Best Programming Languages for Web Development in 2023
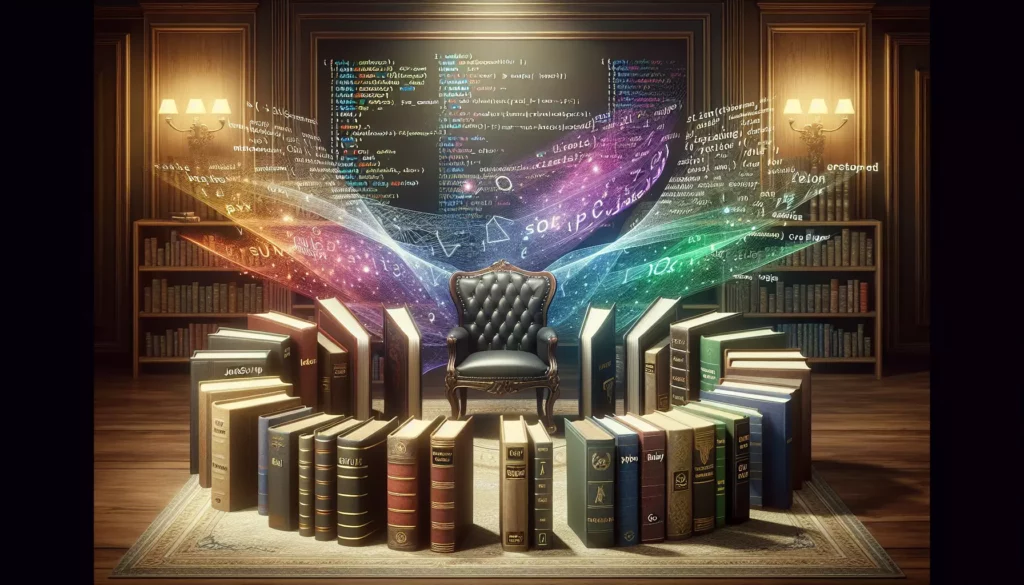
In the ever-evolving landscape of web development, choosing the right programming language is crucial for building robust, efficient, and scalable web applications. As we navigate through 2023, the demand for skilled web developers continues to soar, making it essential for aspiring programmers and seasoned developers alike to stay updated on the most popular and effective languages in the field. This comprehensive guide will explore the best programming languages for web development, their unique features, and how they can be leveraged to create cutting-edge web solutions.
1. JavaScript: The Backbone of Modern Web Development
JavaScript remains the undisputed king of web development, and for good reason. As a versatile, high-level, and interpreted programming language, JavaScript is essential for creating dynamic and interactive web pages.
Key Features:
- Client-side scripting for enhanced user experiences
- Server-side development with Node.js
- Extensive library and framework ecosystem (React, Angular, Vue.js)
- Asynchronous programming capabilities
- JSON support for data interchange
JavaScript’s popularity stems from its ability to run in any web browser without the need for additional software. With the introduction of Node.js, JavaScript has also become a powerful tool for server-side development, allowing developers to use a single language for both front-end and back-end programming.
Example of a simple JavaScript function:
function greet(name) {
return `Hello, ${name}! Welcome to AlgoCademy.`;
}
console.log(greet("Alice")); // Output: Hello, Alice! Welcome to AlgoCademy.
For those looking to excel in web development, mastering JavaScript is non-negotiable. Its versatility and widespread adoption make it an excellent choice for beginners and experienced developers alike.
2. Python: Simplicity Meets Power
Python has gained significant traction in web development due to its simplicity, readability, and robust libraries. While traditionally associated with data science and machine learning, Python has become increasingly popular for web development, especially for backend systems.
Key Features:
- Easy to learn and read syntax
- Extensive standard library and third-party packages
- Powerful web frameworks like Django and Flask
- Strong support for AI and machine learning integration
- Cross-platform compatibility
Python’s “batteries included” philosophy means that it comes with a comprehensive standard library, reducing the need for external dependencies. This, combined with its clean and readable syntax, makes Python an excellent choice for rapid development and prototyping.
Example of a simple Python web server using Flask:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "<p>Welcome to AlgoCademy!</p>"
if __name__ == "__main__":
app.run(debug=True)
Python’s versatility extends beyond web development, making it a valuable language for developers who want to explore other areas of programming, such as data analysis, artificial intelligence, and automation.
3. TypeScript: JavaScript with Superpowers
TypeScript, a superset of JavaScript, has gained immense popularity in recent years, especially for large-scale web applications. It adds optional static typing, classes, and modules to JavaScript, making it easier to write and maintain complex codebases.
Key Features:
- Static typing for improved code quality and maintainability
- Enhanced IDE support with better autocompletion and refactoring tools
- Compatibility with existing JavaScript code
- Object-oriented programming features
- Compile-time error checking
TypeScript is particularly beneficial for large teams working on extensive projects, as it helps catch errors early in the development process and improves code documentation through type annotations.
Example of TypeScript code with type annotations:
interface User {
name: string;
age: number;
}
function greetUser(user: User): string {
return `Hello, ${user.name}! You are ${user.age} years old.`;
}
const alice: User = { name: "Alice", age: 30 };
console.log(greetUser(alice)); // Output: Hello, Alice! You are 30 years old.
As TypeScript compiles to JavaScript, it can be used in any environment that supports JavaScript, making it a versatile choice for both front-end and back-end development.
4. Ruby: Designed for Developer Happiness
Ruby, with its elegant syntax and powerful web framework Ruby on Rails, has long been a favorite among web developers. Known for its emphasis on simplicity and productivity, Ruby is an excellent choice for rapid application development.
Key Features:
- Expressive and readable syntax
- Convention over configuration principle
- Robust ecosystem with gems (libraries)
- Strong community support
- Built-in testing frameworks
Ruby on Rails, the most popular web framework for Ruby, follows the “Don’t Repeat Yourself” (DRY) principle and emphasizes convention over configuration, allowing developers to build web applications quickly and efficiently.
Example of a simple Ruby class:
class Greeter
def initialize(name)
@name = name
end
def greet
puts "Hello, #{@name}! Welcome to AlgoCademy."
end
end
greeter = Greeter.new("Bob")
greeter.greet # Output: Hello, Bob! Welcome to AlgoCademy.
While Ruby may not be as widely used as some other languages on this list, its focus on developer happiness and productivity makes it a valuable skill for web developers, especially those working on startups or agile projects.
5. PHP: The Web’s Workhorse
Despite facing criticism over the years, PHP remains one of the most widely used server-side scripting languages for web development. Its ease of use, extensive documentation, and vast ecosystem make it a popular choice, especially for content management systems and e-commerce platforms.
Key Features:
- Easy to learn and use
- Large community and extensive documentation
- Seamless database integration
- Wide range of frameworks (Laravel, Symfony, CodeIgniter)
- Excellent hosting support
PHP powers many popular content management systems like WordPress, Drupal, and Joomla, making it an essential skill for developers working with these platforms.
Example of a simple PHP script:
<?php
function greet($name) {
return "Hello, $name! Welcome to AlgoCademy.";
}
$message = greet("Charlie");
echo $message; // Output: Hello, Charlie! Welcome to AlgoCademy.
?>
While PHP may not be the trendiest language in web development, its ubiquity and continued evolution (with recent versions significantly improving performance and adding modern features) ensure its relevance in the web development landscape.
6. Java: Enterprise-Grade Web Applications
Java, known for its “write once, run anywhere” philosophy, remains a popular choice for building large-scale, enterprise-level web applications. Its robustness, security features, and extensive ecosystem make it ideal for complex, high-performance web systems.
Key Features:
- Platform independence
- Strong typing and object-oriented programming
- Excellent performance and scalability
- Rich set of APIs and libraries
- Robust security features
Java’s strength lies in its ability to handle complex business logic and integrate with various enterprise systems. Frameworks like Spring and JavaServer Faces (JSF) make it easier to develop web applications using Java.
Example of a simple Java servlet:
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class HelloServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Welcome to AlgoCademy!</h1>");
out.println("</body></html>");
}
}
While Java may have a steeper learning curve compared to some other languages on this list, its widespread use in enterprise environments makes it a valuable skill for developers aiming for careers in large corporations or complex web projects.
7. C#: Microsoft’s Powerhouse for Web Development
C# (pronounced C-sharp) is a versatile, object-oriented programming language developed by Microsoft. While it’s commonly associated with Windows desktop application development, C# has become increasingly popular for web development, especially with the advent of .NET Core and its cross-platform capabilities.
Key Features:
- Strong typing and object-oriented design
- Cross-platform development with .NET Core
- Excellent performance and scalability
- Robust framework support (ASP.NET Core)
- Integration with Microsoft technologies
C# excels in building robust, scalable web applications, particularly in enterprise environments. The ASP.NET Core framework provides a powerful toolset for creating modern web applications and APIs.
Example of a simple C# web API controller:
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("[controller]")]
public class GreetingController : ControllerBase
{
[HttpGet("{name}")]
public IActionResult Get(string name)
{
return Ok($"Hello, {name}! Welcome to AlgoCademy.");
}
}
For developers working in Microsoft-centric environments or those looking to build cross-platform web applications with high performance requirements, C# is an excellent choice.
8. Go: Simplicity and Performance Combined
Go, also known as Golang, is a statically typed, compiled language developed by Google. While relatively young compared to some other languages on this list, Go has gained significant traction in web development, particularly for building microservices and high-performance web applications.
Key Features:
- Simplicity and ease of learning
- Excellent performance and low resource consumption
- Built-in concurrency support
- Fast compilation times
- Strong standard library for web development
Go’s simplicity and performance make it an attractive option for developers building scalable web services, especially in cloud environments. Its built-in concurrency features are particularly useful for handling multiple simultaneous connections efficiently.
Example of a simple Go web server:
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to AlgoCademy!")
})
http.ListenAndServe(":8080", nil)
}
While Go may not have as extensive a library ecosystem as some other languages, its performance benefits and simplicity make it a compelling choice for certain types of web projects, particularly those requiring high concurrency and low latency.
9. Rust: Safety and Performance for the Web
Rust, while primarily known for systems programming, has been gaining traction in web development circles. Its focus on memory safety, concurrency, and performance makes it an interesting choice for developers looking to build high-performance web applications and services.
Key Features:
- Memory safety without garbage collection
- Concurrency without data races
- Zero-cost abstractions
- Pattern matching and powerful type system
- Growing ecosystem for web development
While Rust has a steeper learning curve compared to some other languages on this list, its benefits in terms of performance and safety can be significant for certain types of web applications, particularly those requiring low-level control and high performance.
Example of a simple Rust web server using Rocket:
#[macro_use] extern crate rocket;
#[get("/")]
fn index() -> &'static str {
"Welcome to AlgoCademy!"
}
#[launch]
fn rocket() -> _ {
rocket::build().mount("/", routes![index])
}
Rust’s adoption in web development is still growing, but its unique features make it a language worth watching, especially for developers interested in building high-performance, secure web services.
Choosing the Right Language for Your Web Development Journey
Selecting the best programming language for web development depends on various factors, including:
- Project requirements and scale
- Development team expertise
- Performance needs
- Ecosystem and community support
- Long-term maintainability
While JavaScript remains a must-learn language for any web developer, diversifying your skill set with languages like Python, TypeScript, or Go can open up new opportunities and allow you to tackle a wider range of web development challenges.
At AlgoCademy, we believe in empowering developers with the knowledge and skills needed to excel in the ever-evolving field of web development. Our interactive coding tutorials and AI-powered assistance can help you master these languages and prepare for technical interviews at major tech companies.
Remember, the best language to learn is often the one that aligns with your goals, interests, and the type of web development you want to pursue. Whether you’re building interactive front-end experiences, robust back-end systems, or full-stack applications, there’s a language (or combination of languages) that’s right for you.
Conclusion
The world of web development is vast and constantly evolving. While this list covers some of the best programming languages for web development in 2023, it’s important to stay curious and open to learning new technologies as they emerge. Each language has its strengths and ideal use cases, and becoming proficient in multiple languages can make you a more versatile and valuable web developer.
As you embark on your web development journey or look to expand your skill set, consider exploring the languages that resonate with your interests and career goals. Remember that practical experience is key – build projects, contribute to open-source, and never stop learning. With dedication and the right resources, you can master the languages that will shape the future of the web.
Happy coding, and welcome to the exciting world of web development!