What is GraphQL? A Beginner’s Guide to This Query Language
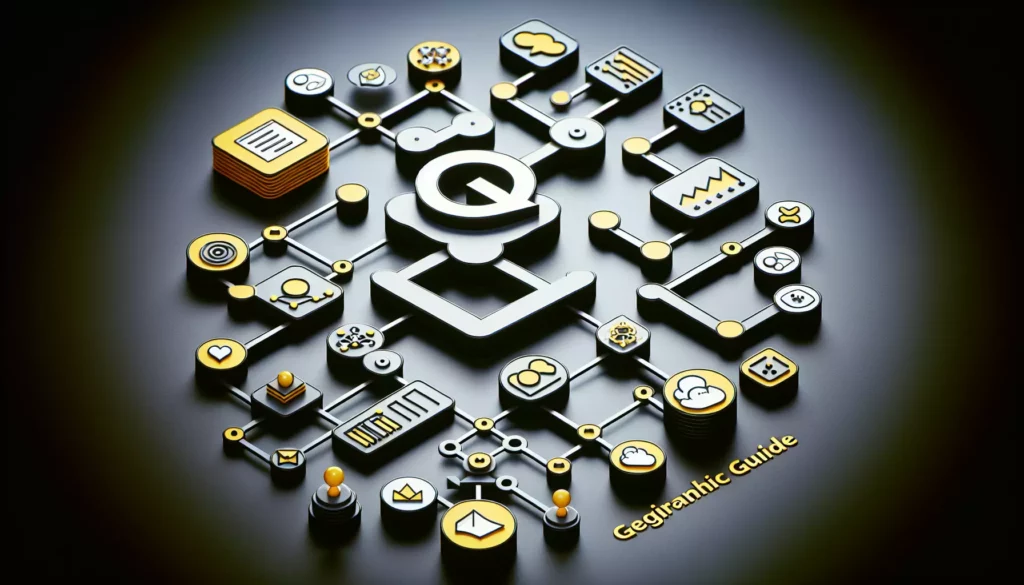
In the ever-evolving world of web development, efficient data fetching and manipulation are crucial for building performant applications. Enter GraphQL, a query language and runtime that has been gaining significant traction in recent years. If you’re a beginner looking to understand what GraphQL is all about, you’ve come to the right place. In this comprehensive guide, we’ll explore GraphQL, its benefits, and how it compares to traditional REST APIs.
Table of Contents
- What is GraphQL?
- A Brief History of GraphQL
- How GraphQL Works
- Key Concepts in GraphQL
- Benefits of Using GraphQL
- GraphQL vs. REST: A Comparison
- When to Use GraphQL
- Getting Started with GraphQL
- Best Practices and Tips
- Challenges and Considerations
- The Future of GraphQL
- Conclusion
What is GraphQL?
GraphQL is a query language and server-side runtime for Application Programming Interfaces (APIs) that prioritizes giving clients exactly the data they request and nothing more. Developed by Facebook in 2012 and publicly released in 2015, GraphQL provides a more efficient, powerful, and flexible alternative to traditional REST API architecture.
At its core, GraphQL allows clients to define the structure of the data required, and the server returns exactly that structure. This approach eliminates the problems of over-fetching and under-fetching data that are common with REST APIs.
A Brief History of GraphQL
The story of GraphQL begins at Facebook. In 2012, the company was facing challenges with its mobile applications due to differences in data requirements between their mobile apps and their website. The existing REST API structure was not flexible enough to cater to these varying needs efficiently.
To address this, Facebook’s engineering team, led by Lee Byron, Nick Schrock, and Dan Schafer, began developing GraphQL. Their goal was to create a data query and manipulation language that would be more efficient and flexible than REST.
After using GraphQL internally for several years, Facebook publicly released it in 2015. Since then, it has gained widespread adoption, with companies like GitHub, Shopify, Twitter, and many others implementing GraphQL in their systems.
How GraphQL Works
GraphQL operates on a few key principles:
- Single Endpoint: Unlike REST APIs that have multiple endpoints for different resources, GraphQL typically uses a single endpoint for all data fetching and manipulation.
- Client-Specified Queries: Clients can specify exactly what data they need in their queries.
- Hierarchical: GraphQL queries mirror the shape of the data they return, making it intuitive to work with nested data structures.
- Strongly Typed: GraphQL uses a strong type system to define the capabilities of an API.
Here’s a simple example of how a GraphQL query might look:
{
user(id: 123) {
name
email
posts {
title
comments {
content
}
}
}
}
In this query, we’re requesting data about a user with ID 123. We want their name, email, and information about their posts, including the title of each post and the content of comments on those posts. The server would respond with exactly this data structure, populated with the requested information.
Key Concepts in GraphQL
To understand GraphQL better, let’s explore some of its key concepts:
1. Schema
The schema is the heart of any GraphQL implementation. It defines the capabilities of the API and specifies how clients can request and manipulate data. The schema is written using GraphQL Schema Definition Language (SDL).
Here’s an example of a simple schema:
type User {
id: ID!
name: String!
email: String!
posts: [Post!]!
}
type Post {
id: ID!
title: String!
content: String!
author: User!
}
type Query {
user(id: ID!): User
allUsers: [User!]!
}
type Mutation {
createUser(name: String!, email: String!): User!
}
2. Types
GraphQL uses a strong type system to describe the data. The most common types are:
- Scalar types: These include String, Int, Float, Boolean, and ID.
- Object types: These are custom types you define, like User or Post in the example above.
- Query type: This special type defines the entry points for read operations.
- Mutation type: This type defines the entry points for write operations.
3. Resolvers
Resolvers are functions that are responsible for populating the data for a single field in your schema. When a query comes in, the GraphQL server calls the appropriate resolvers to fetch the requested data.
4. Queries
Queries in GraphQL are used to fetch data. They look similar to the schema but without the type definitions. Here’s an example query based on our schema:
{
user(id: "123") {
name
email
posts {
title
}
}
}
5. Mutations
Mutations are used to modify server-side data. They are defined in the schema and used by clients to perform write operations. Here’s an example mutation:
mutation {
createUser(name: "John Doe", email: "john@example.com") {
id
name
email
}
}
Benefits of Using GraphQL
GraphQL offers several advantages over traditional API architectures:
- Efficient Data Fetching: Clients can request exactly what they need, eliminating over-fetching and under-fetching of data.
- Flexible: As your API evolves, you can add new fields and types without impacting existing queries.
- Strongly Typed: The schema provides clear contracts between client and server.
- Introspection: GraphQL APIs are self-documenting, making it easier for developers to explore and understand the available data.
- Single Request: Multiple resources can be fetched in a single request, reducing network overhead.
- Code Generation: The strongly typed nature of GraphQL enables automatic code generation for type-safe client-side development.
GraphQL vs. REST: A Comparison
While both GraphQL and REST are used for building APIs, they have some key differences:
Feature | GraphQL | REST |
---|---|---|
Endpoints | Single endpoint | Multiple endpoints |
Data Fetching | Client specifies exact data needs | Server determines the data structure |
Versioning | Easier to add fields and types without versioning | Often requires versioning for significant changes |
Learning Curve | Steeper initial learning curve | Simpler to understand and implement initially |
Caching | Requires more complex caching strategy | Simpler to cache due to the nature of endpoints |
File Uploads | Requires additional tooling | Natively supports file uploads |
When to Use GraphQL
While GraphQL offers many advantages, it’s not always the best solution for every project. Consider using GraphQL when:
- You have complex data requirements with nested and interconnected resources.
- Your application needs to aggregate data from multiple sources.
- You’re building a mobile app that requires efficient data loading.
- You need a flexible API that can evolve without versioning.
- You want to provide a powerful API for third-party developers.
However, REST might be a better choice when:
- You’re building a simple CRUD application with straightforward data requirements.
- Caching is a critical concern, and you need the simplicity of URL-based cache invalidation.
- File uploads are a primary feature of your API.
- You need to stick with industry standards that are more familiar to a broader range of developers.
Getting Started with GraphQL
If you’re ready to dive into GraphQL, here’s a basic guide to get you started:
1. Choose Your Tools
There are many libraries and frameworks available for implementing GraphQL. Some popular choices include:
- Apollo Server (Node.js)
- GraphQL-Java (Java)
- Graphene (Python)
- Absinthe (Elixir)
2. Define Your Schema
Start by defining your GraphQL schema. This will be the blueprint for your API. Here’s a simple example:
type Query {
hello: String
}
schema {
query: Query
}
3. Implement Resolvers
Create resolver functions for each field in your schema. For our simple example:
const resolvers = {
Query: {
hello: () => 'Hello, World!'
}
}
4. Set Up Your Server
Use your chosen GraphQL server library to set up an HTTP server. Here’s an example using Apollo Server:
const { ApolloServer } = require('apollo-server');
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server ready at ${url}`);
});
5. Test Your API
Once your server is running, you can test your API using tools like GraphQL Playground or Altair GraphQL Client. Try running this query:
{
hello
}
You should see the response: { "data": { "hello": "Hello, World!" } }
Best Practices and Tips
As you continue your journey with GraphQL, keep these best practices in mind:
- Design Your Schema Carefully: Your schema is a contract with your clients. Take time to design it thoughtfully.
- Use Fragments: Fragments allow you to reuse parts of your queries, making your code more maintainable.
- Implement Proper Error Handling: GraphQL provides a standardized way to handle errors. Make use of it to provide meaningful error messages to your clients.
- Optimize Performance: Use techniques like dataloader to batch and cache database queries.
- Secure Your API: Implement authentication and authorization. Consider using tools like graphql-shield for declarative permission management.
- Monitor Your API: Use tools like Apollo Studio to gain insights into your API’s performance and usage patterns.
- Keep Your Schema Evolving: One of GraphQL’s strengths is its ability to evolve. Don’t be afraid to add new fields and types as your application grows.
Challenges and Considerations
While GraphQL offers many benefits, it also comes with its own set of challenges:
- Complexity: GraphQL introduces new concepts that can be challenging for teams new to the technology.
- Performance Concerns: Without proper optimization, complex queries can lead to performance issues.
- Caching: Implementing efficient caching strategies can be more complex compared to REST APIs.
- Security: The flexibility of GraphQL queries means you need to be extra careful about securing your API against malicious queries.
- File Uploads: While possible, handling file uploads in GraphQL is not as straightforward as in REST APIs.
The Future of GraphQL
GraphQL continues to evolve and grow in popularity. Some trends and developments to watch out for include:
- Adoption by Major Players: More large companies are adopting GraphQL, driving its growth and ecosystem.
- Improved Tooling: The GraphQL ecosystem is constantly improving, with better development tools, monitoring solutions, and integrations.
- Federation: GraphQL Federation allows for creating a unified graph from multiple services, enabling better scalability for large organizations.
- Subscriptions and Real-Time Data: GraphQL subscriptions are becoming more robust, enabling powerful real-time applications.
- Integration with New Technologies: Expect to see more integration with emerging technologies like serverless computing and edge computing.
Conclusion
GraphQL represents a significant shift in how we think about API design and data fetching. Its flexibility, efficiency, and strong typing make it an attractive option for many modern web and mobile applications. While it comes with its own learning curve and challenges, the benefits often outweigh the costs for complex, data-intensive applications.
As you continue your journey in software development, understanding GraphQL will be a valuable skill. Whether you’re building a new application from scratch or looking to optimize an existing system, GraphQL offers powerful tools to make your APIs more efficient and developer-friendly.
Remember, the best way to learn is by doing. Start small, experiment with GraphQL in your projects, and gradually expand your understanding. Happy coding!