How to Use Your Unfinished Projects as Modern Art Installations
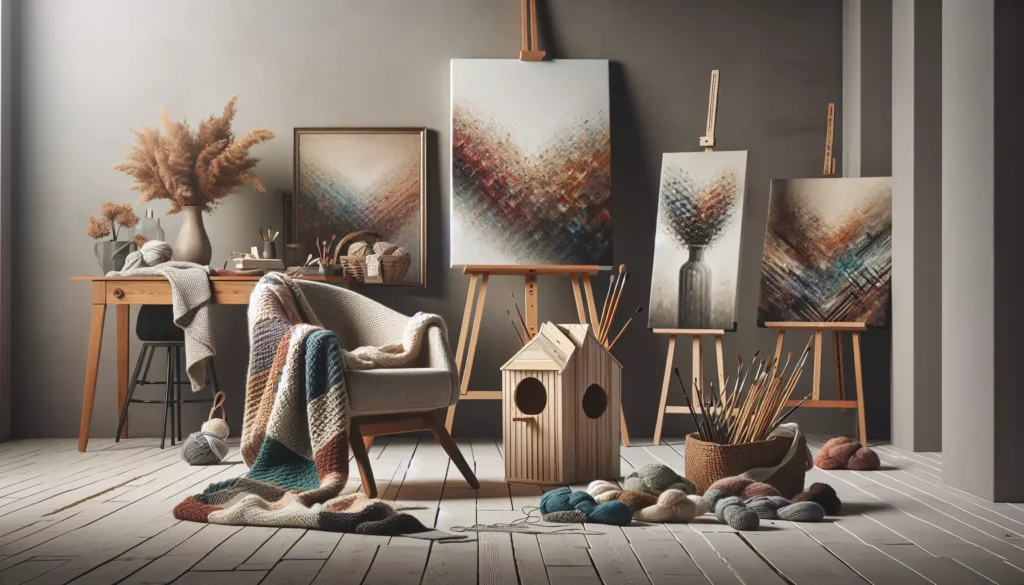
As software developers and coding enthusiasts, we’ve all been there: a graveyard of unfinished projects, half-baked ideas, and abandoned repositories. But what if I told you that these digital relics could be more than just ghosts haunting your GitHub account? Welcome to the intersection of code and art, where your unfinished projects can transform into thought-provoking modern art installations. In this comprehensive guide, we’ll explore how to breathe new life into your coding endeavors and turn them into captivating artistic expressions.
The Art of Unfinished Code
Before we dive into the practical aspects of creating art from code, let’s consider the philosophical implications of unfinished projects. In the world of software development, we often equate completion with success. However, the journey of creation is just as valuable as the destination. Unfinished projects represent potential, creativity, and the ever-evolving nature of technology.
Art has long celebrated the unfinished. From Michelangelo’s “non-finito” sculptures to modern abstract expressionism, incompleteness can be a powerful artistic statement. Your unfinished code projects are no different – they tell a story of exploration, learning, and the creative process.
Preparing Your Code for Artistic Transformation
To begin your journey from coder to artist, you’ll need to prepare your unfinished projects. Here are some steps to get started:
- Inventory Your Projects: Go through your repositories, local folders, and even those long-forgotten USB drives. Make a list of all your unfinished projects.
- Assess the Visual Potential: Look at your code not just as functionality, but as visual elements. Consider the structure, patterns, and even the comments within your code.
- Clean and Curate: While the goal isn’t perfection, you may want to tidy up your code a bit. Remove sensitive information and focus on the most visually or conceptually interesting parts.
- Document the Context: Write a brief description of each project – what was the goal, why did you start it, and why did it remain unfinished? This context will add depth to your art installation.
Transforming Code into Visual Art
Now that you’ve prepared your unfinished projects, let’s explore various ways to transform them into visual art:
1. Code as Typography
One of the simplest yet effective ways to turn code into art is by treating it as typography. The structure and syntax of code can create interesting visual patterns when displayed at scale.
Implementation Ideas:
- Print your code on large canvases or posters
- Use different colors for different elements (e.g., keywords, variables, comments)
- Experiment with fonts that complement the code’s structure
Example:
<canvas id="codeCanvas" width="800" height="600"></canvas>
<script>
const canvas = document.getElementById('codeCanvas');
const ctx = canvas.getContext('2d');
function drawCode(code) {
ctx.font = '12px Courier';
ctx.fillStyle = '#333';
const lines = code.split('\n');
lines.forEach((line, index) => {
ctx.fillText(line, 10, 20 + (index * 15));
});
}
const unfinishedCode = `
function sortAlgorithm(arr) {
// TODO: Implement sorting logic
return arr;
}
class UnfinishedProject {
constructor() {
this.ideas = [];
this.bugs = [];
this.potential = Infinity;
}
// More unfinished methods...
}
`;
drawCode(unfinishedCode);
</script>
This example creates a canvas and draws the unfinished code onto it, creating a visual representation of your project.
2. Interactive Code Sculptures
Take your unfinished projects to the next dimension by creating interactive sculptures that respond to viewer input or environmental factors.
Implementation Ideas:
- Use microcontrollers like Arduino to power physical representations of your code
- Create LED matrices that display snippets of your code based on sensor input
- Build kinetic sculptures that move or change based on the execution of your unfinished algorithms
Example: Arduino-powered LED Matrix
#include <Adafruit_NeoPixel.h>
#define PIN 6
#define NUMPIXELS 64
Adafruit_NeoPixel pixels(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
void setup() {
pixels.begin();
}
void loop() {
displayUnfinishedCode();
delay(5000);
}
void displayUnfinishedCode() {
// Simplified representation of code as light patterns
for(int i=0; i<NUMPIXELS; i++) {
if(i % 8 == 0) {
pixels.setPixelColor(i, pixels.Color(150, 0, 0)); // Red for function starts
} else if(i % 4 == 0) {
pixels.setPixelColor(i, pixels.Color(0, 150, 0)); // Green for variables
} else {
pixels.setPixelColor(i, pixels.Color(0, 0, 150)); // Blue for other code
}
pixels.show();
delay(50);
}
}
This Arduino sketch creates a simple light pattern on an LED matrix, representing different elements of your unfinished code.
3. Generative Art from Code Execution
Turn the execution of your unfinished projects into generative art. Even if the code doesn’t fully work, its partial execution can create interesting visual patterns.
Implementation Ideas:
- Use p5.js or Processing to visualize the flow of data through your algorithms
- Create abstract paintings based on the output of your unfinished functions
- Generate soundscapes from the execution patterns of your code
Example: p5.js Generative Art
<script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script>
<script>
function setup() {
createCanvas(800, 600);
background(220);
noLoop();
}
function draw() {
let unfinishedSort = [5, 2, 8, 1, 9, 3, 7, 6, 4];
for (let i = 0; i < unfinishedSort.length; i++) {
for (let j = 0; j < unfinishedSort.length - i - 1; j++) {
if (unfinishedSort[j] > unfinishedSort[j + 1]) {
// Swap elements
let temp = unfinishedSort[j];
unfinishedSort[j] = unfinishedSort[j + 1];
unfinishedSort[j + 1] = temp;
// Visualize the swap
stroke(random(255), random(255), random(255));
line(j * 80, height, (j + 1) * 80, 0);
}
}
}
}
</script>
This p5.js sketch creates an abstract visualization based on the partial execution of an unfinished sorting algorithm.
Conceptual Art and Code Philosophy
Beyond visual representations, your unfinished projects can serve as the basis for conceptual art pieces that explore the nature of software development, creativity, and technological progress.
1. The Museum of Abandoned Ideas
Create a virtual or physical space where visitors can explore your unfinished projects. Each project becomes an exhibit, complete with:
- The original concept and goals
- Snippets of the unfinished code
- Reasons for abandonment
- Potential future directions
This installation invites viewers to reflect on the creative process and the ephemeral nature of ideas in the fast-paced world of technology.
2. The Infinite Loop of Creation
Design an installation that represents the cyclical nature of coding projects. Use a circular display or projection where your unfinished projects flow in a never-ending loop, symbolizing the continuous cycle of ideation, creation, abandonment, and renewed inspiration.
3. Collaborative Unfinished Symphony
Turn your unfinished projects into a collaborative art piece by inviting other developers to contribute to or finish your code. This can be done through:
- A public GitHub repository where anyone can submit pull requests
- A physical installation where visitors can write suggestions or additional code on walls or screens
- A digital platform that allows real-time collaborative coding
This approach transforms your individual unfinished works into a community-driven exploration of collective creativity and problem-solving.
Technical Considerations for Code-Based Art Installations
As you embark on turning your unfinished projects into art installations, there are several technical aspects to consider:
1. Version Control as Artistic Timeline
Use Git or other version control systems not just as development tools, but as a way to showcase the evolution of your unfinished projects. Each commit becomes a snapshot in the artistic process.
Example: Git Log Visualization
<script>
// Simulated Git log data
const gitLog = [
{ hash: 'a1b2c3d', message: 'Initial commit', date: '2023-01-01' },
{ hash: 'e4f5g6h', message: 'Add main algorithm structure', date: '2023-01-15' },
{ hash: 'i7j8k9l', message: 'Implement partial sorting', date: '2023-02-01' },
{ hash: 'm0n1o2p', message: 'Fix edge case bug', date: '2023-02-15' },
{ hash: 'q3r4s5t', message: 'Abandon project', date: '2023-03-01' }
];
function createGitTimeline() {
const timeline = document.createElement('div');
timeline.style.position = 'relative';
timeline.style.height = '200px';
timeline.style.width = '100%';
gitLog.forEach((commit, index) => {
const node = document.createElement('div');
node.style.position = 'absolute';
node.style.left = `${index * 20}%`;
node.style.top = '50%';
node.style.width = '10px';
node.style.height = '10px';
node.style.borderRadius = '50%';
node.style.backgroundColor = '#333';
node.title = `${commit.hash}: ${commit.message} (${commit.date})`;
timeline.appendChild(node);
});
document.body.appendChild(timeline);
}
createGitTimeline();
</script>
This script creates a visual timeline of your project’s Git history, turning your development process into an artistic representation.
2. Cross-Platform Compatibility
Ensure your code-based art installations can run on various platforms. Consider using web technologies for maximum accessibility, or create platform-specific versions for more complex installations.
3. Performance Optimization
Even though your projects are unfinished, optimize them for performance in an art installation context. This might involve:
- Simplifying complex algorithms for visual effect
- Using efficient rendering techniques for large-scale displays
- Implementing lazy loading for installations with multiple projects
4. Interactivity and User Experience
Design your installations with user interaction in mind. This could include:
- Touch-sensitive displays that reveal code details
- Motion sensors that trigger different visualizations
- AR or VR elements that allow immersive exploration of your code
Example: Interactive Code Explorer
<div id="codeExplorer"></div>
<script>
const unfinishedProjects = [
{ name: 'Sorting Algorithm', code: 'function sort(arr) { /* TODO */ }' },
{ name: 'Neural Network', code: 'class NeuralNetwork { /* Unfinished */ }' },
{ name: 'Game Engine', code: 'const engine = { update: () => { /* Incomplete */ } }' }
];
function createCodeExplorer() {
const explorer = document.getElementById('codeExplorer');
unfinishedProjects.forEach(project => {
const projectElement = document.createElement('div');
projectElement.innerHTML = `<h3>${project.name}</h3><pre><code>${project.code}</code></pre>`;
projectElement.style.cursor = 'pointer';
projectElement.addEventListener('click', () => {
alert(`You've explored "${project.name}". Imagine the possibilities!`);
});
explorer.appendChild(projectElement);
});
}
createCodeExplorer();
</script>
This script creates an interactive display of your unfinished projects, allowing viewers to explore and engage with your code snippets.
Ethical Considerations in Code Art
As you transform your unfinished projects into art, it’s important to consider the ethical implications:
1. Intellectual Property
Ensure that you have the rights to display and use all code in your art installations. If you’ve used open-source libraries or collaborated with others, make sure to provide appropriate attribution and comply with licensing requirements.
2. Data Privacy
If your unfinished projects contain any user data or sensitive information, make sure to thoroughly anonymize or remove this data before including it in your art.
3. Accessibility
Design your installations with accessibility in mind. Provide alternative ways to experience your code art for those with visual, auditory, or mobility impairments.
4. Environmental Impact
For physical installations, consider the environmental impact of your materials and energy usage. Opt for sustainable options and energy-efficient technologies where possible.
Promoting Your Code Art
Once you’ve transformed your unfinished projects into art, it’s time to share your creations with the world:
1. Online Galleries
Create a dedicated website or use platforms like Behance or ArtStation to showcase your code art. Include detailed descriptions of your concept and process.
2. Social Media
Share your work on platforms like Instagram, Twitter, and LinkedIn. Use relevant hashtags like #CodeArt, #GenerativeArt, or #TechArt to reach interested audiences.
3. Tech and Art Conferences
Submit your work to conferences that focus on the intersection of technology and art, such as SIGGRAPH or Ars Electronica.
4. Collaborate with Galleries
Reach out to local art galleries or tech-focused exhibition spaces to explore opportunities for physical installations.
Conclusion: Embracing Imperfection in Code and Art
Transforming your unfinished coding projects into art installations is more than just a creative exercise – it’s a philosophical statement about the nature of creation, technology, and human ambition. By embracing the unfinished, we celebrate the journey of learning and creation that is at the heart of both coding and art.
Your abandoned repositories and half-implemented algorithms are not failures; they are stepping stones in your growth as a developer and now as an artist. They represent moments of inspiration, challenges faced, and paths not taken. By turning them into art, you invite others to reflect on the creative process and the ever-evolving landscape of technology.
So, the next time you feel discouraged by an unfinished project, remember: it might just be your next masterpiece waiting to be unveiled. Embrace the unfinished, for in its incompleteness lies infinite potential and beauty.
Now, go forth and transform your digital graveyards into galleries of inspiration!