How to Get Started with Firebase for Real-Time App Development
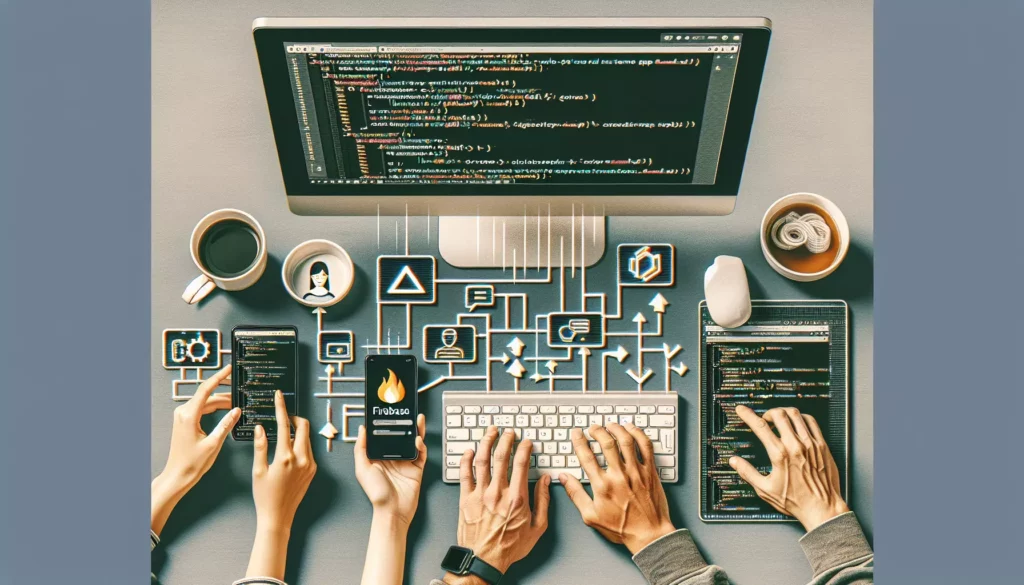
In today’s fast-paced digital world, real-time applications have become increasingly popular and essential. Whether you’re building a chat application, a collaborative tool, or a live-updating dashboard, the ability to provide instant updates and seamless synchronization across devices is crucial. This is where Firebase comes in – a powerful platform developed by Google that simplifies the process of building real-time applications. In this comprehensive guide, we’ll explore how to get started with Firebase for real-time app development, covering everything from setup to implementation of key features.
Table of Contents
- What is Firebase?
- Benefits of Using Firebase for Real-Time Development
- Setting Up Firebase for Your Project
- Firebase Realtime Database: Getting Started
- Cloud Firestore: A More Scalable Solution
- Implementing Authentication with Firebase
- Extending Functionality with Cloud Functions
- Firebase Hosting: Deploying Your App
- Best Practices and Tips for Firebase Development
- Conclusion
What is Firebase?
Firebase is a comprehensive mobile and web application development platform provided by Google. It offers a wide range of tools and services that help developers build high-quality apps, grow their user base, and earn more profit. At its core, Firebase provides a real-time database and backend as a service (BaaS), allowing developers to focus on creating great user experiences without worrying about the complexities of server-side infrastructure.
Some of the key features of Firebase include:
- Realtime Database: A cloud-hosted NoSQL database that lets you store and sync data in real-time.
- Cloud Firestore: A more scalable and flexible database solution for mobile, web, and server development.
- Authentication: Easy-to-use SDKs and ready-made UI libraries for authenticating users.
- Cloud Functions: Run backend code in response to events triggered by Firebase features and HTTPS requests.
- Hosting: Fast and secure web hosting for your static and dynamic content.
- Cloud Storage: Store and serve user-generated content like images, audio, and video.
- Analytics: Free, unlimited reporting on app usage and user engagement.
Benefits of Using Firebase for Real-Time Development
Firebase offers numerous advantages for developers looking to build real-time applications:
- Real-time synchronization: Firebase’s real-time database ensures that data is synced across all connected clients instantly, without the need for manual refreshing.
- Scalability: Firebase can handle millions of concurrent connections, making it suitable for applications of any size.
- Offline support: Firebase provides offline capabilities, allowing your app to work seamlessly even when the user is not connected to the internet.
- Cross-platform compatibility: Firebase SDKs are available for various platforms, including iOS, Android, and web, enabling you to build consistent experiences across different devices.
- Built-in security: Firebase offers robust security rules and authentication mechanisms to protect your data and users.
- Reduced backend complexity: By providing a fully managed backend infrastructure, Firebase allows developers to focus on building great user experiences rather than worrying about server management.
- Integration with Google services: Firebase seamlessly integrates with other Google Cloud services, expanding the possibilities for your application.
Setting Up Firebase for Your Project
To get started with Firebase, follow these steps:
- Create a Firebase project:
- Go to the Firebase Console.
- Click on “Add project” and follow the prompts to set up your new Firebase project.
- Add Firebase to your app:
- In the Firebase Console, click on the project you just created.
- Click on “Add app” and choose your platform (iOS, Android, or Web).
- Follow the setup instructions provided, which typically involve adding the Firebase SDK to your project and initializing Firebase with your project’s configuration.
- Install the Firebase CLI:
- Install Node.js if you haven’t already.
- Open your terminal and run the following command to install the Firebase CLI globally:
npm install -g firebase-tools
- Initialize Firebase in your project directory:
- Navigate to your project directory in the terminal.
- Run the following command and follow the prompts:
firebase init
With these steps completed, you’re ready to start using Firebase in your project!
Firebase Realtime Database: Getting Started
The Firebase Realtime Database is a cloud-hosted NoSQL database that allows you to store and sync data in real-time. Here’s how to get started:
- Enable the Realtime Database:
- In the Firebase Console, go to “Realtime Database” in the left-hand menu.
- Click “Create Database” and choose your security rules (start in test mode for development).
- Read and write data:
Here’s a basic example of how to read and write data using the Firebase SDK in JavaScript:
// Initialize Firebase (make sure you've added your config) import { initializeApp } from 'firebase/app'; import { getDatabase, ref, set, onValue } from 'firebase/database'; const firebaseConfig = { // Your config object here }; const app = initializeApp(firebaseConfig); const database = getDatabase(app); // Write data function writeUserData(userId, name, email) { set(ref(database, 'users/' + userId), { username: name, email: email }); } // Read data const userRef = ref(database, 'users/' + userId); onValue(userRef, (snapshot) => { const data = snapshot.val(); console.log(data); });
The Realtime Database uses a tree-like structure to store data, making it easy to organize and retrieve information efficiently.
Cloud Firestore: A More Scalable Solution
Cloud Firestore is Firebase’s newer, more scalable NoSQL database. It offers more powerful querying and better performance for larger applications. Here’s how to use it:
- Enable Cloud Firestore:
- In the Firebase Console, go to “Firestore Database” in the left-hand menu.
- Click “Create Database” and choose your security rules.
- Read and write data:
Here’s a basic example of how to read and write data using Cloud Firestore in JavaScript:
import { initializeApp } from 'firebase/app'; import { getFirestore, collection, addDoc, doc, getDoc } from 'firebase/firestore'; const firebaseConfig = { // Your config object here }; const app = initializeApp(firebaseConfig); const db = getFirestore(app); // Write data async function addUser(name, email) { try { const docRef = await addDoc(collection(db, "users"), { name: name, email: email }); console.log("Document written with ID: ", docRef.id); } catch (e) { console.error("Error adding document: ", e); } } // Read data async function getUser(id) { const docRef = doc(db, "users", id); const docSnap = await getDoc(docRef); if (docSnap.exists()) { console.log("Document data:", docSnap.data()); } else { console.log("No such document!"); } }
Cloud Firestore uses a document-collection model, which provides more structure and better querying capabilities compared to the Realtime Database.
Implementing Authentication with Firebase
Firebase Authentication provides easy-to-use SDKs and ready-made UI libraries to authenticate users in your app. Here’s how to implement it:
- Enable Authentication:
- In the Firebase Console, go to “Authentication” in the left-hand menu.
- Click “Get started” and enable the sign-in methods you want to use (e.g., email/password, Google, Facebook).
- Implement authentication in your app:
Here’s an example of how to implement email/password authentication:
import { initializeApp } from 'firebase/app'; import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword, onAuthStateChanged } from 'firebase/auth'; const firebaseConfig = { // Your config object here }; const app = initializeApp(firebaseConfig); const auth = getAuth(app); // Sign up function signUp(email, password) { createUserWithEmailAndPassword(auth, email, password) .then((userCredential) => { // Signed in const user = userCredential.user; console.log("User signed up:", user); }) .catch((error) => { const errorCode = error.code; const errorMessage = error.message; console.error("Sign up error:", errorCode, errorMessage); }); } // Sign in function signIn(email, password) { signInWithEmailAndPassword(auth, email, password) .then((userCredential) => { // Signed in const user = userCredential.user; console.log("User signed in:", user); }) .catch((error) => { const errorCode = error.code; const errorMessage = error.message; console.error("Sign in error:", errorCode, errorMessage); }); } // Listen for auth state changes onAuthStateChanged(auth, (user) => { if (user) { // User is signed in console.log("User is signed in:", user); } else { // User is signed out console.log("User is signed out"); } });
Firebase Authentication supports various authentication methods, including email/password, phone number, Google, Facebook, Twitter, and more. You can easily integrate these methods into your app to provide a seamless and secure authentication experience for your users.
Extending Functionality with Cloud Functions
Firebase Cloud Functions allow you to automatically run backend code in response to events triggered by Firebase features and HTTPS requests. This enables you to extend your application’s functionality without managing servers. Here’s how to get started:
- Set up Cloud Functions:
- Make sure you have the Firebase CLI installed and initialized in your project.
- Run the following command to set up Cloud Functions:
firebase init functions
- Write a Cloud Function:
Here’s an example of a simple HTTP function:
const functions = require('firebase-functions'); exports.helloWorld = functions.https.onRequest((request, response) => { response.send("Hello from Firebase!"); });
- Deploy your function:
Deploy your function using the following command:
firebase deploy --only functions
Cloud Functions can react to various events, such as database writes, authentication events, and HTTP requests. They’re perfect for tasks like data validation, notifications, and integrating with third-party services.
Firebase Hosting: Deploying Your App
Firebase Hosting provides fast and secure hosting for your web app, static and dynamic content, and microservices. Here’s how to deploy your app:
- Set up Firebase Hosting:
- If you haven’t already, initialize Firebase in your project:
firebase init
- Select “Hosting” when prompted for which Firebase features to set up.
- Configure your app:
- Firebase will create a
firebase.json
file in your project directory. - Ensure that the “public” directory in this file points to your app’s build output directory.
- Firebase will create a
- Build your app:
- Run your app’s build command (e.g.,
npm run build
for many React apps).
- Run your app’s build command (e.g.,
- Deploy your app:
- Run the following command to deploy your app:
firebase deploy
After deployment, Firebase will provide you with a URL where your app is hosted. You can also set up custom domains in the Firebase Console.
Best Practices and Tips for Firebase Development
As you develop your real-time app with Firebase, keep these best practices in mind:
- Security Rules: Always implement proper security rules for your database and storage to protect your data and ensure that users can only access what they’re supposed to.
- Offline Persistence: Enable offline persistence in your app to improve performance and allow it to work without an internet connection.
- Data Structure: Plan your data structure carefully. For Realtime Database, keep it flat. For Firestore, use subcollections for complex data structures.
- Indexing: Create appropriate indexes for your Firestore queries to ensure good performance.
- Error Handling: Implement robust error handling in your app, especially for network-related operations.
- Testing: Use Firebase Local Emulator Suite for testing your app without affecting production data.
- Monitoring: Utilize Firebase Performance Monitoring and Crashlytics to keep track of your app’s performance and stability.
- Cost Management: Keep an eye on your usage to avoid unexpected costs. Set up budget alerts in the Google Cloud Console.
Conclusion
Firebase provides a powerful and flexible platform for building real-time applications. By leveraging its various services like Realtime Database, Cloud Firestore, Authentication, Cloud Functions, and Hosting, you can create sophisticated, scalable apps with minimal backend code.
As you continue your journey with Firebase, remember to explore its extensive documentation and community resources. The Firebase team regularly updates the platform with new features and improvements, so staying informed about the latest developments can help you make the most of this powerful tool.
Whether you’re building a chat app, a collaborative tool, or any other real-time application, Firebase offers the tools and infrastructure you need to bring your ideas to life quickly and efficiently. Happy coding!