How to Identify Patterns in Coding Challenges: A Comprehensive Guide
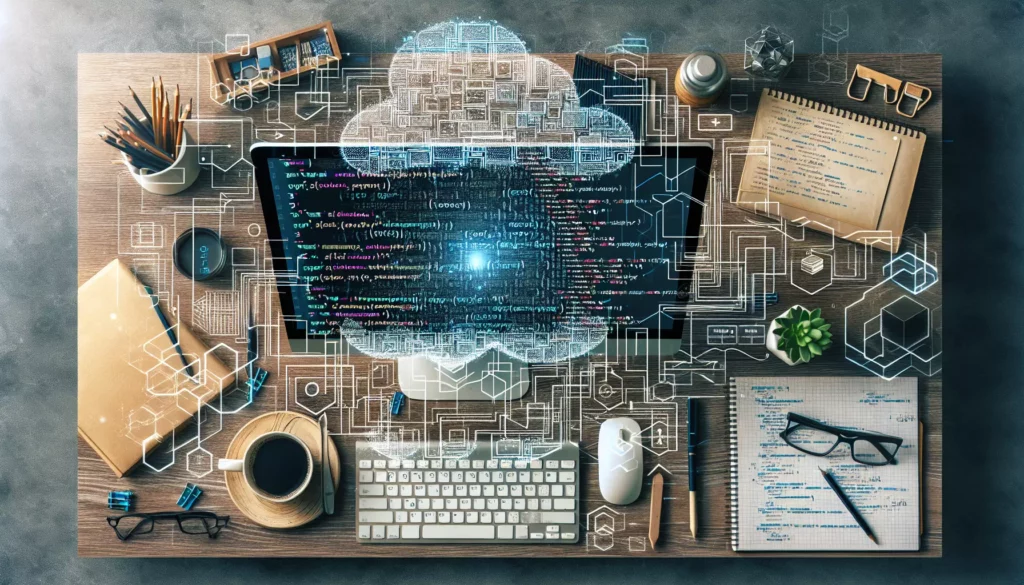
In the world of programming and software development, the ability to identify patterns in coding challenges is a crucial skill that can significantly enhance your problem-solving capabilities. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, recognizing common patterns can help you approach problems more efficiently and develop elegant solutions. In this comprehensive guide, we’ll explore various strategies and techniques to help you identify patterns in coding challenges, ultimately improving your algorithmic thinking and programming skills.
1. Understanding the Importance of Pattern Recognition
Before diving into specific techniques, it’s essential to understand why pattern recognition is so valuable in coding challenges:
- Efficiency: Recognizing patterns allows you to solve problems more quickly by applying known solutions to similar challenges.
- Problem-solving: Patterns help you break down complex problems into manageable components.
- Code reusability: Identifying common patterns enables you to create reusable code structures and algorithms.
- Interview preparation: Many technical interviews, especially at FAANG companies, focus on algorithmic problem-solving, where pattern recognition is crucial.
2. Common Patterns in Coding Challenges
To start identifying patterns, it’s helpful to familiarize yourself with some of the most common patterns encountered in coding challenges:
2.1. Two-Pointer Technique
The two-pointer technique involves using two pointers to traverse an array or linked list, often moving in opposite directions or at different speeds. This pattern is useful for problems involving searching, reversing, or finding pairs in sorted arrays.
Example problem: Find a pair of numbers in a sorted array that sum up to a target value.
def find_pair(arr, target):
left = 0
right = len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None
2.2. Sliding Window
The sliding window pattern is used to perform operations on a specific window of elements in an array or string, which expands or contracts depending on certain conditions. This pattern is often used for problems involving subarrays or substring calculations.
Example problem: Find the longest substring with at most k distinct characters.
from collections import defaultdict
def longest_substring_with_k_distinct(s, k):
char_count = defaultdict(int)
max_length = 0
left = 0
for right in range(len(s)):
char_count[s[right]] += 1
while len(char_count) > k:
char_count[s[left]] -= 1
if char_count[s[left]] == 0:
del char_count[s[left]]
left += 1
max_length = max(max_length, right - left + 1)
return max_length
2.3. Fast and Slow Pointers
This pattern uses two pointers moving at different speeds through a sequence, often used to detect cycles in linked lists or arrays.
Example problem: Detect a cycle in a linked list.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
2.4. Merge Intervals
This pattern deals with problems involving overlapping intervals, such as merging or finding intersections between intervals.
Example problem: Merge overlapping intervals.
def merge_intervals(intervals):
if not intervals:
return []
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
2.5. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used for optimization problems and involves storing intermediate results to avoid redundant calculations.
Example problem: Calculate the nth Fibonacci number.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
3. Strategies for Identifying Patterns
Now that we’ve covered some common patterns, let’s explore strategies to help you identify patterns in coding challenges:
3.1. Analyze the Problem Statement
Carefully read and analyze the problem statement. Look for key phrases or requirements that might hint at a specific pattern:
- “Find a subarray” or “Find a substring” often suggests a sliding window approach.
- “Detect a cycle” might indicate the use of fast and slow pointers.
- “Merge overlapping intervals” clearly points to the merge intervals pattern.
- “Optimize” or “Find the maximum/minimum” could suggest dynamic programming.
3.2. Consider the Input Data Structure
The input data structure can provide clues about which pattern might be applicable:
- Sorted arrays often work well with the two-pointer technique.
- Linked lists are frequently used with fast and slow pointers.
- Trees and graphs might suggest recursive or depth-first search approaches.
- Strings often involve sliding window or dynamic programming techniques.
3.3. Visualize the Problem
Try to visualize the problem or draw diagrams to help identify patterns:
- Draw arrays as boxes and use arrows to represent pointers.
- Sketch intervals on a number line to visualize overlaps.
- Create tree or graph diagrams to understand relationships between elements.
3.4. Start with Brute Force
Begin by solving the problem using a brute force approach. This can help you understand the problem better and potentially reveal patterns or optimizations:
- Identify repeated calculations or operations that could be optimized.
- Look for opportunities to reduce time complexity by eliminating nested loops.
- Consider how you can reuse previously computed results.
3.5. Practice and Build a Pattern Catalog
The more problems you solve, the better you’ll become at recognizing patterns. Build your own catalog of patterns and problems:
- Keep notes on problems you’ve solved and the patterns used.
- Categorize problems based on their underlying patterns.
- Review your catalog regularly to reinforce your pattern recognition skills.
4. Advanced Pattern Recognition Techniques
As you become more experienced with pattern recognition, you can employ more advanced techniques:
4.1. Combine Multiple Patterns
Some complex problems may require combining multiple patterns. For example, you might use both the sliding window technique and dynamic programming in a single solution.
4.2. Adapt and Modify Patterns
Learn to adapt known patterns to fit new problem requirements. This flexibility allows you to tackle a wider range of challenges.
4.3. Recognize Anti-Patterns
Identify common pitfalls or inefficient approaches (anti-patterns) to avoid them in your solutions.
4.4. Analyze Time and Space Complexity
Understanding the time and space complexity of different patterns can help you choose the most appropriate approach for a given problem.
5. Applying Pattern Recognition in Technical Interviews
Pattern recognition is particularly valuable during technical interviews, especially at major tech companies. Here’s how to leverage this skill effectively:
5.1. Communicate Your Thought Process
When you recognize a pattern, explain your reasoning to the interviewer. This demonstrates your problem-solving approach and analytical skills.
5.2. Consider Multiple Approaches
Even if you recognize a pattern quickly, consider alternative approaches. This shows your ability to think critically and evaluate different solutions.
5.3. Optimize Your Solution
After implementing a solution based on a recognized pattern, discuss potential optimizations or trade-offs with the interviewer.
5.4. Practice with Mock Interviews
Conduct mock interviews to practice applying your pattern recognition skills under time pressure and with verbal communication.
6. Tools and Resources for Improving Pattern Recognition
To enhance your pattern recognition skills, consider using the following tools and resources:
6.1. Online Coding Platforms
Platforms like LeetCode, HackerRank, and CodeSignal offer a wide variety of coding challenges categorized by patterns and difficulty levels.
6.2. Algorithm Visualization Tools
Tools like VisuAlgo and Algorithm Visualizer can help you understand how different algorithms and patterns work through interactive visualizations.
6.3. Books on Algorithms and Data Structures
Classic books like “Introduction to Algorithms” by Cormen et al. and “Cracking the Coding Interview” by Gayle Laakmann McDowell provide in-depth coverage of common patterns and problem-solving techniques.
6.4. Online Courses and Tutorials
Platforms like Coursera, edX, and Udacity offer courses on algorithms and data structures that can help you develop your pattern recognition skills.
7. Common Mistakes to Avoid in Pattern Recognition
As you work on improving your pattern recognition skills, be aware of these common pitfalls:
7.1. Overreliance on Memorization
While it’s important to know common patterns, avoid simply memorizing solutions. Focus on understanding the underlying principles and reasoning behind each pattern.
7.2. Forcing Patterns
Don’t try to force a familiar pattern onto a problem where it doesn’t fit. Be open to new approaches or combinations of patterns.
7.3. Neglecting Edge Cases
When applying a recognized pattern, don’t forget to consider edge cases that might require special handling.
7.4. Ignoring Problem Constraints
Always consider the specific constraints of the problem, such as time or space complexity requirements, which may influence your choice of pattern or implementation.
Conclusion
Identifying patterns in coding challenges is a valuable skill that can significantly improve your problem-solving abilities and prepare you for technical interviews at top tech companies. By familiarizing yourself with common patterns, practicing regularly, and developing effective strategies for pattern recognition, you’ll be better equipped to tackle a wide range of coding challenges.
Remember that pattern recognition is not about memorizing solutions but about developing a deeper understanding of problem-solving techniques. As you continue to practice and refine your skills, you’ll find that your ability to recognize and apply patterns becomes more intuitive, allowing you to approach even the most complex coding challenges with confidence.
Keep challenging yourself with diverse problems, stay curious about new patterns and techniques, and don’t be afraid to adapt and combine patterns in creative ways. With dedication and practice, you’ll be well on your way to mastering the art of pattern recognition in coding challenges and excelling in your programming career.