Why Your Code Editor is Your Therapist Now
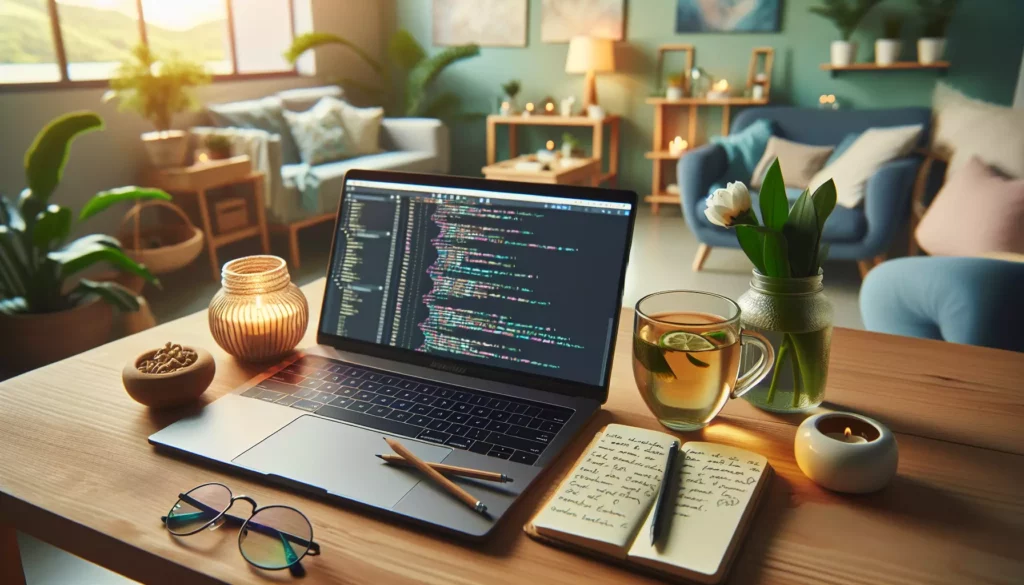
In the fast-paced world of software development, where deadlines loom and bugs seem to multiply faster than rabbits, it’s easy to feel overwhelmed. But what if I told you that the very tool you use to write code could also be your greatest ally in maintaining mental health and productivity? Welcome to the era where your code editor isn’t just a text processor—it’s your digital therapist.
The Evolution of Code Editors: From Text to Therapy
Gone are the days when code editors were mere text manipulation tools. Today’s integrated development environments (IDEs) and advanced code editors have evolved into sophisticated platforms that not only help you write better code but also support your overall well-being as a developer.
Smart Suggestions: A Confidence Boost
Modern code editors come equipped with intelligent code completion and suggestions. These features act like a supportive friend, always ready to lend a helping hand. When you’re stuck on syntax or can’t quite remember that method name, your editor whispers helpful hints, boosting your confidence and reducing frustration.
// Example of smart suggestions in action
function calculateArea(
// Your editor might suggest:
// length: number, width: number
)
This constant positive reinforcement can work wonders for your self-esteem as a programmer, especially when you’re tackling complex problems or learning a new language.
Error Highlighting: The Gentle Critic
Remember the days of compiling code only to be bombarded with a wall of error messages? Modern editors highlight errors in real-time, acting as a gentle critic that points out issues before they become major problems. This immediate feedback loop reduces anxiety and helps maintain a state of flow.
const greeting = "Hello, World!"
console.log(greting) // Your editor will likely underline 'greting' in red
By catching typos and syntax errors early, your editor helps prevent the buildup of stress that comes with debugging a large codebase.
The Zen of Code Formatting
One of the most therapeutic aspects of modern code editors is their ability to automatically format your code. This feature is like having a personal organizer for your digital workspace.
Auto-formatting: The Digital Declutterer
Just as a tidy room can lead to a tidy mind, well-formatted code can lead to clearer thinking. Auto-formatting tools built into editors like Visual Studio Code or plugins for Sublime Text can transform messy, inconsistent code into a beautifully structured masterpiece with just a keystroke.
// Before formatting
function messyFunction(a,b,c){
if(a>b){return c;}else{
return a+b;
}
}
// After formatting
function neatFunction(a, b, c) {
if (a > b) {
return c;
} else {
return a + b;
}
}
This instant transformation can be incredibly satisfying, providing a sense of order and control in the often chaotic world of coding.
Customizable Themes: Setting the Mood
Just as a therapist might use calming colors and comfortable furniture to create a soothing environment, your code editor allows you to customize its appearance to suit your mood and preferences. Whether you prefer a dark theme to reduce eye strain or a vibrant color scheme to energize your coding sessions, the right visual environment can significantly impact your mental state.
Popular themes like Monokai, Solarized, or Dracula aren’t just about aesthetics—they’re about creating a comfortable space where you can focus and feel at ease with your code.
The Power of Snippets: Affirmations in Code
Code snippets are like positive affirmations for developers. They allow you to quickly insert commonly used code patterns, reducing repetitive tasks and boosting productivity. But more than that, they serve as reminders that you have the tools and knowledge to tackle complex problems.
Creating Personal Snippets: Self-Empowerment
By creating your own snippets, you’re essentially building a personal library of coding wisdom. Each snippet represents a problem you’ve solved before, a concept you’ve mastered, or a best practice you’ve internalized.
// Example of a custom snippet for a React component
"React Functional Component": {
"prefix": "rfc",
"body": [
"import React from 'react';",
"",
"const ${1:ComponentName} = () => {",
" return (",
" <div>",
" $0",
" </div>",
" );",
"};",
"",
"export default ${1:ComponentName};"
],
"description": "Creates a React functional component"
}
Every time you use a snippet, it’s a small victory—a reminder of your growth and capabilities as a developer.
Version Control Integration: A Safety Net for Your Mind
The integration of version control systems like Git directly into code editors provides a psychological safety net that can significantly reduce coding anxiety.
Commit History: A Record of Progress
Being able to view your commit history right in your editor is like flipping through a journal of your coding journey. It’s a tangible record of problems solved, features implemented, and progress made. On tough days, this can serve as a powerful reminder of your capabilities and growth.
Branch Visualization: Mapping Your Thoughts
Many modern editors offer visual representations of Git branches. This feature isn’t just about managing code—it’s about organizing your thoughts and approaches to problem-solving. Seeing your branches laid out visually can help clarify your thinking and reduce the mental load of juggling multiple ideas or features.
AI-Powered Coding Assistants: Your Digital Rubber Duck
The latest evolution in code editors is the integration of AI-powered coding assistants. These tools, like GitHub Copilot or TabNine, act as a digital rubber duck—a concept in software engineering where explaining your code to an inanimate object (traditionally a rubber duck) can help you solve problems.
Code Suggestions: Brainstorming Partner
AI assistants can suggest entire functions or blocks of code based on comments or partial implementations. This feature isn’t just about saving time—it’s about stimulating your problem-solving skills and offering new perspectives on coding challenges.
// Example: Let AI suggest an implementation
// Function to calculate the Fibonacci sequence up to n terms
function fibonacci(n) {
// AI might suggest:
// let sequence = [0, 1];
// for (let i = 2; i < n; i++) {
// sequence[i] = sequence[i-1] + sequence[i-2];
// }
// return sequence;
}
These suggestions can serve as a starting point for your own implementations, reducing the anxiety of facing a blank screen.
Natural Language Processing: Explaining Code in Plain English
Some AI assistants can explain code in natural language or even generate code from natural language descriptions. This feature bridges the gap between your thoughts and the code, making the development process feel more intuitive and less intimidating.
Debugging Tools: Confronting Your Fears
Debugging is often seen as one of the most stressful aspects of programming. However, modern code editors turn debugging from a dreaded chore into an empowering problem-solving exercise.
Integrated Debuggers: Step-by-Step Problem Solving
Integrated debuggers allow you to step through your code line by line, inspect variables, and understand the flow of your program. This methodical approach to problem-solving can be incredibly calming, turning the chaotic process of bug hunting into a structured, manageable task.
Breakpoints and Watch Windows: Mindful Coding
Setting breakpoints and using watch windows to monitor variable values encourages a more mindful approach to coding. It slows you down, forcing you to consider each step of your program’s execution. This deliberate practice can lead to deeper understanding and, ultimately, more robust code.
Extension Ecosystems: Personalizing Your Digital Sanctuary
One of the most powerful aspects of modern code editors is their extensibility. The ability to add extensions turns your editor into a highly personalized tool tailored to your specific needs and preferences.
Productivity Extensions: Building Healthy Habits
Extensions like Pomodoro timers or habit trackers integrated directly into your editor can help you maintain a healthy work-life balance. These tools remind you to take breaks, stay hydrated, or even practice mindfulness between coding sessions.
Language-Specific Extensions: Confidence in Diversity
As you expand your coding repertoire, language-specific extensions can provide targeted support for each new language you learn. This tailored assistance can boost your confidence when venturing into unfamiliar territory, making the learning process less daunting.
Community Features: You’re Not Alone
Many modern code editors include features that connect you to the broader developer community, reminding you that you’re part of something bigger.
Live Share: Collaborative Problem-Solving
Features like Visual Studio Code’s Live Share allow for real-time collaborative coding. This can be incredibly reassuring, especially when you’re stuck on a problem. The ability to easily pair program or seek help from a colleague can significantly reduce feelings of isolation and frustration.
Extension Ratings and Reviews: Collective Wisdom
The ability to see ratings and reviews for extensions taps into the collective wisdom of the developer community. This feature not only helps you make informed decisions about which tools to use but also provides a sense of connection to other developers facing similar challenges.
The Mindful Coder: Embracing Your Editor as a Wellness Tool
As we’ve explored, modern code editors offer far more than just a place to write code. They provide a supportive environment that can significantly impact your mental well-being as a developer. By embracing your editor as a wellness tool, you can transform your coding experience from one of stress and anxiety to one of growth and satisfaction.
Customization is Self-Care
Take the time to customize your editor. Choose themes that soothe you, set up snippets that empower you, and select extensions that support your workflow. Remember, investing time in personalizing your digital environment is a form of self-care.
Embrace the Learning Process
Use features like AI assistants and integrated documentation not just as productivity tools, but as learning aids. Allow yourself to be curious about suggestions and explanations, turning every coding session into an opportunity for growth.
Practice Mindful Coding
Use debugging tools and version control not just to fix errors, but to deepen your understanding of your code. Approach each bug as a puzzle to be solved rather than a frustration to be endured. This mindset shift can transform debugging from a stressful experience into a rewarding one.
Connect and Collaborate
Don’t underestimate the power of community features. Reach out for help when you need it, and offer assistance to others when you can. Remember, every developer, no matter how experienced, was once a beginner.
Conclusion: Your Editor, Your Ally
In the demanding world of software development, your code editor stands as a silent ally, ready to support you through the ups and downs of your coding journey. From catching errors before they become problems to providing a personalized, soothing environment for your work, your editor is indeed your digital therapist.
As you continue to code, remember to leverage these powerful features not just for productivity, but for your mental well-being. Embrace your editor as a partner in your development process, and you may find that the challenges of coding become not just manageable, but enjoyable.
So the next time you open your code editor, take a moment to appreciate this sophisticated tool. It’s not just helping you write better code—it’s helping you become a more confident, balanced, and mindful developer. In the therapy session that is your coding workflow, your editor is always there, ready to listen, support, and guide you towards your best work.