Introduction to Swift for iOS Development Beginners
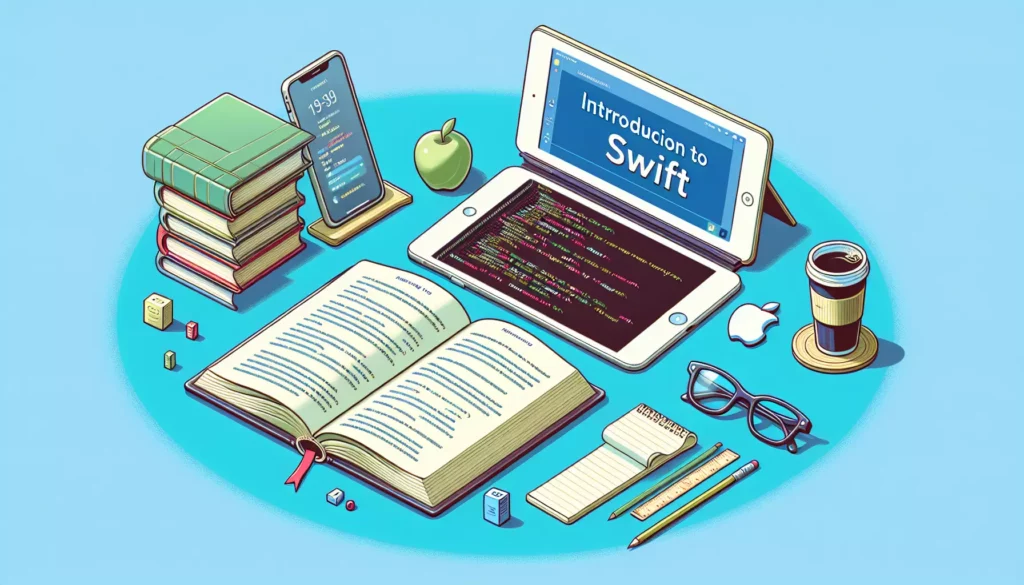
Welcome to the exciting world of iOS development! If you’re a beginner looking to create apps for Apple devices, you’ve come to the right place. In this comprehensive guide, we’ll introduce you to Swift, Apple’s powerful and intuitive programming language designed specifically for iOS, macOS, watchOS, and tvOS development. By the end of this article, you’ll have a solid foundation in Swift and be ready to start your journey as an iOS developer.
Table of Contents
- What is Swift?
- Why Choose Swift for iOS Development?
- Setting Up Your Development Environment
- Swift Basics: Syntax and Fundamentals
- Variables and Constants
- Data Types in Swift
- Control Flow: Conditionals and Loops
- Functions and Closures
- Classes and Structures
- Optionals and Optional Chaining
- Error Handling in Swift
- Collections: Arrays, Sets, and Dictionaries
- Protocols and Extensions
- Memory Management and ARC
- Next Steps in Your iOS Development Journey
1. What is Swift?
Swift is a modern, fast, and safe programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. Introduced in 2014, Swift was designed to replace Objective-C as the primary language for Apple platforms. It combines the best features of various programming languages, offering a clean syntax, powerful features, and excellent performance.
Key characteristics of Swift include:
- Safety: Swift includes features that help prevent common programming errors.
- Speed: Swift code compiles to optimized native code, offering performance comparable to C++.
- Expressiveness: Its clean syntax makes Swift easy to read and write.
- Modern language features: Swift supports functional programming patterns, generics, and protocol-oriented programming.
2. Why Choose Swift for iOS Development?
There are several compelling reasons to choose Swift for iOS development:
- Native language: Swift is designed specifically for Apple platforms, ensuring seamless integration with iOS frameworks and APIs.
- Performance: Swift offers excellent runtime performance, crucial for creating responsive and efficient apps.
- Safety features: Swift’s design helps prevent common programming errors, leading to more stable and secure applications.
- Readability: Swift’s clean and expressive syntax makes it easier to write and maintain code.
- Interoperability: Swift can work alongside Objective-C in the same project, allowing gradual migration of existing codebases.
- Open-source: Swift is open-source, fostering a growing community and ecosystem of tools and libraries.
- Playground support: Swift Playgrounds provide an interactive environment for learning and experimenting with code.
3. Setting Up Your Development Environment
To start developing iOS apps with Swift, you’ll need to set up your development environment. Here’s what you’ll need:
- Mac computer: iOS development requires a Mac running macOS.
- Xcode: Apple’s integrated development environment (IDE) for creating iOS, macOS, watchOS, and tvOS applications.
Follow these steps to set up your environment:
- Ensure your Mac is running the latest version of macOS.
- Open the App Store on your Mac.
- Search for “Xcode” and click “Get” or “Install” to download and install Xcode.
- Once installed, open Xcode to complete any additional installations or configurations.
With Xcode installed, you’re ready to start coding in Swift!
4. Swift Basics: Syntax and Fundamentals
Let’s dive into the basics of Swift syntax and fundamental concepts. Swift’s syntax is designed to be clear and concise, making it easy for beginners to learn and understand.
Comments
Comments in Swift can be single-line or multi-line:
// This is a single-line comment
/* This is a
multi-line comment */
Semicolons
Unlike many other programming languages, Swift doesn’t require semicolons at the end of each statement. However, you can use them if you prefer or if you want to write multiple statements on a single line:
let name = "John" // No semicolon needed
let age = 30; let height = 180 // Semicolons used for multiple statements on one line
Print Statements
To output text to the console, use the print()
function:
print("Hello, World!")
print("My name is \(name) and I'm \(age) years old.")
5. Variables and Constants
In Swift, you can declare variables using var
and constants using let
. Constants are immutable, meaning their values cannot be changed once set.
var mutableVariable = 42
mutableVariable = 43 // This is allowed
let immutableConstant = 100
// immutableConstant = 101 // This would cause an error
Swift uses type inference to determine the type of a variable or constant based on its initial value. You can also explicitly declare the type:
var explicitInteger: Int = 42
let explicitDouble: Double = 3.14159
6. Data Types in Swift
Swift provides several built-in data types:
- Int: Whole numbers (e.g., 42, -17)
- Double: Floating-point numbers (e.g., 3.14159)
- Float: Single-precision floating-point numbers
- Bool: Boolean values (true or false)
- String: Text (e.g., “Hello, World!”)
- Character: Single Unicode characters (e.g., “A”, “😊”)
Example usage:
let integerValue: Int = 42
let doubleValue: Double = 3.14159
let floatValue: Float = 2.71828
let boolValue: Bool = true
let stringValue: String = "Hello, Swift!"
let characterValue: Character = "A"
7. Control Flow: Conditionals and Loops
Swift provides various control flow statements to manage the flow of your code.
Conditionals
The if
statement is used for conditional execution:
let temperature = 25
if temperature > 30 {
print("It's hot outside!")
} else if temperature < 10 {
print("It's cold outside!")
} else {
print("The weather is pleasant.")
}
Swift also offers a switch statement for more complex conditionals:
let dayOfWeek = "Monday"
switch dayOfWeek {
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday":
print("It's a weekday.")
case "Saturday", "Sunday":
print("It's the weekend!")
default:
print("Invalid day of the week.")
}
Loops
Swift provides several types of loops:
For-in loop:
for i in 1...5 {
print(i)
}
While loop:
var counter = 0
while counter < 5 {
print(counter)
counter += 1
}
Repeat-while loop (do-while in other languages):
var number = 1
repeat {
print(number)
number *= 2
} while number < 100
8. Functions and Closures
Functions in Swift are defined using the func
keyword:
func greet(name: String) -> String {
return "Hello, \(name)!"
}
print(greet(name: "Alice")) // Outputs: Hello, Alice!
Swift also supports default parameter values and variadic parameters:
func greetWithEmoji(name: String, emoji: String = "👋") -> String {
return "\(emoji) Hello, \(name)!"
}
print(greetWithEmoji(name: "Bob")) // Outputs: 👋 Hello, Bob!
print(greetWithEmoji(name: "Charlie", emoji: "😊")) // Outputs: 😊 Hello, Charlie!
Closures are self-contained blocks of functionality that can be passed around and used in your code:
let numbers = [1, 2, 3, 4, 5]
let squaredNumbers = numbers.map { $0 * $0 }
print(squaredNumbers) // Outputs: [1, 4, 9, 16, 25]
9. Classes and Structures
Swift uses classes and structures to create custom types. Classes support inheritance, while structures are value types and do not support inheritance.
Class example:
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func introduce() {
print("Hi, I'm \(name) and I'm \(age) years old.")
}
}
let john = Person(name: "John", age: 30)
john.introduce() // Outputs: Hi, I'm John and I'm 30 years old.
Structure example:
struct Point {
var x: Double
var y: Double
func distanceFromOrigin() -> Double {
return sqrt(x*x + y*y)
}
}
let point = Point(x: 3, y: 4)
print(point.distanceFromOrigin()) // Outputs: 5.0
10. Optionals and Optional Chaining
Optionals are a powerful feature in Swift that allow you to represent the absence of a value. An optional can either contain a value or be nil
.
var optionalName: String? = "John"
optionalName = nil // This is allowed
var requiredName: String = "Alice"
// requiredName = nil // This would cause an error
To safely unwrap an optional, you can use optional binding:
if let name = optionalName {
print("Hello, \(name)!")
} else {
print("Name is nil")
}
Optional chaining allows you to call properties, methods, and subscripts on an optional that might be nil:
struct Book {
var title: String
var author: String?
}
let book = Book(title: "Swift Programming", author: nil)
let authorName = book.author?.uppercased()
print(authorName) // Outputs: nil
11. Error Handling in Swift
Swift provides built-in support for throwing, catching, propagating, and manipulating recoverable errors at runtime.
enum VendingMachineError: Error {
case invalidSelection
case insufficientFunds(coinsNeeded: Int)
case outOfStock
}
func buySnack(name: String, coinsInserted: Int) throws {
guard name == "Candy Bar" else {
throw VendingMachineError.invalidSelection
}
guard coinsInserted >= 100 else {
throw VendingMachineError.insufficientFunds(coinsNeeded: 100 - coinsInserted)
}
print("Dispensing \(name)")
}
do {
try buySnack(name: "Candy Bar", coinsInserted: 50)
} catch VendingMachineError.invalidSelection {
print("Invalid snack selection.")
} catch VendingMachineError.insufficientFunds(let coinsNeeded) {
print("Not enough coins. Please insert \(coinsNeeded) more coins.")
} catch {
print("Unexpected error: \(error)")
}
12. Collections: Arrays, Sets, and Dictionaries
Swift provides three primary collection types: arrays, sets, and dictionaries.
Arrays
Arrays are ordered collections of values:
var fruits = ["Apple", "Banana", "Orange"]
fruits.append("Mango")
print(fruits[0]) // Outputs: Apple
Sets
Sets are unordered collections of unique values:
var numbers: Set<Int> = [1, 2, 3, 4, 5]
numbers.insert(6)
print(numbers.contains(3)) // Outputs: true
Dictionaries
Dictionaries store key-value associations:
var capitals = ["France": "Paris", "Italy": "Rome", "Japan": "Tokyo"]
capitals["Spain"] = "Madrid"
print(capitals["Italy"] ?? "Unknown") // Outputs: Rome
13. Protocols and Extensions
Protocols define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality:
protocol Drawable {
func draw()
}
class Circle: Drawable {
func draw() {
print("Drawing a circle")
}
}
let circle = Circle()
circle.draw() // Outputs: Drawing a circle
Extensions allow you to add new functionality to an existing type:
extension String {
func repeated(times: Int) -> String {
return String(repeating: self, count: times)
}
}
let hello = "Hello"
print(hello.repeated(times: 3)) // Outputs: HelloHelloHello
14. Memory Management and ARC
Swift uses Automatic Reference Counting (ARC) to track and manage your app’s memory usage. In most cases, memory management “just works” in Swift, but it’s important to understand the concept of strong reference cycles and how to avoid them:
class Person {
let name: String
var apartment: Apartment?
init(name: String) {
self.name = name
}
deinit {
print("\(name) is being deinitialized")
}
}
class Apartment {
let unit: String
weak var tenant: Person?
init(unit: String) {
self.unit = unit
}
deinit {
print("Apartment \(unit) is being deinitialized")
}
}
var john: Person? = Person(name: "John")
var unit4A: Apartment? = Apartment(unit: "4A")
john?.apartment = unit4A
unit4A?.tenant = john
john = nil
unit4A = nil
// Outputs:
// John is being deinitialized
// Apartment 4A is being deinitialized
15. Next Steps in Your iOS Development Journey
Congratulations! You’ve now got a solid foundation in Swift programming. Here are some suggested next steps to continue your iOS development journey:
- Practice, practice, practice: The best way to improve your Swift skills is to write code regularly. Try solving coding challenges or building small projects.
- Learn UIKit or SwiftUI: These are the primary frameworks for building user interfaces in iOS apps. UIKit is the older, more established framework, while SwiftUI is Apple’s modern declarative UI framework.
- Explore iOS frameworks: Familiarize yourself with other important iOS frameworks like Foundation, Core Data, and Core Animation.
- Study iOS app architecture: Learn about common architectural patterns like MVC, MVVM, and Clean Architecture.
- Build complete apps: Start working on more complex projects that incorporate multiple aspects of iOS development.
- Join the community: Engage with other Swift developers through forums, social media, or local meetups.
- Keep learning: Stay up-to-date with the latest Swift and iOS developments by following Apple’s documentation and WWDC sessions.
Remember, becoming proficient in iOS development takes time and practice. Be patient with yourself, stay curious, and enjoy the learning process!
As you continue your journey into iOS development with Swift, you’ll discover the power and flexibility of this modern programming language. Swift’s safety features, performance, and expressiveness make it an excellent choice for building robust and efficient iOS applications. Whether you’re creating a simple utility app or a complex, data-driven application, Swift provides the tools and capabilities you need to bring your ideas to life on Apple’s platforms.
Happy coding, and welcome to the exciting world of iOS development with Swift!