The Hidden Connection Between LeetCode and Your Favorite Soap Opera
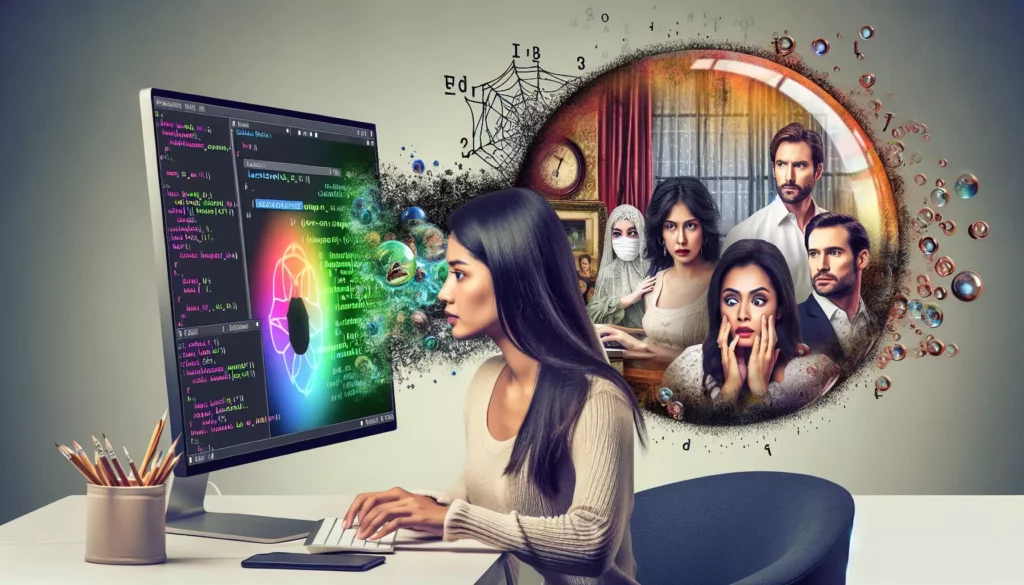
At first glance, LeetCode and soap operas might seem worlds apart. One is a platform for honing coding skills, while the other is a form of dramatic entertainment. However, as we delve deeper into these seemingly unrelated realms, we’ll uncover surprising parallels that not only entertain but also offer valuable insights for aspiring programmers. In this article, we’ll explore the unexpected connections between LeetCode challenges and the dramatic twists of your favorite soap operas, all while improving our coding skills and understanding of algorithmic thinking.
1. The Drama of Problem-Solving
Just as soap operas keep viewers on the edge of their seats with unexpected plot twists, LeetCode presents programmers with challenging problems that require creative solutions. Both experiences engage our problem-solving skills and keep us coming back for more.
Soap Opera Plot Twists
In a typical soap opera, you might encounter a scenario like this:
“Jessica discovers that her long-lost twin sister, whom she never knew existed, is actually married to her ex-boyfriend and is plotting to take over the family business.”
This kind of plot twist requires viewers to quickly process new information, reassess relationships, and anticipate potential outcomes – skills that are surprisingly similar to those needed in coding.
LeetCode Challenge Parallels
Now, let’s look at a LeetCode problem that mirrors this complexity:
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.
According to the definition of LCA on Wikipedia: "The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself)."
Just like unraveling the soap opera plot, solving this LeetCode challenge requires:
- Processing new information (the structure of the binary tree)
- Reassessing relationships (between nodes)
- Anticipating outcomes (finding the common ancestor)
Both scenarios engage our analytical thinking and problem-solving skills, albeit in different contexts.
2. Character Development and Code Refactoring
Soap opera characters often undergo significant transformations throughout a series. Similarly, our code evolves and improves as we refactor and optimize our solutions on LeetCode.
Character Arcs in Soap Operas
Consider this common soap opera trope:
“John, once a ruthless businessman, experiences a life-changing event that leads him to reevaluate his priorities and become a philanthropist.”
This character development arc shows growth, change, and improvement over time.
Code Refactoring in LeetCode
In LeetCode, we often start with a basic solution and then refactor it to improve efficiency. Let’s look at an example of solving the “Two Sum” problem:
Initial brute force solution:
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
for (int i = 0; i < nums.size(); i++) {
for (int j = i + 1; j < nums.size(); j++) {
if (nums[i] + nums[j] == target) {
return {i, j};
}
}
}
return {};
}
};
Refactored and optimized solution:
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> num_map;
for (int i = 0; i < nums.size(); i++) {
int complement = target - nums[i];
if (num_map.find(complement) != num_map.end()) {
return {num_map[complement], i};
}
num_map[nums[i]] = i;
}
return {};
}
};
Just as John’s character evolved, our code has transformed from a simple, brute-force approach to a more efficient, optimized solution using a hash map.
3. The Cliffhanger Effect
Soap operas are famous for their cliffhangers, leaving viewers in suspense and eager for the next episode. LeetCode challenges can create a similar effect, keeping programmers engaged and motivated to solve the next problem.
Soap Opera Cliffhangers
A typical soap opera cliffhanger might look like this:
“As Sarah walks down the aisle, ready to marry the love of her life, the church doors burst open to reveal her presumed-dead husband. The screen fades to black as gasps echo through the church.”
This cliffhanger creates suspense and a strong desire to know what happens next.
LeetCode’s “Just One More Problem” Effect
LeetCode creates a similar effect by presenting a series of related problems or by suggesting similar challenges after you complete one. For example, after solving the “Two Sum” problem, LeetCode might suggest:
- Three Sum
- Four Sum
- Two Sum II – Input Array Is Sorted
This progression of challenges keeps programmers engaged, always curious about the next problem and how it builds on their current knowledge.
4. Recurring Themes and Design Patterns
Soap operas often revisit familiar themes like love triangles, betrayal, and redemption. Similarly, LeetCode problems frequently incorporate common design patterns and algorithmic themes.
Soap Opera Recurring Themes
A classic soap opera theme might be:
“The wealthy heir falls in love with the girl from the wrong side of the tracks, facing disapproval from his family and societal pressures.”
This theme of forbidden love and class struggle is a staple in many soap operas, recurring with variations across different storylines.
LeetCode Design Patterns
In LeetCode, we often encounter recurring design patterns and problem-solving techniques. For example, the “sliding window” technique is a common pattern used in many problems. Let’s look at how it’s applied to the “Maximum Subarray” problem:
class Solution {
public:
int maxSubArray(vector<int>& nums) {
int max_sum = nums[0];
int current_sum = nums[0];
for (int i = 1; i < nums.size(); i++) {
current_sum = max(nums[i], current_sum + nums[i]);
max_sum = max(max_sum, current_sum);
}
return max_sum;
}
};
This sliding window approach can be applied to various other problems, such as:
- Longest Substring Without Repeating Characters
- Minimum Size Subarray Sum
- Longest Repeating Character Replacement
Recognizing these patterns in LeetCode problems is similar to identifying recurring themes in soap operas – it helps us approach new challenges with familiar strategies.
5. The Ensemble Cast and Data Structures
Soap operas are known for their large ensemble casts, with multiple characters interacting in complex ways. This mirrors the various data structures we use in programming, each with its own characteristics and interactions.
Soap Opera Ensemble
In a soap opera, you might have:
- The protagonist couple
- The scheming villain
- The wise elder
- The comic relief character
- Various supporting characters with their own subplots
Each character plays a specific role and interacts with others in unique ways to drive the story forward.
Data Structures in LeetCode
Similarly, in LeetCode problems, we work with various data structures, each serving a specific purpose:
- Arrays: The workhorses of many algorithms
- Linked Lists: For dynamic data management
- Trees: Hierarchical data representation
- Graphs: Complex relationship modeling
- Hash Tables: Fast data retrieval
Let’s look at how different data structures can be used to solve the same problem. Consider the “Valid Parentheses” problem:
Using a stack:
class Solution {
public:
bool isValid(string s) {
stack<char> st;
for (char c : s) {
if (c == '(' || c == '[' || c == '{') {
st.push(c);
} else {
if (st.empty()) return false;
if (c == ')' && st.top() != '(') return false;
if (c == ']' && st.top() != '[') return false;
if (c == '}' && st.top() != '{') return false;
st.pop();
}
}
return st.empty();
}
};
Using a hash map for faster matching:
class Solution {
public:
bool isValid(string s) {
stack<char> st;
unordered_map<char, char> matches = {{')', '('}, {']', '['}, {'}', '{'}};
for (char c : s) {
if (matches.find(c) == matches.end()) {
st.push(c);
} else {
if (st.empty() || st.top() != matches[c]) return false;
st.pop();
}
}
return st.empty();
}
};
Just as different characters in a soap opera ensemble interact to create a compelling story, various data structures in our code interact to create efficient solutions.
6. Time Complexity and Episode Runtime
In the world of algorithms, we often discuss time complexity – how the runtime of our code grows as the input size increases. This concept has an interesting parallel in the world of soap operas.
Soap Opera Episode Length
Soap operas come in various formats:
- Daily 30-minute episodes
- Weekly hour-long episodes
- Limited series with varying episode lengths
The length and frequency of episodes affect how much content can be covered and how quickly the plot progresses.
Time Complexity in LeetCode
In LeetCode, we strive to optimize our solutions for better time complexity. Let’s compare different time complexities using the “Merge Sort” algorithm as an example:
class Solution {
public:
void merge(vector<int>& nums, int left, int mid, int right) {
vector<int> temp(right - left + 1);
int i = left, j = mid + 1, k = 0;
while (i <= mid && j <= right) {
if (nums[i] <= nums[j]) {
temp[k++] = nums[i++];
} else {
temp[k++] = nums[j++];
}
}
while (i <= mid) {
temp[k++] = nums[i++];
}
while (j <= right) {
temp[k++] = nums[j++];
}
for (int p = 0; p < k; p++) {
nums[left + p] = temp[p];
}
}
void mergeSort(vector<int>& nums, int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(nums, left, mid);
mergeSort(nums, mid + 1, right);
merge(nums, left, mid, right);
}
}
vector<int> sortArray(vector<int>& nums) {
mergeSort(nums, 0, nums.size() - 1);
return nums;
}
};
Merge Sort has a time complexity of O(n log n), which is more efficient than simpler sorting algorithms like Bubble Sort (O(n²)) for large datasets.
Just as soap opera writers must balance story progression with episode length, programmers must balance algorithm efficiency with problem constraints.
7. Fan Theories and Algorithmic Intuition
Soap opera fans often develop theories about future plot developments based on subtle hints and character behaviors. Similarly, experienced programmers develop intuition about potential solutions to coding problems.
Soap Opera Fan Theories
A fan theory might look like this:
“I think Dr. Smith is actually the long-lost son of the hospital’s founder because he always looks wistfully at the founder’s portrait and has unexplained knowledge of the hospital’s history.”
Fans piece together clues to form these theories, much like how programmers approach problem-solving.
Algorithmic Intuition in LeetCode
When approaching a new LeetCode problem, experienced programmers often have intuitions about potential solutions. For example, when seeing a problem involving a sorted array, one might immediately think of binary search.
Let’s look at the “Search in Rotated Sorted Array” problem:
class Solution {
public:
int search(vector<int>& nums, int target) {
int left = 0, right = nums.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) {
return mid;
}
if (nums[left] <= nums[mid]) {
if (target >= nums[left] && target < nums[mid]) {
right = mid - 1;
} else {
left = mid + 1;
}
} else {
if (target > nums[mid] && target <= nums[right]) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
return -1;
}
};
An experienced programmer might intuit that this problem can be solved using a modified binary search, even though the array is rotated. This intuition, like fan theories, comes from recognizing patterns and applying previous knowledge to new situations.
8. Character Backstories and Code Documentation
Soap operas often reveal character backstories to add depth and context to current events. Similarly, good code documentation provides context and explains the reasoning behind certain implementations.
Soap Opera Backstories
A character backstory might be:
“Before becoming a successful lawyer, Amanda grew up in foster care, which drives her passion for child advocacy cases and explains her trust issues in relationships.”
This backstory provides context for the character’s actions and motivations in the current storyline.
Code Documentation in LeetCode
In LeetCode solutions, good documentation can explain the thought process and provide context for the implemented algorithm. Let’s look at a documented solution for the “Longest Palindromic Substring” problem:
class Solution {
public:
string longestPalindrome(string s) {
if (s.empty()) return "";
int start = 0, max_length = 1;
// Dynamic programming table
vector<vector<bool>> dp(s.length(), vector<bool>(s.length(), false));
// All substrings of length 1 are palindromes
for (int i = 0; i < s.length(); i++) {
dp[i][i] = true;
}
// Check for substrings of length 2
for (int i = 0; i < s.length() - 1; i++) {
if (s[i] == s[i+1]) {
dp[i][i+1] = true;
start = i;
max_length = 2;
}
}
// Check for substrings of length 3 or more
for (int len = 3; len <= s.length(); len++) {
for (int i = 0; i < s.length() - len + 1; i++) {
int j = i + len - 1;
if (s[i] == s[j] && dp[i+1][j-1]) {
dp[i][j] = true;
if (len > max_length) {
start = i;
max_length = len;
}
}
}
}
return s.substr(start, max_length);
}
};
This documented solution explains the dynamic programming approach, making it easier for others (or yourself in the future) to understand the logic behind the implementation.
9. Plot Twists and Edge Cases
Soap operas are known for their shocking plot twists that keep viewers engaged. In programming, edge cases serve a similar purpose, challenging our assumptions and testing the robustness of our solutions.
Soap Opera Plot Twists
A classic soap opera plot twist might be:
“It’s revealed that the kind, elderly neighbor who’s been helping the protagonist all along is actually the mastermind behind all the misfortunes that have befallen the town.”
This twist forces viewers to reevaluate everything they thought they knew about the story and its characters.
Edge Cases in LeetCode
In LeetCode problems, edge cases force us to reconsider our initial assumptions and make our solutions more robust. Let’s look at the “Reverse Integer” problem and how we need to handle edge cases:
class Solution {
public:
int reverse(int x) {
int reversed = 0;
while (x != 0) {
int pop = x % 10;
x /= 10;
// Check for overflow before updating reversed
if (reversed > INT_MAX/10 || (reversed == INT_MAX/10 && pop > 7)) return 0;
if (reversed < INT_MIN/10 || (reversed == INT_MIN/10 && pop < -8)) return 0;
reversed = reversed * 10 + pop;
}
return reversed;
}
};
In this solution, we need to handle the edge cases of integer overflow, which might not be immediately obvious when first approaching the problem. These edge cases, like plot twists, add complexity and depth to our coding challenges.
10. Series Finales and Comprehensive Testing
The series finale of a soap opera often ties up loose ends and provides closure to long-running storylines. Similarly, comprehensive testing in programming ensures that all aspects of our code work correctly and handles various scenarios.
Soap Opera Series Finale
A series finale might include:
- Resolving major conflicts
- Revealing final secrets
- Providing closure for character arcs
- Hinting at future possibilities
Comprehensive Testing in LeetCode
When solving LeetCode problems, thorough testing is crucial to ensure our solution works for all possible inputs. Let’s look at a comprehensive testing approach for the “Valid Palindrome” problem:
class Solution {
public:
bool isPalindrome(string s) {
int left = 0, right = s.length() - 1;
while (left < right) {
while (left < right && !isalnum(s[left])) left++;
while (left < right && !isalnum(s[right])) right--;
if (tolower(s[left]) != tolower(s[right])) {
return false;
}
left++;
right--;
}
return true;
}
};
// Comprehensive test cases
void runTests() {
Solution sol;
// Test case 1: Normal palindrome
assert(sol.isPalindrome("A man, a plan, a canal: Panama") == true);
// Test case 2: Non-palindrome
assert(sol.isPalindrome("race a car") == false);
// Test case 3: Empty string
assert(sol.isPalindrome("") == true);
// Test case 4: Single character
assert(sol.isPalindrome("a") == true);
// Test case 5: All non-alphanumeric characters
assert(sol.isPalindrome(" ") == true);
// Test case 6: Mixed case palindrome
assert(sol.isPalindrome("AbBa") == true);
// Test case 7: Palindrome with numbers
assert(sol.isPalindrome("12321") == true);
cout << "All test cases passed!" << endl;
}
This comprehensive testing approach, like a well-crafted series finale, ensures that all aspects of our solution are working correctly and provides confidence in its robustness.
Conclusion
As we’ve explored throughout this article, the world of LeetCode and the realm of soap operas share surprising parallels. From the drama of problem-solving to the importance of character development (or code refactoring), these two seemingly disparate domains offer valuable insights into each other.
By recognizing these connections, we can approach our coding challenges with renewed enthusiasm and creativity. The next time you’re stuck on a particularly tricky LeetCode problem, why not draw inspiration from your favorite soap opera? Consider the plot twists, character arcs, and dramatic revelations – you might just find the key to unlocking your next coding breakthrough.
Remember, whether you’re binge-watching a soap opera marathon or diving into a LeetCode coding session, you’re exercising your brain’s problem-solving muscles. So embrace the drama, anticipate the plot twists, and keep coding – your next big algorithm revelation might be just around the corner!