Top Frameworks for Web Developers in 2024
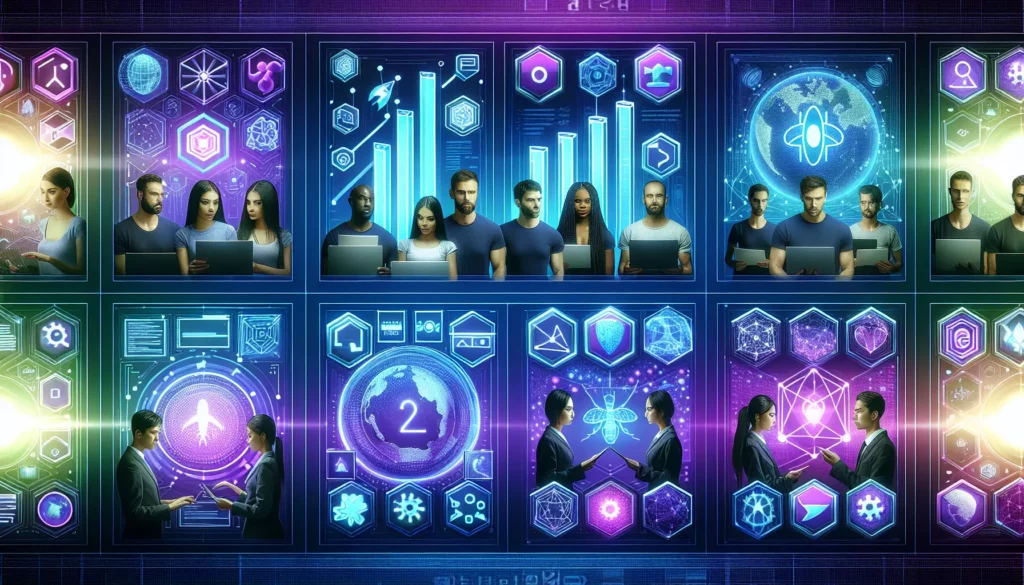
As we step into 2024, the landscape of web development continues to evolve at a rapid pace. For developers looking to stay ahead of the curve and build cutting-edge applications, choosing the right framework is crucial. In this comprehensive guide, we’ll explore the top frameworks that are shaping the future of web development. Whether you’re a seasoned developer or just starting your journey in coding, understanding these frameworks will be essential for your success in the ever-changing world of web development.
1. React
React, developed and maintained by Facebook, continues to dominate the front-end development scene in 2024. Its component-based architecture and virtual DOM make it a go-to choice for building dynamic and responsive user interfaces.
Key Features:
- Virtual DOM for optimized rendering
- Component-based architecture
- JSX syntax for easy integration of HTML with JavaScript
- Large ecosystem and community support
- React Native for cross-platform mobile development
React’s popularity stems from its flexibility and the ability to create reusable UI components. It’s particularly well-suited for single-page applications (SPAs) and large-scale projects that require complex state management.
Example of a Simple React Component:
import React from 'react';
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
This simple example demonstrates the basic structure of a React component, showcasing the use of JSX and props.
2. Vue.js
Vue.js has gained significant traction in recent years and continues to be a favorite among developers in 2024. Known for its gentle learning curve and excellent documentation, Vue.js is perfect for both small projects and large-scale applications.
Key Features:
- Progressive framework that can be adopted incrementally
- Virtual DOM for efficient updates
- Reactive data binding
- Component-based architecture
- Official routing and state management libraries
Vue.js excels in creating interactive web interfaces and is often praised for its simplicity and performance. It’s an excellent choice for developers who want a powerful framework without the steep learning curve.
Example of a Vue.js Component:
<template>
<div>
<h1>{{ message }}</h1>
<button @click="reverseMessage">Reverse Message</button>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello Vue!'
}
},
methods: {
reverseMessage() {
this.message = this.message.split('').reverse().join('')
}
}
}
</script>
This example showcases Vue’s template syntax and reactivity system, demonstrating how easy it is to create interactive components.
3. Angular
Angular, maintained by Google, remains a powerhouse in the world of web development frameworks in 2024. It’s a complete solution for building complex, enterprise-grade applications.
Key Features:
- TypeScript-based, offering strong typing and OOP features
- Comprehensive framework with built-in tools for routing, forms, and HTTP clients
- Dependency injection for better testability and maintainability
- Powerful CLI for project scaffolding and management
- RxJS integration for reactive programming
Angular is ideal for large-scale applications that require a robust, opinionated framework. Its structure enforces best practices and maintainability, making it a favorite for enterprise development.
Example of an Angular Component:
import { Component } from '@angular/core';
@Component({
selector: 'app-hello',
template: `
<h1>Hello, {{ name }}!</h1>
<button (click)="greet()">Greet</button>
`
})
export class HelloComponent {
name: string = 'World';
greet() {
alert(`Hello, ${this.name}!`);
}
}
This example demonstrates Angular’s component structure, including the use of decorators, templates, and TypeScript.
4. Svelte
Svelte has been gaining momentum and is considered one of the rising stars in the web development world in 2024. Unlike traditional frameworks, Svelte shifts much of its work to compile-time, resulting in smaller bundle sizes and better runtime performance.
Key Features:
- Compile-time framework with no virtual DOM
- Reactive by default
- Less boilerplate code
- Built-in state management
- Smaller bundle sizes compared to other frameworks
Svelte is excellent for projects where performance is a top priority. Its simplicity and efficiency make it a great choice for both small and large applications.
Example of a Svelte Component:
<script>
let count = 0;
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
This example shows how Svelte combines HTML, CSS, and JavaScript in a single file, with reactive variables that automatically update the DOM.
5. Next.js
Next.js, built on top of React, has become increasingly popular for its server-side rendering capabilities and excellent developer experience. In 2024, it’s a top choice for building performant and SEO-friendly React applications.
Key Features:
- Server-side rendering and static site generation
- Automatic code splitting for faster page loads
- Built-in CSS support
- API routes for building API endpoints
- Easy deployment with Vercel platform
Next.js is particularly well-suited for content-heavy websites, e-commerce platforms, and applications that require SEO optimization.
Example of a Next.js Page:
import { useEffect, useState } from 'react'
function HomePage() {
const [data, setData] = useState(null)
useEffect(() => {
fetch('/api/data')
.then((res) => res.json())
.then((data) => setData(data))
}, [])
if (!data) return <div>Loading...</div>
return (
<main>
<h1>{data.title}</h1>
<p>{data.description}</p>
</main>
)
}
export default HomePage
This example demonstrates a basic Next.js page with server-side data fetching, showcasing how easy it is to create dynamic, SEO-friendly pages.
6. Express.js
While front-end frameworks often steal the spotlight, back-end frameworks are equally crucial. Express.js remains the go-to choice for building robust, scalable server-side applications with Node.js in 2024.
Key Features:
- Minimalist and flexible web application framework
- Easy integration with various databases and ORMs
- Middleware support for extending functionality
- Large ecosystem of plugins and extensions
- Excellent performance and scalability
Express.js is ideal for building RESTful APIs, microservices, and full-stack JavaScript applications. Its simplicity and flexibility make it a favorite among developers of all skill levels.
Example of an Express.js Server:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
This simple example shows how easy it is to set up a basic server with Express.js, handling a GET request to the root route.
7. Django
For Python enthusiasts, Django continues to be a powerful and popular framework for web development in 2024. Known for its “batteries-included” philosophy, Django provides a complete solution for building web applications quickly and efficiently.
Key Features:
- ORM (Object-Relational Mapping) for database interactions
- Built-in admin interface
- Robust security features
- URL routing
- Template engine for dynamic HTML
- Extensive ecosystem of packages and plugins
Django is particularly well-suited for content management systems, social networks, and scientific computing platforms. Its emphasis on the DRY (Don’t Repeat Yourself) principle and rapid development makes it a favorite for startups and established companies alike.
Example of a Django View:
from django.http import HttpResponse
from django.views import View
class HelloWorldView(View):
def get(self, request):
return HttpResponse("Hello, World!")
# In urls.py
from django.urls import path
from .views import HelloWorldView
urlpatterns = [
path('hello/', HelloWorldView.as_view(), name='hello_world'),
]
This example demonstrates a simple Django view and how to route it, showcasing Django’s class-based views and URL routing system.
8. Ruby on Rails
Ruby on Rails, often simply called Rails, continues to be a popular choice for rapid web application development in 2024. Known for its convention over configuration approach, Rails allows developers to build robust web applications with less code and in less time.
Key Features:
- MVC (Model-View-Controller) architecture
- Active Record for database management
- Built-in testing framework
- RESTful application design
- Rich ecosystem of gems (plugins)
- Asset pipeline for managing static assets
Rails is particularly well-suited for startups and projects that require rapid prototyping and iteration. Its emphasis on developer happiness and productivity makes it a favorite for building content management systems, e-commerce platforms, and social networking sites.
Example of a Rails Controller:
class ArticlesController < ApplicationController
def index
@articles = Article.all
end
def show
@article = Article.find(params[:id])
end
def new
@article = Article.new
end
def create
@article = Article.new(article_params)
if @article.save
redirect_to @article
else
render 'new'
end
end
private
def article_params
params.require(:article).permit(:title, :text)
end
end
This example shows a basic Rails controller for managing articles, demonstrating Rails’ RESTful conventions and strong params for security.
9. Flutter (for Web)
While primarily known for mobile development, Flutter’s web support has matured significantly by 2024, making it a compelling option for cross-platform development that includes web applications.
Key Features:
- Single codebase for mobile, web, and desktop applications
- Rich set of pre-designed widgets
- Hot reload for rapid development
- High performance with custom rendering engine
- Strong typing with Dart language
Flutter for web is particularly useful for businesses looking to maintain a consistent user experience across multiple platforms with a single codebase.
Example of a Flutter Web Component:
import 'package:flutter/material.dart';
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Web Demo'),
),
body: Center(
child: Text(
'Welcome to Flutter for Web!',
style: Theme.of(context).textTheme.headline4,
),
),
);
}
}
This example demonstrates a simple Flutter widget that can be used in a web application, showcasing Flutter’s widget-based UI construction.
10. Phoenix (Elixir)
For developers looking to leverage the power of functional programming in web development, Phoenix, built on the Elixir language, has gained significant traction by 2024. It’s known for its high performance and scalability, making it an excellent choice for real-time applications.
Key Features:
- Built on the Elixir language, offering functional programming paradigms
- Real-time capabilities with Phoenix Channels
- High concurrency and fault-tolerance with the Erlang VM
- Productivity-focused with features like live reloading
- Strong support for WebSockets
Phoenix is particularly well-suited for applications that require real-time features, high concurrency, and scalability, such as chat applications, gaming platforms, and IoT systems.
Example of a Phoenix Controller:
defmodule MyAppWeb.PageController do
use MyAppWeb, :controller
def index(conn, _params) do
render(conn, "index.html")
end
def hello(conn, %{"name" => name}) do
render(conn, "hello.html", name: name)
end
end
This example shows a basic Phoenix controller with two actions, demonstrating Phoenix’s simplicity in handling HTTP requests and rendering responses.
Conclusion
As we navigate through 2024, the world of web development continues to offer a diverse array of frameworks and tools. From the established giants like React and Angular to rising stars like Svelte and Phoenix, there’s no shortage of options for developers to build powerful, efficient, and scalable web applications.
When choosing a framework, consider factors such as:
- The specific requirements of your project
- Your team’s expertise and learning curve
- Performance needs
- Scalability requirements
- Community support and ecosystem
Remember, the best framework is often the one that aligns most closely with your project goals and team capabilities. As the web development landscape continues to evolve, staying informed about these top frameworks will help you make informed decisions and build cutting-edge web applications.
Whether you’re a seasoned developer or just starting your journey in web development, mastering one or more of these frameworks will undoubtedly enhance your skills and open up new opportunities in the ever-expanding world of web development. Happy coding!