Why Companies Use Coding Challenges in the Hiring Process
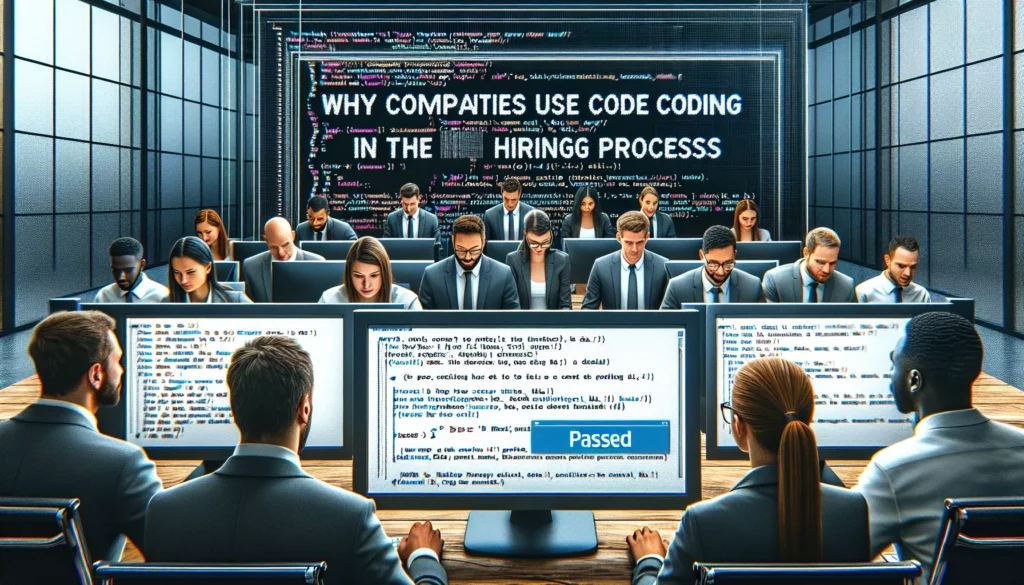
In today’s competitive tech landscape, companies are constantly seeking ways to identify and attract top talent. One method that has gained significant popularity in recent years is the use of coding challenges during the hiring process. These challenges, ranging from simple algorithmic problems to complex system design tasks, have become a staple in the recruitment strategies of tech giants and startups alike. But why exactly do companies rely on these coding tests, and what benefits do they offer to both employers and candidates? Let’s dive deep into the world of coding challenges and explore their role in modern tech recruitment.
The Rise of Coding Challenges in Tech Recruitment
The tech industry has always been at the forefront of innovation, not just in product development but also in hiring practices. As the demand for skilled software engineers continues to outpace supply, companies have had to evolve their recruitment strategies to efficiently identify candidates who possess not just theoretical knowledge, but practical coding skills.
Coding challenges emerged as a response to this need. They provide a standardized way to assess a candidate’s ability to write clean, efficient code and solve real-world problems. Unlike traditional interviews or resume screenings, coding challenges offer a more objective and skill-focused evaluation method.
Key Reasons Companies Use Coding Challenges
1. Assessing Practical Skills
One of the primary reasons companies use coding challenges is to evaluate a candidate’s practical coding skills. While resumes and academic qualifications can provide insight into a person’s background, they don’t necessarily reflect their ability to write functional code or solve problems efficiently.
Coding challenges allow companies to see how candidates approach problems, structure their code, and implement solutions. This hands-on assessment is crucial in determining whether a candidate can contribute effectively to the company’s projects from day one.
2. Standardizing the Evaluation Process
Coding challenges provide a standardized method for comparing candidates. By presenting all applicants with the same set of problems, companies can create a level playing field and make more objective comparisons between candidates.
This standardization is particularly valuable when dealing with a large number of applicants, as it allows recruiters and hiring managers to quickly identify top performers based on consistent criteria.
3. Filtering Candidates Efficiently
In a world where job postings can attract hundreds or even thousands of applicants, coding challenges serve as an effective initial filter. By requiring candidates to complete a coding test early in the application process, companies can quickly narrow down the pool to those who demonstrate the necessary technical skills.
This efficiency is crucial for both the company and the candidates. It saves time and resources by focusing subsequent interview stages on the most promising applicants.
4. Assessing Problem-Solving Abilities
Beyond just writing code, software development requires strong problem-solving skills. Coding challenges are designed to test a candidate’s ability to analyze problems, break them down into manageable components, and develop effective solutions.
These challenges often simulate real-world scenarios that developers might encounter on the job, providing insight into how candidates approach complex issues and adapt their strategies when faced with obstacles.
5. Evaluating Code Quality and Best Practices
In addition to functionality, coding challenges allow companies to assess the quality of a candidate’s code. This includes factors such as:
- Code readability and organization
- Adherence to coding standards and best practices
- Efficiency and optimization of solutions
- Proper use of data structures and algorithms
- Error handling and edge case consideration
By examining these aspects, companies can gauge whether a candidate’s coding style aligns with their own standards and practices.
6. Simulating Real Work Environments
Many coding challenges are designed to mimic the types of tasks and problems that developers face in their day-to-day work. This simulation helps companies assess how well a candidate might perform in their specific work environment.
For example, a challenge might involve working with a particular programming language, framework, or tool that the company uses regularly. This allows employers to evaluate not just general coding ability, but also specific skills relevant to their tech stack.
7. Identifying Passion and Dedication
Completing coding challenges, especially more complex ones, requires time and effort from candidates. Those who put in the work to solve these challenges often demonstrate a genuine passion for coding and a commitment to their craft.
This dedication is a valuable trait that companies look for in potential employees, as it often translates to a strong work ethic and a drive for continuous learning and improvement.
Types of Coding Challenges Used in Hiring
Companies employ various types of coding challenges, each designed to assess different aspects of a candidate’s skills and abilities. Some common types include:
1. Algorithmic Challenges
These challenges focus on a candidate’s ability to implement efficient algorithms and data structures to solve specific problems. They often test knowledge of fundamental computer science concepts and the ability to optimize for time and space complexity.
Example: Implement a function to find the longest palindromic substring in a given string.
def longest_palindrome(s):
if not s:
return ""
start = 0
max_length = 1
for i in range(len(s)):
# Check for odd-length palindromes
left, right = i, i
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
# Check for even-length palindromes
left, right = i, i + 1
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
return s[start:start+max_length]
2. System Design Challenges
These challenges assess a candidate’s ability to design and architect large-scale systems. They often involve considerations of scalability, reliability, and efficiency.
Example: Design a URL shortening service like bit.ly.
3. Debugging Challenges
Candidates are presented with a piece of code containing bugs or inefficiencies. Their task is to identify and fix these issues, demonstrating their ability to read, understand, and improve existing code.
Example: Debug and optimize the following function that is supposed to check if a number is prime:
def is_prime(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
4. Code Completion Challenges
These challenges provide partial code implementations and require candidates to complete the missing parts. This tests their ability to understand existing code and seamlessly integrate new functionality.
Example: Complete the following function to implement a binary search algorithm:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
# TODO: Implement the binary search logic here
pass
return -1 # Target not found
5. Full-Stack Challenges
For roles that require both frontend and backend skills, companies may use challenges that involve building a small application or feature. This assesses a candidate’s ability to work across the entire stack.
Example: Create a simple todo list application with a React frontend and a Node.js backend.
The Benefits of Coding Challenges for Candidates
While coding challenges are primarily used by companies to assess candidates, they also offer several benefits to job seekers:
1. Opportunity to Showcase Skills
Coding challenges provide candidates with a platform to demonstrate their technical abilities beyond what can be conveyed in a resume or traditional interview. This is particularly valuable for self-taught programmers or those with non-traditional backgrounds who might not have extensive work experience or formal education in computer science.
2. Practice for Real-World Scenarios
Many coding challenges are designed to reflect real-world problems that developers encounter in their day-to-day work. By engaging with these challenges, candidates can gain valuable experience in problem-solving and coding under pressure, skills that are directly applicable to their future roles.
3. Learning and Improvement
Regardless of the outcome, participating in coding challenges can be a significant learning experience. Candidates often receive feedback on their solutions, which can help them identify areas for improvement and refine their skills.
4. Insight into Company Culture and Expectations
The nature and content of coding challenges can provide candidates with insights into a company’s technical focus, coding standards, and overall approach to software development. This information can be valuable in determining whether the company is a good fit for their career goals and working style.
5. Confidence Building
Successfully completing coding challenges can boost a candidate’s confidence in their abilities. This increased self-assurance can be beneficial not only for the current application process but also for future job searches and career development.
Potential Drawbacks and Criticisms of Coding Challenges
While coding challenges have become a standard part of the tech hiring process, they are not without their critics. Some common concerns include:
1. Time Constraints
Many coding challenges are timed, which can create unnecessary pressure and may not accurately reflect a candidate’s ability to solve problems in a real work environment where time constraints are often more flexible.
2. Lack of Real-World Context
Some argue that coding challenges, especially algorithmic ones, don’t accurately represent the day-to-day work of most software developers. Critics contend that these challenges place too much emphasis on computer science theory rather than practical programming skills.
3. Potential for Bias
There’s a concern that coding challenges may inadvertently favor candidates who have more free time to practice or those who have recently studied algorithms and data structures, potentially disadvantaging experienced developers who may be rusty on these topics.
4. Stress and Anxiety
The high-stakes nature of coding challenges in the hiring process can cause significant stress and anxiety for candidates, potentially impacting their performance and not accurately reflecting their true abilities.
5. Overemphasis on Speed
Some coding challenges prioritize speed of completion over other important factors like code quality, readability, and maintainability. This can lead to a mismatch between challenge performance and actual job performance.
Best Practices for Companies Using Coding Challenges
To maximize the effectiveness of coding challenges while addressing some of the criticisms, companies can adopt the following best practices:
1. Tailor Challenges to the Role
Ensure that the coding challenges are relevant to the specific position being filled. For example, a frontend developer role might focus more on JavaScript and UI-related problems, while a backend role might emphasize server-side logic and database interactions.
2. Provide Realistic Time Frames
Allow candidates sufficient time to complete challenges, mirroring realistic work scenarios. Consider offering take-home assignments with reasonable deadlines instead of strictly timed tests.
3. Offer a Variety of Challenge Types
Include a mix of algorithmic problems, practical coding tasks, and system design questions to get a well-rounded view of a candidate’s abilities.
4. Focus on Problem-Solving Process
Evaluate not just the final solution, but also the candidate’s approach to solving the problem. Consider asking candidates to explain their thought process or provide comments in their code.
5. Provide Clear Instructions and Expectations
Ensure that challenge instructions are clear and unambiguous. Specify what aspects of the solution will be evaluated (e.g., correctness, efficiency, code style).
6. Offer Feedback
Provide constructive feedback to candidates on their performance, regardless of whether they move forward in the process. This helps candidates improve and maintains a positive relationship with potential future applicants.
7. Use Coding Challenges as Part of a Holistic Assessment
Don’t rely solely on coding challenges to make hiring decisions. Use them in conjunction with interviews, portfolio reviews, and other assessment methods to get a comprehensive view of each candidate.
Preparing for Coding Challenges: Tips for Candidates
For job seekers looking to excel in coding challenges, consider the following tips:
1. Practice Regularly
Utilize online platforms like LeetCode, HackerRank, or AlgoCademy to practice coding problems regularly. Focus on a variety of problem types and difficulty levels.
2. Study Core Computer Science Concepts
Review fundamental data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming). Understand their time and space complexities.
3. Improve Problem-Solving Skills
Work on breaking down complex problems into smaller, manageable parts. Practice explaining your thought process out loud as you solve problems.
4. Learn Multiple Programming Languages
While mastering one language is important, having familiarity with multiple languages can be beneficial. Many companies allow candidates to choose their preferred language for coding challenges.
5. Focus on Code Quality
Practice writing clean, readable, and well-commented code. Pay attention to naming conventions, code organization, and adherence to best practices.
6. Time Management
Practice solving problems under time constraints to improve your efficiency. Learn when to move on from a problem that’s taking too long.
7. Mock Interviews
Participate in mock technical interviews with peers or mentors to simulate the pressure of real coding challenges and receive feedback on your performance.
The Future of Coding Challenges in Tech Hiring
As the tech industry continues to evolve, so too will the methods used to assess and hire talent. While coding challenges are likely to remain a significant part of the hiring process, we can expect to see some changes and innovations:
1. More Realistic Assessments
There’s a growing trend towards creating coding challenges that more closely mimic real-world scenarios. This might include working with existing codebases, debugging live systems, or collaborating on team projects.
2. AI-Powered Evaluations
Artificial intelligence and machine learning are being increasingly used to analyze candidates’ code, assessing not just correctness but also style, efficiency, and problem-solving approach.
3. Holistic Skill Assessment
Future coding challenges may incorporate elements that assess soft skills such as communication, teamwork, and adaptability alongside technical abilities.
4. Continuous Assessment
Some companies are exploring models where candidates engage in longer-term projects or apprenticeships as part of the hiring process, allowing for a more comprehensive evaluation of their skills and fit within the organization.
5. Focus on Ethical Considerations
As awareness of bias in hiring practices grows, there will likely be increased emphasis on creating fair and inclusive coding challenges that don’t disadvantage certain groups of candidates.
Conclusion
Coding challenges have become an integral part of the tech hiring process for good reason. They offer companies a standardized, objective way to assess candidates’ practical skills, problem-solving abilities, and coding proficiency. For candidates, these challenges provide an opportunity to showcase their talents and gain valuable experience.
However, it’s crucial for companies to implement coding challenges thoughtfully, ensuring they are fair, relevant, and part of a holistic assessment process. By following best practices and continually refining their approach, companies can use coding challenges effectively to identify top talent while providing a positive experience for candidates.
As the tech industry continues to evolve, we can expect coding challenges to adapt as well, potentially incorporating more realistic scenarios, AI-powered evaluations, and assessments of a broader range of skills. Regardless of how they change, coding challenges are likely to remain a key tool in connecting talented developers with exciting opportunities in the ever-growing tech sector.