How to Master Node.js for Full Stack Development
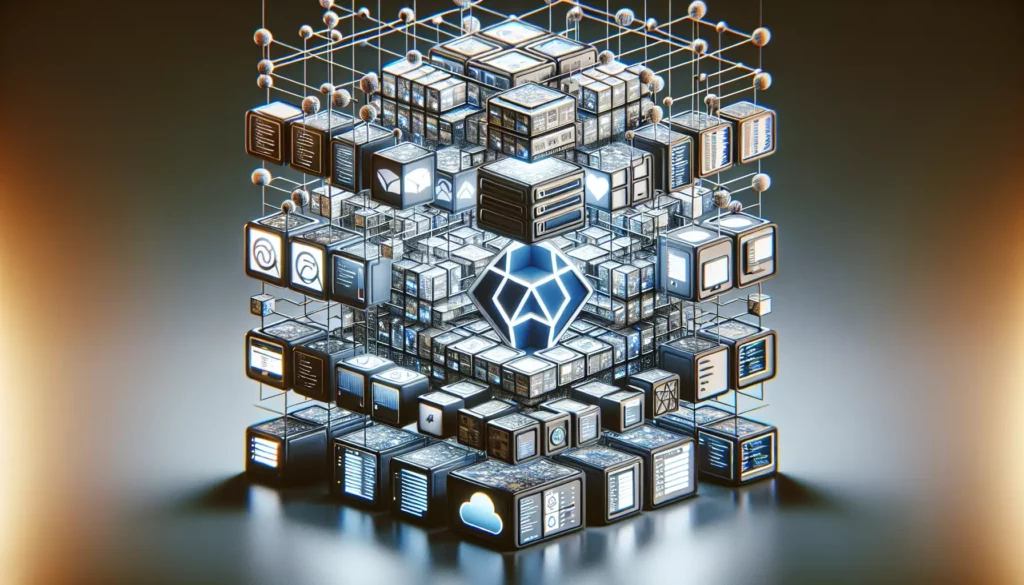
In the ever-evolving landscape of web development, mastering Node.js has become an essential skill for aspiring full stack developers. As a powerful JavaScript runtime built on Chrome’s V8 JavaScript engine, Node.js enables developers to use JavaScript for server-side programming, creating a unified language environment for both front-end and back-end development. This comprehensive guide will walk you through the process of mastering Node.js, from understanding its core concepts to implementing advanced features in full stack applications.
1. Understanding the Basics of Node.js
Before diving into the intricacies of Node.js, it’s crucial to grasp its fundamental concepts and architecture.
1.1 What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a web browser. It uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
1.2 Key Features of Node.js
- Asynchronous and Event-Driven
- Single-threaded but Highly Scalable
- No Buffering
- License – Released under the MIT license
1.3 Setting Up Your Development Environment
To start working with Node.js, you’ll need to set up your development environment:
- Download and install Node.js from the official website (nodejs.org)
- Verify the installation by running
node -v
andnpm -v
in your terminal - Choose an Integrated Development Environment (IDE) or text editor (e.g., Visual Studio Code, Sublime Text)
2. Core Concepts of Node.js
To become proficient in Node.js, you must understand its core concepts and how they contribute to its efficiency and performance.
2.1 The Event Loop
The event loop is the heart of Node.js, allowing it to perform non-blocking I/O operations despite JavaScript being single-threaded. It works by offloading operations to the system kernel whenever possible and executing callbacks when operations complete.
2.2 Modules
Node.js uses a module system to organize and reuse code. There are three types of modules:
- Core Modules: Built-in modules that come with Node.js installation
- Local Modules: Modules created locally in your Node.js application
- Third-party Modules: Modules installed via npm (Node Package Manager)
Here’s an example of how to use a core module:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
2.3 npm (Node Package Manager)
npm is the default package manager for Node.js. It allows you to install, share, and manage dependencies in your projects. Understanding how to use npm effectively is crucial for Node.js development.
2.4 Asynchronous Programming
Node.js heavily relies on asynchronous programming to achieve non-blocking I/O operations. This is typically done using callbacks, promises, or async/await syntax.
3. Building RESTful APIs with Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It’s an essential tool for building RESTful APIs in Node.js.
3.1 Setting up an Express.js Application
To create a basic Express.js application, follow these steps:
- Initialize a new Node.js project:
npm init -y
- Install Express:
npm install express
- Create an
app.js
file and set up a basic server:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
3.2 Routing in Express.js
Express.js provides a simple and intuitive way to define routes for your application. Here’s an example of how to set up routes for a RESTful API:
app.get('/api/users', (req, res) => {
// Logic to fetch users
});
app.post('/api/users', (req, res) => {
// Logic to create a new user
});
app.put('/api/users/:id', (req, res) => {
// Logic to update a user
});
app.delete('/api/users/:id', (req, res) => {
// Logic to delete a user
});
3.3 Middleware in Express.js
Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle, commonly denoted by a variable named next
.
Here’s an example of a custom middleware function:
const loggerMiddleware = (req, res, next) => {
console.log(`${new Date().toISOString()} - ${req.method} ${req.url}`);
next();
};
app.use(loggerMiddleware);
4. Working with Databases
As a full stack developer, you’ll need to interact with databases to store and retrieve data. Node.js supports various databases, both SQL and NoSQL.
4.1 MongoDB with Mongoose
MongoDB is a popular NoSQL database that works well with Node.js. Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js.
To get started with MongoDB and Mongoose:
- Install Mongoose:
npm install mongoose
- Connect to MongoDB:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/myapp', {
useNewUrlParser: true,
useUnifiedTopology: true
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', function() {
console.log('Connected to MongoDB');
});
4.2 SQL Databases with Sequelize
For SQL databases, Sequelize is a popular ORM (Object-Relational Mapping) tool that supports PostgreSQL, MySQL, SQLite, and more.
To use Sequelize with PostgreSQL:
- Install Sequelize and PostgreSQL driver:
npm install sequelize pg pg-hstore
- Set up a connection:
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: 'postgres'
});
async function testConnection() {
try {
await sequelize.authenticate();
console.log('Connection has been established successfully.');
} catch (error) {
console.error('Unable to connect to the database:', error);
}
}
testConnection();
5. Authentication and Authorization
Implementing secure authentication and authorization is crucial for protecting your application and its users.
5.1 JSON Web Tokens (JWT)
JWT is a popular method for implementing authentication in Node.js applications. Here’s a basic example of how to use JWT:
const jwt = require('jsonwebtoken');
// Generate a token
const token = jwt.sign({ userId: user.id }, 'your-secret-key', { expiresIn: '1h' });
// Verify a token
jwt.verify(token, 'your-secret-key', (err, decoded) => {
if (err) {
// Token is invalid
} else {
// Token is valid, decoded contains the payload
}
});
5.2 Passport.js
Passport is authentication middleware for Node.js that supports various authentication strategies, including local, OAuth, and more.
To use Passport with local strategy:
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
passport.use(new LocalStrategy(
function(username, password, done) {
User.findOne({ username: username }, function (err, user) {
if (err) { return done(err); }
if (!user) { return done(null, false); }
if (!user.verifyPassword(password)) { return done(null, false); }
return done(null, user);
});
}
));
6. Testing Node.js Applications
Testing is an integral part of the development process. Node.js has several testing frameworks and libraries to help you write and run tests.
6.1 Mocha and Chai
Mocha is a feature-rich JavaScript test framework running on Node.js, making asynchronous testing simple and fun. Chai is a BDD / TDD assertion library that can be paired with any JavaScript testing framework.
Here’s an example of a simple test using Mocha and Chai:
const chai = require('chai');
const expect = chai.expect;
describe('Array', function() {
describe('#indexOf()', function() {
it('should return -1 when the value is not present', function() {
expect([1, 2, 3].indexOf(4)).to.equal(-1);
});
});
});
6.2 Jest
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works with projects using Babel, TypeScript, Node, React, Angular, Vue, and more.
Here’s a basic Jest test:
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
7. Deployment and DevOps
Once you’ve built your Node.js application, you’ll need to deploy it to a production environment.
7.1 Containerization with Docker
Docker allows you to package your application and its dependencies into a container, ensuring consistency across different environments.
Here’s a basic Dockerfile for a Node.js application:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 8080
CMD [ "node", "app.js" ]
7.2 Continuous Integration and Deployment (CI/CD)
Implementing a CI/CD pipeline automates the process of testing and deploying your application. Popular tools for CI/CD include Jenkins, Travis CI, and GitLab CI.
7.3 Cloud Platforms
There are several cloud platforms where you can deploy your Node.js applications:
- Heroku
- AWS Elastic Beanstalk
- Google App Engine
- DigitalOcean
8. Performance Optimization
Optimizing your Node.js application’s performance is crucial for handling high traffic and providing a smooth user experience.
8.1 Caching
Implementing caching can significantly improve your application’s performance. Redis is a popular in-memory data structure store that can be used as a cache:
const redis = require('redis');
const client = redis.createClient();
// Set a value in Redis
client.set('key', 'value', redis.print);
// Get a value from Redis
client.get('key', (err, reply) => {
console.log(reply);
});
8.2 Load Balancing
Load balancing distributes incoming network traffic across multiple servers to ensure no single server bears too much demand. Node.js’s cluster module can be used to create a load-balanced server:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers.
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`worker ${worker.process.pid} died`);
});
} else {
// Workers can share any TCP connection
// In this case it is an HTTP server
http.createServer((req, res) => {
res.writeHead(200);
res.end('hello world\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
9. Security Best Practices
Ensuring the security of your Node.js application is paramount. Here are some best practices:
9.1 Input Validation
Always validate and sanitize user input to prevent injection attacks. You can use libraries like validator.js for this purpose:
const validator = require('validator');
if (validator.isEmail(email)) {
// Proceed with email
} else {
// Handle invalid email
}
9.2 HTTPS
Always use HTTPS in production to encrypt data in transit. You can use the https module in Node.js to create an HTTPS server:
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('key.pem'),
cert: fs.readFileSync('cert.pem')
};
https.createServer(options, function (req, res) {
res.writeHead(200);
res.end("hello world\n");
}).listen(8000);
9.3 Dependency Management
Regularly update your dependencies and use tools like npm audit to check for vulnerabilities:
npm audit
npm audit fix
10. Staying Updated and Continuous Learning
The Node.js ecosystem is constantly evolving, so it’s important to stay updated with the latest developments and best practices.
10.1 Official Documentation
Regularly refer to the official Node.js documentation (nodejs.org/docs) for the most up-to-date information on Node.js features and APIs.
10.2 Community Resources
Engage with the Node.js community through forums, blogs, and social media. Some popular resources include:
- Node.js Foundation Blog
- Node Weekly Newsletter
- Stack Overflow
- GitHub repositories of popular Node.js projects
10.3 Continuous Practice
Keep honing your skills by working on personal projects, contributing to open-source projects, or participating in coding challenges. Platforms like AlgoCademy can help you improve your algorithmic thinking and problem-solving skills, which are crucial for efficient Node.js development.
Conclusion
Mastering Node.js for full stack development is a journey that requires dedication, practice, and continuous learning. By understanding the core concepts, working with frameworks like Express.js, managing databases, implementing authentication and security measures, and staying updated with the latest trends and best practices, you can become a proficient Node.js developer.
Remember that becoming a master in any technology takes time and experience. Don’t be discouraged by challenges; instead, view them as opportunities to learn and grow. With persistence and passion, you’ll be well on your way to becoming a skilled Node.js full stack developer.
Happy coding!