Why Solving the Same Problem in Multiple Ways Improves Your Skills
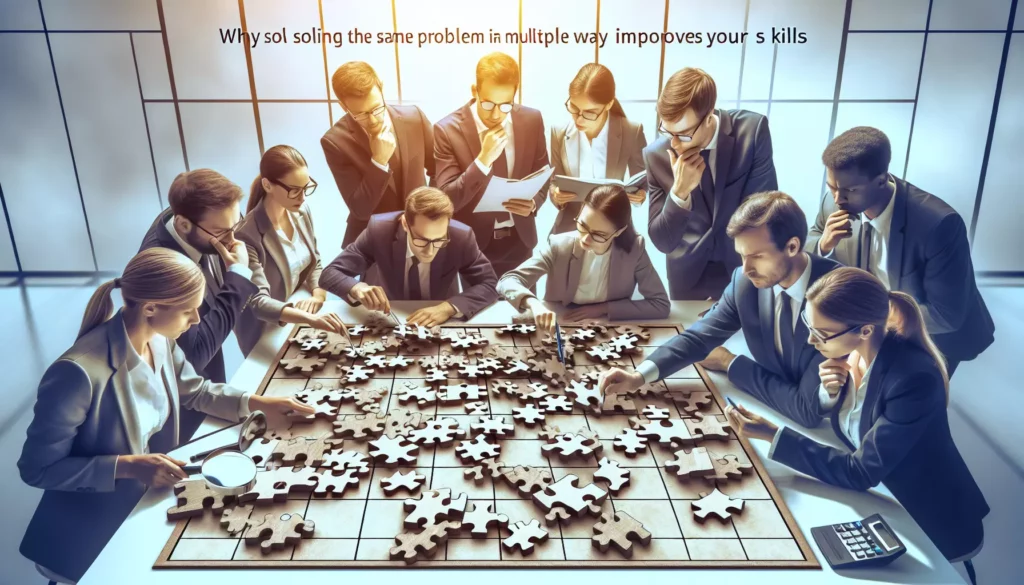
In the world of programming and software development, there’s a common misconception that once you’ve solved a problem, you should move on to the next challenge. However, experienced developers and coding educators know that there’s immense value in revisiting problems and solving them in multiple ways. This practice, often overlooked by beginners, is a powerful technique for improving your coding skills, deepening your understanding of algorithms, and preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
In this comprehensive guide, we’ll explore why solving the same problem in multiple ways is not just beneficial, but essential for your growth as a programmer. We’ll dive into the various advantages this approach offers, provide practical examples, and offer strategies for incorporating this technique into your learning routine.
The Power of Multiple Solutions
Before we delve into the specific benefits, let’s consider why solving a problem in multiple ways is so powerful:
- Deepens understanding: Each new solution approach forces you to look at the problem from a different angle, enhancing your comprehension of the underlying concepts.
- Improves problem-solving skills: By exploring various solutions, you develop a more versatile toolkit for tackling new challenges.
- Enhances creativity: Thinking of alternative solutions encourages creative thinking and helps you break out of fixed mindsets.
- Prepares for interviews: Technical interviews often require you to optimize solutions or approach problems from different perspectives.
- Builds confidence: Mastering multiple solutions to a problem boosts your confidence in your problem-solving abilities.
Now, let’s explore these benefits in more detail and see how they can significantly improve your coding skills.
1. Deepening Your Understanding
When you solve a problem in one way, you might understand that specific solution, but you may not fully grasp the underlying principles or the problem’s nuances. By approaching the same problem from different angles, you force yourself to understand it at a deeper level.
Consider the classic problem of finding the nth Fibonacci number. Here are three different approaches:
Recursive Solution
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
Iterative Solution
def fibonacci_iterative(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n+1):
a, b = b, a + b
return b
Dynamic Programming Solution
def fibonacci_dp(n):
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
By implementing all three solutions, you gain insights into recursion, iteration, and dynamic programming. You learn about trade-offs between time and space complexity, and you develop a more nuanced understanding of the Fibonacci sequence itself.
2. Improving Problem-Solving Skills
Every time you approach a problem from a new angle, you’re exercising your problem-solving muscles. This practice helps you develop a more diverse set of strategies for tackling coding challenges.
Let’s look at the problem of reversing a string. Here are multiple approaches:
Using Built-in Functions
def reverse_string_builtin(s):
return s[::-1]
Using a Loop
def reverse_string_loop(s):
return ''.join(s[i] for i in range(len(s)-1, -1, -1))
Using Recursion
def reverse_string_recursive(s):
if len(s) <= 1:
return s
return reverse_string_recursive(s[1:]) + s[0]
Using Two Pointers
def reverse_string_two_pointers(s):
s = list(s)
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
By implementing these different solutions, you’re not just learning how to reverse a string; you’re exploring various problem-solving techniques that can be applied to a wide range of coding challenges.
3. Enhancing Creativity
Creativity in programming isn’t just about coming up with new ideas; it’s also about finding novel ways to solve existing problems. When you push yourself to find multiple solutions, you’re training your brain to think creatively and break out of conventional patterns.
Let’s consider the problem of finding the maximum subarray sum. Here are different creative approaches:
Brute Force Approach
def max_subarray_brute_force(nums):
max_sum = float('-inf')
for i in range(len(nums)):
current_sum = 0
for j in range(i, len(nums)):
current_sum += nums[j]
max_sum = max(max_sum, current_sum)
return max_sum
Kadane’s Algorithm
def max_subarray_kadane(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Divide and Conquer Approach
def max_subarray_divide_conquer(nums):
def max_crossing_sum(nums, low, mid, high):
left_sum = float('-inf')
sum = 0
for i in range(mid, low - 1, -1):
sum += nums[i]
left_sum = max(left_sum, sum)
right_sum = float('-inf')
sum = 0
for i in range(mid + 1, high + 1):
sum += nums[i]
right_sum = max(right_sum, sum)
return left_sum + right_sum
def max_subarray_sum(nums, low, high):
if low == high:
return nums[low]
mid = (low + high) // 2
return max(max_subarray_sum(nums, low, mid),
max_subarray_sum(nums, mid + 1, high),
max_crossing_sum(nums, low, mid, high))
return max_subarray_sum(nums, 0, len(nums) - 1)
Each of these solutions represents a different creative approach to the same problem. By exploring these various methods, you’re training your mind to think outside the box and approach problems from multiple angles.
4. Preparing for Technical Interviews
In technical interviews, especially at top tech companies like FAANG, it’s not enough to solve a problem; you need to be able to optimize your solution and discuss alternatives. By practicing multiple solutions to the same problem, you’re preparing yourself for these high-pressure situations.
Let’s look at a common interview problem: finding all pairs in an array that sum to a target value. We’ll explore multiple solutions with different time and space complexities:
Brute Force Approach (O(n^2) time, O(1) space)
def find_pairs_brute_force(nums, target):
pairs = []
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
pairs.append((nums[i], nums[j]))
return pairs
Using a Hash Table (O(n) time, O(n) space)
def find_pairs_hash(nums, target):
pairs = []
seen = set()
for num in nums:
complement = target - num
if complement in seen:
pairs.append((complement, num))
seen.add(num)
return pairs
Two-Pointer Approach (O(n log n) time, O(1) space if we can modify the input)
def find_pairs_two_pointers(nums, target):
nums.sort()
pairs = []
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
pairs.append((nums[left], nums[right]))
left += 1
right -= 1
elif current_sum < target:
left += 1
else:
right -= 1
return pairs
By knowing multiple solutions, you can discuss trade-offs between time and space complexity, demonstrating a deeper understanding of algorithmic efficiency to your interviewer.
5. Building Confidence
As you become proficient in solving problems in multiple ways, you’ll notice a significant boost in your confidence. This confidence is not just about feeling good; it translates into tangible benefits in your coding journey:
- Tackling new challenges: When you encounter a new problem, you’ll have a diverse set of approaches to try.
- Code reviews: You’ll be better equipped to suggest alternative solutions during code reviews, adding value to your team.
- Learning new technologies: Your improved problem-solving skills will help you adapt more quickly to new programming languages and frameworks.
- Technical discussions: You’ll be able to engage in deeper technical discussions with colleagues and mentors, further accelerating your learning.
Strategies for Practicing Multiple Solutions
Now that we understand the benefits, let’s look at some strategies for incorporating this practice into your coding routine:
- Start with classic problems: Begin with well-known problems like FizzBuzz, palindrome checking, or binary search. These problems often have multiple well-documented solutions.
- Use coding platforms: Websites like LeetCode, HackerRank, and AlgoCademy often show multiple solutions after you solve a problem. Study these alternatives.
- Join coding communities: Participate in forums or local coding meetups where you can discuss different approaches to problems with other developers.
- Review and refactor old code: Revisit problems you’ve solved in the past and try to implement them in a different way.
- Challenge yourself: After solving a problem, challenge yourself to find a solution with better time complexity, less space usage, or using a different programming paradigm.
- Study algorithms and data structures: A strong foundation in these areas will give you more tools to approach problems in diverse ways.
Conclusion
Solving the same problem in multiple ways is not just an academic exercise; it’s a powerful technique for improving your coding skills, preparing for technical interviews, and becoming a more well-rounded developer. By deepening your understanding, improving your problem-solving skills, enhancing your creativity, preparing for interviews, and building your confidence, this practice can significantly accelerate your growth as a programmer.
Remember, the goal is not just to accumulate solutions, but to understand the thought process behind each approach. As you practice this technique, you’ll find yourself becoming more adaptable, creative, and confident in your coding abilities. Whether you’re a beginner looking to solidify your fundamentals or an experienced developer preparing for a FAANG interview, embracing the power of multiple solutions will serve you well in your programming journey.
So, the next time you solve a coding problem, don’t stop at the first solution. Challenge yourself to find another way, and then another. Your future self will thank you for the diverse problem-solving toolkit you’re building today.