Getting Started with Laravel for PHP Developers
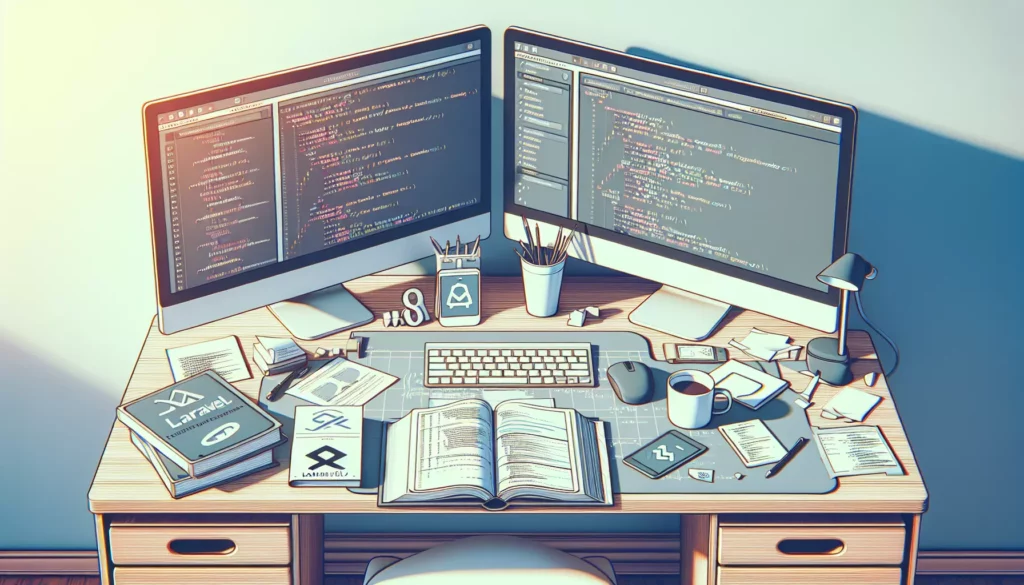
Laravel has become one of the most popular PHP frameworks in recent years, and for good reason. Its elegant syntax, powerful features, and extensive ecosystem make it an excellent choice for both beginners and experienced developers. In this comprehensive guide, we’ll walk you through the process of getting started with Laravel, covering everything from installation to building your first application.
Table of Contents
- What is Laravel?
- Prerequisites
- Installing Laravel
- Laravel Project Structure
- Routing in Laravel
- Controllers
- Views and Blade Templating
- Models and Eloquent ORM
- Database Migrations
- Building Your First Laravel Application
- Advanced Laravel Concepts
- Conclusion
What is Laravel?
Laravel is a free, open-source PHP web application framework created by Taylor Otwell. It follows the model-view-controller (MVC) architectural pattern and aims to make the development process more enjoyable for developers by simplifying common tasks used in many web projects.
Some key features of Laravel include:
- Expressive, elegant syntax
- Powerful dependency injection container
- Robust database abstraction layer
- Queue library for asynchronous processing
- Real-time event broadcasting
- Task scheduling for automated jobs
Prerequisites
Before we dive into Laravel, make sure you have the following prerequisites installed on your system:
- PHP 7.3 or higher
- Composer (dependency manager for PHP)
- Node.js and NPM (for frontend assets)
- A web server like Apache or Nginx
- A database system (MySQL, PostgreSQL, SQLite, or SQL Server)
Installing Laravel
There are two main ways to install Laravel: via Composer or using the Laravel installer. We’ll cover both methods.
Method 1: Using Composer
To install Laravel using Composer, open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel my-laravel-project
This command will create a new Laravel project in a directory called “my-laravel-project”.
Method 2: Using Laravel Installer
First, install the Laravel installer globally using Composer:
composer global require laravel/installer
Make sure to add the Composer bin directory to your system’s PATH. Then, you can create a new Laravel project by running:
laravel new my-laravel-project
After installation, navigate to your project directory:
cd my-laravel-project
Laravel Project Structure
Understanding the Laravel project structure is crucial for effective development. Here’s an overview of the main directories and files:
- app/: Contains the core code of your application
- bootstrap/: Contains files that bootstrap the framework
- config/: All of your application’s configuration files
- database/: Database migrations, factories, and seeds
- public/: The document root for your web server
- resources/: Views, raw assets (SASS, JS, etc.), and language files
- routes/: All route definitions for your application
- storage/: Compiled Blade templates, file-based sessions, file caches, and other files generated by the framework
- tests/: Automated tests
- vendor/: Composer dependencies
Routing in Laravel
Routing is a fundamental concept in Laravel. It allows you to map URLs to specific actions or controllers in your application. Routes are defined in the routes/web.php
file for web routes and routes/api.php
for API routes.
Here’s a simple example of a route:
Route::get('/hello', function () {
return 'Hello, World!';
});
This route will respond with “Hello, World!” when you visit /hello
in your browser.
You can also route to controller methods:
Route::get('/users', [UserController::class, 'index']);
This route will call the index
method of the UserController
when you visit /users
.
Controllers
Controllers are responsible for handling requests and returning responses. They help organize your application logic into discrete classes. To create a controller, you can use the Artisan command-line tool:
php artisan make:controller UserController
This will create a new controller file in app/Http/Controllers/UserController.php
. Here’s an example of a simple controller method:
public function index()
{
$users = User::all();
return view('users.index', ['users' => $users]);
}
This method retrieves all users from the database and passes them to a view called users.index
.
Views and Blade Templating
Views in Laravel are responsible for presenting your application’s user interface. Laravel uses the Blade templating engine, which provides a powerful set of tools for working with your application’s presentation layer.
Blade views are stored in the resources/views
directory. Here’s an example of a simple Blade template:
<!-- resources/views/users/index.blade.php -->
<h1>Users</h1>
<ul>
@foreach ($users as $user)
<li>{{ $user->name }}</li>
@endforeach
</ul>
Blade provides various directives like @foreach
, @if
, and @include
that make it easy to work with data and create reusable layouts.
Models and Eloquent ORM
Laravel includes Eloquent, an object-relational mapper (ORM) that makes it easy to interact with your database. Each database table has a corresponding “Model” that is used to interact with that table.
To create a model, use the Artisan command:
php artisan make:model User
This will create a new model file in app/Models/User.php
. Here’s an example of a simple model:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email'];
}
You can then use Eloquent to query the database:
$users = User::all();
$user = User::find(1);
$activeUsers = User::where('active', true)->get();
Database Migrations
Migrations are like version control for your database. They allow you to define and share your application’s database schema. To create a migration, use the Artisan command:
php artisan make:migration create_users_table
This will create a new migration file in the database/migrations
directory. Here’s an example of a migration:
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('users');
}
To run your migrations, use:
php artisan migrate
Building Your First Laravel Application
Now that we’ve covered the basics, let’s build a simple task list application to put everything together.
Step 1: Create the Task Model and Migration
php artisan make:model Task -m
This command creates both a Task model and a migration for the tasks table. Edit the migration file to define the table structure:
public function up()
{
Schema::create('tasks', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description')->nullable();
$table->boolean('completed')->default(false);
$table->timestamps();
});
}
Run the migration:
php artisan migrate
Step 2: Create the TaskController
php artisan make:controller TaskController --resource
This creates a controller with resource methods (index, create, store, etc.).
Step 3: Define Routes
Add the following to your routes/web.php
file:
use App\Http\Controllers\TaskController;
Route::resource('tasks', TaskController::class);
Step 4: Implement Controller Methods
Edit the TaskController to implement the necessary methods. Here’s an example of the index and store methods:
use App\Models\Task;
public function index()
{
$tasks = Task::all();
return view('tasks.index', compact('tasks'));
}
public function store(Request $request)
{
$validatedData = $request->validate([
'title' => 'required|max:255',
'description' => 'nullable',
]);
Task::create($validatedData);
return redirect()->route('tasks.index')->with('success', 'Task created successfully.');
}
Step 5: Create Views
Create a new file resources/views/tasks/index.blade.php
:
<!-- resources/views/tasks/index.blade.php -->
<h1>Task List</h1>
<form action="{{ route('tasks.store') }}" method="POST">
@csrf
<input type="text" name="title" placeholder="Task title" required>
<textarea name="description" placeholder="Task description"></textarea>
<button type="submit">Add Task</button>
</form>
<ul>
@foreach ($tasks as $task)
<li>{{ $task->title }} - {{ $task->completed ? 'Completed' : 'Pending' }}</li>
@endforeach
</ul>
This simple application allows you to create and view tasks. You can expand on this by adding edit, delete, and mark as completed functionality.
Advanced Laravel Concepts
As you become more comfortable with Laravel, you’ll want to explore some of its more advanced features:
1. Authentication and Authorization
Laravel provides a built-in authentication system and various ways to authorize user actions:
- Use
php artisan make:auth
to scaffold basic login and registration views - Implement role-based access control using Gates and Policies
- Utilize middleware for protecting routes
2. API Development
Laravel makes it easy to build APIs:
- Use API resources for transforming models and collections
- Implement API authentication using Laravel Passport or Sanctum
- Version your APIs for better maintainability
3. Job Queues
For handling time-consuming tasks:
- Use Laravel’s queue system to offload long-running tasks
- Implement job batching for processing multiple jobs together
- Schedule recurring tasks using the task scheduler
4. Testing
Laravel provides a rich testing ecosystem:
- Write unit tests using PHPUnit
- Perform feature tests to test entire HTTP requests
- Use database factories and seeders for testing with realistic data
5. Caching
Improve your application’s performance:
- Use Laravel’s caching system to store frequently accessed data
- Implement model caching for faster database queries
- Utilize Redis for advanced caching scenarios
6. Events and Listeners
Implement a robust event-driven architecture:
- Use Laravel’s event system to decouple various aspects of your application
- Implement event listeners to respond to specific events
- Utilize event broadcasting for real-time applications
Conclusion
Laravel offers a rich ecosystem for PHP developers, providing a wide range of tools and features to build robust web applications. This guide has covered the basics of getting started with Laravel, from installation to building a simple application. As you continue your Laravel journey, be sure to explore the official Laravel documentation for in-depth information on all aspects of the framework.
Remember that becoming proficient in Laravel takes time and practice. Don’t be afraid to experiment with different features and build small projects to reinforce your learning. As you gain experience, you’ll discover the true power and flexibility that Laravel offers, allowing you to create sophisticated, scalable web applications with ease.
Happy coding, and welcome to the world of Laravel development!