What Is TensorFlow? A Guide to Getting Started with Machine Learning
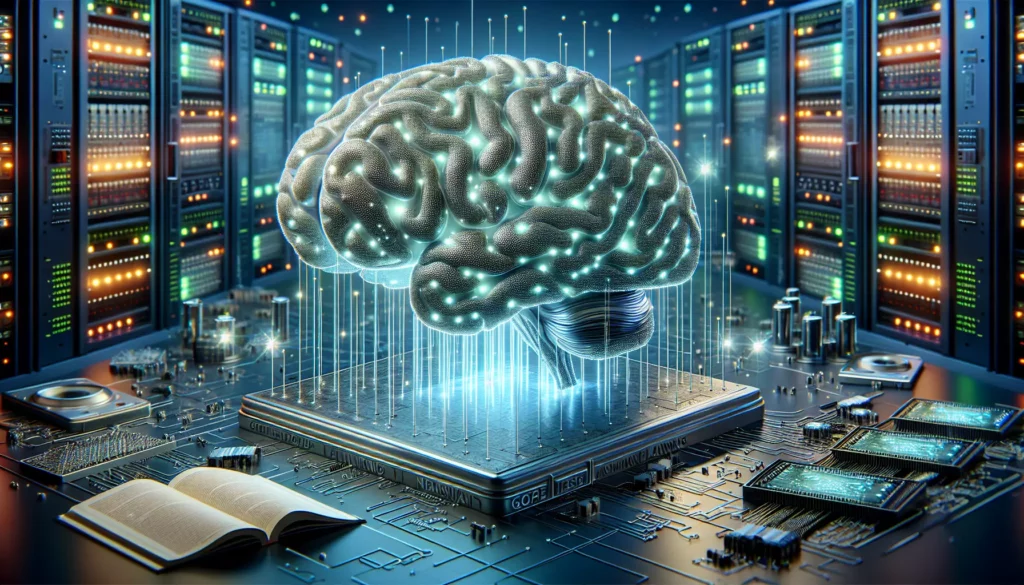
In the rapidly evolving world of technology, machine learning has emerged as a game-changing field with countless applications across industries. At the heart of many cutting-edge machine learning projects lies TensorFlow, an open-source library that has revolutionized the way developers and researchers approach artificial intelligence. In this comprehensive guide, we’ll explore what TensorFlow is, why it’s important, and how you can get started with this powerful tool for machine learning.
Understanding TensorFlow
TensorFlow is an open-source software library for numerical computation and large-scale machine learning. Developed by the Google Brain team, TensorFlow was released to the public in 2015 and has since become one of the most popular frameworks for building and deploying machine learning models.
The name “TensorFlow” comes from the operations that neural networks perform on multidimensional data arrays, which are referred to as “tensors.” In essence, TensorFlow allows you to create dataflow graphs—structures that describe how data moves through a graph, or a series of processing nodes. Each node in the graph represents a mathematical operation, while the edges represent the multidimensional data arrays (tensors) that flow between them.
Key Features of TensorFlow
- Flexibility: TensorFlow can be used for a wide range of tasks, from image and speech recognition to natural language processing and reinforcement learning.
- Scalability: It can run on multiple CPUs and GPUs, as well as mobile devices and large-scale distributed systems.
- Visualization: TensorBoard, a suite of visualization tools, allows you to inspect and understand your TensorFlow models and training processes.
- Ecosystem: A rich ecosystem of tools, libraries, and community resources supports TensorFlow development.
- Production-ready: TensorFlow models can be easily deployed in production environments across various platforms.
Why TensorFlow Matters
TensorFlow has become a cornerstone in the machine learning community for several reasons:
- Accessibility: Its high-level APIs, particularly Keras, make it easier for beginners to start building neural networks.
- Performance: TensorFlow is optimized for performance, allowing for efficient computation on various hardware platforms.
- Community Support: With a large and active community, you can find numerous resources, tutorials, and pre-trained models.
- Industry Adoption: Many tech giants and startups use TensorFlow in production, making it a valuable skill in the job market.
- Research to Production: TensorFlow bridges the gap between research and production, allowing for seamless deployment of models.
Getting Started with TensorFlow
Now that we understand what TensorFlow is and why it’s important, let’s dive into how you can start using it for your machine learning projects.
1. Installation
The first step is to install TensorFlow. The easiest way to do this is using pip, Python’s package installer. Open your terminal and run:
pip install tensorflow
For GPU support, which is recommended for faster computations, especially with large datasets, you’ll need to install the GPU version:
pip install tensorflow-gpu
Note that GPU support requires additional setup, including installing CUDA and cuDNN libraries compatible with your TensorFlow version.
2. Importing TensorFlow
Once installed, you can import TensorFlow in your Python script:
import tensorflow as tf
print(tf.__version__)
This will print the version of TensorFlow you have installed.
3. Understanding Tensors
Tensors are the fundamental building blocks in TensorFlow. They are multi-dimensional arrays that can hold data. Here’s a simple example of creating a tensor:
import tensorflow as tf
# Create a constant tensor
tensor = tf.constant([[1, 2], [3, 4]])
print(tensor)
This will create a 2×2 tensor and print it.
4. Building a Simple Neural Network
Let’s create a basic neural network using TensorFlow’s Keras API, which provides a high-level interface for building models:
import tensorflow as tf
from tensorflow import keras
# Define the model
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(10,)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(1)
])
# Compile the model
model.compile(optimizer='adam',
loss='mean_squared_error',
metrics=['mae'])
# Print model summary
model.summary()
This code defines a simple feedforward neural network with two hidden layers and an output layer. The model.summary()
function will print an overview of the model architecture.
5. Training the Model
To train the model, you’ll need some data. Let’s create some dummy data for this example:
import numpy as np
# Generate dummy data
x_train = np.random.random((1000, 10))
y_train = np.random.random((1000, 1))
# Train the model
history = model.fit(x_train, y_train, epochs=100, batch_size=32, validation_split=0.2, verbose=1)
This will train the model for 100 epochs on the dummy data we created.
6. Making Predictions
After training, you can use the model to make predictions:
# Generate some test data
x_test = np.random.random((100, 10))
# Make predictions
predictions = model.predict(x_test)
print(predictions[:5]) # Print first 5 predictions
7. Visualizing Training Progress
TensorFlow provides TensorBoard for visualizing various aspects of model training. Here’s how you can use it:
import datetime
log_dir = "logs/fit/" + datetime.datetime.now().strftime("%Y%m%d-%H%M%S")
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir=log_dir, histogram_freq=1)
# Train the model with TensorBoard callback
model.fit(x_train, y_train, epochs=100, batch_size=32,
validation_split=0.2, callbacks=[tensorboard_callback])
After training, you can launch TensorBoard by running the following command in your terminal:
tensorboard --logdir logs/fit
This will start a local server where you can view training metrics in your web browser.
Advanced TensorFlow Concepts
As you become more comfortable with TensorFlow basics, you can explore more advanced concepts:
1. Custom Layers and Models
TensorFlow allows you to create custom layers and models by subclassing tf.keras.layers.Layer
and tf.keras.Model
:
class MyCustomLayer(tf.keras.layers.Layer):
def __init__(self, output_dim, **kwargs):
self.output_dim = output_dim
super(MyCustomLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.kernel = self.add_weight(name='kernel',
shape=(input_shape[1], self.output_dim),
initializer='uniform',
trainable=True)
def call(self, inputs):
return tf.matmul(inputs, self.kernel)
# Use the custom layer
model = tf.keras.Sequential([
MyCustomLayer(64),
tf.keras.layers.Activation('relu')
])
2. TensorFlow Data API
For handling large datasets efficiently, TensorFlow provides the tf.data
API:
dataset = tf.data.Dataset.from_tensor_slices((x_train, y_train))
dataset = dataset.shuffle(buffer_size=1024).batch(32)
model.fit(dataset, epochs=100)
3. TensorFlow Distributed Strategy
For training on multiple GPUs or machines, you can use TensorFlow’s distributed strategy:
strategy = tf.distribute.MirroredStrategy()
with strategy.scope():
model = keras.Sequential([...])
model.compile(...)
4. TensorFlow Serving
For deploying models in production, TensorFlow Serving is a flexible, high-performance serving system:
import tensorflow as tf
model.save('my_model/1/')
# Convert the model
converter = tf.lite.TFLiteConverter.from_saved_model('my_model/1/')
tflite_model = converter.convert()
# Save the model
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
Best Practices for TensorFlow Development
As you continue your journey with TensorFlow, keep these best practices in mind:
- Use High-Level APIs: Start with Keras for most tasks. It’s user-friendly and can be customized when needed.
- Leverage Pre-trained Models: Use transfer learning with pre-trained models from TensorFlow Hub to speed up development and improve performance.
- Optimize for Performance: Use
tf.function
to create graph-compiled versions of your functions for better performance. - Monitor and Debug: Utilize TensorBoard for visualizing model architecture, training progress, and debugging.
- Version Control: Use version control for your code and consider using tools like MLflow for experiment tracking.
- Stay Updated: TensorFlow is constantly evolving. Keep up with the latest updates and best practices through the official documentation and community resources.
Resources for Further Learning
To deepen your understanding of TensorFlow and machine learning, consider exploring these resources:
- Official TensorFlow Tutorials
- TensorFlow in Practice Specialization on Coursera
- Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow by Aurélien Géron
- Kaggle for practical machine learning competitions and datasets
- TensorFlow GitHub Repository for the latest updates and community contributions
Conclusion
TensorFlow has revolutionized the field of machine learning, making it more accessible to developers and researchers alike. By providing a flexible and powerful framework for building and deploying machine learning models, TensorFlow has become an essential tool in the AI ecosystem.
As you begin your journey with TensorFlow, remember that the key to mastery is practice and persistence. Start with simple projects, gradually increase complexity, and don’t hesitate to experiment. The TensorFlow community is vast and supportive, so don’t hesitate to seek help when you encounter challenges.
Whether you’re aiming to build the next breakthrough AI application or simply wanting to understand the technology shaping our future, TensorFlow provides the tools and ecosystem to help you achieve your goals. As you progress, you’ll find that the skills you develop with TensorFlow are highly transferable and in high demand across various industries.
So, dive in, start coding, and unlock the potential of machine learning with TensorFlow. The future of AI is waiting for your contributions!