The LeetCode Drinking Game: Take a Shot Every Time You Use a Brute Force Solution
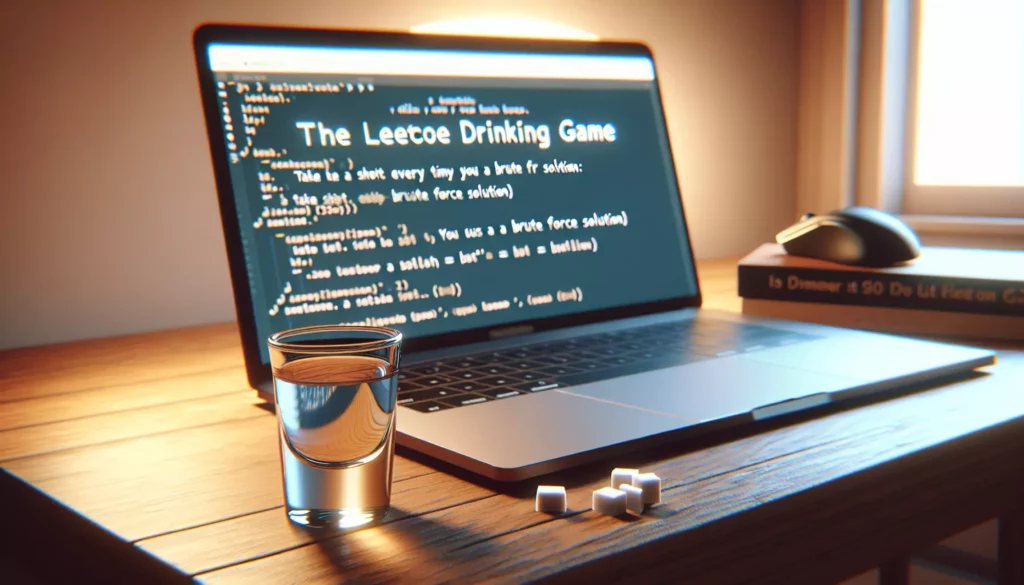
Welcome to the wild world of coding challenges, where frustration meets fun, and algorithms dance with alcohol! Today, we’re diving into a topic that’s sure to make your coding sessions a little more… spirited. Get ready for “The LeetCode Drinking Game: Take a Shot Every Time You Use a Brute Force Solution.” But before we crack open that bottle, let’s set the stage for why this game exists and why it’s more than just a humorous take on coding challenges.
The Rise of LeetCode and Coding Challenges
In recent years, platforms like LeetCode have become the go-to resource for aspiring software engineers and seasoned professionals alike. These platforms offer a vast array of coding problems, ranging from easy warm-ups to brain-melting hard challenges. They’ve become an integral part of the tech industry, often serving as a benchmark for technical interviews at major companies like Google, Facebook, Amazon, Apple, and Netflix (collectively known as FAANG).
The popularity of these platforms stems from their ability to:
- Sharpen algorithmic thinking skills
- Improve problem-solving abilities
- Prepare candidates for technical interviews
- Provide a standardized way to assess coding proficiency
However, with great popularity comes great pressure. Many developers find themselves spending countless hours grinding through problems, often resorting to brute force solutions when elegant algorithms elude them. And that’s where our drinking game comes in – a lighthearted way to acknowledge the struggles and triumphs of the coding challenge journey.
Understanding Brute Force Solutions
Before we dive into the game rules, let’s clarify what we mean by a “brute force solution.” In computer science, a brute force algorithm is a problem-solving technique that enumerates all possible candidates for the solution and checks whether each candidate satisfies the problem statement.
Characteristics of brute force solutions include:
- Simplicity in implementation
- Guaranteed to find a solution if it exists
- Often inefficient for large inputs
- Typically have higher time complexity (e.g., O(n^2), O(n!), etc.)
While brute force solutions are valid and sometimes necessary, they’re often frowned upon in coding interviews and efficiency-focused environments. The goal is usually to find more optimized solutions using data structures and algorithms that reduce time and space complexity.
The LeetCode Drinking Game Rules
Now, let’s get to the fun part – the rules of our LeetCode Drinking Game. Remember, this is all in good fun, and we absolutely do not endorse excessive drinking. Please drink responsibly, or better yet, use non-alcoholic alternatives!
- Take a shot when:
- You implement a brute force solution for a medium or hard problem
- Your solution exceeds the time limit due to inefficiency
- You use nested loops when a more efficient data structure would suffice
- You realize you’ve been staring at the problem description for over 15 minutes without writing a single line of code
- Take two shots when:
- You submit a brute force solution and it actually passes all test cases
- You spend an hour optimizing your solution only to find out the brute force approach was accepted
- You use a HashMap when the problem clearly hints at using a different data structure
- Finish your drink when:
- You give up on a problem and check the solution, only to find out it was a one-liner
- You accidentally solve a hard problem with an optimal solution on your first try
- You realize you’ve been solving the wrong problem for the last hour
Remember, the goal here isn’t to encourage reckless behavior, but to add a touch of humor to the often frustrating process of solving coding challenges. It’s a way to laugh at our mistakes and celebrate our small victories along the way.
The Importance of Efficient Solutions
While our drinking game pokes fun at brute force solutions, it’s crucial to understand why efficient solutions matter. In the real world of software development, efficiency can make or break an application. Here’s why optimized solutions are essential:
- Scalability: Efficient algorithms allow your applications to handle larger datasets without significant performance degradation.
- User Experience: Faster algorithms lead to quicker response times, enhancing the overall user experience.
- Resource Management: Optimized code uses fewer computational resources, reducing costs in cloud environments and improving battery life on mobile devices.
- Interview Success: Demonstrating the ability to optimize solutions shows problem-solving skills and deep understanding of data structures and algorithms.
Let’s look at a simple example to illustrate the difference between a brute force approach and an optimized solution.
Example: Two Sum Problem
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Brute Force Solution:
class Solution {
public int[] twoSum(int[] nums, int target) {
for (int i = 0; i < nums.length; i++) {
for (int j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] == target) {
return new int[] { i, j };
}
}
}
return new int[] {};
}
}
This solution has a time complexity of O(n^2) due to the nested loops.
Optimized Solution using HashMap:
class Solution {
public int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
return new int[] {};
}
}
This solution has a time complexity of O(n) and demonstrates how using appropriate data structures can significantly improve efficiency.
Strategies to Avoid Brute Force Solutions
Now that we’ve had our fun with the drinking game and understood the importance of efficient solutions, let’s explore some strategies to help you avoid relying on brute force approaches:
- Identify Patterns: Many LeetCode problems follow common patterns. Recognizing these can help you apply the right algorithm or data structure.
- Master Core Data Structures: Become proficient with arrays, linked lists, trees, graphs, stacks, queues, and hash tables. Understanding their strengths and use cases is crucial.
- Learn Algorithm Paradigms: Familiarize yourself with divide and conquer, dynamic programming, greedy algorithms, and backtracking. These paradigms often lead to more efficient solutions.
- Practice Time and Space Complexity Analysis: Being able to quickly analyze the efficiency of your solution can help you identify when you need to optimize.
- Start with a Brute Force Approach, Then Optimize: Sometimes, implementing a brute force solution can help you understand the problem better, making it easier to see optimization opportunities.
- Use Problem-Solving Frameworks: Techniques like the UMPIRE method (Understand, Match, Plan, Implement, Review, Evaluate) can guide you towards more efficient solutions.
The Role of AlgoCademy in Improving Your Skills
While our LeetCode Drinking Game is all in good fun, platforms like AlgoCademy offer a more structured and educational approach to mastering coding challenges. AlgoCademy focuses on:
- Interactive Tutorials: Step-by-step guidance through various coding concepts and problem-solving techniques.
- AI-Powered Assistance: Get personalized hints and explanations when you’re stuck on a problem.
- Comprehensive Problem Sets: A curated collection of problems that cover various difficulty levels and topics.
- Progress Tracking: Monitor your improvement over time and identify areas that need more focus.
- Interview Preparation: Tailored content to help you ace technical interviews at top tech companies.
By leveraging platforms like AlgoCademy, you can develop a systematic approach to problem-solving, reducing your reliance on brute force solutions and improving your overall coding skills.
Balancing Fun and Learning in Coding Challenges
While our LeetCode Drinking Game adds a humorous twist to coding challenges, it’s essential to maintain a balance between fun and serious learning. Here are some tips to keep your coding journey enjoyable and productive:
- Set Realistic Goals: Don’t aim to solve 50 problems in a day. Start with a manageable number and gradually increase as you improve.
- Celebrate Small Wins: Solved a problem without looking at the solution? That’s worth celebrating (responsibly, of course)!
- Join Coding Communities: Platforms like Reddit’s r/leetcode or Discord coding servers can provide support, motivation, and a sense of camaraderie.
- Take Breaks: Coding fatigue is real. Remember to step away from the screen regularly to refresh your mind.
- Reflect on Your Progress: Regularly review your solved problems and the techniques you’ve learned. This reinforces your knowledge and boosts confidence.
- Apply Your Skills to Real Projects: Don’t just solve isolated problems. Try to apply the algorithms and data structures you learn to personal or open-source projects.
The Ethical Considerations of Coding Challenges
As we navigate the world of coding challenges and our playful drinking game, it’s important to address some ethical considerations:
- Interview Fairness: The prevalence of platforms like LeetCode has led to debates about the fairness of using these types of problems in technical interviews. Critics argue that they may not accurately reflect real-world coding skills.
- Accessibility: Not everyone has the time or resources to dedicate to extensive LeetCode practice, which can create disparities in job opportunities.
- Mental Health: The pressure to excel at coding challenges can lead to stress and burnout. It’s crucial to maintain a healthy perspective and balance.
- Diversity in Tech: The focus on algorithm-heavy interviews may inadvertently favor certain educational backgrounds or learning styles, potentially impacting diversity in the tech industry.
As aspiring or professional developers, it’s important to be aware of these issues and contribute to discussions about creating more equitable and comprehensive assessment methods in the tech industry.
Conclusion: Embracing the Journey of Continuous Learning
As we wrap up our exploration of “The LeetCode Drinking Game: Take a Shot Every Time You Use a Brute Force Solution,” let’s remember that the journey of becoming a proficient coder is filled with challenges, frustrations, and moments of triumph. While our drinking game adds a touch of humor to the process, the real goal is continuous improvement and learning.
Coding challenges, whether on LeetCode, AlgoCademy, or other platforms, are valuable tools for honing your skills, preparing for interviews, and deepening your understanding of computer science concepts. The key is to approach them with a growth mindset, seeing each problem as an opportunity to learn rather than a test to pass or fail.
Remember these key takeaways:
- Brute force solutions are a starting point, not the end goal. Strive to optimize and learn from each problem you encounter.
- Efficient algorithms and data structures are crucial for scalable, performant software. Invest time in mastering them.
- Utilize resources like AlgoCademy to structure your learning and get personalized guidance.
- Balance serious study with fun and community engagement to maintain motivation and prevent burnout.
- Stay aware of the broader implications and ethical considerations surrounding coding challenges and technical interviews.
Whether you’re just starting your coding journey or you’re a seasoned professional, remember that every great developer was once a beginner. Embrace the challenges, celebrate your progress, and don’t be afraid to laugh at yourself along the way. And who knows? Maybe the next time you encounter a particularly tricky problem, you’ll raise a glass (responsibly) to the journey of continuous learning and improvement in the fascinating world of coding.
Happy coding, and may your algorithms be ever efficient!