Building a Web App as a Portfolio Project: Step-by-Step Guide
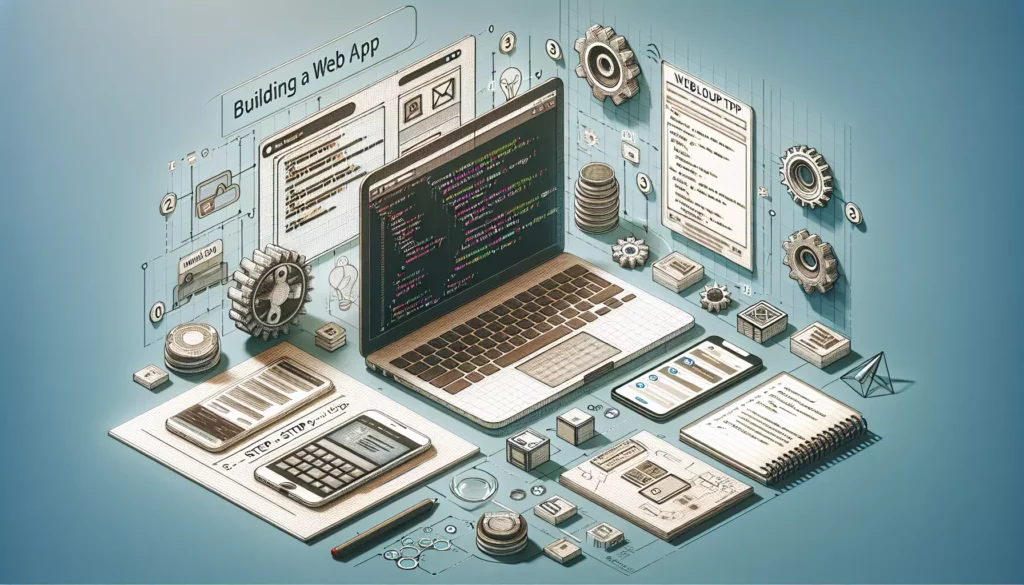
In today’s competitive tech landscape, having a strong portfolio is crucial for aspiring developers. One of the most effective ways to showcase your skills and stand out to potential employers is by building a web application from scratch. This comprehensive guide will walk you through the process of creating a web app as a portfolio project, covering everything from planning and design to deployment and maintenance.
Table of Contents
- Planning Your Web App
- Designing the User Interface
- Developing the Frontend
- Building the Backend
- Implementing the Database
- Testing and Debugging
- Deploying Your Web App
- Maintenance and Updates
- Showcasing Your Project
- Conclusion
1. Planning Your Web App
Before diving into coding, it’s essential to have a clear plan for your web app. This stage involves defining your project’s purpose, target audience, and core features.
Define Your Project’s Purpose
Start by answering these questions:
- What problem does your web app solve?
- Who is your target audience?
- What are the main features and functionalities?
Choose Your Tech Stack
Select the technologies you’ll use for your project. Consider factors like your existing skills, learning goals, and project requirements. A typical full-stack web app might include:
- Frontend: HTML, CSS, JavaScript (React, Vue, or Angular)
- Backend: Node.js, Python (Django or Flask), Ruby on Rails, or Java (Spring)
- Database: MySQL, PostgreSQL, MongoDB, or Firebase
Create a Project Roadmap
Break down your project into smaller, manageable tasks. Use project management tools like Trello or GitHub Projects to organize your workflow.
2. Designing the User Interface
A well-designed user interface (UI) is crucial for a successful web app. Follow these steps to create an appealing and user-friendly design:
Wireframing
Start by creating low-fidelity wireframes to outline the basic structure of your app. Tools like Balsamiq or Figma can help you quickly sketch out your ideas.
Mockups and Prototypes
Create high-fidelity mockups to visualize the final look of your app. Use tools like Adobe XD, Sketch, or Figma to design detailed mockups and interactive prototypes.
Design System
Develop a consistent design system, including:
- Color palette
- Typography
- Icons and illustrations
- Spacing and layout guidelines
3. Developing the Frontend
With your design in place, it’s time to bring your web app to life by developing the frontend.
Set Up Your Development Environment
Install the necessary tools and frameworks for your chosen tech stack. For a React-based project, you might use:
- Node.js and npm
- Create React App or Next.js
- Code editor (e.g., Visual Studio Code)
- Git for version control
Implement the UI Components
Start by creating reusable UI components based on your design system. For a React project, this might include:
<!-- Example of a Button component in React -->
import React from 'react';
const Button = ({ text, onClick, variant = 'primary' }) => {
return (
<button
className={`btn btn-${variant}`}
onClick={onClick}
>
{text}
</button>
);
};
export default Button;
Implement State Management
Choose a state management solution appropriate for your project’s complexity. Options include:
- React’s built-in useState and useContext hooks for simpler apps
- Redux or MobX for more complex state management
Handle Routing
Implement client-side routing to create a smooth, single-page application experience. For React, you can use React Router:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
}
4. Building the Backend
The backend of your web app handles data processing, business logic, and communication with the database. Here’s how to approach backend development:
Choose a Backend Framework
Select a backend framework that aligns with your chosen programming language. Popular options include:
- Node.js with Express.js
- Python with Django or Flask
- Ruby on Rails
- Java with Spring Boot
Set Up API Endpoints
Design and implement RESTful API endpoints for your web app. For an Express.js backend, this might look like:
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// Logic to fetch users from database
res.json({ users: [] });
});
app.post('/api/users', (req, res) => {
// Logic to create a new user
res.status(201).json({ message: 'User created successfully' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Implement Authentication and Authorization
Secure your web app by implementing user authentication and authorization. Consider using industry-standard protocols like OAuth 2.0 or JSON Web Tokens (JWT).
Handle Data Validation and Error Handling
Implement robust data validation and error handling to ensure your app’s reliability and security. Use libraries like Joi or express-validator for input validation.
5. Implementing the Database
Choosing and implementing the right database is crucial for storing and managing your web app’s data efficiently.
Select a Database System
Choose a database system that fits your project requirements:
- Relational databases: MySQL, PostgreSQL
- NoSQL databases: MongoDB, Cassandra
- Cloud-based solutions: Firebase Realtime Database, Amazon DynamoDB
Design the Database Schema
Create a well-structured database schema that efficiently represents your data model. For a relational database, this involves designing tables and relationships. For NoSQL databases, focus on optimizing data access patterns.
Implement Database Operations
Develop functions or methods to perform CRUD (Create, Read, Update, Delete) operations on your database. Here’s an example using Mongoose with MongoDB:
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
email: String,
password: String
});
const User = mongoose.model('User', userSchema);
// Create a new user
async function createUser(userData) {
const user = new User(userData);
await user.save();
return user;
}
// Fetch all users
async function getAllUsers() {
return await User.find();
}
// Update a user
async function updateUser(id, updateData) {
return await User.findByIdAndUpdate(id, updateData, { new: true });
}
// Delete a user
async function deleteUser(id) {
return await User.findByIdAndDelete(id);
}
6. Testing and Debugging
Thorough testing is essential to ensure your web app functions correctly and provides a smooth user experience.
Unit Testing
Write unit tests for individual components and functions. Use testing frameworks like Jest for JavaScript or pytest for Python. Here’s an example of a simple Jest test:
import { sum } from './math';
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Integration Testing
Perform integration tests to ensure different parts of your application work together correctly. Tools like Supertest can help test your API endpoints:
const request = require('supertest');
const app = require('../app');
describe('GET /api/users', () => {
it('responds with json containing a list of users', async () => {
const response = await request(app)
.get('/api/users')
.expect('Content-Type', /json/)
.expect(200);
expect(response.body).toHaveProperty('users');
expect(Array.isArray(response.body.users)).toBeTruthy();
});
});
End-to-End Testing
Conduct end-to-end tests to simulate real user interactions with your web app. Tools like Cypress or Selenium can help automate these tests.
Debugging Techniques
Familiarize yourself with debugging tools and techniques:
- Use browser developer tools for frontend debugging
- Implement logging in your backend code
- Use debugging features in your IDE or code editor
7. Deploying Your Web App
Once your web app is tested and ready, it’s time to deploy it to a production environment.
Choose a Hosting Platform
Select a hosting platform that suits your needs and budget. Popular options include:
- Heroku: Easy deployment for various technologies
- Netlify or Vercel: Great for static sites and JAMstack apps
- Amazon Web Services (AWS): Scalable and feature-rich cloud platform
- DigitalOcean: Developer-friendly cloud infrastructure
Set Up Continuous Integration and Deployment (CI/CD)
Implement a CI/CD pipeline to automate your deployment process. Tools like GitHub Actions, GitLab CI, or Jenkins can help you achieve this.
Configure Environment Variables
Ensure sensitive information like API keys and database credentials are stored as environment variables, not in your source code.
Monitor Your Deployed App
Set up monitoring and logging tools to track your app’s performance and quickly identify issues. Consider using services like New Relic, Datadog, or ELK stack (Elasticsearch, Logstash, Kibana).
8. Maintenance and Updates
Maintaining and updating your web app is an ongoing process that ensures its continued functionality and relevance.
Regular Code Reviews
Conduct regular code reviews to maintain code quality and identify potential improvements or security vulnerabilities.
Performance Optimization
Continuously monitor and optimize your app’s performance:
- Implement caching strategies
- Optimize database queries
- Minimize and compress frontend assets
Security Updates
Stay informed about security vulnerabilities in your dependencies and apply updates promptly. Use tools like npm audit or Dependabot to automate this process.
Feature Enhancements
Based on user feedback and changing requirements, plan and implement new features or improvements to existing functionality.
9. Showcasing Your Project
Your portfolio project is complete! Now it’s time to showcase your work effectively to potential employers or clients.
Create a Compelling README
Write a detailed README file for your project repository, including:
- Project overview and features
- Technologies used
- Installation and setup instructions
- Screenshots or demo videos
- Future improvements or known issues
Deploy a Live Demo
Ensure your web app is accessible online for easy demonstration. Include the live demo link in your project documentation and portfolio.
Document Your Process
Consider writing a blog post or creating a video explaining your development process, challenges faced, and lessons learned. This demonstrates your problem-solving skills and ability to communicate technical concepts.
Update Your Portfolio
Add your web app project to your portfolio website or professional profiles (LinkedIn, GitHub, etc.), highlighting key features and your role in its development.
10. Conclusion
Building a web app as a portfolio project is an excellent way to showcase your skills, learn new technologies, and stand out in the competitive tech industry. By following this comprehensive guide, you’ll not only create a functional web application but also gain valuable experience in the entire software development lifecycle.
Remember that the process of building a web app is iterative and continuous. Don’t be afraid to start small and gradually add features and improvements. Each step of the process, from planning to deployment and maintenance, offers opportunities to learn and grow as a developer.
As you work on your project, consider the following tips to maximize its impact on your portfolio:
- Focus on solving a real problem or addressing a specific need in your chosen domain.
- Pay attention to code quality, documentation, and best practices throughout the development process.
- Seek feedback from peers, mentors, or online communities to improve your project and skills.
- Be prepared to discuss your project in detail during job interviews, highlighting your decision-making process and problem-solving approach.
- Continue learning and staying updated with the latest trends and technologies in web development.
By investing time and effort into building a high-quality web app portfolio project, you’re not just creating a showcase of your technical skills – you’re also demonstrating your ability to manage a project, solve complex problems, and deliver a polished product. These are all valuable qualities that employers look for in potential candidates.
So, roll up your sleeves, start planning your web app, and embark on this exciting journey of creating a standout portfolio project. With dedication and perseverance, you’ll not only enhance your skills but also open doors to new opportunities in your development career.