The Philosophical Implications of Choosing Tabs Over Spaces
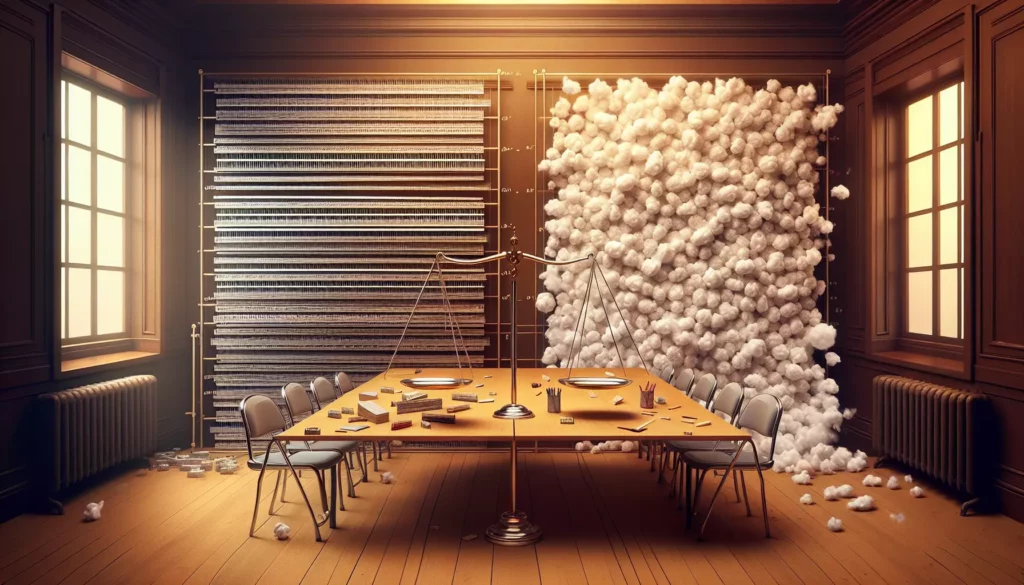
In the vast realm of programming, few debates have sparked as much passion and controversy as the age-old question: tabs or spaces? This seemingly trivial choice in code indentation has divided developers for decades, leading to heated discussions, memes, and even plot points in popular TV shows. But beyond the surface-level arguments about efficiency and aesthetics, there lies a deeper philosophical dimension to this debate that reflects fundamental aspects of human nature, cognitive processes, and the very essence of how we approach problem-solving in the digital age.
The Great Divide: Understanding the Tabs vs. Spaces Debate
Before delving into the philosophical implications, it’s crucial to understand the basics of the tabs vs. spaces debate. In programming, indentation is used to organize code and make it more readable. There are two primary methods for achieving this:
- Tabs: Using the tab character to create indentation.
- Spaces: Using a specific number of space characters (usually 2 or 4) to create indentation.
At first glance, this choice might seem inconsequential. After all, both methods achieve the same visual result: properly indented code. However, the implications of this choice run much deeper than mere aesthetics.
The Philosophical Underpinnings
1. Absolutism vs. Relativism
The choice between tabs and spaces can be seen as a manifestation of the philosophical debate between absolutism and relativism. Tabs represent a more absolutist approach: one tab character always represents one level of indentation, regardless of how it’s displayed. This aligns with the idea of absolute truths or universal standards.
Spaces, on the other hand, embody a more relativist perspective. The number of spaces used for indentation can vary (2, 4, or even 8), reflecting the idea that truth or standards can be relative to individuals or contexts.
2. Efficiency vs. Precision
The tabs vs. spaces debate also touches on the philosophical question of prioritizing efficiency or precision. Tabs are often touted as more efficient: they require fewer keystrokes and take up less file space. This efficiency-first approach aligns with utilitarian philosophies that prioritize maximizing overall benefit or output.
Spaces, while potentially less efficient, offer more precise control over code appearance across different editors and environments. This precision-focused approach resonates with deontological ethical frameworks that emphasize adherence to principles or rules, regardless of consequences.
3. Universalism vs. Particularism
The debate also reflects the philosophical tension between universalism and particularism. Tabs represent a more universalist approach: a single, universal character that can be interpreted differently based on individual preferences. This aligns with philosophical ideas about universal truths or principles that transcend individual contexts.
Spaces, conversely, embody a more particularist view. By explicitly defining the exact number of spaces, developers are choosing a specific, particular representation of indentation. This resonates with philosophical perspectives that emphasize the importance of context and reject universal absolutes.
Cognitive Implications: How Our Choices Shape Our Thinking
The tabs vs. spaces debate isn’t just about philosophy; it also has implications for how we think about and approach coding problems.
1. Abstract vs. Concrete Thinking
Choosing tabs often requires a more abstract way of thinking about code structure. Developers must conceptualize indentation levels without being tied to a specific visual representation. This abstract thinking can translate to other areas of programming, potentially influencing how developers approach algorithm design or system architecture.
Spaces, on the other hand, encourage a more concrete, visually-oriented approach. This concrete thinking might lead to stronger emphasis on code readability and visual structure, potentially influencing coding styles and documentation practices.
2. Flexibility vs. Consistency
The choice between tabs and spaces also reflects different cognitive approaches to flexibility and consistency. Tabs offer flexibility: each developer can set their preferred tab width without changing the underlying code. This flexible mindset might translate to more adaptable problem-solving approaches in other areas of programming.
Spaces prioritize consistency: the code looks the same for everyone, regardless of individual settings. This consistency-focused approach might lead to more standardized coding practices and a stronger emphasis on team-wide conventions.
Social and Cultural Implications
The tabs vs. spaces debate extends beyond individual choices, reflecting broader social and cultural aspects of the programming community.
1. Tribalism and Identity
The strong opinions held by developers on this issue often lead to a form of tribalism. “Tabs people” and “spaces people” can form distinct groups, complete with their own in-jokes, memes, and shared experiences. This tribalism reflects broader human tendencies to form group identities around shared beliefs or practices.
In the context of coding education platforms like AlgoCademy, this tribalism can manifest in how learners approach different coding styles or conventions. It’s crucial for educators to address these biases and encourage open-mindedness to different approaches.
2. Authority and Rebellion
The choice between tabs and spaces can also be seen as a reflection of attitudes towards authority and convention. Some developers might choose one method simply because it’s the standard in their organization or the broader community, reflecting a respect for established norms. Others might deliberately choose the less common option as a form of rebellion or assertion of individuality.
This dynamic plays out in broader contexts within the tech industry, influencing how developers approach new technologies, coding paradigms, or industry standards.
Practical Implications for Coding and Collaboration
While the philosophical implications are fascinating, it’s important to consider the practical impact of the tabs vs. spaces choice on actual coding practices and collaboration.
1. Code Readability and Maintenance
One of the primary arguments for spaces is consistency in code appearance across different editors and environments. This consistency can enhance code readability and make maintenance easier, especially in large teams or open-source projects.
Here’s an example of how tabs might appear differently in different editors:
function exampleFunction() {
<tab>if (condition) {
<tab><tab>console.log("This is indented with tabs");
<tab>}
}
Depending on the tab width setting, this code might look differently to different developers.
In contrast, spaces ensure consistent appearance:
function exampleFunction() {
if (condition) {
console.log("This is indented with spaces");
}
}
This code will look the same regardless of editor settings.
2. Version Control and Diffs
The choice between tabs and spaces can impact version control systems and how code changes are displayed. Spaces can sometimes make diffs (the display of changes between versions) cleaner and easier to read, as each space is treated as a separate character.
For example, a diff with tabs might look like this:
- function oldFunction() {
- <tab>console.log("Old code");
- }
+ function newFunction() {
+ <tab>console.log("New code");
+ }
While a diff with spaces might appear as:
- function oldFunction() {
- console.log("Old code");
- }
+ function newFunction() {
+ console.log("New code");
+ }
The spaces version can be easier to read and understand, especially when dealing with small changes in indentation.
3. File Size and Performance
One argument often made in favor of tabs is that they can lead to smaller file sizes, as a single tab character represents what might otherwise be multiple space characters. While this difference is negligible for small projects, it could potentially impact larger codebases or situations where every byte counts.
Here’s a simple comparison:
// Using tabs (file size: 59 bytes)
function example() {
<tab>if (condition) {
<tab><tab>return true;
<tab>}
<tab>return false;
}
// Using spaces (file size: 67 bytes)
function example() {
if (condition) {
return true;
}
return false;
}
While the difference is small in this example, it could add up in larger projects.
The Role of Style Guides and Linters
In professional settings, the tabs vs. spaces debate is often resolved through the use of style guides and linting tools. These tools enforce consistent coding styles across a project or organization, often including rules about indentation.
1. Popular Style Guides
Many popular style guides have taken a stance on the tabs vs. spaces issue:
- Google’s Style Guides: Generally recommend 2 spaces for indentation in most languages.
- Airbnb JavaScript Style Guide: Recommends 2 spaces for indentation.
- PEP 8 (Python): Recommends 4 spaces for indentation.
These style guides often influence broader community practices, shaping how developers approach indentation and other coding conventions.
2. Linting Tools
Linters are tools that analyze code for potential errors and style violations. Many linters include rules about indentation, allowing teams to enforce consistent use of tabs or spaces.
For example, in ESLint (a popular JavaScript linter), you can set indentation rules like this:
{
"rules": {
"indent": ["error", 2] // Enforce 2 spaces for indentation
}
}
This rule would flag any code that doesn’t use 2 spaces for indentation as an error.
The Impact on Coding Education
For coding education platforms like AlgoCademy, the tabs vs. spaces debate presents both challenges and opportunities.
1. Teaching Best Practices
While it’s important to acknowledge the ongoing debate, coding education platforms often need to take a stance to teach consistent practices. This might involve:
- Choosing a default indentation style for all examples and exercises
- Explaining the reasoning behind the chosen style
- Discussing the broader debate and its implications
2. Flexibility in Learning Environments
Platforms like AlgoCademy can use the tabs vs. spaces debate as an opportunity to teach about coding flexibility and the importance of adapting to different team standards. This might include:
- Allowing learners to choose their preferred indentation style in coding exercises
- Providing options to view code examples in both tabs and spaces
- Teaching how to configure editors and linters to enforce specific indentation styles
3. Preparing for Real-World Scenarios
By addressing the tabs vs. spaces debate, coding education platforms can prepare learners for the diverse preferences they’ll encounter in real-world development teams. This preparation might involve:
- Exercises in reading and working with code that uses different indentation styles
- Discussions about how to handle style disagreements in a team setting
- Lessons on using version control systems with different indentation styles
The Future of the Debate
As programming languages and development tools evolve, the tabs vs. spaces debate may take new forms or potentially become obsolete.
1. Intelligent Editors
Modern code editors are becoming increasingly intelligent, with features like:
- Automatic indentation detection and adaptation
- Options to display tabs as a specific number of spaces
- Real-time collaboration tools that handle indentation differences seamlessly
These advancements may reduce the practical impact of the tabs vs. spaces choice, though the philosophical implications may persist.
2. New Programming Paradigms
As new programming paradigms emerge, the way we think about code structure and indentation may change. For example:
- Visual programming languages might eliminate the need for text-based indentation entirely
- AI-assisted coding might handle indentation automatically based on code context and team preferences
- New markup or formatting systems might provide alternatives to traditional indentation methods
Conclusion: The Deeper Meaning of a Simple Choice
The tabs vs. spaces debate, while often treated as a lighthearted topic in programming circles, reveals profound insights into how developers think, collaborate, and approach problem-solving. It touches on fundamental philosophical questions about absolutism vs. relativism, efficiency vs. precision, and universalism vs. particularism.
For platforms like AlgoCademy, understanding these implications is crucial for providing comprehensive coding education. By addressing not just the technical aspects of coding but also the underlying philosophical and social dimensions, we can prepare learners for the complex, multifaceted world of professional software development.
Ultimately, whether you choose tabs or spaces, the most important thing is consistency and clear communication within your team or community. The debate itself serves as a valuable lesson in the importance of understanding different perspectives, adapting to team standards, and thinking critically about the tools and practices we use in our daily work as developers.
As we continue to push the boundaries of technology and explore new frontiers in software development, let’s remember that even the smallest choices can reflect our deepest values and shape the way we approach the complex challenges of our field. Whether you’re a steadfast supporter of tabs, a dedicated spaces enthusiast, or somewhere in between, your choice is a reflection of your unique perspective as a developer – and that diversity of thought is what drives our industry forward.