing your solution with small inputs where you can manually verify the results. This helps in catching errors early in the development process.
Advanced Dynamic Programming Techniques
As you become more comfortable with basic dynamic programming, you can explore advanced techniques:
1. Bitmasking DP
This technique uses bitmasks to represent sets, allowing efficient handling of subset-related problems.
2. Digit DP
Digit DP is used to solve problems involving digit properties, often in number theory problems.
3. Tree DP
This technique applies dynamic programming concepts to tree structures, often used in graph-related problems.
4. Probability DP
Used in problems involving probability calculations, where the state transitions have associated probabilities.
Dynamic Programming in Competitive Programming
Dynamic programming plays a crucial role in competitive programming and coding interviews. Many classic problems in these contexts are solved using DP:
- Longest Increasing Subsequence (LIS)
- Matrix Chain Multiplication
- Coin Change Problem
- Edit Distance
- Palindrome Partitioning
Mastering these problems and understanding their DP solutions can significantly improve your problem-solving skills and performance in coding competitions.
Dynamic Programming in Real-world Applications
Beyond academic and competitive contexts, dynamic programming finds applications in various real-world scenarios:
1. Natural Language Processing
DP is used in various NLP tasks, including speech recognition, machine translation, and text summarization.
2. Computer Graphics
In computer graphics, DP is used for tasks like seam carving for content-aware image resizing.
3. Artificial Intelligence
DP is a fundamental technique in reinforcement learning, used in training agents to make sequences of decisions.
4. Network Routing
DP algorithms like the Bellman-Ford algorithm are used in network routing protocols.
Future Trends in Dynamic Programming
As the field of computer science evolves, so does the application of dynamic programming:
1. Integration with Machine Learning
There’s growing interest in combining DP with machine learning techniques, particularly in areas like reinforcement learning and neural architecture search.
2. Parallel and Distributed DP
With the rise of parallel and distributed computing, there’s ongoing research into adapting DP algorithms to these paradigms for improved performance.
3. Quantum Dynamic Programming
As quantum computing advances, researchers are exploring how DP algorithms can be adapted to quantum systems for potential speedups.
Conclusion
Dynamic programming is a powerful problem-solving technique that has revolutionized the way we approach complex computational problems. Its ability to break down complex problems into manageable subproblems and avoid redundant computations makes it an indispensable tool in a programmer’s arsenal.
From classic algorithmic challenges to cutting-edge applications in AI and bioinformatics, dynamic programming continues to play a crucial role in advancing the field of computer science. As we’ve seen, mastering this technique not only enhances problem-solving skills but also opens doors to tackling a wide range of real-world optimization problems.
As you continue your journey in computer science and software engineering, investing time in understanding and practicing dynamic programming will undoubtedly pay dividends. Whether you’re preparing for technical interviews, participating in coding competitions, or working on complex software projects, the principles and techniques of dynamic programming will serve you well.
Remember, like any skill, proficiency in dynamic programming comes with practice. Start with simple problems, gradually work your way up to more complex ones, and don’t hesitate to revisit and optimize your solutions. With time and effort, you’ll find that dynamic programming becomes an intuitive and powerful tool in your problem-solving toolkit.