The Secret Society of People Who Pretend to Understand Recursion
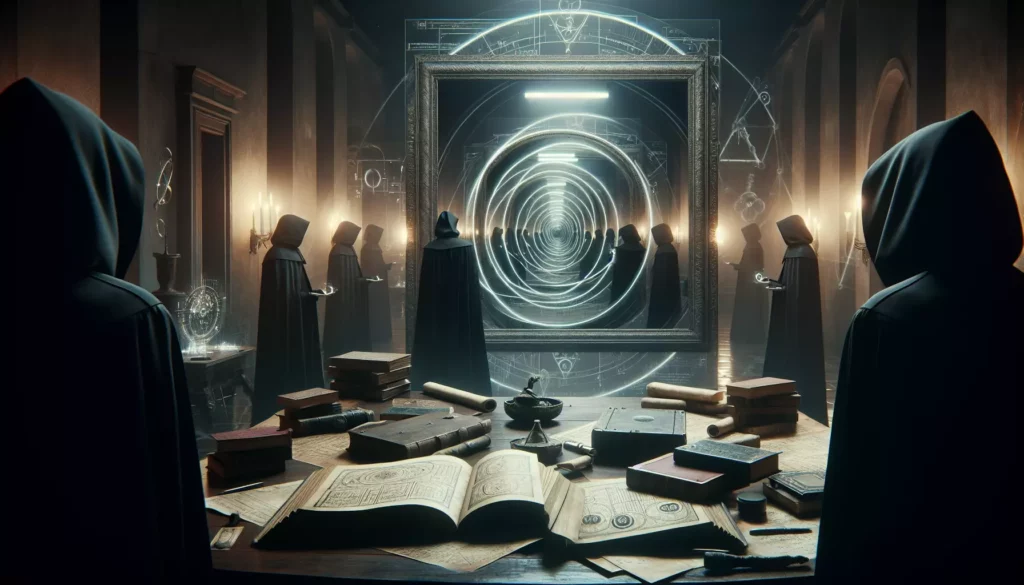
Welcome, fellow coders and aspiring programmers! Today, we’re diving into a topic that’s both fascinating and slightly intimidating: recursion. But don’t worry, we’re not here to explain it (because let’s face it, who really understands it?). Instead, we’re going to explore the secret society of people who pretend to understand recursion. So, put on your best “I totally get this” face, and let’s begin!
What is Recursion? (Don’t Worry, We’re All Faking It)
Before we delve into our secret society, let’s briefly touch on what recursion is supposed to be. In programming, recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It’s like a function that calls itself, which calls itself, which calls itself… until it doesn’t.
If that explanation made perfect sense to you, congratulations! You’re either a recursion genius or an excellent pretender. For the rest of us, let’s move on to the fun part.
The Initiation Ritual
To join the Secret Society of People Who Pretend to Understand Recursion, you must pass a rigorous initiation ritual. Here’s what it entails:
- Nod knowingly whenever someone mentions recursion.
- Use the phrase “It’s recursion all the way down” at least once in every coding conversation.
- Draw confusing diagrams on whiteboards that vaguely resemble trees or spirals.
- Memorize and recite the Fibonacci sequence (but only the first 8 numbers, we’re not monsters).
- Learn to say “base case” and “recursive case” with unwavering confidence.
Once you’ve mastered these skills, you’re ready to join our illustrious ranks!
The Art of Pretending
Now that you’re in, it’s crucial to maintain the illusion of understanding. Here are some tried-and-true techniques used by our most esteemed members:
1. The Thoughtful Pause
When someone asks you about recursion, pause for a moment, furrow your brow, and say, “Ah, recursion. It’s quite elegant, isn’t it?” This buys you time and makes you seem contemplative.
2. The Analogy Deflection
Comparing recursion to something completely unrelated is a great way to confuse everyone and avoid actual explanations. Try: “Recursion is like a Russian nesting doll, but the dolls are functions, and they’re all named Dave.”
3. The Code Snippet Bluff
Always have a recursive code snippet ready to show off. Here’s one you can use:
def pretend_to_understand_recursion(depth):
if depth == 0:
return "Base case reached!"
else:
return pretend_to_understand_recursion(depth - 1)
print(pretend_to_understand_recursion(5))
When asked to explain it, just say, “It’s pretty self-explanatory, don’t you think?”
4. The Philosophical Approach
Turn the conversation philosophical by saying something like, “But what is recursion, really? Isn’t life itself a recursive function, calling itself over and over until we reach the ultimate base case?”
5. The Meme Master
Keep a folder of recursion memes on your phone. When cornered, quickly pull one up and say, “This meme explains it perfectly!” Here’s a classic:
To understand recursion, you must first understand recursion.
Famous Members of Our Society
While we can’t name names (it’s a secret society, after all), here are some archetypes you might recognize:
- The “I Wrote a Recursive Function Once” Guy
- The “It’s Just Like Inception” Explainer
- The “Recursive Acronym” Enthusiast (GNU’s Not Unix, anyone?)
- The “I Dream in Recursive Structures” Programmer
- The “Tail Call Optimization” Name-Dropper
Real-World Applications (Or So We’ve Heard)
While we may not fully grasp recursion, it apparently has some real-world uses. Here are a few we’ve gathered from eavesdropping on actual computer scientists:
1. Fractal Generation
Those mesmerizing, self-similar patterns you see in nature and art? Apparently, they can be generated using recursive algorithms. When someone mentions this, just nod and say, “Ah yes, the Mandelbrot set. Fascinating.”
2. Tree Traversal
Something about binary trees and traversing them recursively. If pressed, mutter something about “in-order, pre-order, and post-order traversal” and quickly change the subject.
3. Divide and Conquer Algorithms
These algorithms supposedly break down problems into smaller subproblems. Mention “merge sort” or “quicksort” to sound knowledgeable, but avoid getting into specifics.
4. File System Navigation
Apparently, recursion is useful for navigating nested folder structures. If someone brings this up, say, “Oh yes, I use that all the time in my shell scripts!” (Note: Do not attempt to write said shell scripts.)
The Recursion Paradox
Here’s a mind-bending thought: What if our society is itself recursive? What if there’s a secret society within our secret society of people who pretend to understand the people who pretend to understand recursion? It’s pretenders all the way down!
Surviving Technical Interviews
As a member of our esteemed society, you might find yourself in a technical interview where recursion comes up. Fear not! Here are some strategies to help you bluff your way through:
1. The Whiteboard Warrior
If asked to solve a problem using recursion, approach the whiteboard with confidence. Draw a large tree-like structure, add some arrows pointing in various directions, and sprinkle in a few function calls. The messier it looks, the more convincing it appears!
2. The Time Complexity Tangent
When discussing the efficiency of a recursive solution, throw in some big O notation. Say something like, “Well, the time complexity here is O(n), but we could optimize it to O(log n) with memoization.” (Don’t worry if you don’t know what memoization is – neither do we!)
3. The Stack Overflow Excuse
If your recursive solution doesn’t quite work, blame it on potential stack overflow issues. Say, “Of course, we’d need to consider the call stack depth here. In production, we might want to use an iterative approach to avoid stack overflow.”
4. The Tail Recursion Gambit
Mention tail recursion optimization as a way to improve your solution. It doesn’t matter if it applies or not – most interviewers will be impressed that you brought it up.
Famous Recursive Algorithms (That We Pretend to Understand)
To really sell your membership in our society, it’s crucial to have a few famous recursive algorithms up your sleeve. Here are some you can name-drop with confidence:
1. The Fibonacci Sequence
This is the classic example of recursion. The sequence goes 0, 1, 1, 2, 3, 5, 8, 13, and so on, where each number is the sum of the two preceding ones. The recursive function might look something like this:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
Pro tip: Mention that while this is a great example of recursion, it’s terribly inefficient and should never be used in production code. This shows you’re both knowledgeable and practical!
2. The Tower of Hanoi
This classic puzzle involves moving a stack of disks from one peg to another, with some specific rules. It’s often solved recursively, but don’t worry about understanding how. Just say, “Ah, the Tower of Hanoi. A beautiful demonstration of recursive problem-solving!”
3. Quicksort
Quicksort is a divide-and-conquer algorithm that uses recursion to sort an array. If someone asks you about it, just say, “Quicksort? Oh yes, it’s recursive nature makes it efficient for large datasets, but we need to be careful about choosing the right pivot to avoid worst-case scenarios.”
The Recursive Joke Section
No discussion of recursion would be complete without some recursive humor. Here are a few jokes to add to your repertoire:
- To understand recursion, see joke #1.
- Why do programmers prefer dark mode? Because light attracts bugs!
- How do you explain recursion to a child? First, explain it to an adult…
- What’s the best way to understand recursion? [This punchline intentionally left recursive]
Remember, the key to selling these jokes is to laugh knowingly, even if you don’t quite get them. It’s all part of the act!
The Recursion Support Group
Even the best pretenders sometimes need support. That’s why we’ve established the Recursion Support Group (RSG). The first rule of RSG is: you do not talk about understanding recursion. The second rule of RSG is: you DO NOT talk about understanding recursion.
In these meetings, members can share their close calls and near-exposures. It’s a safe space to admit that you once confused recursion with iteration, or that you secretly think loops are easier to understand.
Advanced Pretending Techniques
For those who want to take their pretending to the next level, here are some advanced techniques:
1. The Recursive Lambda
Casually mention that you prefer using lambda functions for recursion. Throw out this mind-bender:
factorial = lambda n: 1 if n == 0 else n * factorial(n-1)
Follow it up with, “It’s so elegant, don’t you think?” before anyone can ask you to explain it.
2. The Mutual Recursion Drop
Bring up mutual recursion in conversation. It’s when two or more functions call each other recursively. Don’t worry about the details; just say, “I find mutual recursion particularly elegant for certain problems.”
3. The Recursion Scheme Scholar
Mention “recursion schemes” and watch as even experienced developers start to sweat. Talk about catamorphisms, anamorphisms, and hylomorphisms with a straight face. If anyone asks for clarification, just say, “It’s a bit too complex to get into right now.”
The Ethical Dilemma
As members of this secret society, we must occasionally grapple with the ethics of our actions. Are we doing a disservice to the programming community by perpetuating this charade? Should we come clean and admit our lack of understanding?
The answer, of course, is a resounding “No!” Our society serves a crucial purpose: it helps maintain the mystique and allure of recursion. Without us, recursion might lose its status as one of programming’s most enigmatic concepts. We’re not just pretenders; we’re guardians of programming’s great mysteries!
Conclusion: The Recursive Nature of Understanding
As we wrap up our journey through the Secret Society of People Who Pretend to Understand Recursion, let’s reflect on what we’ve learned. Or rather, what we’ve pretended to learn.
Remember, understanding recursion is like understanding life itself – just when you think you’ve got it figured out, you realize there’s another layer of complexity waiting to be unraveled. So keep pretending, keep nodding knowingly, and keep drawing those confusing diagrams on whiteboards.
And if you ever find yourself truly understanding recursion? Well, then it’s time to start pretending that you don’t. After all, that’s the most recursive thing you can do!
Until next time, keep recursing… or at least keep pretending to!
Bonus: The Ultimate Recursive Function
We’ll leave you with this ultimate recursive function. It perfectly encapsulates everything we’ve discussed today:
def understand_recursion():
try:
return understand_recursion()
except RecursionError:
return "I understand recursion!"
print(understand_recursion())
Remember, in the world of recursion, it’s not about the destination; it’s about the journey. A journey that, much like this article, seems to go on forever until it suddenly doesn’t. Happy pretending!