The Hidden Messages in Your Console.log() Statements: A Psychoanalysis
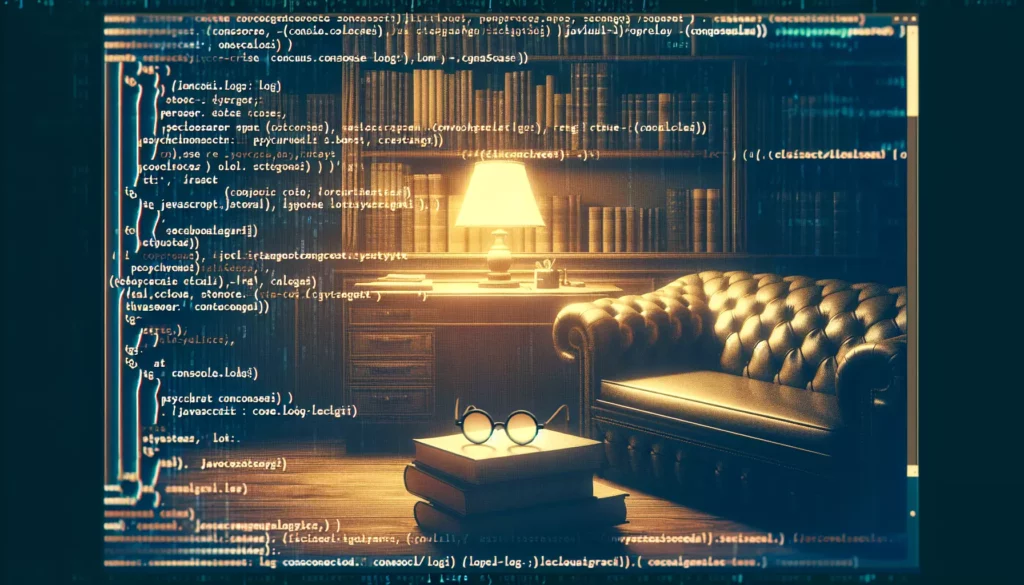
As developers, we often find ourselves in the trenches of debugging, armed with our trusty console.log()
statements. These seemingly innocuous lines of code are our go-to tool for peering into the inner workings of our programs. But have you ever stopped to consider what your console.log()
messages might reveal about you as a programmer? Welcome to a whimsical journey into the psyche of developers, as seen through the lens of their debugging statements.
The Psychology Behind Debugging
Before we dive into the specifics of console.log()
psychoanalysis, let’s take a moment to consider the psychological aspects of debugging. Debugging is more than just a technical process; it’s a cognitive challenge that requires problem-solving skills, patience, and often, a good sense of humor.
When we debug, we’re essentially playing detective in our own code. We’re looking for clues, following leads, and trying to piece together a narrative of what’s going wrong. This process can be frustrating, enlightening, and sometimes even entertaining. The way we approach debugging, and the tools we use, can say a lot about our personality and coding style.
The Classic “Hello World” Developer
Let’s start with the most basic of console.log()
statements:
console.log("Hello World");
If you find yourself frequently using this classic phrase in your debugging, you might be a traditionalist at heart. You appreciate the fundamentals and have a nostalgic streak. There’s a good chance you learned to code from textbooks or formal courses, and you have a deep respect for the history of programming.
Psychoanalysis: You’re reliable and consistent. Your code is likely well-documented and follows best practices. However, you might sometimes struggle with adopting newer, trendier approaches to problem-solving.
The “What the…?” Developer
Have you ever caught yourself writing something like this?
console.log("What the...?");
console.log("How did we get here?");
console.log("This shouldn't be possible!");
If your console.log()
statements often express bewilderment or disbelief, you might be what we call a “What the…?” developer. You’re not afraid to express your emotions through code, and you approach debugging with a sense of adventure and surprise.
Psychoanalysis: You’re likely an creative problem-solver who enjoys tackling complex issues. Your code might be innovative, but it could also benefit from more rigorous planning and structure to avoid those “What the…?” moments.
The Sherlock Holmes Developer
Do your debugging sessions look something like this?
console.log("Entering function X");
console.log("Variable Y value:", y);
console.log("Condition Z met:", z === true);
console.log("Exiting function X");
If you meticulously log every step of your program’s execution, you might be a Sherlock Holmes developer. You leave no stone unturned in your quest to understand exactly what’s happening in your code.
Psychoanalysis: You’re detail-oriented and thorough. Your code is likely to be well-tested and robust. However, you might sometimes get lost in the details and overlook bigger-picture issues.
The Emoji Enthusiast
Have you embraced the world of emoji in your debugging?
console.log("🚀 Function launched!");
console.log("🔠Searching for bug...");
console.log("🛠Bug found!");
console.log("🎉 Problem solved!");
If your console is filled with colorful characters, you’re likely an Emoji Enthusiast developer. You bring a sense of fun and visual flair to even the most mundane debugging tasks.
Psychoanalysis: You’re probably a creative and visually-oriented programmer. You might excel at front-end development or creating user-friendly interfaces. However, be careful not to let your love of aesthetics overshadow the need for clear, concise communication in your code.
The Minimalist
Does this look familiar?
console.log(1);
console.log(2);
console.log(3);
If your console.log()
statements are stripped down to the bare essentials, you might be a Minimalist developer. You believe in simplicity and efficiency, even in your debugging process.
Psychoanalysis: You’re likely to write clean, efficient code that gets straight to the point. However, your minimalist approach might sometimes make it difficult for others (or even yourself in the future) to understand the context of your debugging statements.
The Shakespeare Developer
Do your debugging statements read like soliloquies?
console.log("To log, or not to log: that is the question:");
console.log("Whether 'tis nobler in the mind to suffer");
console.log("The slings and arrows of outrageous bugs,");
console.log("Or to take arms against a sea of errors...");
If you find yourself waxing poetic in your console.log()
statements, you might be a Shakespeare developer. You see coding as an art form and bring a literary flair to your debugging process.
Psychoanalysis: You’re likely to be creative and expressive in your coding style. Your code might be a joy to read, but be careful not to sacrifice clarity for creativity, especially in collaborative environments.
The Time Traveler
Does your debugging look like a journey through time?
console.log("[10:15:30] Starting function");
console.log("[10:15:31] Processing data");
console.log("[10:15:32] Error occurred");
console.log("[10:15:33] Retrying...");
If you’re meticulous about logging timestamps, you might be a Time Traveler developer. You’re keenly aware of the temporal aspects of your code’s execution.
Psychoanalysis: You’re likely to excel at performance optimization and debugging timing-related issues. Your code is probably well-structured and efficient. However, be careful not to get too caught up in micro-optimizations at the expense of overall functionality.
The Comedian
Do your debugging sessions sometimes feel like a stand-up routine?
console.log("Why did the programmer quit his job? He didn't get arrays!");
console.log("Here's another bug. I'm really excelling at finding these!");
console.log("If debugging is the process of removing bugs, then programming must be the process of putting them in.");
If your console.log()
statements are peppered with jokes and puns, you might be a Comedian developer. You believe that a sense of humor is essential for maintaining sanity during long debugging sessions.
Psychoanalysis: You likely have a positive attitude towards problem-solving and can keep your cool under pressure. Your lighthearted approach might help create a positive team atmosphere. However, make sure your jokes don’t obscure important information in your logs.
The Drill Sergeant
Does your debugging sound like boot camp?
console.log("ATTENTION! Entering function perimeter!");
console.log("REPORT! Variable status: undefined");
console.log("ALERT! Exception detected! Brace for impact!");
console.log("RETREAT! Exiting function with error code 1");
If your console is shouting orders, you might be a Drill Sergeant developer. You approach debugging with military precision and a no-nonsense attitude.
Psychoanalysis: You’re likely to be highly organized and disciplined in your coding practices. Your code probably follows strict patterns and is well-documented. However, your intense approach might be intimidating to less experienced team members.
The Conspiracy Theorist
Does your debugging process feel like unraveling a complex plot?
console.log("The function says it returned true, but can we really trust it?");
console.log("This variable changed when no one was looking. Coincidence? I think not!");
console.log("The API says the request failed, but what if it's just hiding something?");
If you find yourself questioning every aspect of your code’s behavior, you might be a Conspiracy Theorist developer. You leave no stone unturned and trust no function implicitly.
Psychoanalysis: Your skeptical approach can be valuable in uncovering subtle bugs and edge cases. You’re likely to write robust, well-tested code. However, be careful not to let your suspicions paralyze your progress or lead you down unproductive rabbit holes.
The Archaeologist
Does your debugging feel like an excavation?
console.log("=== Layer 1: Recent code ===");
console.log("=== Layer 2: Last month's refactor ===");
console.log("=== Layer 3: Ancient legacy code ===");
console.log("=== Layer 4: Here be dragons ===");
If you find yourself digging through layers of code history during your debugging sessions, you might be an Archaeologist developer. You’re not afraid to explore the deepest, dustiest corners of your codebase.
Psychoanalysis: You likely have a deep understanding of your project’s evolution and can provide valuable historical context. Your willingness to engage with legacy code is admirable. However, be careful not to get too bogged down in old code at the expense of moving forward.
The Zen Master
Is your debugging process a journey to enlightenment?
console.log("Breathe in. The function begins.");
console.log("Be mindful of the variables as they change.");
console.log("Embrace the error, for it teaches us.");
console.log("Breathe out. The function ends.");
If your console.log()
statements read like meditation instructions, you might be a Zen Master developer. You approach debugging with calm and mindfulness, seeing each bug as an opportunity for growth.
Psychoanalysis: Your peaceful approach likely helps you maintain composure in stressful debugging situations. You’re probably patient and persistent in solving complex problems. However, make sure your serene attitude doesn’t lead to complacency or a lack of urgency when dealing with critical issues.
The Implications of Your Debugging Style
While this psychoanalysis is tongue-in-cheek, your debugging style can indeed reveal aspects of your personality and approach to problem-solving. Understanding your debugging tendencies can help you leverage your strengths and address potential weaknesses:
- Communication: Your
console.log()
statements are a form of communication, not just with yourself but potentially with other developers who might read your code. Clear, informative logs can greatly assist in collaborative debugging efforts. - Problem-solving approach: The way you structure your debugging process can reflect your overall approach to tackling coding challenges. Are you methodical and systematic, or more intuitive and exploratory?
- Attention to detail: The level of detail in your logs can indicate how thorough you are in your coding practices. Detailed logs can be helpful, but too much information can also obscure the important details.
- Creativity: Even in something as technical as debugging, there’s room for creativity. Your unique debugging style can bring fresh perspectives to problem-solving.
- Emotional resilience: Debugging can be frustrating. The tone of your log messages might reflect how you handle the emotional aspects of troubleshooting difficult issues.
Evolving Your Debugging Technique
Regardless of which debugging personality type you identify with, there’s always room for growth and improvement. Here are some tips to evolve your debugging technique:
- Be clear and concise: While creative log messages can be fun, make sure they don’t obscure the important information. Strive for clarity in your debugging statements.
- Use appropriate tools: While
console.log()
is a great starting point, familiarize yourself with more advanced debugging tools like breakpoints, watch expressions, and step-through debugging in your IDE. - Structure your logs: Consider using a consistent format for your log messages. This can make it easier to parse logs, especially when dealing with large amounts of output.
- Log smartly: Instead of logging everything, focus on the most relevant information. Strategic logging can help you pinpoint issues more quickly.
- Clean up after yourself: Remember to remove or comment out unnecessary log statements before committing your code. Excessive logging can clutter your codebase and potentially impact performance.
- Learn from others: Pay attention to how your colleagues debug. You might pick up new techniques or perspectives that can enhance your own debugging process.
Conclusion: Embracing Your Debugging Persona
Whether you’re a Sherlock Holmes, a Zen Master, or a unique combination of multiple personas, your debugging style is an integral part of your identity as a developer. Embrace your quirks, but also strive to refine your technique. Effective debugging is a crucial skill in any developer’s toolkit, and your personal flair can make the process more engaging and enjoyable.
Remember, the next time you find yourself knee-deep in console.log()
statements, you’re not just debugging—you’re expressing your unique programmer personality. Happy debugging, and may your console always be filled with insightful (and perhaps occasionally humorous) messages!
As you continue your journey in software development, consider how your debugging style aligns with your overall approach to coding and problem-solving. Are there areas where you could improve? Are there aspects of your style that particularly serve you well? Reflecting on these questions can help you become not just a better debugger, but a more well-rounded developer overall.
And who knows? Maybe one day, we’ll have AI sophisticated enough to psychoanalyze our code and debugging patterns, providing insights into our programming psyche. Until then, keep logging, keep learning, and most importantly, keep coding!