Learning Specific Languages and Frameworks: A Comprehensive Guide
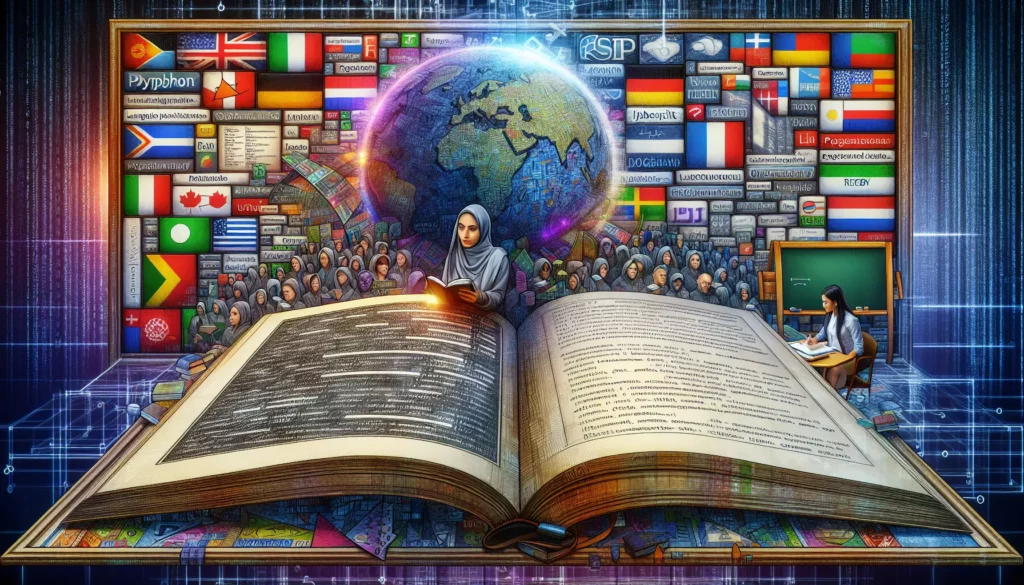
In the ever-evolving world of technology, mastering specific programming languages and frameworks is crucial for aspiring developers and seasoned professionals alike. Whether you’re just starting your coding journey or looking to expand your skill set, understanding how to effectively learn and apply these tools is essential. This comprehensive guide will walk you through the process of learning specific languages and frameworks, providing valuable insights and strategies to help you succeed in your programming endeavors.
Why Focus on Specific Languages and Frameworks?
Before diving into the learning process, it’s important to understand why focusing on specific languages and frameworks is beneficial:
- Specialization: Mastering particular languages and frameworks allows you to become an expert in specific areas of development.
- Career Opportunities: Many job listings require proficiency in certain languages or frameworks, making specialization a valuable asset in the job market.
- Efficiency: Knowing the right tool for the job can significantly increase your productivity and the quality of your work.
- Problem-Solving: Different languages and frameworks offer unique approaches to problem-solving, broadening your perspective as a developer.
Choosing the Right Language or Framework
Selecting the appropriate language or framework to learn is a critical first step. Consider the following factors:
- Career Goals: Research the technologies used in your desired field or industry.
- Project Requirements: If you have a specific project in mind, choose technologies that align with its needs.
- Learning Curve: Consider your current skill level and the complexity of the language or framework.
- Community and Resources: Look for languages and frameworks with active communities and abundant learning resources.
- Market Demand: Research job listings to identify in-demand skills in your area or desired job market.
Effective Learning Strategies
Once you’ve chosen a language or framework to focus on, employ these strategies to maximize your learning:
1. Start with the Fundamentals
Begin by mastering the basics of the language or framework. This includes:
- Syntax and basic structures
- Data types and variables
- Control structures (if statements, loops, etc.)
- Functions and methods
- Object-oriented programming concepts (if applicable)
For frameworks, understand the core concepts and architecture before diving into specific features.
2. Utilize Official Documentation
Official documentation is often the most reliable and up-to-date source of information. Make it a habit to refer to the documentation regularly as you learn and work with the language or framework.
3. Practice Regularly
Consistent practice is key to mastering any programming skill. Set aside dedicated time each day or week to work on coding exercises, projects, or tutorials.
4. Build Projects
Apply your knowledge by building real-world projects. This hands-on experience will help solidify your understanding and give you practical skills to showcase to potential employers.
5. Engage with the Community
Join online forums, attend meetups, or participate in open-source projects related to your chosen language or framework. Engaging with the community can provide valuable insights, networking opportunities, and support.
6. Use Interactive Learning Platforms
Platforms like AlgoCademy offer interactive coding tutorials and resources specifically designed to help learners progress from beginner-level coding to advanced topics. These platforms often provide:
- Step-by-step guidance
- Hands-on coding exercises
- AI-powered assistance
- Progress tracking
7. Read and Analyze Code
Study well-written code in your chosen language or framework. This can help you understand best practices, coding styles, and advanced techniques.
8. Teach Others
Explaining concepts to others can deepen your own understanding. Consider starting a blog, creating tutorials, or mentoring other learners.
Common Challenges and How to Overcome Them
Learning a new language or framework can be challenging. Here are some common obstacles and strategies to overcome them:
1. Information Overload
Challenge: The vast amount of information available can be overwhelming.
Solution: Break your learning into manageable chunks and focus on one concept at a time. Create a structured learning plan and stick to it.
2. Imposter Syndrome
Challenge: Feeling like you’re not making progress or that you’re not “good enough” compared to others.
Solution: Remember that everyone starts as a beginner. Track your progress, celebrate small wins, and focus on your own growth rather than comparing yourself to others.
3. Debugging Difficulties
Challenge: Struggling to identify and fix errors in your code.
Solution: Develop strong debugging skills by:
- Learning to use debugging tools effectively
- Breaking down problems into smaller, manageable parts
- Practicing rubber duck debugging (explaining your code out loud)
- Seeking help from the community when stuck
4. Keeping Up with Updates
Challenge: Languages and frameworks evolve rapidly, making it difficult to stay current.
Solution: Follow official blogs, subscribe to newsletters, and regularly review release notes. Focus on understanding core concepts, which often remain stable even as syntax or features change.
Advanced Learning Techniques
As you progress in your learning journey, consider these advanced techniques to further enhance your skills:
1. Deep Dive into Source Code
For open-source languages and frameworks, studying the source code can provide valuable insights into how things work under the hood. This can lead to a deeper understanding and the ability to contribute to the project.
2. Contribute to Open Source
Contributing to open-source projects is an excellent way to gain real-world experience, collaborate with other developers, and improve your coding skills. Start with small contributions like documentation improvements or bug fixes, and gradually work your way up to more complex features.
3. Implement Design Patterns
Learn and implement common design patterns in your chosen language or framework. This will improve your code structure and problem-solving abilities. Some important design patterns to study include:
- Singleton
- Factory
- Observer
- Strategy
- Decorator
4. Write Unit Tests
Develop the habit of writing unit tests for your code. This practice will help you:
- Ensure your code works as expected
- Improve code quality and maintainability
- Catch bugs early in the development process
- Gain a deeper understanding of your code’s behavior
5. Explore Advanced Features
Once you’ve mastered the basics, delve into advanced features and concepts specific to your chosen language or framework. For example:
- Asynchronous programming
- Metaprogramming
- Memory management
- Performance optimization techniques
6. Cross-pollinate with Related Technologies
Expand your knowledge by learning related technologies that complement your chosen language or framework. For instance, if you’re focusing on a front-end framework like React, consider learning about:
- State management libraries (e.g., Redux, MobX)
- Build tools (e.g., Webpack, Babel)
- Testing frameworks (e.g., Jest, Cypress)
Preparing for Technical Interviews
As you progress in your learning journey, you may want to prepare for technical interviews, especially if you’re aiming for positions at major tech companies. Here are some tips to help you prepare:
1. Focus on Algorithmic Thinking
Many technical interviews, especially at FAANG companies, emphasize algorithmic problem-solving. Practice solving algorithmic problems using your chosen language. Platforms like AlgoCademy offer resources specifically tailored for this purpose.
2. Master Data Structures
Ensure you have a solid understanding of common data structures and their implementations in your chosen language. Key data structures to focus on include:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
3. Time and Space Complexity Analysis
Learn to analyze the time and space complexity of your solutions. This skill is crucial for optimizing your code and is often a focus in technical interviews.
4. Mock Interviews
Practice with mock interviews, either with peers or using online platforms that simulate interview conditions. This will help you get comfortable with explaining your thought process and coding under pressure.
5. System Design
For more senior positions, study system design principles and practice designing scalable systems. This often involves understanding:
- Distributed systems
- Database design
- Caching strategies
- Load balancing
- Microservices architecture
Continuing Education and Staying Current
The field of technology is constantly evolving, and it’s important to stay up-to-date with the latest developments in your chosen language or framework. Here are some strategies for continuing your education:
1. Follow Industry Blogs and Publications
Stay informed about new features, best practices, and industry trends by regularly reading relevant blogs and publications. Some popular resources include:
- Official blogs of the language or framework
- Tech news websites (e.g., TechCrunch, Hacker News)
- Developer-focused platforms (e.g., Dev.to, Medium)
2. Attend Conferences and Workshops
Participate in conferences, workshops, and webinars related to your chosen technology. These events often showcase the latest developments and provide opportunities to network with other professionals.
3. Experiment with New Features
When new features are released, take the time to experiment with them and understand their use cases. This hands-on approach will help you stay current and may inspire new ideas for your projects.
4. Engage in Continuous Learning
Set aside time regularly to learn new concepts or improve your existing skills. This could involve:
- Taking online courses
- Reading technical books
- Working on side projects
- Participating in coding challenges
5. Diversify Your Skill Set
While specializing in a particular language or framework is valuable, having a broader understanding of different technologies can make you a more well-rounded developer. Consider learning complementary skills, such as:
- DevOps practices
- Cloud computing platforms
- Mobile development
- Machine learning and AI
Conclusion
Learning specific languages and frameworks is a journey that requires dedication, persistence, and a strategic approach. By following the strategies outlined in this guide, you can effectively master your chosen technology and continuously improve your skills. Remember that the key to success lies in consistent practice, engaging with the community, and staying curious about new developments in your field.
As you progress in your learning journey, platforms like AlgoCademy can provide valuable resources and support, especially when preparing for technical interviews or tackling advanced topics. Embrace the challenges, celebrate your progress, and enjoy the process of becoming a skilled developer in your chosen language or framework.
Happy coding, and may your learning journey be both rewarding and enjoyable!