Essential Python Libraries Every Developer Should Know
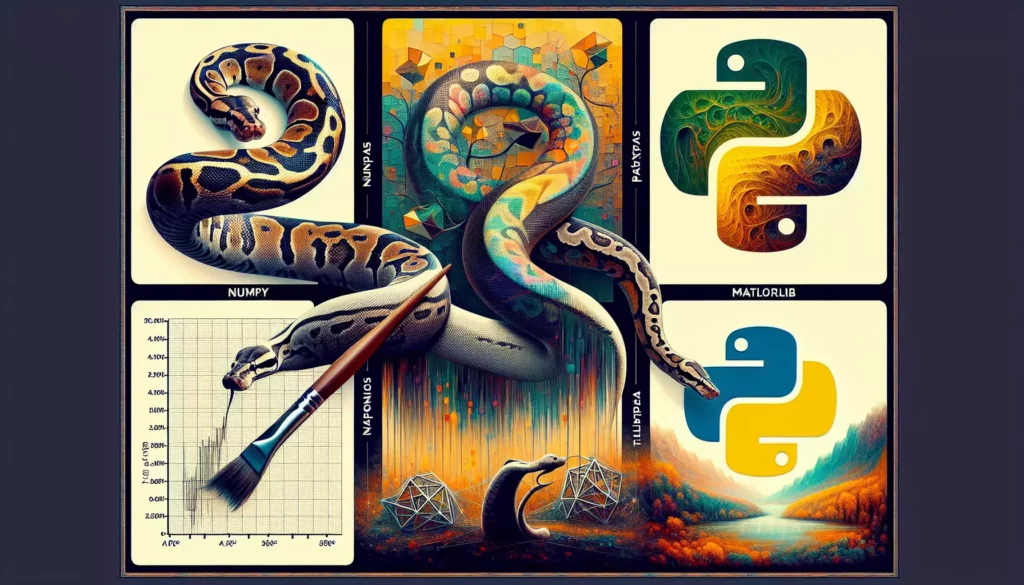
Python’s popularity and versatility stem from its extensive ecosystem of libraries and frameworks. These libraries extend Python’s capabilities, making it a go-to language for various applications, from web development to data science and machine learning. In this comprehensive guide, we’ll explore the essential Python libraries that every developer should be familiar with, regardless of their specialization.
1. NumPy
NumPy (Numerical Python) is the foundation for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently.
Key features:
- Multi-dimensional array objects
- Advanced mathematical functions
- Tools for integrating C/C++ and Fortran code
- Linear algebra, Fourier transform, and random number capabilities
Example usage:
import numpy as np
# Create a 2D array
arr = np.array([[1, 2, 3], [4, 5, 6]])
# Perform element-wise operations
print(arr * 2)
# Output:
# [[2 4 6]
# [8 10 12]]
# Calculate the mean of the array
print(np.mean(arr))
# Output: 3.5
2. Pandas
Pandas is a powerful data manipulation and analysis library. It provides data structures like DataFrames and Series, making it easy to work with structured data.
Key features:
- DataFrame and Series data structures
- Reading and writing data in various formats (CSV, Excel, SQL databases)
- Data alignment and integrated handling of missing data
- Merging and joining datasets
- Time series functionality
Example usage:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'San Francisco', 'Los Angeles']
})
# Display the DataFrame
print(df)
# Output:
# Name Age City
# 0 Alice 25 New York
# 1 Bob 30 San Francisco
# 2 Charlie 35 Los Angeles
# Filter the DataFrame
print(df[df['Age'] > 28])
# Output:
# Name Age City
# 1 Bob 30 San Francisco
# 2 Charlie 35 Los Angeles
3. Matplotlib
Matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. It’s widely used in conjunction with NumPy and Pandas for data visualization.
Key features:
- Creation of a wide range of plots and charts
- Customizable plot elements (colors, fonts, axes, etc.)
- Export to various file formats
- Integration with Jupyter notebooks for interactive plotting
Example usage:
import matplotlib.pyplot as plt
import numpy as np
# Generate data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a line plot
plt.plot(x, y)
plt.title('Sine Wave')
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.show()
4. Requests
Requests is a simple yet elegant HTTP library for Python. It simplifies the process of sending HTTP/1.1 requests and handling responses.
Key features:
- Intuitive API for making HTTP requests
- Automatic decompression of response content
- Connection pooling
- Cookie persistence across sessions
- Support for international domains and URLs
Example usage:
import requests
# Make a GET request
response = requests.get('https://api.github.com')
# Check the status code
print(response.status_code)
# Output: 200
# Print the response content
print(response.json())
5. SQLAlchemy
SQLAlchemy is a SQL toolkit and Object-Relational Mapping (ORM) library for Python. It provides a full suite of well-known enterprise-level persistence patterns, designed for efficient and high-performing database access.
Key features:
- ORM (Object-Relational Mapping)
- SQL Expression Language
- Schema migration tools
- Connection pooling
- Support for multiple database engines
Example usage:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Create an engine and base
engine = create_engine('sqlite:///example.db', echo=True)
Base = declarative_base()
# Define a model
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
# Create the table
Base.metadata.create_all(engine)
# Create a session
Session = sessionmaker(bind=engine)
session = Session()
# Add a new user
new_user = User(name='Alice', age=30)
session.add(new_user)
session.commit()
# Query the database
users = session.query(User).all()
for user in users:
print(f"{user.name}, {user.age} years old")
6. TensorFlow
TensorFlow is an open-source machine learning framework developed by Google. It’s widely used for building and deploying machine learning models, particularly deep learning models.
Key features:
- Flexible ecosystem of tools, libraries, and community resources
- Easy model building using high-level APIs like Keras
- Robust machine learning production anywhere
- Powerful experimentation for research
Example usage:
import tensorflow as tf
# Create a simple neural network
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(10,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# Generate some dummy data
import numpy as np
data = np.random.random((1000, 10))
labels = np.random.randint(2, size=(1000, 1))
# Train the model
model.fit(data, labels, epochs=10, batch_size=32)
7. Scikit-learn
Scikit-learn is a machine learning library for Python. It features various classification, regression, and clustering algorithms, including support vector machines, random forests, gradient boosting, k-means, and DBSCAN.
Key features:
- Simple and efficient tools for data mining and data analysis
- Accessible to everybody and reusable in various contexts
- Built on NumPy, SciPy, and matplotlib
- Open source, commercially usable – BSD license
Example usage:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load the iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create and train the model
clf = RandomForestClassifier(n_estimators=100)
clf.fit(X_train, y_train)
# Make predictions and calculate accuracy
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
8. Flask
Flask is a lightweight WSGI web application framework. It’s designed to make getting started quick and easy, with the ability to scale up to complex applications.
Key features:
- Built-in development server and debugger
- Integrated unit testing support
- RESTful request dispatching
- Jinja2 templating
- Support for secure cookies (client-side sessions)
Example usage:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/hello')
def hello():
return jsonify(message='Hello, World!')
if __name__ == '__main__':
app.run(debug=True)
9. Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It follows the model-template-view architectural pattern and is known for its “batteries included” philosophy.
Key features:
- ORM (Object-Relational Mapping)
- URL routing
- HTML templating
- Form handling
- Authentication system
- Admin interface
Example usage:
# In models.py
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
publication_date = models.DateField()
def __str__(self):
return self.title
# In views.py
from django.shortcuts import render
from .models import Book
def book_list(request):
books = Book.objects.all()
return render(request, 'books/book_list.html', {'books': books})
# In urls.py
from django.urls import path
from . import views
urlpatterns = [
path('books/', views.book_list, name='book_list'),
]
10. Pytest
Pytest is a testing framework that makes it easy to write simple and scalable test cases for your Python code. It’s widely used for all types of testing: from unit tests to functional and integration tests.
Key features:
- Simple syntax for writing tests
- Powerful fixture model
- Ability to reuse Django and Flask test suites
- Parallel test execution
- Detailed info on failing assert statements
Example usage:
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(-1, -1) == -2
# Run with: pytest test_file.py
Conclusion
These ten Python libraries represent a solid foundation for any Python developer, covering a wide range of applications from data analysis and machine learning to web development and testing. By mastering these libraries, you’ll be well-equipped to tackle various programming challenges and build robust, efficient applications.
Remember, the Python ecosystem is vast and constantly evolving. While these libraries are essential, it’s always beneficial to explore other libraries specific to your domain or project requirements. Continuous learning and staying updated with the latest developments in the Python community will help you become a more proficient and versatile developer.
As you progress in your Python journey, consider diving deeper into each of these libraries, exploring their advanced features and best practices. Additionally, don’t forget to practice implementing these libraries in real-world projects to gain hands-on experience and solidify your understanding.
Happy coding, and may your Python adventures be filled with exciting discoveries and successful projects!