Getting Started with Vue.js: A Lightweight Frontend Framework
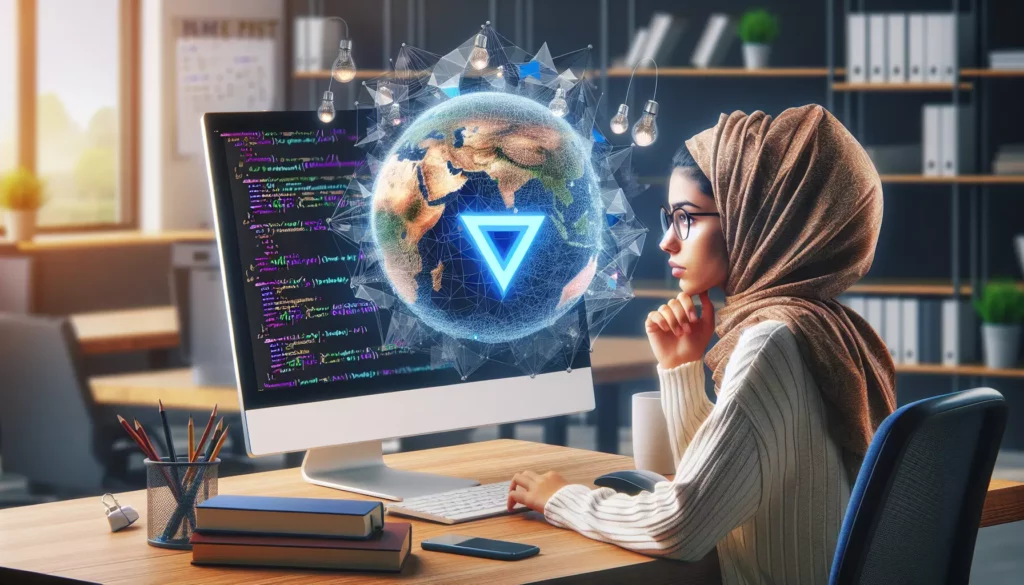
In the ever-evolving world of web development, choosing the right frontend framework can make a significant difference in your project’s success. Vue.js has emerged as a popular choice among developers due to its simplicity, flexibility, and powerful features. In this comprehensive guide, we’ll explore Vue.js, its core concepts, and how to get started with this lightweight frontend framework.
What is Vue.js?
Vue.js is a progressive JavaScript framework for building user interfaces. Created by Evan You in 2014, Vue.js has gained tremendous popularity due to its approachable learning curve and excellent performance. It allows developers to create dynamic and interactive web applications with ease.
Some key features of Vue.js include:
- Virtual DOM for efficient rendering
- Component-based architecture
- Reactive data binding
- Directives for extending HTML with custom behavior
- Computed properties and watchers
- Built-in state management with Vuex
- Vue Router for single-page applications
Setting Up Your Development Environment
Before we dive into Vue.js, let’s set up our development environment. You’ll need Node.js and npm (Node Package Manager) installed on your system. If you haven’t already, you can download and install them from the official Node.js website.
Once you have Node.js and npm installed, you can use the Vue CLI (Command Line Interface) to quickly set up a new Vue.js project. Install the Vue CLI globally by running the following command in your terminal:
npm install -g @vue/cli
With the Vue CLI installed, you can create a new Vue.js project by running:
vue create my-vue-project
Follow the prompts to select your preferred configuration. For beginners, it’s recommended to choose the default preset.
Understanding Vue.js Core Concepts
To effectively work with Vue.js, it’s essential to understand its core concepts. Let’s explore some of the fundamental ideas that make Vue.js powerful and flexible.
1. Vue Instance
At the heart of every Vue application is the Vue instance. It’s the starting point of your app and serves as the root component. Here’s a simple example of creating a Vue instance:
const app = new Vue({
el: '#app',
data: {
message: 'Hello, Vue!'
}
})
In this example, we create a new Vue instance and mount it to an element with the ID ‘app’. The `data` property contains reactive data that can be used in your template.
2. Template Syntax
Vue.js uses an HTML-based template syntax that allows you to declaratively bind the rendered DOM to the underlying Vue instance’s data. The most basic form of data binding is text interpolation using the “Mustache” syntax (double curly braces):
<div id="app">
{{ message }}
</div>
This will render the value of the `message` data property in the DOM.
3. Directives
Directives are special attributes with the `v-` prefix. They apply special reactive behavior to the rendered DOM. Some commonly used directives include:
- `v-bind`: Dynamically bind attributes or component props
- `v-if`: Conditionally render elements
- `v-for`: Render elements or components multiple times based on an array
- `v-on`: Attach event listeners to elements
- `v-model`: Create two-way data bindings on form inputs
Here’s an example using some of these directives:
<div id="app">
<p v-if="showMessage">{{ message }}</p>
<button v-on:click="toggleMessage">Toggle Message</button>
<ul>
<li v-for="item in items">{{ item }}</li>
</ul>
</div>
<script>
new Vue({
el: '#app',
data: {
message: 'Hello, Vue!',
showMessage: true,
items: ['Apple', 'Banana', 'Orange']
},
methods: {
toggleMessage() {
this.showMessage = !this.showMessage;
}
}
})
</script>
4. Components
Components are reusable Vue instances with a name. They allow you to split the UI into independent, reusable pieces. Here’s an example of a simple component:
Vue.component('greeting', {
props: ['name'],
template: '<p>Hello, {{ name }}!</p>'
})
new Vue({
el: '#app'
})
You can then use this component in your HTML like this:
<div id="app">
<greeting name="John"></greeting>
<greeting name="Jane"></greeting>
</div>
Building Your First Vue.js Application
Now that we’ve covered the basics, let’s build a simple Vue.js application to demonstrate these concepts in action. We’ll create a todo list application that allows users to add, remove, and mark tasks as completed.
First, create a new file called `index.html` and add the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue.js Todo List</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h1>{{ title }}</h1>
<input v-model="newTask" @keyup.enter="addTask" placeholder="Add a new task">
<button @click="addTask">Add Task</button>
<ul>
<li v-for="(task, index) in tasks" :key="index">
<input type="checkbox" v-model="task.completed">
<span :class="{ completed: task.completed }">{{ task.text }}</span>
<button @click="removeTask(index)">Remove</button>
</li>
</ul>
</div>
<script>
new Vue({
el: '#app',
data: {
title: 'Vue.js Todo List',
newTask: '',
tasks: []
},
methods: {
addTask() {
if (this.newTask.trim().length === 0) {
return;
}
this.tasks.push({
text: this.newTask,
completed: false
});
this.newTask = '';
},
removeTask(index) {
this.tasks.splice(index, 1);
}
}
});
</script>
<style>
.completed {
text-decoration: line-through;
color: #888;
}
</style>
</body>
</html>
This simple application demonstrates several key Vue.js concepts:
- Data binding with `{{ }}` syntax
- Two-way data binding with `v-model`
- Event handling with `@click` and `@keyup.enter`
- List rendering with `v-for`
- Conditional class binding with `:class`
- Methods for adding and removing tasks
Advanced Vue.js Concepts
As you become more comfortable with Vue.js, you’ll want to explore more advanced concepts to build more complex applications. Here are some topics to consider:
1. Computed Properties
Computed properties are cached, reactive data properties for your component. They’re useful for performing complex calculations that depend on other data. Here’s an example:
new Vue({
data: {
items: [...]
},
computed: {
filteredItems() {
// Return filtered items based on some condition
}
}
})
2. Watchers
Watchers allow you to observe changes in your data and react to those changes. They’re useful for performing asynchronous operations or complex logic in response to data changes:
new Vue({
data: {
searchQuery: ''
},
watch: {
searchQuery(newValue, oldValue) {
// Perform some action when searchQuery changes
}
}
})
3. Lifecycle Hooks
Vue components have a lifecycle, and Vue provides hooks that allow you to run code at specific stages of a component’s lifecycle. Some commonly used hooks include:
- `created`: Called after the instance is created
- `mounted`: Called after the instance has been mounted to the DOM
- `updated`: Called after a data change causes the virtual DOM to be re-rendered
- `destroyed`: Called after the instance has been destroyed
new Vue({
created() {
console.log('Component created');
},
mounted() {
console.log('Component mounted');
}
})
4. Vue Router
Vue Router is the official routing library for Vue.js. It allows you to create single-page applications with dynamic routing. Here’s a basic example of how to set up Vue Router:
import Vue from 'vue'
import VueRouter from 'vue-router'
import Home from './components/Home.vue'
import About from './components/About.vue'
Vue.use(VueRouter)
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About }
]
const router = new VueRouter({
routes
})
new Vue({
router,
render: h => h(App)
}).$mount('#app')
5. Vuex for State Management
Vuex is Vue’s official state management library. It helps you manage the state of your application in a centralized store. Here’s a simple Vuex store setup:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
},
actions: {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment')
}, 1000)
}
},
getters: {
doubleCount: state => state.count * 2
}
})
Best Practices and Tips for Vue.js Development
As you continue to work with Vue.js, keep these best practices and tips in mind:
- Use Single-File Components: Vue’s single-file components (`.vue` files) allow you to keep your template, script, and styles in one file, making your code more organized and easier to maintain.
- Follow the Vue Style Guide: Vue has an official style guide that provides recommended patterns and best practices for writing Vue.js code.
- Utilize Vue DevTools: The Vue DevTools browser extension is an invaluable tool for debugging and inspecting your Vue applications.
- Keep components small and focused: Each component should have a single responsibility, making them easier to understand, test, and reuse.
- Use props for component communication: Pass data from parent to child components using props, and emit events from child to parent components.
- Leverage Vue’s built-in directives: Familiarize yourself with Vue’s built-in directives like `v-if`, `v-for`, and `v-bind` to write more efficient and readable code.
- Use computed properties for complex calculations: Computed properties are cached and only re-evaluated when their dependencies change, making them more efficient than methods for complex calculations.
- Implement proper error handling: Use Vue’s error handling hooks and consider implementing a global error handler for your application.
- Optimize performance: Use `v-once` for static content, `v-show` for frequently toggled elements, and `keep-alive` for caching component instances.
- Write unit tests: Use tools like Jest and Vue Test Utils to write unit tests for your components and Vuex store.
Conclusion
Vue.js is a powerful and flexible frontend framework that offers a gentle learning curve for beginners while providing advanced features for experienced developers. By understanding its core concepts and following best practices, you can build efficient, maintainable, and scalable web applications.
As you continue your journey with Vue.js, remember to explore the official documentation, engage with the Vue community, and practice building various projects to reinforce your skills. With its growing ecosystem and active community, Vue.js is an excellent choice for developers looking to create modern, reactive web applications.
Whether you’re a beginner just starting out or an experienced developer looking to expand your skillset, Vue.js offers a rewarding path for frontend development. As you progress, you’ll discover that the concepts and practices you learn with Vue.js will also benefit your overall understanding of modern web development, preparing you for success in your coding journey and potentially in technical interviews with major tech companies.
Keep coding, keep learning, and enjoy the process of building amazing web applications with Vue.js!