Why You Should Consider Learning WebAssembly in 2024
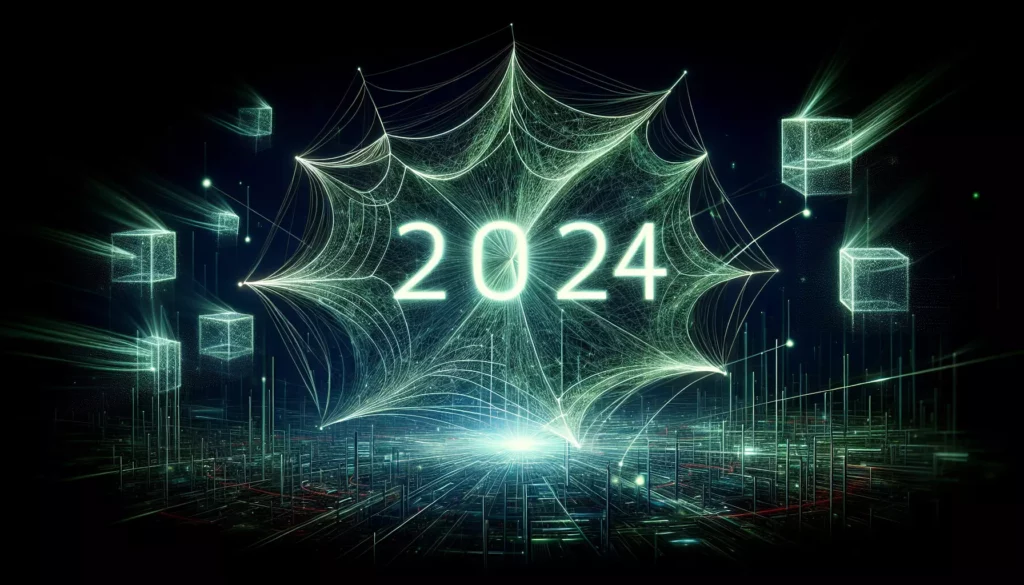
As we approach 2024, the landscape of web development continues to evolve at a rapid pace. Among the emerging technologies that are gaining significant traction, WebAssembly (often abbreviated as Wasm) stands out as a game-changer. If you’re a developer looking to stay ahead of the curve or a coding enthusiast eager to expand your skillset, learning WebAssembly should be high on your priority list. In this comprehensive guide, we’ll explore the reasons why WebAssembly is becoming increasingly important and how it can benefit your career in the coming years.
What is WebAssembly?
Before diving into the reasons to learn WebAssembly, let’s briefly explain what it is. WebAssembly is a low-level, binary instruction format designed for efficient execution in web browsers. It’s not meant to be written by hand but rather to be a compilation target for languages like C, C++, and Rust. WebAssembly aims to enable near-native performance for web applications, opening up new possibilities for complex computations and high-performance tasks in the browser.
1. Performance Boost for Web Applications
One of the primary reasons to consider learning WebAssembly is the significant performance improvements it can bring to web applications. Traditional JavaScript, while versatile and widely used, can sometimes struggle with computationally intensive tasks. WebAssembly, on the other hand, offers near-native speed, allowing developers to write high-performance code that runs efficiently in the browser.
For example, consider a complex algorithm for image processing or 3D rendering. Implementing such algorithms in JavaScript might lead to noticeable lag or poor user experience. With WebAssembly, you can compile these performance-critical parts of your application to run at near-native speeds, providing a smoother and more responsive user experience.
Code Example: Performance Comparison
Here’s a simple example comparing the performance of a factorial calculation in JavaScript versus WebAssembly (compiled from C++):
// JavaScript implementation
function factorialJS(n) {
if (n === 0 || n === 1) return 1;
return n * factorialJS(n - 1);
}
// WebAssembly implementation (C++ code compiled to Wasm)
int factorial(int n) {
if (n == 0 || n == 1) return 1;
return n * factorial(n - 1);
}
// Usage and performance measurement
console.time('JavaScript');
for (let i = 0; i < 1000000; i++) {
factorialJS(20);
}
console.timeEnd('JavaScript');
console.time('WebAssembly');
for (let i = 0; i < 1000000; i++) {
Module.ccall('factorial', 'number', ['number'], [20]);
}
console.timeEnd('WebAssembly');
In this example, you’d likely see the WebAssembly version outperform the JavaScript version, especially for larger numbers or more complex calculations.
2. Cross-Language Development
Another compelling reason to learn WebAssembly is its ability to bring other programming languages to the web. Traditionally, web development has been dominated by JavaScript. While JavaScript is powerful and versatile, it may not always be the best tool for every job. WebAssembly allows developers to use languages like C, C++, Rust, and others to write parts of their web applications.
This cross-language capability opens up several advantages:
- Reuse existing codebases: You can port existing applications or libraries written in other languages to the web.
- Leverage language-specific strengths: Different languages excel at different tasks. WebAssembly allows you to use the best language for each part of your application.
- Expand your skillset: Learning WebAssembly encourages you to become proficient in multiple programming languages, making you a more versatile developer.
Code Example: Using Rust with WebAssembly
Here’s a simple example of how you might use Rust code compiled to WebAssembly in a web application:
// Rust code (lib.rs)
#[no_mangle]
pub extern "C" fn add(a: i32, b: i32) -> i32 {
a + b
}
// JavaScript code
const wasmModule = new WebAssembly.Module(wasmCode);
const wasmInstance = new WebAssembly.Instance(wasmModule);
const add = wasmInstance.exports.add;
console.log(add(5, 3)); // Outputs: 8
This example demonstrates how you can write a simple function in Rust, compile it to WebAssembly, and then use it seamlessly in your JavaScript code.
3. Expanding Web Capabilities
WebAssembly is not just about performance; it’s about expanding what’s possible in web applications. As we move into 2024, we’re seeing an increasing number of complex applications moving to the web. WebAssembly is playing a crucial role in this shift by enabling developers to bring traditionally desktop-only applications to the browser.
Some areas where WebAssembly is making significant impacts include:
- Game Development: WebAssembly allows for high-performance game engines to run in the browser, enabling more complex and graphically intensive games on the web.
- Scientific Computing: Complex simulations and data analysis that once required dedicated software can now run efficiently in web browsers.
- Audio and Video Processing: Real-time audio and video manipulation becomes more feasible with WebAssembly’s performance capabilities.
- Virtual and Augmented Reality: WebAssembly can power more immersive and responsive VR/AR experiences on the web.
Code Example: 3D Rendering with WebAssembly
Here’s a conceptual example of how WebAssembly might be used to enhance 3D rendering performance in a web application:
// C++ code for a 3D vertex shader (compiled to WebAssembly)
extern "C" void vertexShader(float* vertex, float* mvpMatrix, float* output) {
// Perform matrix multiplication and other computations
// This would be much faster than equivalent JavaScript code
}
// JavaScript code
const vertexShaderWasm = new WebAssembly.Instance(wasmModule).exports.vertexShader;
function render3DScene() {
// For each vertex in the 3D scene
vertices.forEach(vertex => {
const outputVertex = new Float32Array(4);
vertexShaderWasm(vertex, mvpMatrix, outputVertex);
// Use the transformed vertex for rendering
});
// Render the scene
}
This example illustrates how WebAssembly can be used to offload performance-critical operations like vertex transformations in a 3D rendering pipeline, potentially leading to smoother and more complex 3D web applications.
4. Improved Security
As web applications become more complex and handle increasingly sensitive data, security becomes paramount. WebAssembly offers some inherent security benefits that make it an attractive option for developers concerned about application security:
- Sandboxed Execution: WebAssembly modules run in a sandboxed environment, isolated from the rest of the web page and the user’s system. This isolation helps prevent malicious code from accessing or manipulating data outside its intended scope.
- Type Safety: WebAssembly is designed with strong type checking, which helps prevent many common programming errors and potential vulnerabilities.
- Memory Safety: Unlike languages like C or C++, WebAssembly’s memory model prevents buffer overflows and other memory-related vulnerabilities.
- Reduced Attack Surface: By moving critical parts of an application to WebAssembly, you can reduce the amount of JavaScript code exposed to potential attackers, potentially improving overall security.
Code Example: Secure Password Hashing
Here’s a conceptual example of how WebAssembly might be used to implement secure password hashing in a web application:
// Rust code for password hashing (compiled to WebAssembly)
use argon2::{self, Config};
#[no_mangle]
pub extern "C" fn hash_password(password: *const u8, password_len: usize) -> *mut u8 {
let password = unsafe { std::slice::from_raw_parts(password, password_len) };
let salt = b"randomsalt"; // In practice, use a cryptographically secure random salt
let config = Config::default();
let hash = argon2::hash_encoded(password, salt, &config).unwrap();
let hash_ptr = hash.as_ptr() as *mut u8;
std::mem::forget(hash); // Prevent Rust from freeing the memory
hash_ptr
}
// JavaScript code
const wasmInstance = new WebAssembly.Instance(wasmModule);
const hashPassword = wasmInstance.exports.hash_password;
function secureHash(password) {
const encoder = new TextEncoder();
const passwordBuffer = encoder.encode(password);
const hashPtr = hashPassword(passwordBuffer, passwordBuffer.length);
const hash = new Uint8Array(wasmMemory.buffer, hashPtr, 100); // Assume max hash length of 100
const hashString = new TextDecoder().decode(hash);
return hashString.trim();
}
console.log(secureHash("myPassword123"));
This example demonstrates how WebAssembly can be used to implement security-critical functions like password hashing, leveraging the performance and security benefits of languages like Rust while still integrating seamlessly with JavaScript in a web application.
5. Future-Proofing Your Skills
As we look towards 2024 and beyond, it’s clear that WebAssembly is not just a passing trend but a technology that’s here to stay. Major tech companies and web standards bodies are investing heavily in WebAssembly, indicating its growing importance in the web development ecosystem.
Learning WebAssembly now puts you ahead of the curve and prepares you for the future of web development. Here are some reasons why WebAssembly skills will be valuable in the coming years:
- Growing Adoption: More companies are adopting WebAssembly for performance-critical parts of their web applications.
- Expanding Use Cases: As WebAssembly matures, its use cases are expanding beyond just web browsers to server-side applications, edge computing, and more.
- Integration with Emerging Technologies: WebAssembly is being integrated with other cutting-edge technologies like WebGPU, opening up even more possibilities for high-performance web applications.
- Career Opportunities: As demand for WebAssembly skills grows, developers proficient in this technology will likely see increased job opportunities and potentially higher salaries.
The WebAssembly System Interface (WASI)
One exciting development in the WebAssembly ecosystem is the WebAssembly System Interface (WASI). WASI aims to standardize how WebAssembly modules interact with system resources, potentially allowing WebAssembly to be used for server-side and desktop applications, not just in web browsers.
Here’s a simple example of how a WASI-compliant WebAssembly module might look:
// Rust code for a WASI module
use std::io::Write;
fn main() {
let mut stdout = std::io::stdout();
writeln!(stdout, "Hello from WASI!").unwrap();
}
This Rust code could be compiled to a WebAssembly module that, when run in a WASI-compliant runtime, would print “Hello from WASI!” to the console. This demonstrates how WebAssembly is expanding beyond just web browsers, opening up new possibilities for cross-platform development.
6. Enhanced Interoperability
One of the key strengths of WebAssembly is its ability to interoperate seamlessly with JavaScript. This interoperability allows developers to gradually adopt WebAssembly in their projects, using it where it makes the most sense while still leveraging the vast ecosystem of JavaScript libraries and frameworks.
As we move into 2024, this interoperability is likely to become even more seamless and powerful. Here are some ways WebAssembly enhances interoperability:
- Gradual Adoption: You can start by moving performance-critical parts of your application to WebAssembly while keeping the rest in JavaScript.
- Best of Both Worlds: Use WebAssembly for computationally intensive tasks and JavaScript for DOM manipulation and user interface logic.
- Ecosystem Integration: WebAssembly modules can be easily integrated into existing JavaScript frameworks and build pipelines.
- Language Diversity: WebAssembly allows you to use multiple programming languages in a single project, choosing the best language for each specific task.
Code Example: WebAssembly and JavaScript Interop
Here’s an example demonstrating how WebAssembly and JavaScript can work together in a web application:
// Rust code (compiled to WebAssembly)
#[no_mangle]
pub extern "C" fn fibonacci(n: i32) -> i32 {
if n < 2 { return n; }
fibonacci(n - 1) + fibonacci(n - 2)
}
// JavaScript code
let wasmInstance = null;
WebAssembly.instantiateStreaming(fetch('fibonacci.wasm'))
.then(obj => {
wasmInstance = obj.instance;
console.log("WebAssembly module loaded");
});
function calculateFibonacci() {
const input = document.getElementById('fibInput').value;
const result = wasmInstance.exports.fibonacci(parseInt(input));
document.getElementById('fibResult').textContent = `Fibonacci(${input}) = ${result}`;
}
// HTML
<input id="fibInput" type="number">
<button onclick="calculateFibonacci()">Calculate Fibonacci</button>
<p id="fibResult"></p>
In this example, the computationally intensive Fibonacci calculation is implemented in Rust and compiled to WebAssembly for performance, while the user interface and interaction are handled by JavaScript and HTML. This demonstrates how WebAssembly and JavaScript can complement each other in a web application.
7. Community and Ecosystem Growth
As we approach 2024, the WebAssembly community and ecosystem are experiencing rapid growth. This growth is creating a wealth of resources, tools, and libraries that make working with WebAssembly easier and more powerful. Here are some aspects of this ecosystem growth that make learning WebAssembly an attractive prospect:
- Tooling Improvements: The tools for developing, debugging, and optimizing WebAssembly are becoming more sophisticated and user-friendly.
- Growing Library Ecosystem: More libraries and frameworks are being developed specifically for or ported to WebAssembly, expanding its capabilities.
- Community Support: A growing community means more resources for learning, troubleshooting, and sharing best practices.
- Integration with Existing Tools: WebAssembly is being integrated into existing development tools and workflows, making adoption easier.
Popular Tools and Frameworks
Here are some popular tools and frameworks in the WebAssembly ecosystem that you might encounter:
- Emscripten: A complete compiler toolchain to WebAssembly, primarily used for compiling C and C++ to WebAssembly.
- Rust and wasm-pack: Rust has first-class support for WebAssembly, and wasm-pack makes it easy to build and publish Rust-generated WebAssembly packages.
- AssemblyScript: A TypeScript-like language that compiles to WebAssembly, offering an easier entry point for JavaScript developers.
- Wasmer: A universal WebAssembly runtime that allows running WebAssembly outside the browser.
- WASM-4: A fantasy console for building small games with WebAssembly.
Code Example: Using AssemblyScript
Here’s a simple example of using AssemblyScript to create a WebAssembly module:
// AssemblyScript code (math.ts)
export function add(a: i32, b: i32): i32 {
return a + b;
}
export function factorial(n: i32): i32 {
if (n === 0 || n === 1) return 1;
return n * factorial(n - 1);
}
// Compile with: asc math.ts -o math.wasm
// JavaScript code to use the WebAssembly module
const wasmModule = await WebAssembly.instantiateStreaming(fetch('math.wasm'));
const { add, factorial } = wasmModule.instance.exports;
console.log(add(5, 3)); // Outputs: 8
console.log(factorial(5)); // Outputs: 120
This example demonstrates how AssemblyScript provides a familiar syntax for JavaScript developers while still compiling to efficient WebAssembly code.
Conclusion
As we’ve explored in this article, there are compelling reasons to consider learning WebAssembly as we approach 2024. From performance improvements and expanded web capabilities to enhanced security and future-proofing your skills, WebAssembly offers a wealth of benefits for developers looking to stay at the forefront of web technology.
The growing ecosystem, improving tooling, and increasing adoption by major tech companies all point to WebAssembly playing a significant role in the future of web development. By starting to learn WebAssembly now, you’ll be well-positioned to take advantage of these trends and enhance your capabilities as a developer.
Remember, learning WebAssembly doesn’t mean abandoning JavaScript or other web technologies. Instead, it’s about expanding your toolkit and being able to choose the right tool for each job. As web applications continue to become more complex and performance-demanding, having WebAssembly in your skillset will be increasingly valuable.
So, whether you’re a seasoned developer looking to expand your skills or a coding enthusiast eager to explore new frontiers in web technology, 2024 is shaping up to be an excellent time to dive into the world of WebAssembly. The future of web development is here, and it’s running at near-native speed!