Top 5 Backend Frameworks You Should Know
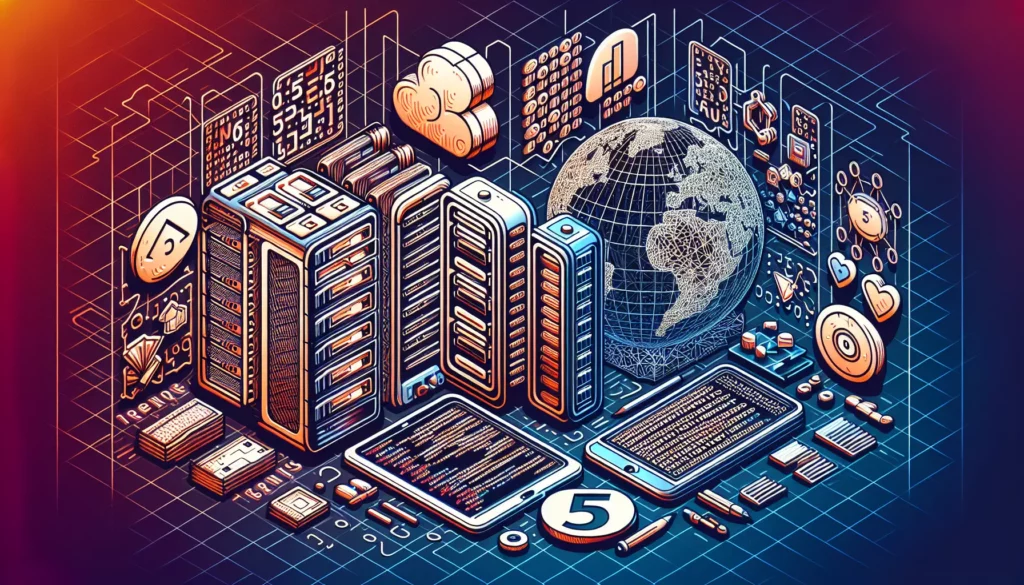
In the ever-evolving world of web development, backend frameworks play a crucial role in building robust, scalable, and efficient web applications. As aspiring developers or seasoned programmers looking to expand their skill set, it’s essential to be familiar with the most popular and powerful backend frameworks in the industry. In this comprehensive guide, we’ll explore the top 5 backend frameworks you should know, diving into their features, advantages, and use cases.
1. Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It’s known for its speed and simplicity, making it an excellent choice for both beginners and experienced developers.
Key Features:
- Minimalist and unopinionated
- Fast and lightweight
- Extensive middleware support
- Easy routing
- Template engine support
- Excellent performance and high test coverage
Advantages:
- Easy to learn and use
- Flexible and customizable
- Large community and ecosystem
- Great for building RESTful APIs
- Seamless integration with frontend JavaScript frameworks
Use Cases:
- Single-page applications
- Real-time applications (e.g., chat apps)
- RESTful API development
- Microservices architecture
Example Code:
Here’s a simple Express.js application that sets up a basic server and handles a GET request:
const express = require("express");
const app = express();
const port = 3000;
app.get("/", (req, res) => {
res.send("Hello, World!");
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
2. Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It follows the “batteries included” philosophy, providing a wide range of built-in features out of the box.
Key Features:
- ORM (Object-Relational Mapping) for database operations
- Built-in admin interface
- URL routing
- Template engine
- Form handling
- Authentication system
- Caching
Advantages:
- Rapid development
- Scalability
- Security features built-in
- Versatility (suitable for various types of projects)
- Large and active community
- Excellent documentation
Use Cases:
- Content Management Systems (CMS)
- E-commerce platforms
- Scientific computing platforms
- Large-scale web applications
Example Code:
Here’s a simple Django view function that renders a template:
from django.shortcuts import render
def hello_world(request):
context = {"message": "Hello, World!"}
return render(request, "hello.html", context)
3. Ruby on Rails
Ruby on Rails, often simply called Rails, is a server-side web application framework written in Ruby. It follows the Model-View-Controller (MVC) architectural pattern and emphasizes the use of well-known software engineering patterns and principles, such as convention over configuration (CoC) and don’t repeat yourself (DRY).
Key Features:
- MVC architecture
- Active Record ORM
- RESTful application design
- Built-in testing framework
- Asset pipeline
- Action Mailer for sending emails
- Active Job for background processing
Advantages:
- Rapid development
- Convention over configuration
- Strong focus on testing
- Large ecosystem of gems (plugins)
- Active and supportive community
- Great for prototyping and MVP development
Use Cases:
- Startups and rapid prototyping
- E-commerce platforms
- Social networking sites
- Content management systems
Example Code:
Here’s a simple Ruby on Rails controller action:
class UsersController < ApplicationController
def index
@users = User.all
end
def show
@user = User.find(params[:id])
end
end
4. Spring Boot
Spring Boot is an open-source Java-based framework used to create microservices and stand-alone applications. It’s built on top of the Spring Framework and designed to simplify the development process by providing a set of pre-configured settings and dependencies.
Key Features:
- Opinionated starter dependencies
- Embedded server (Tomcat, Jetty, or Undertow)
- Auto-configuration
- Production-ready features (metrics, health checks, externalized configuration)
- Spring Boot Actuator for application monitoring
- Easy integration with Spring ecosystem
Advantages:
- Reduces boilerplate code
- Simplifies dependency management
- Provides production-ready applications out of the box
- Supports both traditional deployment and cloud-native patterns
- Large and mature ecosystem
- Excellent for microservices architecture
Use Cases:
- Microservices
- RESTful APIs
- Web applications
- Enterprise applications
Example Code:
Here’s a simple Spring Boot application with a REST controller:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
@GetMapping("/")
public String hello() {
return "Hello, World!";
}
}
5. Laravel
Laravel is a free, open-source PHP web framework designed for the development of web applications following the model–view–controller (MVC) architectural pattern. It aims to make the development process a pleasing experience for the developer without sacrificing application functionality.
Key Features:
- Eloquent ORM (Object-Relational Mapping)
- Blade templating engine
- Routing system
- Authentication and authorization
- Migration system for database version control
- Built-in testing tools
- Task scheduling
Advantages:
- Expressive and elegant syntax
- Robust ecosystem with many packages available
- Strong security features
- Built-in tools for common tasks
- Great documentation and community support
- Follows modern PHP best practices
Use Cases:
- Web applications
- E-commerce platforms
- Content management systems
- RESTful APIs
Example Code:
Here’s a simple Laravel route and controller method:
// routes/web.php
Route::get("/", "HomeController@index");
// app/Http/Controllers/HomeController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function index()
{
return view("welcome", ["message" => "Hello, World!"]);
}
}
Choosing the Right Backend Framework
When selecting a backend framework for your project, consider the following factors:
- Language Preference: Choose a framework that aligns with your team’s expertise or the language you want to learn.
- Project Requirements: Consider the specific needs of your project, such as scalability, performance, and features required.
- Learning Curve: Some frameworks are easier to learn than others. Factor in the time you have available for learning and development.
- Community and Ecosystem: A large, active community means better support, more resources, and a wider range of third-party packages.
- Performance: Evaluate the framework’s performance characteristics, especially if you’re building a high-traffic application.
- Scalability: Consider how well the framework supports scaling your application as it grows.
- Job Market: If you’re learning for career purposes, research which frameworks are in high demand in your target job market.
Conclusion
In this comprehensive guide, we’ve explored the top 5 backend frameworks you should know: Express.js, Django, Ruby on Rails, Spring Boot, and Laravel. Each of these frameworks has its own strengths and is suited for different types of projects and development styles.
As you continue your journey in web development, it’s valuable to gain experience with multiple frameworks. This not only broadens your skill set but also gives you the flexibility to choose the best tool for each project you undertake. Remember, the best framework is often the one that aligns with your project requirements, team expertise, and development goals.
Whether you’re building a simple web application, a complex enterprise system, or anything in between, understanding these popular backend frameworks will give you a solid foundation in modern web development. As you explore these frameworks, don’t forget to practice your skills by building real projects and contributing to open-source communities. Happy coding!
Further Learning Resources
To deepen your understanding of these frameworks and improve your backend development skills, consider exploring the following resources:
- Official Documentation: Each framework has comprehensive documentation that serves as the best starting point for learning.
- Online Courses: Platforms like Udemy, Coursera, and edX offer in-depth courses on these frameworks.
- Coding Bootcamps: Intensive programs that often cover one or more of these frameworks in detail.
- GitHub Repositories: Explore open-source projects built with these frameworks to see real-world applications.
- Tech Blogs and Tutorials: Many developers and companies share their experiences and best practices through blog posts and tutorials.
- Coding Challenges: Platforms like HackerRank and LeetCode can help you practice your programming skills, which are fundamental to using any framework effectively.
Remember, becoming proficient in a backend framework takes time and practice. Start with small projects, gradually increase complexity, and don’t hesitate to seek help from the developer community when you encounter challenges. With persistence and dedication, you’ll be building robust backend systems in no time!