How to Turn Your Abandoned GitHub Repositories into a Haunted Code Forest
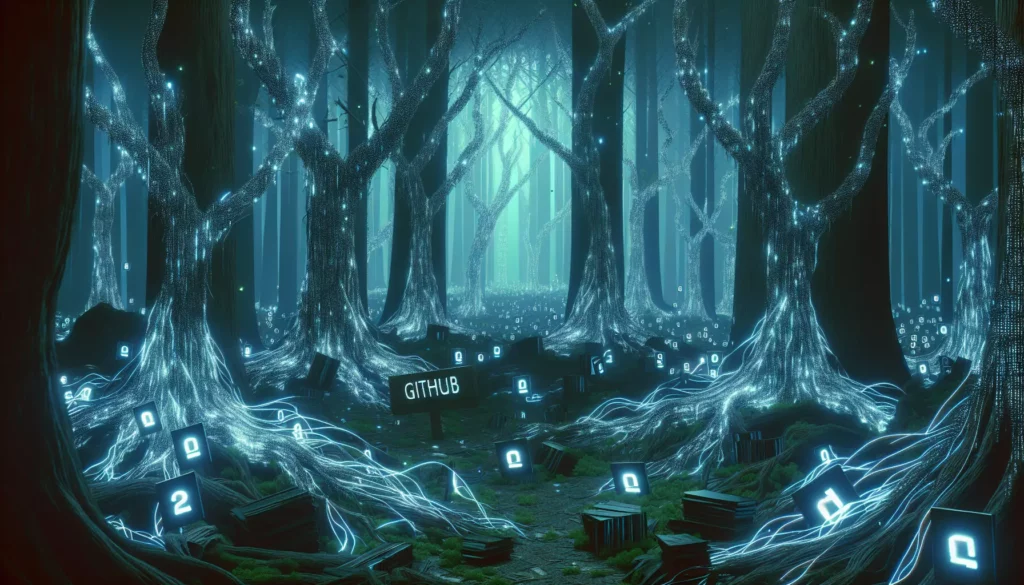
As developers, we’ve all been there. We start a project with enthusiasm, work on it for a while, and then… life happens. Our once-cherished repositories become digital graveyards, filled with half-finished features and forgotten ambitions. But what if we could breathe new life into these abandoned projects? What if we could transform our GitHub graveyard into a haunted code forest that both entertains and educates?
In this comprehensive guide, we’ll explore creative ways to repurpose your abandoned GitHub repositories, turning them into a spooky yet educational coding adventure. We’ll dive into techniques that not only showcase your coding skills but also provide valuable learning experiences for others. So, grab your digital shovel, and let’s start digging up those old repos!
Table of Contents
- Understanding Abandoned Repositories
- Preparing Your Haunted Code Forest
- Telling Ghost Stories with Code Comments
- Hiding Eerie Easter Eggs
- Creating Cursed Commits
- Implementing Phantom Features
- Crafting Spooky Documentation
- Setting Up a Haunted CI/CD Pipeline
- Resurrecting Zombie Issues
- Implementing Spectral Security Measures
- Maximizing Educational Value
- Engaging the Developer Community
- Conclusion
1. Understanding Abandoned Repositories
Before we start our haunting journey, let’s take a moment to understand what makes a repository “abandoned.” Typically, an abandoned repository has the following characteristics:
- No commits in the last 6-12 months
- Unresolved open issues and pull requests
- Outdated dependencies
- Incomplete or non-existent documentation
- Low or no recent engagement from contributors
These repositories often represent unfinished ideas, learning projects, or initiatives that lost steam over time. However, they can still hold valuable code, interesting algorithms, or unique approaches to problem-solving. By transforming them into a haunted code forest, we can preserve their essence while adding an entertaining twist.
2. Preparing Your Haunted Code Forest
To begin the transformation, we need to set the stage for our haunted code forest. Here’s how to get started:
2.1. Create a Spooky Landing Page
Update your repository’s README.md file to set the eerie tone. Use markdown to create a visually appealing and mysterious introduction. Here’s an example:
<!-- README.md -->
# 🌳👻 Welcome to the Haunted Code Forest 👻🌳
Dare you enter these cursed grounds, where abandoned code comes to life?
Beware of phantom functions and ghostly variables that lurk in the shadows.
## What awaits you:
- ðŸ•¯ï¸ Flickering code snippets
- 🦇 Batty algorithms
- 🎃 Pumpkin-spiced bugs
- 👻 Spectral data structures
*Enter at your own risk, for knowledge comes at a price in the Haunted Code Forest...*
2.2. Theme Your Repository
GitHub allows you to add a custom theme to your repository. Choose a dark theme or create a custom CSS file to give your repo a spooky appearance. You can do this by going to your repository’s settings and selecting the “Pages” tab.
2.3. Organize Your Haunted Directories
Rename your directories and files to fit the haunted theme. For example:
src/
becomeshaunted_grounds/
tests/
becomescursed_trials/
docs/
becomesancient_scrolls/
scripts/
becomesdark_rituals/
3. Telling Ghost Stories with Code Comments
One of the most effective ways to create a spooky atmosphere in your code is through creative commenting. Use comments to tell ghost stories, add eerie warnings, or create a sense of mystery. Here are some ideas:
3.1. The Cursed Function
// WARNING: This function is cursed.
// Legend has it that every developer who has tried to refactor it
// has mysteriously disappeared. Proceed with caution.
function summonDarkPowers(sacrifice) {
// TODO: Implement the ritual
throw new Error("The dark powers are not pleased with your offering");
}
3.2. The Ghostly Variable
let ghostlyWhisper = ""; // Listen closely, for its value changes when no one is looking
// Sometimes, late at night, developers swear they can hear it murmuring...
setInterval(() => {
if (Math.random() < 0.01) {
ghostlyWhisper = "Help me...";
}
}, 1000);
3.3. The Phantom Loop
// This loop is said to run for eternity, trapping the souls of unwary programmers
while (true) {
// The code that goes here has been lost to time
// Only the bravest dare to implement it
}
By peppering your code with these spooky comments, you create an immersive experience for anyone browsing your repository. It’s a fun way to engage readers and potentially teach them about code structure and documentation practices.
4. Hiding Eerie Easter Eggs
Easter eggs are a fantastic way to reward curious developers who dive deep into your code. In our haunted code forest, these easter eggs can take on a spooky twist. Here are some ideas for implementing eerie easter eggs:
4.1. The Whispering Function
Create a function that, when called with a specific set of parameters, reveals a hidden message:
function whisperSecret(x, y, z) {
if (x === 13 && y === "friday" && z === "midnight") {
console.log("You've discovered the secret passage! But beware what lies beyond...");
// TODO: Implement secret feature here
} else {
console.log("The forest remains silent...");
}
}
4.2. The Haunted ASCII Art
Hide some spooky ASCII art in your code that only appears under certain conditions:
function revealHauntedMansion(isFullMoon) {
if (isFullMoon) {
console.log(`
/\
/ \
/\ / \ /\
/ \ / \ / \
/ \ / \ / \
/ / \ \
/ / \ \
/______/______________\______\
| | ____ | |
| | | | | |
| | | | | |
|______|_____|____|_____|______|
`);
} else {
console.log("The mist is too thick to see anything...");
}
}
4.3. The Cryptic Comment
Leave a cryptic comment that, when deciphered, leads to a hidden feature or message:
// Decipher the code: 20-8-5 19-5-3-18-5-20 12-9-5-19 9-14 20-8-5 22-15-9-4
// Hint: A=1, B=2, C=3, ...
function unlockSecret(code) {
// TODO: Implement decryption logic
// If decrypted correctly, reveal the secret
}
These easter eggs not only add an element of fun to your repository but also encourage developers to explore your code more thoroughly. They can be used to highlight interesting coding techniques or to lead users to discover hidden features of your project.
5. Creating Cursed Commits
Your commit history is a powerful tool for storytelling. By crafting “cursed” commits, you can create an narrative arc that adds to the spooky atmosphere of your haunted code forest. Here are some ideas:
5.1. The Midnight Commit
Make a commit exactly at midnight with a cryptic message:
git commit -m "As the clock strikes twelve, the code awakens..."
5.2. The Vanishing Code
Create a commit that adds a significant amount of code, followed immediately by another commit that removes it all:
git commit -m "Implemented the summoning ritual"
git commit -m "The code has vanished without a trace..."
5.3. The Possessed Merge
When merging branches, use commit messages that suggest a supernatural event:
git merge feature/ghost-detection -m "Merging the spirit realm with our mortal code"
5.4. The Prophetic TODO
Add TODOs in your commits that foreshadow ominous events:
git commit -m "TODO: Prevent the awakening of the ancient bug in version 1.3.0"
These creative commits not only add to the haunted atmosphere but also demonstrate good Git practices, such as clear and descriptive commit messages, even if they’re playfully spooky.
6. Implementing Phantom Features
Phantom features are partially implemented functionalities that add an air of mystery to your codebase. They can be used to showcase interesting coding concepts or to hint at future developments. Here’s how to create them:
6.1. The Ghostly API
Create an API endpoint that only works under specific, mysterious conditions:
app.get('/api/phantom', (req, res) => {
const currentHour = new Date().getHours();
if (currentHour === 3) { // The witching hour
res.json({ message: "The veil between worlds has thinned. Proceed with caution." });
} else {
res.status(404).json({ message: "The phantom API is currently dormant." });
}
});
6.2. The Shapeshifting Function
Implement a function that behaves differently based on seemingly random conditions:
function shapeshifter(input) {
const randomFactor = Math.random();
if (randomFactor < 0.33) {
return input.toUpperCase();
} else if (randomFactor < 0.66) {
return input.split('').reverse().join('');
} else {
return input.repeat(3);
}
}
6.3. The Curse Breaker Challenge
Create a series of functions that must be called in a specific order to “break the curse”:
let curseLevel = 5;
function findAncientTome() {
console.log("You've discovered an ancient tome...");
curseLevel--;
}
function decipherRunes() {
console.log("The runes begin to make sense...");
curseLevel--;
}
function brewPotion() {
console.log("A mysterious potion bubbles in your cauldron...");
curseLevel--;
}
function castSpell() {
console.log("You utter words of power...");
curseLevel--;
}
function breakCurse() {
if (curseLevel === 0) {
console.log("The curse is broken! The repository is free!");
} else {
console.log(`The curse remains. Current level: ${curseLevel}`);
}
}
These phantom features not only add to the haunted atmosphere but also provide opportunities to demonstrate various coding techniques and concepts. They can be used to teach about API design, randomization, state management, and more.
7. Crafting Spooky Documentation
Documentation is crucial for any project, and in our haunted code forest, it’s an opportunity to blend helpful information with eerie storytelling. Here’s how to create documentation that’s both informative and atmospheric:
7.1. The Grimoire of Functions
Instead of a traditional function reference, create a “grimoire” that describes each function as if it were a magical spell:
## The Grimoire of Dark Functions
### Summon Ethereal Array
*Incantation:* `summonEtherealArray(size: number): any[]`
This arcane function conjures an array from the void, filled with ethereal elements.
Use with caution, for the contents of the array are unpredictable and may change
when observed.
**Parameters:**
- `size`: The desired size of the array. Beware, for numbers above 13 may anger the spirits.
**Returns:**
An array of `size` length, containing elements from beyond the veil.
**Example:**
```javascript
const etherealElements = summonEtherealArray(5);
console.log(etherealElements); // [undefined, "whisper", 👻, null, 42]
```
*Warning: Do not attempt to sort this array, lest you tear the fabric of reality.*
7.2. The Cursed Changelog
Transform your changelog into a record of the repository’s haunted history:
# The Cursed Changelog
## [1.3.0] - 2023-10-31 - The Awakening
### Added
- Summoned three new spectral entities to haunt the codebase
- Implemented a blood moon event that occurs every 28 commits
### Changed
- The `ghostlyWhisper` variable now changes its value when no one is looking
- Refactored the `curseBreaker` function to require a sacrifice
### Fixed
- Exorcised a bug that was possessing the login form
- Sealed a portal to the void that was leaking memory
### Removed
- Banished deprecated functions to the shadow realm
7.3. Installation Ritual
Turn your installation instructions into a mystical ritual:
## The Sacred Installation Ritual
To install this accursed package, follow these steps with utmost precision:
1. Prepare your development environment by drawing a salt circle around your computer.
2. Light a black candle and recite the following incantation:
```
npm install --save haunted-code-forest
```
3. If the installation is successful, you'll hear a faint whisper from your speakers.
If not, check your npm configuration and ensure you've appeased the code spirits.
4. To verify the installation, run the following command under a full moon:
```
npx haunted-code-forest --verify
```
5. If you see a spectral ASCII art appear in your console, the ritual is complete.
You may now begin to explore the haunted code forest.
*Note: We are not responsible for any paranormal activities that may occur in your codebase after installation.*
By creating documentation in this style, you not only provide necessary information but also immerse the reader in the haunted theme of your repository. This approach can make reading documentation more engaging and memorable.
8. Setting Up a Haunted CI/CD Pipeline
Continuous Integration and Continuous Deployment (CI/CD) are essential practices in modern software development. In our haunted code forest, we can give these processes a spooky twist while still maintaining their functionality. Here’s how to create a haunted CI/CD pipeline:
8.1. The Spectral Build Process
Rename your build stages to fit the haunted theme. For example, in a GitHub Actions workflow:
name: Haunted CI/CD Pipeline
on:
push:
branches: [ "main" ]
pull_request:
branches: [ "main" ]
jobs:
summon_the_code:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Gather the sacred artifacts
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Invoke the spirits of dependency
run: npm ci
- name: Perform the build ritual
run: npm run build
exorcise_the_bugs:
needs: summon_the_code
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Prepare the testing grounds
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Summon the test demons
run: npm test
deploy_to_the_nether:
needs: exorcise_the_bugs
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Invoke the deployment specter
uses: some-deployment-action@v1
with:
host: ${{ secrets.NETHER_HOST }}
username: ${{ secrets.NETHER_USERNAME }}
key: ${{ secrets.NETHER_SSHKEY }}
8.2. Cursed Build Notifications
Customize your build notifications to fit the haunted theme. For example, you could use a bot to post GitHub comments on pull requests:
const octokit = new Octokit({ auth: `token ${process.env.GITHUB_TOKEN}` });
async function postHauntedComment(status) {
const messages = {
success: "The spirits are pleased. Your offering has been accepted.",
failure: "A terrible curse has befallen your code. The build has failed.",
canceled: "The ritual was interrupted. The spirits grow restless..."
};
await octokit.issues.createComment({
owner: context.repo.owner,
repo: context.repo.repo,
issue_number: context.issue.number,
body: messages[status] || "The outcome is shrouded in mystery..."
});
}
// Use this function in your CI/CD pipeline
8.3. The Ethereal Deployment
Create a deployment script that adds a touch of mystery to the process:
#!/bin/bash
echo "Preparing the ethereal plane for deployment..."
sleep 2
echo "Channeling the spirits of the code..."
git pull origin main
echo "Invoking the build incantation..."
npm run build
echo "Sacrificing the old build to the digital void..."
rm -rf /var/www/html/*
echo "Manifesting the new build into reality..."
cp -R build/* /var/www/html/
echo "Awakening the server guardians..."
sudo systemctl restart nginx
echo "The deployment ritual is complete. May your code haunt in peace."
By theming your CI/CD pipeline in this way, you maintain all the best practices of automated testing and deployment while adding an extra layer of entertainment and engagement for developers working on or reviewing your project.
9. Resurrecting Zombie Issues
In many abandoned repositories, there are often unresolved issues that have been left to gather dust. Instead of simply closing these, we can “resurrect” them as zombie issues, adding to our haunted theme while potentially addressing real concerns. Here’s how to bring your issues back from the dead:
9.1. The Zombie Issue Template
Create a template for resurrecting old issues:
## 🧟 Zombie Issue: [Original Issue Title]
*This issue has risen from the grave, hungering for resolution.*
**Original Issue:** #[Issue Number]
**Time in Limbo:** [Time since last activity]
### Curse Description
[Brief description of the original issue]
### Necromancer's Notes
[Your assessment of the current relevance of this issue]
### Resurrection Ritual
To resolve this zombie issue, the following steps must be taken:
1. [ ] Examine the ancient code related to this issue
2. [ ] Perform the necessary incantations (code changes)
3. [ ] Offer a sacrifice (create a pull request)
4. [ ] Await judgment from the elder maintainers
### Warnings
- Approach with caution: This code may bite
- Failure to resolve may result in further hauntings
9.2. The Curse Breaker Board
Create a project board specifically for these resurrected issues. You can name it “The Curse Breaker’s Quest” and organize it with columns like:
- Awakened Curses (New)
- Haunting in Progress (In Progress)
- Exorcism Pending (Ready for Review)
- Curse Broken (Resolved)
9.3. The Summoning Ritual
Write a script to automatically resurrect old issues periodically. This could be a GitHub Action that runs on a schedule:
name: Summon Zombie Issues
on:
schedule:
- cron: '0 0 13 * *' # Runs at midnight on the 13th of every month
jobs:
resurrect_issues:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Resurrect Old Issues
env:
GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
run: |
# Use the GitHub CLI or API to find old, unresolved issues
# For each issue, create a new "zombie" issue using the template
# Add the new issue to "The Curse Breaker's Quest" board
9.4. The Exorcism Ceremony
When a zombie issue is resolved, perform an “exorcism ceremony” by posting a celebratory comment:
## ðŸ•¯ï¸ Exorcism Complete 🕯ï¸
The curse has been lifted, and this issue has been laid to rest.
**Exorcist:** @[Resolver's Username]
**Method of Banishment:** [Brief description of the solution]
May this code now rest in peace. 🪦
*The Haunted Code Forest grows quieter, but other spirits still lurk...*
By resurrecting and theming old issues in this way, you can bring attention to previously overlooked problems, encourage community engagement, and maintain the spooky atmosphere of your haunted code forest.
10. Implementing Spectral Security Measures
Security is a crucial aspect of any software project, and in our haunted code forest, we can theme our security measures to fit the spooky atmosphere while still maintaining robust protection. Here’s how to implement spectral security measures:
10.1. The Ethereal Firewall
Implement and document your security measures with a ghostly theme:
// Ethereal Firewall: Protects against spectral intrusions
const etherealFirewall = (req, res, next) => {
const forbiddenRealms = ['hacker.com', 'malware.org', 'evilspirit.net'];
const origin = req.get('Origin');
if (forbiddenRealms.some(realm => origin.includes(realm))) {
return res.status(403).json({ message: "You are banished from this realm!" });
}
next();
};
app.use(etherealFirewall);
10.2. The Cryptic Encryption
When implementing encryption, use spooky terminology in your comments and variable names:
const crypto = require('crypto');
function sealInCrypt(data, secretKey) {
const cipher = crypto.createCipher('aes-256-cbc', secretKey);
let encryptedData = cipher.update(data, 'utf8', 'hex');
encryptedData += cipher.final('hex');
return encryptedData;
}
function unleashFromCrypt(encryptedData, secretKey) {
const decipher = crypto.createDecipher('aes-256-cbc', secretKey);
let decryptedData = decipher.update(encryptedData, 'hex', 'utf8');
decryptedData += decipher.final('utf8');
return decryptedData;
}
// Usage
const secretScroll = "The ghost in the machine knows all";
const cryptKey = "13thHourOfTheHauntedNight";
const sealedScroll = sealInCrypt(secretScroll, cryptKey);
console.log("The sealed scroll:", sealedScroll);
const revealedContents = unleashFromCrypt(sealedScroll, cryptKey);
console.log("The scroll's contents:", revealedContents);
10.3. The Phantom Permissions
Create a role-based access control system with a supernatural twist:
const phantomPermissions = {
MORTAL: 0,
MEDIUM: 1,
GHOST: 2,
POLTERGEIST: 3,
SUPREME_SPIRIT: 4
};
function hasPhantomPermission(user, requiredLevel) {
return user.permissionLevel >= phantomPermissions[requiredLevel];
}
function summonSecretTome(user) {
if (hasPhantomPermission(user, 'GHOST')) {
return "The secret tome materializes in your spectral hands...";
} else {
return "The tome remains invisible to your mortal eyes.";
}
}
10.4. The Curse of Input Validation
Implement input validation with error messages that fit the haunted theme:
function validateSummoningCircle(input) {
const forbiddenSymbols = ['<script>', '</script>', 'DROP TABLE', 'rm -rf'];
if (forbiddenSymbols.some(symbol => input.includes(symbol))) {
throw new Error("Your summoning circle is corrupted with dark magic!");
}
if (input.length > 100) {
throw new Error("Your incantation is too long and has awakened angry spirits!");
}
return input.trim();
}
try {
const userInput = validateSummoningCircle(request.body.summoningCircle);
// Process the validated input
} catch (error) {
response.status(400).json({ message: error.message });
}
10.5. The Spectral Logging System
Implement a logging system that records security events with a ghostly flair:
const winston = require('winston');
const spectralLogger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.printf(({ level, message, timestamp }) => {
return `${timestamp} [${level.toUpperCase()}]: 👻 ${message}`;
})
),
transports: [
new winston.transports.File({ filename: 'spectral_activity.log' })
]
});
// Usage
spectralLogger.info('A new spirit has entered the code forest');
spectralLogger.warn('Unusual ectoplasmic activity detected in the database');
spectralLogger.error('Malevolent entity attempted to breach the ethereal firewall');
By implementing these spectral security measures, you not only protect your project but also maintain the haunted atmosphere of your code forest. This approach can make security considerations more engaging for developers and potentially more memorable, which is crucial for maintaining good security practices.
11. Maximizing Educational Value
While creating a haunted code forest is fun and engaging, it’s important to ensure that your project still provides educational value. Here are some ways to maximize the learning potential of your spooky repository:
11.1. Code Comments as Learning Tools
Use your ghostly code comments to explain complex concepts or interesting coding patterns:
// The Curse of Recursion
// Beware, for this function calls itself, much like a ghost
// reliving its final moments for all eternity...
function hauntedFactorial(n) {
// The base case: when n reaches 1, the haunting stops
if (n === 1) return 1;
// The recursive case: the function summons itself with n-1
return n * hauntedFactorial(n - 1);
}
// Usage:
console.log(hauntedFactorial(5)); // Outputs: 120
11.2. Algorithmic Curses
Implement classic algorithms with a spooky twist, providing explanations in the comments:
// The Spectral Sort: A haunted version of the Bubble Sort algorithm
function spectralSort(arr) {
let n = arr.length;
let swapped;
do {
swapped = false;
// The ghost of comparison floats through the array...
for (let i = 0; i