How to Get Past Plateaus in Algorithm Mastery
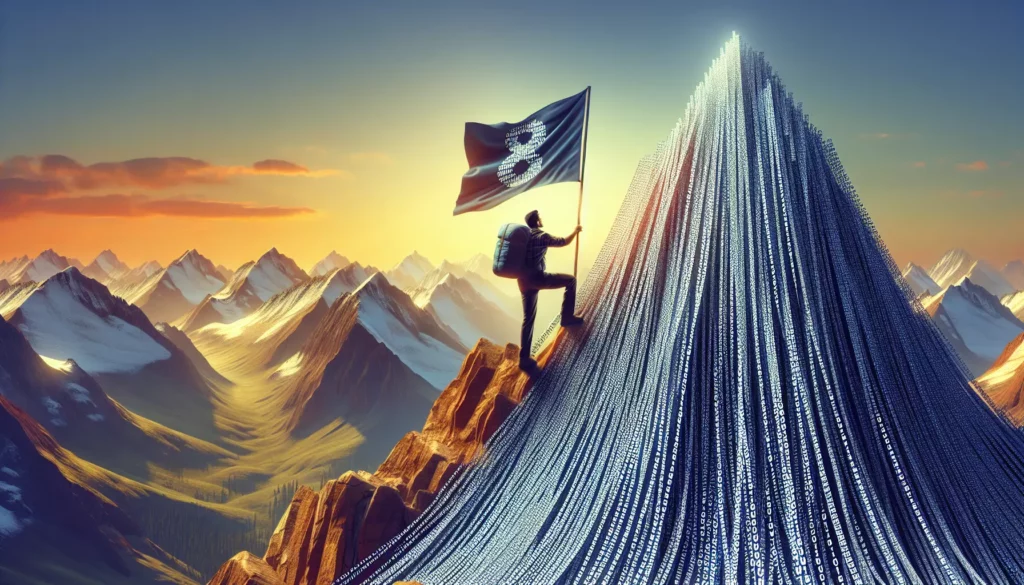
As you embark on your journey to master algorithms, you’ll likely encounter moments where progress seems to slow down or come to a halt. These plateaus are a natural part of the learning process, but they can be frustrating and demotivating. In this comprehensive guide, we’ll explore effective strategies to overcome these plateaus and continue your growth in algorithm mastery.
Understanding Plateaus in Algorithm Learning
Before diving into solutions, it’s essential to understand what causes plateaus in algorithm learning:
- Cognitive overload: As you tackle increasingly complex algorithms, your brain may struggle to process and retain new information.
- Comfort zone: Sticking to familiar problem types and avoiding challenging concepts can lead to stagnation.
- Lack of structured learning: Without a clear learning path, you may miss crucial concepts or fail to build upon foundational knowledge.
- Insufficient practice: Algorithms require consistent practice to internalize concepts and improve problem-solving skills.
- Burnout: Intense study sessions without proper breaks can lead to mental fatigue and decreased productivity.
Now that we’ve identified common causes of plateaus, let’s explore strategies to overcome them and continue your progress in algorithm mastery.
1. Diversify Your Learning Approach
One of the most effective ways to break through a plateau is to diversify your learning approach. This can help stimulate different areas of your brain and prevent boredom or monotony in your studies.
Try Different Learning Resources
Don’t limit yourself to a single learning resource. Explore various platforms and materials to gain different perspectives on algorithmic concepts:
- Online courses: Platforms like Coursera, edX, and Udacity offer structured algorithm courses from top universities.
- Textbooks: Classic algorithm textbooks like “Introduction to Algorithms” by Cormen et al. provide in-depth explanations and analysis.
- Video tutorials: YouTube channels and online coding platforms often offer visual explanations of complex algorithms.
- Coding websites: Platforms like LeetCode, HackerRank, and CodeForces offer a wide range of algorithm problems and challenges.
- Interactive learning tools: Websites like VisuAlgo provide interactive visualizations of various algorithms and data structures.
Implement Algorithms in Different Programming Languages
If you’re comfortable with one programming language, try implementing algorithms in a different language. This can help you:
- Gain a deeper understanding of the algorithm’s core concepts
- Learn language-specific optimizations and best practices
- Improve your overall programming skills
- Discover new ways to approach problem-solving
For example, if you’re proficient in Python, try implementing the same algorithm in Java or C++. This exercise can reveal nuances in language features and performance characteristics.
2. Focus on Fundamentals and Build a Strong Foundation
Sometimes, plateaus occur because of gaps in foundational knowledge. Revisiting and strengthening your understanding of fundamental concepts can help you overcome these obstacles.
Review Basic Data Structures
Ensure you have a solid grasp of essential data structures, as they form the building blocks of more complex algorithms:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
For each data structure, focus on:
- Time and space complexity of common operations
- Implementation details and trade-offs
- Real-world applications and use cases
Master Algorithm Design Techniques
Familiarize yourself with common algorithm design paradigms and techniques:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Graph Algorithms
- Two Pointers
- Sliding Window
Understanding these techniques will help you recognize patterns in problem-solving and apply appropriate strategies to new challenges.
3. Practice Deliberate and Focused Problem-Solving
Quality practice is crucial for overcoming plateaus in algorithm mastery. Instead of solving random problems, focus on deliberate and targeted practice.
Implement the “Five Problem” Approach
Adopt a structured approach to problem-solving by following this method:
- Solve an easy problem to build confidence and warm up your problem-solving skills.
- Tackle a medium difficulty problem that you’re familiar with to reinforce your knowledge.
- Attempt a medium difficulty problem that’s new to you, pushing your boundaries.
- Try a hard problem that challenges you and requires creative thinking.
- Research and study a complex algorithm or data structure you haven’t encountered before.
This approach ensures a balanced practice session that covers various difficulty levels and exposes you to new concepts.
Time-Box Your Problem-Solving Sessions
Set time limits for each problem to simulate real interview conditions and improve your efficiency:
- Easy problems: 15-20 minutes
- Medium problems: 30-45 minutes
- Hard problems: 45-60 minutes
If you can’t solve a problem within the allotted time, review the solution, understand the approach, and try to implement it yourself without referring to the original solution.
Analyze and Learn from Your Mistakes
After solving a problem or reviewing a solution, take time to reflect on your approach:
- Identify areas where you struggled or made mistakes
- Analyze more efficient solutions and understand why they work better
- Document key insights and lessons learned for future reference
- Revisit challenging problems after a few days to reinforce your understanding
4. Leverage Spaced Repetition and Active Recall
To overcome plateaus and retain knowledge more effectively, incorporate spaced repetition and active recall techniques into your learning process.
Implement a Spaced Repetition System
Spaced repetition involves reviewing information at increasing intervals to improve long-term retention. You can use tools like Anki or create your own system to review algorithms and concepts:
- Create flashcards for key algorithm concepts, time complexities, and implementation details
- Review new cards daily and gradually increase the interval for cards you consistently remember
- Prioritize reviewing difficult concepts more frequently
Practice Active Recall
Instead of passively reading solutions or watching video explanations, actively engage with the material:
- Attempt to solve problems from memory before checking the solution
- Explain algorithm concepts out loud or write them down without referring to notes
- Teach algorithms to others or participate in study groups to reinforce your understanding
5. Collaborate and Seek Feedback
Learning algorithms in isolation can lead to plateaus. Engaging with others can provide new perspectives and motivation to overcome challenges.
Participate in Coding Communities
Join online forums, Discord servers, or local meetups focused on algorithms and competitive programming:
- Share your solutions and ask for feedback
- Discuss different approaches to solving problems
- Participate in coding contests or virtual hackathons
- Collaborate on open-source projects that involve algorithm implementation
Find a Study Partner or Mentor
Having a dedicated study partner or mentor can provide accountability and support:
- Schedule regular problem-solving sessions together
- Conduct mock interviews to practice explaining your thought process
- Share resources and learning strategies
- Seek guidance on areas where you’re struggling
6. Apply Algorithms to Real-World Projects
To solidify your understanding and maintain motivation, apply algorithmic concepts to practical projects or real-world scenarios.
Develop Personal Projects
Create projects that incorporate algorithms you’ve learned:
- Build a pathfinding visualizer using graph algorithms
- Implement a simple search engine using indexing and ranking algorithms
- Create a recommendation system using collaborative filtering algorithms
- Develop a data compression tool using various compression algorithms
Contribute to Open Source Projects
Find open-source projects that involve algorithm implementation or optimization:
- Look for issues labeled “good first issue” or “help wanted” on GitHub
- Contribute to algorithm libraries or educational resources
- Optimize existing implementations for better performance
- Add new features or algorithms to existing projects
7. Embrace the Power of Visualization
Visual representations can significantly enhance your understanding of complex algorithms and data structures.
Use Algorithm Visualization Tools
Leverage online tools and resources to visualize algorithm execution:
- VisuAlgo: Offers interactive visualizations for various algorithms and data structures
- Algorithm Visualizer: Allows you to visualize code execution step-by-step
- Sorting.at: Provides animations of different sorting algorithms
Create Your Own Visualizations
Develop your own visualizations to deepen your understanding:
- Use drawing tools or whiteboarding apps to sketch out algorithm steps
- Implement simple graphical representations using libraries like Pygame or Processing
- Create animated GIFs or videos to explain complex algorithms
8. Develop a Growth Mindset
Overcoming plateaus requires persistence and a positive attitude towards learning and challenges.
Embrace Challenges as Opportunities
Reframe difficult problems or concepts as opportunities for growth:
- Celebrate small victories and incremental progress
- View mistakes as valuable learning experiences
- Set realistic goals and track your improvement over time
Practice Self-Reflection and Mindfulness
Regularly assess your learning process and mental state:
- Keep a learning journal to track your progress and insights
- Practice mindfulness techniques to reduce stress and improve focus
- Take breaks and engage in activities that recharge your mental energy
9. Optimize Your Learning Environment
Creating an optimal learning environment can significantly impact your ability to overcome plateaus and maintain progress.
Set Up a Dedicated Study Space
Designate a specific area for algorithm practice and study:
- Ensure good lighting and comfortable seating
- Minimize distractions by using noise-canceling headphones or white noise
- Keep necessary resources (textbooks, notes, water) within reach
Use Productivity Techniques
Implement proven productivity methods to maintain focus and motivation:
- Pomodoro Technique: Work in focused 25-minute intervals with short breaks
- Time-blocking: Schedule specific time slots for different learning activities
- Eisenhower Matrix: Prioritize tasks based on importance and urgency
10. Continuously Assess and Adjust Your Learning Strategy
Regularly evaluate your progress and be willing to adjust your approach as needed.
Conduct Regular Self-Assessments
Periodically assess your skills and knowledge:
- Take practice tests or participate in coding competitions
- Review your performance in mock interviews
- Evaluate your ability to solve problems of varying difficulty levels
Adapt Your Learning Plan
Based on your assessments, adjust your learning strategy:
- Identify areas that need more focus or practice
- Experiment with different learning techniques and resources
- Set new goals and challenges to maintain motivation
Conclusion
Overcoming plateaus in algorithm mastery requires a multifaceted approach that combines diverse learning strategies, deliberate practice, collaboration, and a growth mindset. By implementing the techniques discussed in this guide, you can break through barriers, maintain steady progress, and continue your journey towards algorithm expertise.
Remember that plateaus are a natural part of the learning process and often indicate that you’re on the cusp of a significant breakthrough. Embrace the challenges, stay persistent, and trust in your ability to grow and improve. With consistent effort and the right strategies, you’ll be well-equipped to tackle even the most complex algorithmic problems and excel in technical interviews.
Keep pushing forward, and don’t hesitate to revisit these strategies whenever you feel stuck. Your algorithm mastery journey is a marathon, not a sprint, and each obstacle overcome is a step towards becoming a more skilled and confident programmer.