The Unexpected Parallels Between Debugging and Crime Scene Investigation
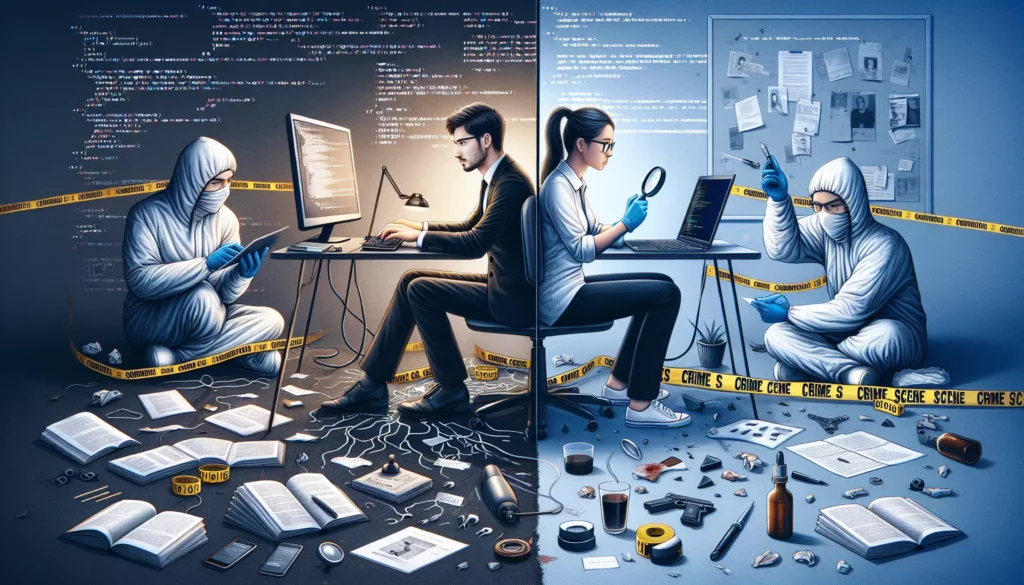
In the world of software development, debugging is an essential skill that every programmer must master. It’s the process of identifying, analyzing, and fixing errors or “bugs” in code. On the surface, this might seem like a purely technical endeavor, far removed from the dramatic world of crime scene investigation (CSI) we see on television. However, upon closer examination, these two fields share surprising similarities in their approaches, methodologies, and the mindset required to excel.
In this article, we’ll explore the fascinating parallels between debugging and crime scene investigation, uncovering how the skills and techniques used in both fields can inform and enhance each other. Whether you’re a seasoned developer looking to sharpen your debugging skills or a curious beginner eager to understand the detective-like nature of programming, this exploration will provide valuable insights and perhaps a new perspective on the art of troubleshooting code.
1. The Crime Scene vs. The Bug Report
Just as a detective arrives at a crime scene, a programmer often begins their debugging journey with a bug report. Both scenarios present an initial set of clues and information that serve as the starting point for the investigation.
The Crime Scene:
- Physical evidence
- Witness statements
- Preliminary observations
The Bug Report:
- Error messages
- User-reported symptoms
- Log files
In both cases, the initial information can be incomplete, misleading, or even contradictory. The skill lies in knowing how to interpret these initial clues and where to look for additional evidence.
2. Gathering Evidence
Once the initial assessment is made, both crime scene investigators and debuggers move on to the crucial phase of gathering evidence. This step is all about collecting as much relevant information as possible to build a comprehensive understanding of the situation.
CSI Techniques:
- Fingerprint collection
- DNA sampling
- Photographing the scene
- Interviewing witnesses
Debugging Techniques:
- Log analysis
- Stack trace examination
- Code review
- Reproducing the bug
In debugging, tools like debuggers, profilers, and logging frameworks serve as the “forensic tools” of the programming world. Just as CSI investigators use specialized equipment to uncover hidden evidence, programmers use these tools to reveal the inner workings of their code and identify anomalies.
// Example of adding logging for debugging
function processData(data) {
console.log("Entering processData with data:", data);
// ... processing logic ...
if (someCondition) {
console.log("Condition met, executing branch A");
// ... branch A logic ...
} else {
console.log("Condition not met, executing branch B");
// ... branch B logic ...
}
console.log("Exiting processData");
}
3. Forming Hypotheses
As evidence is collected, both investigators and debuggers begin to form hypotheses about what might have occurred. This is where experience, intuition, and analytical thinking come into play.
CSI Hypothesis Formation:
- Considering possible scenarios based on evidence
- Evaluating the likelihood of different theories
- Identifying potential suspects or causes
Debugging Hypothesis Formation:
- Identifying potential causes of the bug
- Considering different code paths that could lead to the error
- Evaluating recent changes or updates that might be responsible
In both fields, the ability to think creatively and consider multiple possibilities is crucial. Sometimes, the most obvious explanation is not the correct one, and it takes a keen eye and open mind to uncover the truth.
4. Testing and Experimentation
Once hypotheses are formed, the next step is to test them. This is where the scientific method comes into play in both crime scene investigation and debugging.
CSI Testing:
- Recreating crime scenarios
- Conducting forensic tests on evidence
- Verifying alibis and timelines
Debugging Testing:
- Writing and running unit tests
- Using breakpoints and step-through debugging
- Isolating components for testing
In programming, unit tests serve as a powerful tool for verifying hypotheses and ensuring that fixes don’t introduce new problems. Here’s an example of a simple unit test in JavaScript:
// Function to be tested
function add(a, b) {
return a + b;
}
// Unit test
test("add function correctly adds two numbers", () => {
expect(add(2, 3)).toBe(5);
expect(add(-1, 1)).toBe(0);
expect(add(0, 0)).toBe(0);
});
5. Reconstructing the Scene
An essential aspect of both CSI and debugging is the ability to reconstruct the sequence of events that led to the crime or bug. This reconstruction helps in understanding the root cause and preventing similar issues in the future.
CSI Reconstruction:
- Creating timelines of events
- Using forensic evidence to piece together what happened
- Identifying the series of actions that led to the crime
Debugging Reconstruction:
- Tracing the execution path that led to the bug
- Identifying the specific conditions that trigger the error
- Understanding the interaction between different parts of the code
In debugging, tools like stack traces and call hierarchies help in reconstructing the sequence of function calls that led to an error. Here’s an example of how a stack trace might look:
Error: Division by zero
at divide (calculator.js:15)
at calculateResult (calculator.js:25)
at processUserInput (app.js:42)
at handleSubmit (app.js:78)
at HTMLFormElement.onsubmit (index.html:22)
This stack trace helps the developer understand the sequence of function calls that led to the division by zero error, much like how a CSI investigator might reconstruct the sequence of events leading up to a crime.
6. Collaboration and Expertise
Both crime scene investigation and debugging often require collaboration and the input of various experts. No single individual typically possesses all the knowledge and skills needed to solve complex cases or bugs.
CSI Collaboration:
- Forensic specialists (e.g., ballistics experts, toxicologists)
- Profilers
- Legal experts
Debugging Collaboration:
- Backend developers
- Frontend developers
- Database administrators
- DevOps engineers
In the world of software development, tools like version control systems (e.g., Git) and issue trackers facilitate collaboration and knowledge sharing among team members. Here’s an example of how a Git commit message might document a collaborative debugging effort:
commit a1b2c3d4e5f6g7h8i9j0k1l2m3n4o5p6q7r8s9t0
Author: Jane Doe <jane.doe@example.com>
Date: Mon Apr 10 14:30:00 2023 -0700
Fix race condition in user authentication
- Identified intermittent login failures under high load
- Collaborated with DB team to optimize query performance
- Implemented thread-safe session handling
- Added unit tests to verify fix
Closes #1234
7. The Importance of Documentation
Thorough documentation is crucial in both CSI and debugging. It serves multiple purposes: recording findings, communicating with team members, and providing a reference for future investigations or debugging sessions.
CSI Documentation:
- Crime scene reports
- Evidence logs
- Forensic analysis results
- Witness statements
Debugging Documentation:
- Bug reports
- Code comments
- Commit messages
- Technical documentation
In programming, clear and concise documentation can save countless hours when revisiting code or when new team members join the project. Here’s an example of well-documented code:
/**
* Calculates the factorial of a given number.
*
* @param {number} n - The number to calculate the factorial for.
* @returns {number} The factorial of n.
* @throws {Error} If n is negative or not an integer.
*/
function factorial(n) {
if (n < 0 || !Number.isInteger(n)) {
throw new Error("Input must be a non-negative integer");
}
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
8. Continuous Learning and Improvement
Both fields require a commitment to continuous learning and improvement. As new technologies and techniques emerge, professionals in both CSI and debugging must stay updated to remain effective.
CSI Continuous Learning:
- Staying updated on new forensic technologies
- Learning about evolving criminal techniques
- Studying past cases and their resolutions
Debugging Continuous Learning:
- Keeping up with new programming languages and frameworks
- Learning about emerging security threats and vulnerabilities
- Studying common bug patterns and their solutions
In the world of programming, platforms like AlgoCademy play a crucial role in this continuous learning process. They provide interactive coding tutorials, resources for learners, and tools to help individuals progress from beginner-level coding to preparing for technical interviews at major tech companies.
9. The Role of Intuition and Experience
While both CSI and debugging rely heavily on scientific methods and systematic approaches, the role of intuition and experience cannot be understated. Seasoned professionals in both fields often develop a “sixth sense” that allows them to quickly identify promising leads or potential problem areas.
CSI Intuition:
- Recognizing patterns from previous cases
- Sensing when a witness might be withholding information
- Identifying seemingly insignificant details that turn out to be crucial
Debugging Intuition:
- Recognizing common bug patterns
- Sensing when a particular part of the code is likely to be problematic
- Quickly identifying potential side effects of code changes
This intuition, often referred to as “developer instinct” in the programming world, is honed through years of experience and exposure to a wide variety of codebases and bug scenarios. It’s one of the reasons why senior developers can often spot issues or suggest solutions much faster than their less experienced counterparts.
10. The Satisfaction of Solving the Puzzle
Perhaps one of the most striking similarities between CSI and debugging is the immense satisfaction that comes from solving a complex puzzle. Whether it’s cracking a difficult case or fixing an elusive bug, both fields offer a unique sense of accomplishment when the mystery is finally unraveled.
CSI Satisfaction:
- Bringing closure to victims and their families
- Ensuring justice is served
- Contributing to public safety
Debugging Satisfaction:
- Improving software quality and user experience
- Preventing potential security breaches or data loss
- Gaining deeper understanding of complex systems
This sense of satisfaction is often what drives professionals in both fields to continue honing their skills and tackling increasingly complex challenges. It’s the thrill of the hunt, the joy of discovery, and the pride in a job well done that makes both CSI and debugging such rewarding pursuits.
Conclusion: The Detective Mindset in Programming
As we’ve explored throughout this article, the parallels between debugging and crime scene investigation are numerous and significant. Both fields require a unique blend of analytical thinking, attention to detail, perseverance, and creativity. They demand professionals who can think both logically and outside the box, who can follow systematic processes while also trusting their instincts.
For programmers, embracing this “detective mindset” can be incredibly beneficial. It encourages a more holistic approach to problem-solving, where each bug is treated as a mystery to be unraveled rather than just a technical issue to be fixed. This perspective can lead to more thorough investigations, more robust solutions, and ultimately, better software.
Moreover, the skills developed through debugging – such as analytical thinking, hypothesis formation, and methodical investigation – are highly transferable. They can enhance a programmer’s ability to design systems, anticipate potential issues, and even communicate complex technical concepts to non-technical stakeholders.
As you continue your journey in software development, whether you’re just starting out or you’re a seasoned professional, consider approaching your next debugging session with the mindset of a crime scene investigator. Treat each bug as a case to be solved, each error message as a clue, and each fix as a step towards justice in your codebase. You might find that this perspective not only makes debugging more engaging but also helps you develop into a more well-rounded and effective programmer.
Remember, in the world of coding, every developer is a detective, and every bug is a mystery waiting to be solved. Happy sleuthing!