How to Improve Your Problem Solving Through Daily Practice
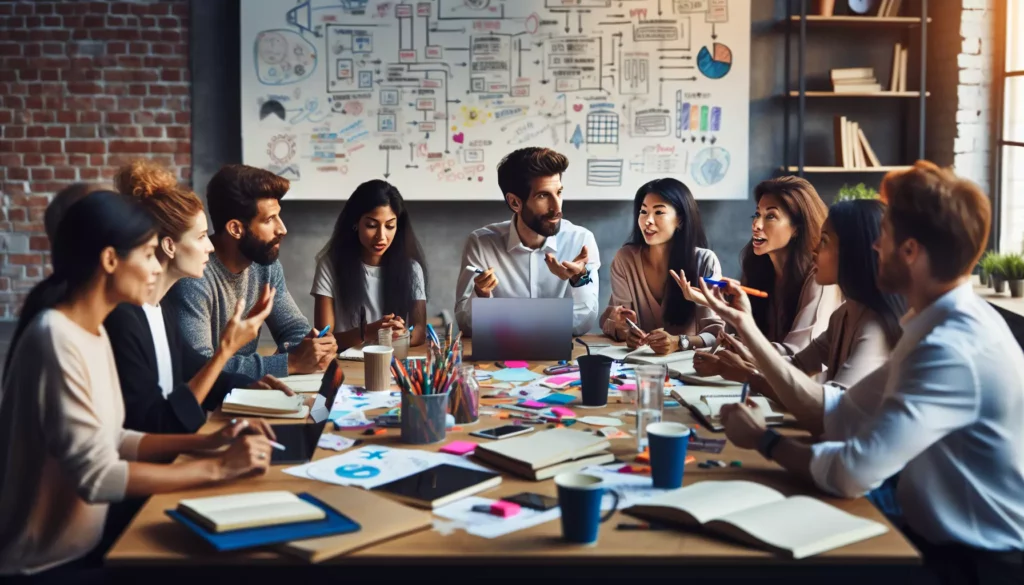
In the ever-evolving world of technology and programming, problem-solving skills are paramount. Whether you’re a beginner coder or an experienced developer aiming for a position at a major tech company, honing your problem-solving abilities is crucial. This article will explore effective strategies to enhance your problem-solving skills through daily practice, with a focus on coding and algorithmic thinking.
Understanding the Importance of Problem-Solving Skills
Before diving into the practical aspects of improving problem-solving skills, it’s essential to understand why these skills are so valuable, especially in the context of coding and tech careers:
- Career Advancement: Strong problem-solving skills are highly sought after by top tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
- Efficient Coding: Better problem-solving leads to more efficient and elegant code solutions.
- Adaptability: As technology evolves, the ability to solve new and complex problems becomes increasingly important.
- Innovation: Problem-solving skills fuel creativity and innovation in software development.
Daily Practices to Enhance Problem-Solving Skills
1. Solve Coding Challenges Regularly
One of the most effective ways to improve your problem-solving skills is to tackle coding challenges on a daily basis. Platforms like AlgoCademy offer a wide range of problems suitable for various skill levels.
- Start with easier problems and gradually increase difficulty.
- Aim to solve at least one problem every day.
- Focus on understanding the problem before jumping into coding.
- Try to solve problems within a time limit to improve efficiency.
2. Learn and Apply Different Problem-Solving Techniques
Familiarize yourself with various problem-solving techniques and apply them to different scenarios:
- Divide and Conquer: Break down complex problems into smaller, manageable parts.
- Dynamic Programming: Solve problems by breaking them down into simpler subproblems.
- Greedy Algorithms: Make locally optimal choices at each step to find a global optimum.
- Backtracking: Build solutions incrementally and abandon those that fail to satisfy constraints.
3. Analyze and Optimize Your Solutions
After solving a problem, take time to analyze and optimize your solution:
- Review your code for potential improvements in efficiency or readability.
- Consider alternative approaches to the same problem.
- Analyze the time and space complexity of your solution.
- Compare your solution with others to learn different perspectives.
4. Implement Data Structures from Scratch
Understanding and implementing fundamental data structures is crucial for problem-solving in programming:
- Implement common data structures like arrays, linked lists, stacks, and queues.
- Practice more advanced structures such as trees, graphs, and hash tables.
- Understand the pros and cons of each data structure and when to use them.
5. Study Algorithms and Their Applications
Deepen your understanding of algorithms and their real-world applications:
- Study classic algorithms like sorting, searching, and graph traversal.
- Understand the theory behind algorithms and their time/space complexities.
- Practice implementing algorithms from scratch.
- Explore how these algorithms are used in real-world applications.
6. Engage in Peer Programming and Code Reviews
Collaborating with others can significantly enhance your problem-solving skills:
- Participate in pair programming sessions to learn different approaches.
- Engage in code reviews to gain insights into other coding styles and solutions.
- Join coding communities or study groups to discuss problems and solutions.
7. Practice Explaining Your Solutions
Being able to articulate your problem-solving process is as important as finding the solution itself:
- Practice explaining your solutions out loud or in writing.
- Focus on clearly communicating your thought process and reasoning.
- Prepare for technical interviews by simulating explanation scenarios.
Leveraging Tools and Resources for Daily Practice
1. Online Coding Platforms
Utilize online platforms designed for coding practice and skill development:
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance.
- LeetCode: Provides a vast array of coding challenges and interview preparation resources.
- HackerRank: Offers coding challenges and skill certifications.
2. Programming Books and Online Courses
Supplement your practice with structured learning materials:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Online courses on platforms like Coursera, edX, or Udacity focusing on algorithms and data structures
3. Version Control Systems
Use version control systems to track your progress and manage your code:
- Git and GitHub for storing and sharing your solutions
- Create repositories for different types of problems or algorithms
- Use commit messages to document your thought process and improvements
Creating a Daily Problem-Solving Routine
To make the most of your daily practice, establish a consistent routine:
1. Set Specific Goals
- Define clear, achievable daily or weekly goals (e.g., solve two medium-difficulty problems per day).
- Track your progress and adjust goals as you improve.
2. Allocate Dedicated Time
- Set aside a specific time each day for problem-solving practice.
- Start with shorter sessions (30 minutes) and gradually increase as you build the habit.
3. Mix Problem Types
- Vary the types of problems you tackle to cover different concepts and difficulty levels.
- Revisit previously solved problems periodically to reinforce learning.
4. Reflect and Review
- Keep a journal or log of the problems you’ve solved and the concepts you’ve learned.
- Regularly review your progress and identify areas for improvement.
Overcoming Common Challenges in Daily Practice
As you embark on your journey of daily problem-solving practice, you may encounter some challenges. Here are strategies to overcome them:
1. Dealing with Frustration
It’s normal to feel frustrated when faced with difficult problems. To manage this:
- Take short breaks when you feel stuck.
- Practice mindfulness or meditation to maintain focus.
- Remember that struggle is part of the learning process.
2. Maintaining Motivation
Keeping up with daily practice can be challenging. To stay motivated:
- Celebrate small victories and progress.
- Join coding challenges or competitions for added excitement.
- Connect with a community of learners for mutual support and encouragement.
3. Balancing Breadth and Depth
It’s important to strike a balance between exploring various topics and diving deep into specific areas:
- Alternate between broad exploration and focused study of particular topics.
- Create a learning roadmap to ensure you cover essential concepts over time.
- Periodically reassess your goals and adjust your focus as needed.
Advanced Techniques for Problem-Solving Mastery
As you progress in your problem-solving journey, consider incorporating these advanced techniques:
1. Time-Boxed Problem Solving
Simulate interview conditions by setting strict time limits for solving problems:
- Start with longer time frames and gradually reduce them as you improve.
- Practice quickly identifying the problem type and selecting appropriate strategies.
- Learn to manage your time effectively, balancing between understanding the problem, designing a solution, and implementing it.
2. Mock Interviews
Engage in mock technical interviews to practice problem-solving under pressure:
- Use platforms like Pramp or InterviewBit for peer mock interviews.
- Practice explaining your thought process clearly while coding.
- Learn to handle hints and feedback constructively during the problem-solving process.
3. System Design Problems
Expand your problem-solving skills to include system design challenges:
- Study scalable system architectures and design patterns.
- Practice designing high-level systems for common applications (e.g., a social media platform, a ride-sharing service).
- Learn to balance trade-offs in system design decisions.
4. Competitive Programming
Participate in competitive programming contests to challenge yourself:
- Join platforms like Codeforces or TopCoder for regular contests.
- Learn advanced algorithms and optimization techniques.
- Improve your ability to solve problems quickly and efficiently.
Integrating Problem-Solving Skills into Real-World Projects
To truly master problem-solving, apply your skills to real-world scenarios:
1. Contribute to Open Source Projects
- Find open-source projects that interest you on platforms like GitHub.
- Start with small contributions and gradually take on more complex issues.
- Learn to navigate and understand large codebases.
2. Build Personal Projects
- Develop your own applications or tools to solve real-world problems.
- Challenge yourself to implement efficient algorithms and data structures in your projects.
- Document your problem-solving process throughout the development.
3. Participate in Hackathons
- Join hackathons to solve problems under time constraints and in team settings.
- Practice rapid prototyping and iterative problem-solving.
- Learn to balance feature implementation with code quality and efficiency.
Measuring Your Progress
To ensure you’re making steady improvements, it’s crucial to measure your progress:
1. Track Problem-Solving Metrics
- Record the number and types of problems solved.
- Monitor improvements in solving time for similar problem types.
- Keep track of the concepts and algorithms you’ve mastered.
2. Periodic Self-Assessment
- Regularly attempt problems you found challenging in the past to gauge improvement.
- Take online coding assessments or participate in coding competitions to benchmark your skills.
- Reflect on your problem-solving process and identify areas for further improvement.
3. Seek Feedback
- Share your solutions with peers or mentors for constructive feedback.
- Participate in code reviews to gain insights into your problem-solving approach.
- Use platforms that provide automated feedback on code efficiency and style.
Conclusion
Improving your problem-solving skills through daily practice is a journey that requires dedication, consistency, and a strategic approach. By incorporating the techniques and strategies outlined in this article, you can significantly enhance your ability to tackle complex coding challenges and prepare yourself for success in technical interviews and your programming career.
Remember that the key to mastering problem-solving lies in regular practice, continuous learning, and applying your skills to diverse scenarios. Embrace the challenges, learn from your mistakes, and celebrate your progress along the way. With persistence and the right approach, you’ll develop the problem-solving prowess that sets apart exceptional programmers in the competitive tech industry.
As you continue your journey, leverage resources like AlgoCademy to access interactive tutorials, AI-powered assistance, and a wealth of coding challenges. Stay curious, keep practicing, and watch as your problem-solving skills transform, opening doors to exciting opportunities in the world of technology and beyond.