How to Choose Between Kotlin and Swift for Mobile Development
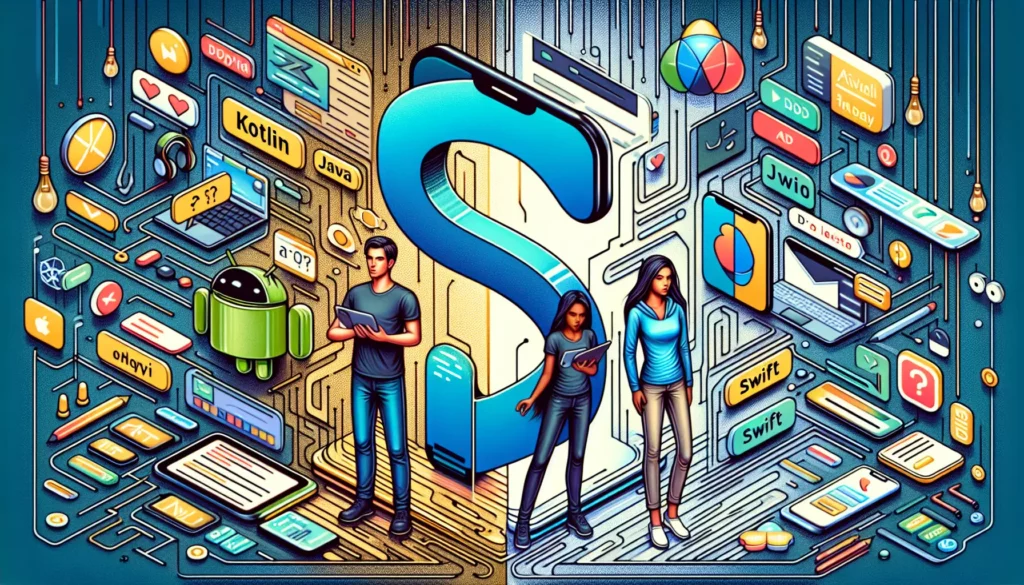
In the ever-evolving world of mobile app development, choosing the right programming language is crucial for the success of your project. Two popular languages that have gained significant traction in recent years are Kotlin and Swift. Both are modern, powerful, and designed with mobile development in mind. But how do you decide which one to use for your next mobile app? In this comprehensive guide, we’ll explore the key factors to consider when choosing between Kotlin and Swift for mobile development.
Understanding Kotlin and Swift
Before diving into the comparison, let’s briefly introduce both languages:
Kotlin
Kotlin is a statically typed programming language developed by JetBrains. It’s fully interoperable with Java and runs on the Java Virtual Machine (JVM). Google officially supports Kotlin for Android development, making it a popular choice for building Android apps.
Swift
Swift is a powerful and intuitive programming language created by Apple for iOS, macOS, watchOS, and tvOS development. It’s designed to work with Apple’s Cocoa and Cocoa Touch frameworks and can be integrated with existing Objective-C code.
Factors to Consider When Choosing Between Kotlin and Swift
1. Target Platform
The most significant factor in choosing between Kotlin and Swift is the platform you’re targeting:
- Android Development: Kotlin is the preferred choice for Android app development. It’s officially supported by Google and integrates seamlessly with Android Studio.
- iOS Development: Swift is the go-to language for iOS app development. It’s designed specifically for Apple’s ecosystem and offers the best performance and integration with iOS frameworks.
If you’re developing for both platforms, you’ll need to consider cross-platform solutions or be prepared to maintain two separate codebases.
2. Learning Curve
Both Kotlin and Swift are designed to be more concise and easier to learn compared to their predecessors (Java and Objective-C, respectively). However, there are some differences in their learning curves:
Kotlin:
- Easier transition for Java developers due to its interoperability with Java
- Concise syntax that reduces boilerplate code
- Gradual learning curve, allowing developers to start with basic Java-like syntax and progressively adopt Kotlin-specific features
Swift:
- Designed to be beginner-friendly with a clean and expressive syntax
- Playground feature in Xcode allows for interactive learning and experimentation
- Steeper learning curve for developers without prior Apple ecosystem experience
3. Performance
Both Kotlin and Swift offer excellent performance for mobile app development. However, there are some nuances to consider:
Kotlin:
- Compiles to Java bytecode, which runs on the JVM
- Performance is generally on par with Java
- Some features like coroutines can improve performance in concurrent programming scenarios
Swift:
- Compiled directly to machine code, offering potential performance advantages
- Optimized for Apple hardware, leading to excellent performance on iOS devices
- Automatic Reference Counting (ARC) for efficient memory management
In practice, the performance differences between Kotlin and Swift are often negligible for most mobile apps. The efficiency of your algorithms and overall app architecture will have a more significant impact on performance than the choice between these two languages.
4. Language Features and Syntax
Both Kotlin and Swift offer modern language features that improve developer productivity and code quality. Let’s compare some key features:
Kotlin Features:
- Null safety with nullable and non-nullable types
- Extension functions for adding functionality to existing classes
- Data classes for creating immutable value objects
- Coroutines for managing asynchronous code and concurrency
- Higher-order functions and lambdas
Swift Features:
- Optionals for handling nullable values
- Protocol-oriented programming for flexible and modular code
- Powerful enums with associated values
- Automatic Reference Counting (ARC) for memory management
- Generics for writing flexible and reusable code
Here’s a simple example comparing the syntax of Kotlin and Swift for defining a class:
Kotlin:
data class Person(val name: String, val age: Int) {
fun greet() {
println("Hello, my name is $name and I'm $age years old.")
}
}
val person = Person("Alice", 30)
person.greet()
Swift:
struct Person {
let name: String
let age: Int
func greet() {
print("Hello, my name is \(name) and I'm \(age) years old.")
}
}
let person = Person(name: "Alice", age: 30)
person.greet()
Both languages offer concise and expressive syntax, making it easy to write clean and maintainable code.
5. Development Tools and IDE Support
The quality of development tools and IDE support can significantly impact your productivity as a developer. Let’s compare the tooling ecosystems for Kotlin and Swift:
Kotlin:
- Excellent support in Android Studio, the official IDE for Android development
- JetBrains IntelliJ IDEA offers top-notch Kotlin support
- Gradle build system for dependency management and build automation
- Extensive plugin ecosystem for Android Studio and IntelliJ IDEA
Swift:
- Xcode, Apple’s IDE, provides comprehensive support for Swift development
- Swift Playgrounds for interactive learning and prototyping
- Xcode’s Interface Builder for visual UI design
- Swift Package Manager for dependency management
Both languages benefit from robust tooling support, making the development experience smooth and efficient.
6. Community and Ecosystem
A strong community and ecosystem can provide valuable resources, libraries, and support for developers. Let’s compare the communities and ecosystems of Kotlin and Swift:
Kotlin:
- Rapidly growing community with strong support from Google and JetBrains
- Access to the vast Java ecosystem and libraries
- Kotlin-specific libraries and frameworks are emerging
- Active community on platforms like Stack Overflow and GitHub
Swift:
- Large and established community of iOS developers
- Rich ecosystem of iOS-specific libraries and frameworks
- Strong support from Apple and frequent language updates
- Active community on platforms like Stack Overflow and GitHub
Both languages have vibrant communities and ecosystems, providing ample resources and support for developers.
7. Cross-Platform Development
While Kotlin and Swift are primarily used for native Android and iOS development respectively, both languages offer options for cross-platform development:
Kotlin:
- Kotlin Multiplatform allows sharing code between Android, iOS, and web projects
- Kotlin/Native enables compilation to native binaries for various platforms
- Frameworks like Kotlin Multiplatform Mobile (KMM) facilitate cross-platform mobile development
Swift:
- Swift for TensorFlow enables machine learning development across platforms
- Server-side Swift frameworks like Vapor allow backend development in Swift
- While primarily focused on Apple platforms, efforts are ongoing to improve Swift’s cross-platform capabilities
If cross-platform development is a priority, Kotlin currently offers more mature solutions for sharing code across platforms.
8. Job Market and Career Opportunities
Considering the job market and career opportunities is important when choosing a programming language to invest your time in. Let’s compare the job markets for Kotlin and Swift developers:
Kotlin:
- Growing demand for Kotlin developers in the Android ecosystem
- Increasing adoption in server-side and full-stack development
- Valuable skill for Java developers looking to transition to modern Android development
Swift:
- Strong demand for Swift developers in the iOS app development market
- Opportunities in macOS, watchOS, and tvOS development
- Emerging opportunities in server-side Swift development
Both Kotlin and Swift offer promising career opportunities, with demand likely to continue growing in their respective ecosystems.
Making the Decision: Kotlin vs. Swift
Now that we’ve explored the key factors to consider, let’s summarize when to choose Kotlin or Swift for your mobile development project:
Choose Kotlin if:
- You’re primarily targeting Android platform
- You have experience with Java and want to leverage that knowledge
- You’re interested in cross-platform development with Kotlin Multiplatform
- You want to use a language that’s also suitable for server-side development
Choose Swift if:
- You’re primarily targeting iOS, macOS, watchOS, or tvOS platforms
- You want to take full advantage of Apple’s ecosystem and frameworks
- You’re interested in developing apps with optimal performance on Apple devices
- You want to leverage Swift’s powerful features for iOS-specific development
Conclusion
Choosing between Kotlin and Swift for mobile development ultimately depends on your target platform, project requirements, and personal or team preferences. Both languages offer modern features, excellent performance, and strong community support.
If you’re developing for Android, Kotlin is the clear choice with its official support from Google and seamless integration with Android Studio. For iOS development, Swift is the way to go, offering the best performance and integration with Apple’s ecosystem.
For developers looking to target both platforms, consider exploring cross-platform solutions like Kotlin Multiplatform or frameworks like React Native or Flutter. Alternatively, maintaining separate native codebases in Kotlin and Swift can provide the best performance and user experience on each platform.
Regardless of which language you choose, both Kotlin and Swift offer exciting opportunities for mobile developers to create innovative and high-quality apps. By understanding the strengths and characteristics of each language, you can make an informed decision that aligns with your project goals and development preferences.
Further Learning Resources
To deepen your knowledge of Kotlin and Swift, consider exploring the following resources:
Kotlin Resources:
- Official Kotlin Documentation
- Android Developers Kotlin Guide
- Kotlin Playground for online experimentation
Swift Resources:
- Official Swift Documentation
- Apple’s Swift Resources
- Hacking with Swift for tutorials and projects
Remember that the best way to learn and decide between these languages is through hands-on practice. Start building small projects, experiment with different features, and gradually work your way up to more complex applications. Happy coding!