The Art of Writing Efficient Algorithms: A Comprehensive Guide
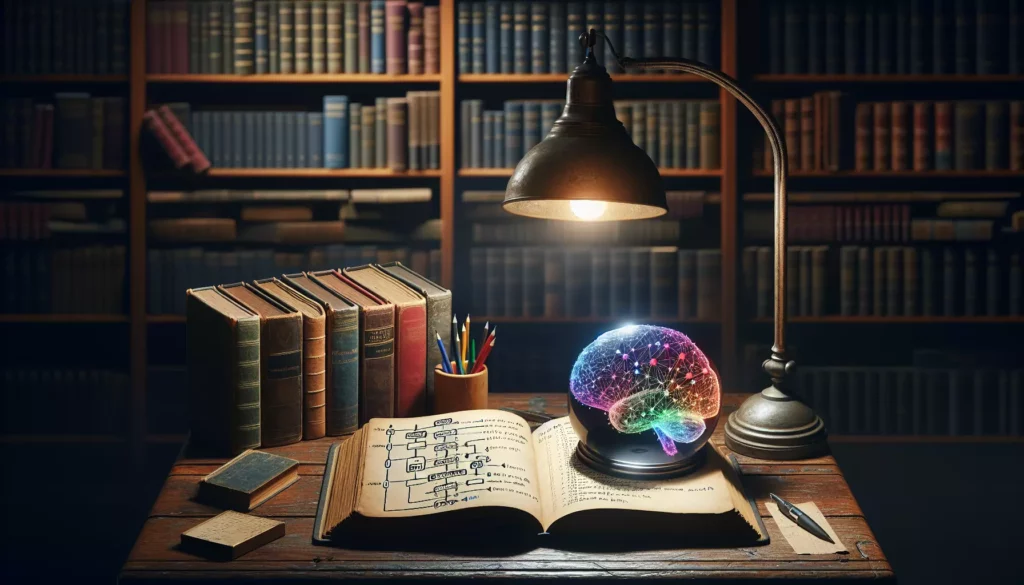
In the world of computer science and software development, the ability to write efficient algorithms is a highly sought-after skill. Whether you’re a beginner programmer or an experienced developer preparing for technical interviews at major tech companies, understanding the principles of algorithm design and optimization is crucial. This comprehensive guide will explore the art of writing efficient algorithms, covering key concepts, techniques, and best practices that will help you elevate your coding skills to the next level.
Table of Contents
- Understanding Algorithms
- The Importance of Efficiency
- Time Complexity Analysis
- Space Complexity Analysis
- Common Algorithmic Techniques
- Optimization Strategies
- Choosing the Right Data Structures
- Coding Best Practices
- Testing and Debugging
- Real-World Examples
- Preparing for Technical Interviews
- Conclusion
1. Understanding Algorithms
Before diving into the intricacies of writing efficient algorithms, it’s essential to have a solid understanding of what algorithms are and why they matter. An algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In the context of computer science, algorithms are the foundation of all software applications, from simple calculators to complex artificial intelligence systems.
Algorithms can be expressed in various ways, including:
- Natural language
- Pseudocode
- Flowcharts
- Programming languages
The key characteristics of a well-designed algorithm include:
- Correctness: The algorithm should solve the problem it was designed for
- Efficiency: It should use computational resources (time and space) effectively
- Simplicity: The algorithm should be easy to understand and implement
- Generality: It should be applicable to a wide range of inputs
2. The Importance of Efficiency
Efficiency is a critical aspect of algorithm design. An efficient algorithm can make the difference between a program that runs in milliseconds and one that takes hours or even days to complete. As datasets grow larger and computational tasks become more complex, the need for efficient algorithms becomes increasingly important.
There are two main aspects of efficiency to consider:
- Time efficiency: How quickly the algorithm performs its task
- Space efficiency: How much memory the algorithm requires
Balancing these two factors is often a key challenge in algorithm design. In some cases, you may need to trade off space efficiency for time efficiency, or vice versa, depending on the specific requirements of your problem.
3. Time Complexity Analysis
Time complexity is a measure of how the running time of an algorithm grows as the size of the input increases. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of the algorithm’s running time.
Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
To analyze the time complexity of an algorithm, you need to:
- Identify the basic operations in the algorithm
- Count how many times each operation is executed
- Express the count in terms of the input size
- Keep only the highest-order term and drop the coefficients
Let’s look at a simple example of time complexity analysis:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
In this algorithm, we iterate through the array once, performing a constant-time comparison for each element. The time complexity is O(n), where n is the length of the input array.
4. Space Complexity Analysis
Space complexity refers to the amount of memory an algorithm uses relative to the size of the input. Like time complexity, it’s often expressed using Big O notation.
When analyzing space complexity, consider:
- The space required by the input
- The auxiliary space used by the algorithm (e.g., additional data structures)
- The space used by the call stack in recursive algorithms
For example, consider this recursive implementation of the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
While this algorithm has a time complexity of O(2^n), its space complexity is O(n) due to the recursive call stack.
5. Common Algorithmic Techniques
Several algorithmic techniques can help you design efficient solutions to a wide range of problems. Some of the most common techniques include:
Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them independently, and then combining the results. Examples include:
- Merge Sort
- Quick Sort
- Binary Search
Dynamic Programming
Dynamic programming is used to solve problems with overlapping subproblems and optimal substructure. It involves breaking down a problem into simpler subproblems and storing their solutions to avoid redundant computations. Examples include:
- Fibonacci sequence (memoized version)
- Longest Common Subsequence
- Knapsack problem
Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. They are often used for optimization problems. Examples include:
- Huffman coding
- Dijkstra’s shortest path algorithm
- Kruskal’s minimum spanning tree algorithm
Backtracking
Backtracking is used to solve problems where you need to find all (or some) solutions to a computational problem, incrementally building candidates and abandoning those that fail to satisfy the constraints. Examples include:
- N-Queens problem
- Sudoku solver
- Generating all permutations of a set
6. Optimization Strategies
Once you have a working algorithm, there are several strategies you can employ to optimize its performance:
Memoization
Memoization involves storing the results of expensive function calls and returning the cached result when the same inputs occur again. This can significantly improve the performance of recursive algorithms with overlapping subproblems.
Here’s an example of memoization applied to the Fibonacci sequence:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
Caching
Caching is similar to memoization but is typically used for storing the results of expensive computations or I/O operations. It can be particularly useful in web applications and distributed systems.
Lazy Evaluation
Lazy evaluation is a strategy where you delay the evaluation of an expression until its value is needed. This can help avoid unnecessary computations and improve performance, especially when dealing with large datasets or infinite sequences.
Parallelization
For algorithms that can be broken down into independent subtasks, parallelization can significantly improve performance by utilizing multiple cores or processors. This is particularly useful for computationally intensive tasks or when processing large datasets.
7. Choosing the Right Data Structures
Selecting appropriate data structures is crucial for writing efficient algorithms. Different data structures have different strengths and weaknesses, and choosing the right one can significantly impact your algorithm’s performance.
Here are some common data structures and their typical use cases:
- Arrays: Fast access by index, contiguous memory allocation
- Linked Lists: Efficient insertions and deletions
- Hash Tables: Fast lookups, insertions, and deletions
- Trees: Hierarchical data representation, efficient searching and sorting
- Heaps: Priority queues, efficient minimum/maximum value retrieval
- Graphs: Representing relationships between entities
- Stacks: Last-in, first-out (LIFO) operations
- Queues: First-in, first-out (FIFO) operations
When choosing a data structure, consider factors such as:
- The types of operations you need to perform (e.g., insertions, deletions, searches)
- The expected size of your data
- The frequency of different operations
- Memory constraints
- The need for ordered or unordered data
8. Coding Best Practices
Writing efficient algorithms isn’t just about choosing the right approach and data structures; it’s also about writing clean, maintainable code. Here are some best practices to keep in mind:
Use Descriptive Variable Names
Choose clear and meaningful names for variables, functions, and classes. This makes your code more readable and easier to understand.
Comment Your Code
While your code should be as self-explanatory as possible, comments can provide valuable context and explain complex logic. Use comments judiciously to clarify your intentions and any non-obvious implementation details.
Follow the DRY Principle
DRY stands for “Don’t Repeat Yourself.” Avoid duplicating code by extracting common functionality into reusable functions or classes.
Use Consistent Formatting
Adopt a consistent coding style throughout your project. This includes consistent indentation, spacing, and naming conventions.
Write Modular Code
Break your code into smaller, focused functions or modules. This improves readability, testability, and reusability.
Optimize for Readability
While performance is important, don’t sacrifice readability for minor optimizations. Clear, understandable code is often more valuable than slightly faster but obscure code.
9. Testing and Debugging
Thorough testing and effective debugging are essential parts of writing efficient algorithms. Here are some strategies to ensure your algorithms are correct and perform as expected:
Unit Testing
Write unit tests for individual functions or components of your algorithm. This helps catch bugs early and ensures that changes don’t introduce regressions.
Edge Case Testing
Test your algorithm with edge cases, such as empty inputs, very large inputs, or inputs with special characteristics. These cases often reveal hidden bugs or performance issues.
Performance Testing
Measure the performance of your algorithm with different input sizes to verify that it behaves as expected in terms of time and space complexity.
Use Debugging Tools
Familiarize yourself with debugging tools provided by your development environment. These can help you step through your code, inspect variables, and identify the root causes of issues.
Logging
Implement logging in your code to track the flow of execution and intermediate results. This can be invaluable when debugging complex algorithms or diagnosing issues in production environments.
10. Real-World Examples
To illustrate the principles we’ve discussed, let’s look at a few real-world examples of algorithm optimization:
Optimizing Database Queries
Consider a web application that needs to display a list of the top 100 most active users. A naive approach might be:
SELECT username, activity_count
FROM users
ORDER BY activity_count DESC
LIMIT 100;
This query could become slow as the number of users grows. An optimized approach might involve:
- Creating an index on the activity_count column
- Caching the results and updating them periodically
- Using a materialized view to pre-compute the result
Improving Search Functionality
For a large-scale search engine, efficient algorithms are crucial. Some optimization techniques might include:
- Using inverted indices for fast keyword lookups
- Implementing efficient string matching algorithms like Boyer-Moore or Knuth-Morris-Pratt
- Utilizing distributed computing to parallelize search operations
Optimizing Image Processing
In image processing applications, performance is often critical. Optimization strategies might include:
- Using vectorized operations to leverage CPU SIMD instructions
- Implementing algorithms on GPUs for parallel processing
- Applying techniques like integral images for fast feature computation
11. Preparing for Technical Interviews
Many technical interviews, especially at major tech companies, focus heavily on algorithmic problem-solving. Here are some tips to help you prepare:
Practice Regularly
Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Aim to solve a few problems each day to build your skills and confidence.
Study Core Algorithms and Data Structures
Make sure you have a solid understanding of fundamental algorithms (e.g., sorting, searching) and data structures (e.g., arrays, linked lists, trees, graphs).
Analyze Time and Space Complexity
For each problem you solve, practice analyzing its time and space complexity. Be prepared to discuss trade-offs between different approaches.
Mock Interviews
Participate in mock interviews with friends or use platforms that offer this service. This helps you get comfortable with explaining your thought process and coding under pressure.
Review Company-Specific Information
Research the types of questions typically asked by the companies you’re interviewing with. Some companies may focus more on certain types of problems or have specific coding style preferences.
Soft Skills Matter
Don’t forget about soft skills. Practice explaining your thought process clearly, asking clarifying questions, and collaborating with your interviewer.
12. Conclusion
Writing efficient algorithms is both an art and a science. It requires a deep understanding of computer science principles, creative problem-solving skills, and attention to detail. By mastering the concepts and techniques outlined in this guide, you’ll be well-equipped to design and implement algorithms that are not only correct but also performant and scalable.
Remember that becoming proficient in algorithm design is an ongoing journey. Continue to challenge yourself with new problems, stay updated with the latest developments in computer science, and never stop learning. Whether you’re preparing for technical interviews or working on complex software projects, the skills you develop in writing efficient algorithms will serve you well throughout your career in software development.
As you continue to hone your skills, don’t forget to leverage resources like AlgoCademy, which offers interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you progress from beginner-level coding to advanced algorithmic thinking. With dedication and practice, you’ll be well on your way to mastering the art of writing efficient algorithms.