Django vs. Flask: Which Python Framework Should You Learn?
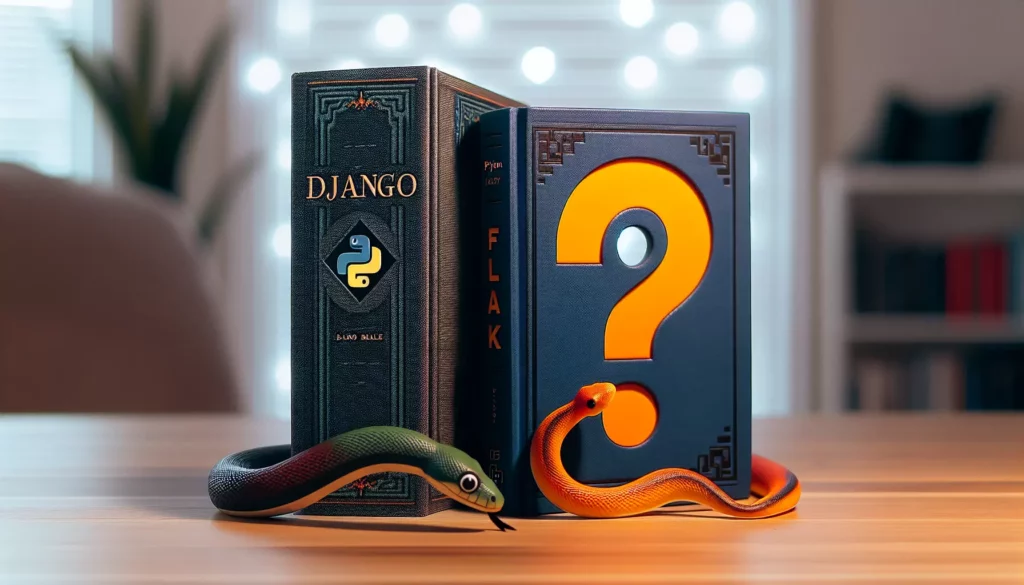
When it comes to web development using Python, two frameworks often stand out: Django and Flask. Both are powerful tools in their own right, but they cater to different needs and scenarios. As aspiring developers or those looking to expand their skillset, it’s crucial to understand the strengths and weaknesses of each framework to make an informed decision about which one to learn. In this comprehensive guide, we’ll dive deep into Django and Flask, comparing their features, use cases, and learning curves to help you decide which framework aligns best with your goals and projects.
Understanding Web Frameworks
Before we delve into the specifics of Django and Flask, let’s briefly discuss what web frameworks are and why they’re essential in modern web development.
A web framework is a collection of tools, libraries, and best practices that simplify the process of building web applications. They provide a structured way to develop web services, APIs, and full-fledged websites. Web frameworks typically handle common tasks such as:
- URL routing
- Database interactions
- Session management
- Authentication and security
- Template rendering
By using a web framework, developers can focus on writing application-specific code rather than reinventing the wheel for common functionalities. This leads to faster development times, more maintainable code, and adherence to established best practices.
Django: The Batteries-Included Framework
Django is often described as a “batteries-included” framework, meaning it comes with a wide array of built-in features and tools right out of the box. Let’s explore what makes Django a popular choice among developers.
Key Features of Django
- ORM (Object-Relational Mapping): Django’s ORM allows developers to interact with databases using Python code instead of writing SQL queries directly. This abstraction makes database operations more intuitive and less error-prone.
- Admin Interface: One of Django’s standout features is its automatically generated admin interface. With minimal configuration, you get a fully-functional CRUD (Create, Read, Update, Delete) interface for managing your application’s data.
- URL Routing: Django uses a URL configuration system that maps URLs to views, making it easy to create clean, SEO-friendly URLs for your web applications.
- Template Engine: Django’s template language is powerful yet easy to use, allowing for the separation of design from Python code.
- Forms: The framework provides a robust form-handling library that simplifies the process of creating and validating HTML forms.
- Authentication: Django includes a full-featured authentication system out of the box, handling user accounts, groups, permissions, and cookie-based user sessions.
- Security Features: Django comes with several security features enabled by default, such as protection against cross-site scripting (XSS), cross-site request forgery (CSRF), SQL injection, and clickjacking.
When to Choose Django
Django is an excellent choice for:
- Large-scale projects that require a robust, scalable foundation
- Applications that need a comprehensive admin interface
- Projects that benefit from Django’s built-in features like authentication and ORM
- Developers who prefer a structured, opinionated framework that enforces best practices
- Rapid development of complex web applications
Django Code Example
Here’s a simple example of how to define a model and create a view in Django:
# models.py
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
publication_date = models.DateField()
def __str__(self):
return self.title
# views.py
from django.shortcuts import render
from .models import Book
def book_list(request):
books = Book.objects.all()
return render(request, 'books/book_list.html', {'books': books})
Flask: The Micro-Framework
Flask, on the other hand, is often referred to as a “micro-framework.” This doesn’t mean it’s less capable than Django, but rather that it provides a lightweight core with the ability to extend functionality as needed. Let’s explore what makes Flask unique.
Key Features of Flask
- Simplicity: Flask has a small and easy-to-extend core, making it simple to get started with and understand.
- Flexibility: With Flask, you have the freedom to choose your own tools and libraries for tasks like database interaction, form validation, and authentication.
- Jinja2 Templating: Flask uses the powerful Jinja2 templating engine, which is similar to Django’s template language but with some additional features.
- WSGI Compliant: Flask is fully WSGI 1.0 compliant, ensuring compatibility with various web servers and platforms.
- Integrated Development Server: Flask comes with a built-in development server and debugger, making local development and testing straightforward.
- RESTful Request Dispatching: Flask provides intuitive handling of HTTP methods, making it easy to create RESTful APIs.
- Unicode Support: Flask has strong Unicode support, which is crucial for developing international applications.
When to Choose Flask
Flask is an excellent choice for:
- Small to medium-sized projects that don’t require a full-stack framework
- Microservices and APIs
- Developers who prefer more control over their project’s architecture and components
- Projects that need to be lightweight and have minimal overhead
- Prototyping and quick proof-of-concept applications
Flask Code Example
Here’s a simple example of how to create a basic route and view in Flask:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/<name>')
def hello(name):
return render_template('hello.html', name=name)
if __name__ == '__main__':
app.run(debug=True)
Comparing Django and Flask
Now that we’ve covered the basics of both frameworks, let’s compare them across various aspects to help you make an informed decision.
1. Learning Curve
Django: Django has a steeper learning curve due to its comprehensive nature. New developers need to understand Django’s architecture, ORM, and various built-in components. However, once mastered, Django’s structure can lead to faster development for complex applications.
Flask: Flask has a gentler learning curve, especially for beginners. Its minimalist core is easy to grasp, and developers can gradually add complexity as they learn. This makes Flask an excellent choice for those new to web development or Python.
2. Project Structure
Django: Django follows the “batteries-included” philosophy, providing a predetermined project structure. This enforced structure can be beneficial for large teams and projects, ensuring consistency across different parts of the application.
Flask: Flask gives developers the freedom to structure their projects as they see fit. While this flexibility can be advantageous for experienced developers, it may lead to inconsistencies in large projects or teams without proper guidelines.
3. Database Handling
Django: Django’s ORM is a powerful tool that abstracts database operations, supporting multiple database backends out of the box. It includes migrations, which make it easy to evolve your database schema over time.
Flask: Flask doesn’t come with an ORM, giving developers the freedom to choose their preferred database toolkit. Popular choices include SQLAlchemy or Flask-SQLAlchemy, which provide similar functionality to Django’s ORM but require additional setup.
4. Admin Interface
Django: One of Django’s standout features is its auto-generated admin interface. This can be a significant time-saver for projects that require a back-end management system.
Flask: Flask doesn’t provide an admin interface out of the box. However, extensions like Flask-Admin can be used to add similar functionality if needed.
5. Routing
Django: Django uses a URL configuration file (urls.py) to map URLs to views. This centralized approach to routing can make it easier to manage complex URL structures in large applications.
Flask: Flask uses decorators for routing, which allows for a more intuitive and straightforward way of mapping URLs to functions. This approach is particularly well-suited for smaller applications and APIs.
6. Template Engine
Django: Django has its own template engine, which is powerful and integrates seamlessly with other Django components. It includes features like template inheritance and filters.
Flask: Flask uses Jinja2, which is similar to Django’s template engine but with some additional features. Jinja2 is also used in other Python projects, making it a versatile skill to learn.
7. Community and Ecosystem
Django: Django has a larger community and ecosystem, with numerous third-party packages available. This can be beneficial when looking for solutions to common problems or seeking community support.
Flask: While Flask’s community is smaller, it’s still active and growing. The ecosystem of Flask extensions is rich, allowing developers to add functionality as needed.
Making Your Decision
Choosing between Django and Flask ultimately depends on your project requirements, personal preferences, and learning goals. Here are some guidelines to help you decide:
Choose Django if:
- You’re building a large, complex web application
- You need a robust admin interface out of the box
- You prefer a “batteries-included” approach with many built-in features
- You’re working on a project that requires rapid development of a full-stack application
- You’re part of a large team that benefits from a structured, opinionated framework
Choose Flask if:
- You’re building a small to medium-sized application or microservice
- You prefer more control over your project’s architecture and components
- You’re creating a RESTful API or a simple web service
- You’re new to web development and want a gentler learning curve
- You need a lightweight framework with minimal overhead
Learning Strategies
Regardless of which framework you choose, here are some strategies to help you learn effectively:
- Start with Python basics: Ensure you have a solid understanding of Python before diving into either framework.
- Follow official tutorials: Both Django and Flask have excellent official documentation and tutorials. Start with these to get a feel for the framework’s structure and philosophy.
- Build small projects: Apply what you’ve learned by building small projects. This hands-on experience is crucial for understanding how the framework works in practice.
- Explore the ecosystem: Familiarize yourself with popular third-party packages and extensions for your chosen framework.
- Join community forums: Participate in community discussions on platforms like Stack Overflow, Reddit, or framework-specific forums to learn from others and get help when needed.
- Contribute to open-source projects: Once you’re comfortable with the basics, consider contributing to open-source projects using your chosen framework. This can provide valuable experience and networking opportunities.
Conclusion
Both Django and Flask are excellent Python web frameworks, each with its own strengths and use cases. Django’s comprehensive approach makes it ideal for large, complex applications that benefit from its built-in features and structured environment. Flask’s simplicity and flexibility make it perfect for smaller projects, APIs, and developers who prefer more control over their application’s architecture.
Remember that the choice between Django and Flask isn’t permanent. Many developers find value in learning both frameworks, as the skills are often transferable. By understanding the strengths and weaknesses of each, you can make an informed decision based on your current needs and future goals.
Whichever framework you choose, the key to success is consistent practice and application of what you learn. Start with small projects, gradually increase complexity, and don’t hesitate to explore the rich ecosystems of both Django and Flask. Happy coding!