How to Use Algorithms to Solve Coding Challenges Efficiently
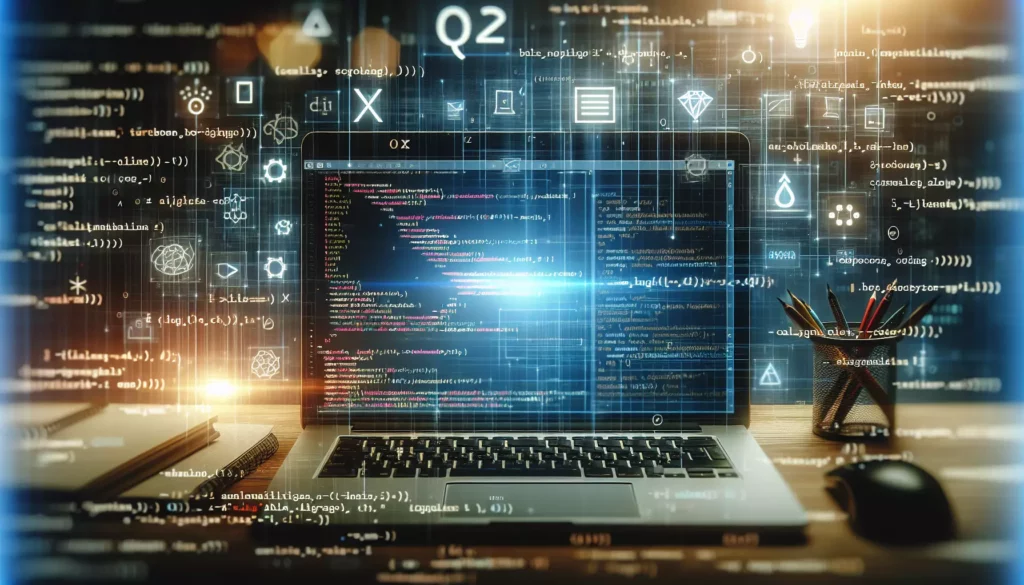
In the world of programming and software development, the ability to solve coding challenges efficiently is a crucial skill. Whether you’re preparing for technical interviews at top tech companies or simply aiming to improve your problem-solving abilities, mastering algorithms is key. This comprehensive guide will walk you through the process of using algorithms to tackle coding challenges effectively, providing you with the tools and strategies you need to excel in your coding journey.
Understanding the Importance of Algorithms in Coding
Before diving into the specifics of using algorithms to solve coding challenges, it’s essential to grasp why algorithms are so important in the first place.
What Are Algorithms?
An algorithm is a step-by-step procedure or formula for solving a problem. In the context of programming, algorithms are the foundation of efficient code. They provide a systematic approach to problem-solving, allowing developers to break down complex tasks into manageable steps.
Why Are Algorithms Crucial?
- Efficiency: Well-designed algorithms can significantly improve the performance of your code, reducing time and space complexity.
- Scalability: Efficient algorithms ensure that your solutions can handle larger inputs and datasets without breaking down.
- Problem-solving: Understanding algorithms enhances your ability to approach and solve a wide range of programming challenges.
- Interview preparation: Many technical interviews, especially at major tech companies, focus heavily on algorithmic problem-solving skills.
The Process of Using Algorithms to Solve Coding Challenges
Now that we understand the importance of algorithms, let’s break down the process of using them to solve coding challenges efficiently.
1. Understand the Problem
The first step in solving any coding challenge is to thoroughly understand the problem at hand. This involves:
- Reading the problem statement carefully
- Identifying the input and expected output
- Recognizing any constraints or special conditions
- Asking clarifying questions if necessary
Take your time with this step, as a clear understanding of the problem is crucial for selecting the appropriate algorithm and developing an effective solution.
2. Analyze the Problem and Identify Patterns
Once you understand the problem, the next step is to analyze it and look for patterns or similarities to known problem types. This step helps you determine which algorithmic approach might be most suitable.
Ask yourself questions like:
- Is this a sorting problem?
- Does it involve searching through data?
- Is it a graph-related problem?
- Does it require dynamic programming?
- Can it be solved using a greedy approach?
Identifying the problem type will guide you towards the most appropriate algorithmic solution.
3. Choose the Right Algorithm
Based on your analysis, select an algorithm that fits the problem. Some common types of algorithms include:
- Sorting algorithms (e.g., Quicksort, Mergesort)
- Searching algorithms (e.g., Binary Search, Depth-First Search)
- Graph algorithms (e.g., Dijkstra’s algorithm, Breadth-First Search)
- Dynamic Programming
- Greedy algorithms
- Divide and Conquer algorithms
Your choice of algorithm will depend on factors such as the problem type, input size, and any time or space constraints specified in the problem statement.
4. Design the Solution
With an algorithm in mind, start designing your solution. This step involves:
- Breaking down the algorithm into clear, logical steps
- Considering edge cases and potential pitfalls
- Planning your data structures (e.g., arrays, hash tables, trees)
- Sketching out a high-level pseudocode or flowchart
Take the time to think through your approach before diving into coding. A well-thought-out design can save you time and frustration later.
5. Implement the Solution
Now it’s time to translate your design into actual code. As you implement your solution:
- Write clean, readable code
- Use meaningful variable and function names
- Add comments to explain complex parts of your logic
- Follow best practices and coding standards for your chosen programming language
Here’s a simple example of implementing a binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
6. Test and Debug
After implementing your solution, it’s crucial to test it thoroughly:
- Start with small, simple test cases
- Gradually move to more complex and edge cases
- Use the provided test cases (if any) and create your own
- Debug any issues you encounter, using print statements or a debugger as needed
Remember, a solution that works for some inputs but fails for others is not a complete solution. Ensure your implementation handles all possible scenarios.
7. Analyze Time and Space Complexity
An essential part of using algorithms efficiently is understanding and optimizing their time and space complexity. After implementing and testing your solution:
- Analyze the time complexity (how the runtime grows with input size)
- Evaluate the space complexity (how memory usage scales with input size)
- Consider if there are ways to optimize your solution further
Understanding Big O notation is crucial for this step. For example, the binary search algorithm implemented above has a time complexity of O(log n), making it very efficient for large sorted arrays.
8. Refine and Optimize
Based on your analysis, look for opportunities to refine and optimize your solution:
- Can you reduce the time complexity?
- Is there a way to use less memory?
- Are there any redundant operations you can eliminate?
- Can you make the code more readable or maintainable?
Remember, the goal is not just to solve the problem, but to solve it efficiently and elegantly.
Common Algorithmic Techniques for Solving Coding Challenges
To further enhance your ability to use algorithms effectively, familiarize yourself with these common algorithmic techniques:
1. Two Pointer Technique
The two pointer technique involves using two pointers to traverse a data structure, often moving in tandem or in opposite directions. This technique is particularly useful for array and linked list problems.
Example problem: Finding a pair of elements in a sorted array that sum to a target value.
def find_pair_with_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return arr[left], arr[right]
elif current_sum < target:
left += 1
else:
right -= 1
return None # No pair found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11]
result = find_pair_with_sum(sorted_array, 14)
print(f"Pair found: {result}")
2. Sliding Window
The sliding window technique is used to perform operations on a specific window of elements in an array or string. It’s particularly useful for substring or subarray problems.
Example problem: Finding the maximum sum of a subarray of size k.
def max_subarray_sum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
array = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
result = max_subarray_sum(array, k)
print(f"Maximum sum of subarray of size {k}: {result}")
3. Divide and Conquer
The divide and conquer approach involves breaking a problem into smaller subproblems, solving them independently, and then combining the results. This technique is the basis for algorithms like Mergesort and Quicksort.
Example: Implementing Mergesort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = merge_sort(unsorted_array)
print(f"Sorted array: {sorted_array}")
4. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and problems with overlapping subproblems.
Example: Calculating Fibonacci numbers using dynamic programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
n = 10
result = fibonacci(n)
print(f"The {n}th Fibonacci number is: {result}")
5. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, aiming to find a global optimum. While not always guaranteed to find the best overall solution, greedy algorithms can be very efficient for certain problems.
Example: Coin change problem using a greedy approach
def coin_change_greedy(amount, coins):
coins.sort(reverse=True) # Sort coins in descending order
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result if amount == 0 else None
# Example usage
coins = [25, 10, 5, 1] # Quarter, dime, nickel, penny
amount = 67
result = coin_change_greedy(amount, coins)
print(f"Coins needed for {amount} cents: {result}")
Tips for Improving Your Algorithmic Problem-Solving Skills
To become proficient at using algorithms to solve coding challenges, consider the following tips:
1. Practice Regularly
Consistent practice is key to improving your algorithmic problem-solving skills. Set aside time each day or week to work on coding challenges. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide variety of problems to practice with.
2. Study Classic Algorithms and Data Structures
Familiarize yourself with fundamental algorithms and data structures. Understanding these building blocks will help you recognize patterns and choose appropriate solutions more quickly.
3. Analyze Multiple Solutions
For each problem you solve, take the time to explore different approaches. Compare their time and space complexities, and understand the trade-offs between different solutions.
4. Learn from Others
After solving a problem, look at solutions submitted by others. This can expose you to new techniques and more efficient implementations. Participate in coding communities and forums to discuss problems and share insights.
5. Focus on Problem-Solving Patterns
As you practice, try to identify common patterns in problem-solving. Recognizing these patterns will help you approach new problems more effectively.
6. Time Yourself
Practice solving problems under time constraints. This will help you prepare for coding interviews and improve your ability to think and code quickly.
7. Explain Your Solutions
Practice explaining your solutions out loud or in writing. This helps reinforce your understanding and prepares you for technical interviews where you’ll need to communicate your thought process.
8. Review and Reflect
Regularly review problems you’ve solved in the past. Reflect on how you might approach them differently with your current knowledge and skills.
Conclusion
Mastering the use of algorithms to solve coding challenges efficiently is a journey that requires dedication, practice, and continuous learning. By understanding the importance of algorithms, following a structured problem-solving process, and familiarizing yourself with common algorithmic techniques, you’ll be well-equipped to tackle a wide range of coding challenges.
Remember that becoming proficient in algorithmic problem-solving takes time. Be patient with yourself, celebrate your progress, and enjoy the process of becoming a more skilled and efficient programmer. Whether you’re preparing for technical interviews or simply aiming to improve your coding abilities, the skills you develop through algorithmic problem-solving will serve you well throughout your programming career.
Keep practicing, stay curious, and never stop learning. With persistence and the right approach, you’ll be solving complex coding challenges with confidence and efficiency in no time.