Learning TypeScript: Why It’s Important for Frontend Developers
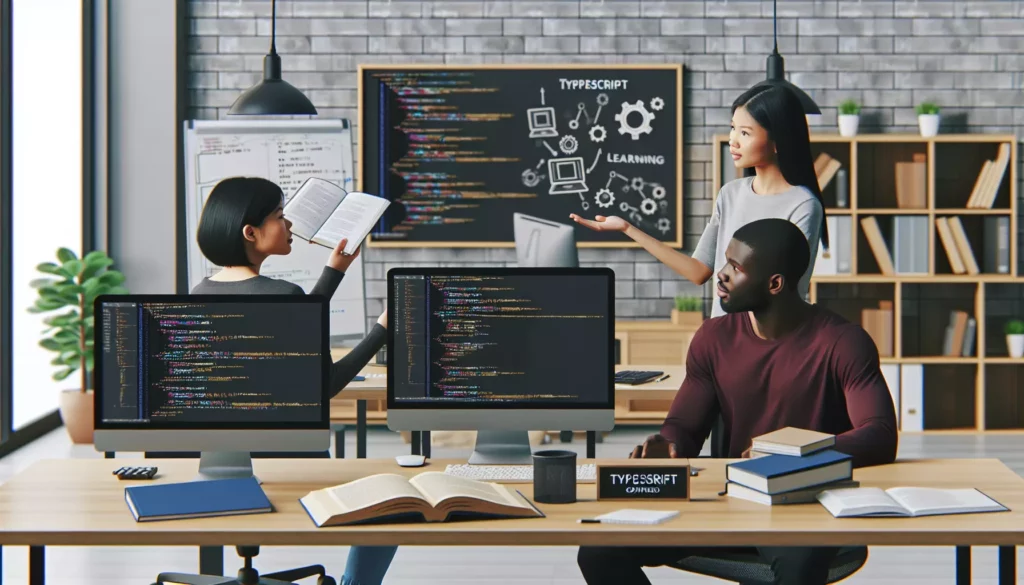
In the ever-evolving landscape of web development, staying ahead of the curve is crucial for frontend developers. As the complexity of web applications continues to grow, so does the need for more robust and scalable codebases. This is where TypeScript comes into play, offering a powerful solution to many of the challenges faced by modern frontend developers. In this comprehensive guide, we’ll explore why learning TypeScript is not just beneficial, but essential for frontend developers looking to elevate their skills and career prospects.
What is TypeScript?
Before diving into the importance of TypeScript, let’s first understand what it is. TypeScript is a statically typed superset of JavaScript developed and maintained by Microsoft. It compiles to plain JavaScript, which means it can run in any environment that supports JavaScript, including browsers, Node.js, and more.
TypeScript adds optional static typing, classes, and modules to JavaScript, enabling developers to write more maintainable and scalable code. It’s designed to develop large applications and transcompiles to JavaScript.
Why TypeScript Matters for Frontend Developers
1. Enhanced Code Quality and Maintainability
One of the primary benefits of TypeScript is its ability to improve code quality and maintainability. By introducing static typing, TypeScript helps catch errors early in the development process, often before you even run your code. This leads to:
- Fewer runtime errors: TypeScript’s compiler catches many errors that would otherwise only be discovered at runtime in JavaScript.
- Easier refactoring: With type information, IDEs can provide better refactoring tools, making it easier to modify and maintain large codebases.
- Improved code readability: Type annotations serve as documentation, making it easier for developers to understand and work with each other’s code.
2. Better Developer Experience
TypeScript significantly enhances the developer experience, particularly when working on large-scale projects. Here’s how:
- Intelligent code completion: IDEs can provide more accurate and context-aware code suggestions, speeding up development.
- Improved debugging: With type information, it’s easier to trace and fix bugs in your code.
- Enhanced tooling support: Many popular development tools and frameworks have excellent TypeScript support, leading to a more seamless development process.
3. Scalability for Large Projects
As frontend applications grow in complexity, maintaining code quality becomes increasingly challenging. TypeScript shines in large-scale projects by:
- Facilitating better architecture: TypeScript’s object-oriented features and modules help in creating more structured and scalable applications.
- Improving team collaboration: With clear interfaces and type definitions, it’s easier for team members to understand and work with shared code.
- Enabling gradual adoption: TypeScript can be incrementally adopted in existing JavaScript projects, allowing teams to migrate at their own pace.
4. Alignment with Modern JavaScript
TypeScript closely follows the development of JavaScript, often implementing new ECMAScript features before they’re widely available. This means:
- Future-proofing your skills: Learning TypeScript keeps you up-to-date with the latest JavaScript features.
- Backward compatibility: TypeScript code can be compiled to run on older JavaScript engines, allowing you to use modern features while maintaining broad browser support.
5. Growing Popularity and Industry Demand
The adoption of TypeScript in the industry has been steadily increasing. Many popular frontend frameworks and libraries, such as Angular, Vue 3, and an increasing number of React projects, are now written in or support TypeScript. This trend translates to:
- Increased job opportunities: More companies are looking for developers with TypeScript experience.
- Higher salary potential: TypeScript skills often command higher salaries due to the increased demand and perceived value.
- Better long-term career prospects: As TypeScript continues to grow in popularity, having these skills will likely become increasingly valuable.
Getting Started with TypeScript
Now that we’ve established the importance of TypeScript, let’s look at how you can start learning and incorporating it into your development workflow.
1. Setting Up Your Development Environment
To get started with TypeScript, you’ll need to set up your development environment. Here’s a basic setup process:
- Install Node.js and npm (Node Package Manager) if you haven’t already.
- Install TypeScript globally using npm:
npm install -g typescript
- Create a new TypeScript file with a .ts extension.
- Compile your TypeScript code to JavaScript using the TypeScript compiler (tsc):
tsc your-file.ts
2. Learning TypeScript Basics
Start by familiarizing yourself with TypeScript’s core concepts:
- Basic Types: Learn about TypeScript’s basic types like number, string, boolean, array, tuple, enum, any, and void.
- Interfaces: Understand how to define and use interfaces to describe object shapes.
- Classes: Explore object-oriented programming concepts in TypeScript.
- Functions: Learn how to add type annotations to function parameters and return values.
- Generics: Understand how to write reusable, type-safe code using generics.
Here’s a simple example demonstrating some of these concepts:
// Interface definition
interface User {
id: number;
name: string;
email: string;
}
// Function with type annotations
function getUserInfo(user: User): string {
return `${user.name} (${user.email})`;
}
// Using the function
const user: User = {
id: 1,
name: "John Doe",
email: "john@example.com"
};
console.log(getUserInfo(user)); // Output: John Doe (john@example.com)
3. Integrating TypeScript with Frontend Frameworks
Many frontend frameworks have excellent TypeScript support. Here’s how you can integrate TypeScript with some popular frameworks:
React with TypeScript
To create a new React project with TypeScript, you can use Create React App with the TypeScript template:
npx create-react-app my-app --template typescript
Here’s a simple React component written in TypeScript:
import React from 'react';
interface GreetingProps {
name: string;
}
const Greeting: React.FC<GreetingProps> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
Vue with TypeScript
Vue 3 has built-in TypeScript support. You can create a new Vue project with TypeScript using the Vue CLI:
vue create my-vue-app
# Select "Manually select features" and choose TypeScript
Here’s a simple Vue component using TypeScript:
import { defineComponent } from 'vue';
export default defineComponent({
name: 'HelloWorld',
props: {
msg: String,
},
setup(props) {
return () => <h1>{props.msg}</h1>;
},
});
Angular with TypeScript
Angular is built with TypeScript, so it has first-class TypeScript support out of the box. To create a new Angular project:
ng new my-angular-app
Here’s a simple Angular component using TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-greeting',
template: '<h1>Hello, {{name}}!</h1>'
})
export class GreetingComponent {
name: string = 'World';
}
Best Practices for Using TypeScript in Frontend Development
As you start incorporating TypeScript into your frontend development workflow, keep these best practices in mind:
1. Use Strict Mode
Enable strict mode in your TypeScript configuration to catch more potential errors and enforce better typing:
{
"compilerOptions": {
"strict": true
// other options...
}
}
2. Leverage Type Inference
TypeScript has powerful type inference capabilities. Use it to your advantage to write cleaner code:
// Instead of this:
let name: string = "John";
// Do this:
let name = "John"; // TypeScript infers the type as string
3. Use Union Types for Better Flexibility
Union types allow a value to be one of several types, providing more flexibility:
function printId(id: number | string) {
console.log("Your ID is: " + id);
}
printId(101); // OK
printId("202"); // OK
printId({ myID: 22342 }); // Error: Argument of type '{ myID: number; }' is not assignable to parameter of type 'string | number'.
4. Utilize Interfaces for Object Shapes
Use interfaces to define the shape of objects, making your code more readable and maintainable:
interface User {
id: number;
name: string;
email?: string; // Optional property
}
function createUser(user: User): User {
// Implementation...
}
5. Leverage Generics for Reusable Components
Generics allow you to create reusable components that can work with a variety of types:
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("myString"); // type of output will be 'string'
6. Use Enum for a Set of Named Constants
Enums are useful when you have a set of related constants:
enum Direction {
Up,
Down,
Left,
Right,
}
let move: Direction = Direction.Up;
Common Challenges and How to Overcome Them
While learning TypeScript, you might encounter some challenges. Here are some common ones and how to address them:
1. Learning Curve
Challenge: TypeScript introduces new concepts that can be overwhelming at first.
Solution: Start with the basics and gradually introduce more advanced concepts. Use online resources, tutorials, and the official TypeScript documentation. Practice regularly with small projects to reinforce your learning.
2. Type Definition Files
Challenge: When using third-party libraries, you might encounter issues with missing type definitions.
Solution: Many popular libraries have type definition files available through the DefinitelyTyped project. You can install these using npm:
npm install --save-dev @types/library-name
If type definitions aren’t available, you can create your own declaration files (.d.ts) for the libraries you’re using.
3. Overuse of ‘any’ Type
Challenge: It’s tempting to use the ‘any’ type to bypass TypeScript’s type checking, but this defeats the purpose of using TypeScript.
Solution: Try to avoid using ‘any’ as much as possible. Instead, use more specific types or create custom types/interfaces when needed. If you must use ‘any’, consider using the more restrictive ‘unknown’ type instead.
4. Configuring TypeScript
Challenge: Setting up and configuring TypeScript correctly can be tricky, especially for beginners.
Solution: Start with a basic tsconfig.json file and gradually add more options as you become more comfortable with TypeScript. Use the official TypeScript documentation to understand different compiler options.
5. Integrating with Existing JavaScript Projects
Challenge: Introducing TypeScript into an existing JavaScript project can be challenging.
Solution: TypeScript allows for gradual adoption. Start by renaming .js files to .ts and addressing errors as they come up. Use the ‘allowJs’ compiler option to mix JavaScript and TypeScript files in your project.
The Future of TypeScript in Frontend Development
As we look to the future, it’s clear that TypeScript will continue to play a significant role in frontend development. Here are some trends and predictions:
1. Increased Adoption
The adoption of TypeScript is likely to continue growing, with more frameworks and libraries embracing it as a first-class citizen. This trend will further cement TypeScript’s position as a crucial skill for frontend developers.
2. Enhanced Integration with Web Standards
As web standards evolve, TypeScript is expected to keep pace, providing type definitions and support for new APIs and features as they become available.
3. Improved Performance
Future versions of TypeScript may focus on improving compilation speed and reducing the overhead of type checking, making it even more attractive for large-scale projects.
4. Better Tooling and IDE Support
As TypeScript matures, we can expect even better tooling and IDE support, further enhancing the developer experience and productivity.
5. Expansion into New Domains
While TypeScript is primarily associated with frontend development, we may see increased adoption in other areas such as backend development, mobile app development with frameworks like React Native, and even systems programming with projects like Deno.
Conclusion
Learning TypeScript is no longer just an option for frontend developers—it’s becoming a necessity. The benefits of improved code quality, enhanced developer experience, and increased career opportunities make TypeScript an invaluable skill in today’s web development landscape.
By embracing TypeScript, you’re not only improving your current development practices but also future-proofing your skills for the evolving world of frontend development. Whether you’re working on small personal projects or large-scale applications, TypeScript provides the tools and features to write more robust, maintainable, and scalable code.
As with any new technology, the key to mastering TypeScript is practice and persistence. Start small, gradually incorporate TypeScript into your projects, and don’t be afraid to dive deep into its more advanced features as you become more comfortable.
Remember, the journey of learning TypeScript is ongoing. The language continues to evolve, and staying up-to-date with the latest features and best practices will ensure that you remain at the forefront of frontend development. Embrace the learning process, engage with the TypeScript community, and watch as your skills and the quality of your code reach new heights.
Happy coding, and welcome to the world of TypeScript!