Understanding Code Optimization: Thinking Smarter, Not Harder
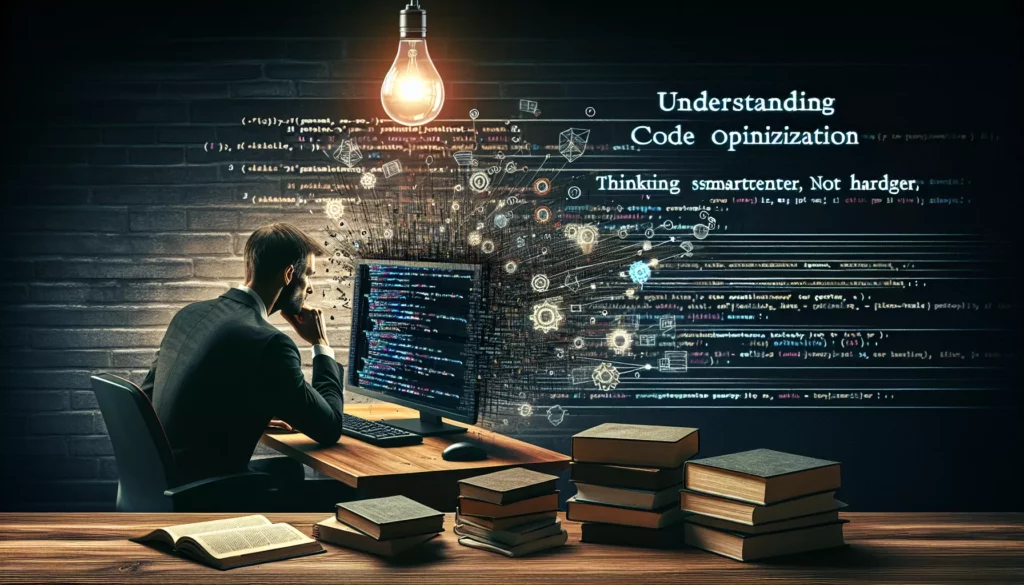
In the world of programming, writing code that works is just the beginning. As you progress in your coding journey, you’ll quickly realize that there’s a significant difference between code that simply functions and code that performs efficiently. This is where code optimization comes into play. In this comprehensive guide, we’ll dive deep into the art and science of code optimization, exploring techniques and strategies that will help you write smarter, more efficient code.
What is Code Optimization?
Code optimization is the process of improving the efficiency of your code without changing its functionality. It’s about making your programs run faster, use less memory, or consume fewer resources. The goal is to achieve better performance without compromising the correctness of the program.
Optimization can occur at various levels:
- Algorithm-level optimization: Choosing the most efficient algorithms for your specific problem.
- Source code optimization: Writing code in a way that compilers can translate more efficiently into machine code.
- Compiler optimization: Letting the compiler automatically improve your code during the compilation process.
- Machine code optimization: Fine-tuning the assembly code generated by the compiler.
In this article, we’ll primarily focus on algorithm-level and source code optimization, as these are the areas where developers have the most direct control.
Why is Code Optimization Important?
You might wonder, “If my code works, why should I bother optimizing it?” Here are several compelling reasons:
- Improved Performance: Optimized code runs faster and uses fewer resources, leading to a better user experience.
- Cost Efficiency: Efficient code can reduce infrastructure costs, especially in cloud environments where resources are billed by usage.
- Scalability: Optimized code performs better as the size of the input or the number of users increases.
- Energy Efficiency: More efficient code consumes less power, which is crucial for mobile devices and environmentally conscious computing.
- Competitive Advantage: In many industries, the speed of your software can be a significant differentiator.
Principles of Code Optimization
Before we dive into specific techniques, let’s establish some guiding principles for code optimization:
- Measure First: Always profile your code before optimizing. Identify the actual bottlenecks rather than making assumptions.
- Optimize the Critical Path: Focus on the parts of your code that are executed most frequently or consume the most resources.
- Consider Trade-offs: Sometimes, optimizing for speed might increase memory usage, or vice versa. Understand the trade-offs and choose based on your priorities.
- Maintain Readability: Don’t sacrifice code clarity for minor performance gains. Readable code is easier to maintain and less prone to bugs.
- Use Built-in Functions and Libraries: Language-provided functions and well-established libraries are often highly optimized.
- Be Aware of Your Hardware: Understanding the underlying hardware can help you make better optimization decisions.
Common Code Optimization Techniques
Now, let’s explore some widely applicable optimization techniques:
1. Algorithm Selection and Improvement
Choosing the right algorithm for your problem is often the most impactful optimization you can make. For example, consider sorting an array of integers:
// Inefficient bubble sort (O(n^2))
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
// More efficient quicksort (O(n log n) on average)
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
For large arrays, quicksort will significantly outperform bubble sort.
2. Avoiding Redundant Computations
Store and reuse results of expensive computations instead of recalculating them:
// Inefficient
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
// Optimized with memoization
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
3. Loop Optimization
Loops are often the most time-consuming parts of a program. Here are some loop optimization techniques:
- Loop Unrolling: Reduce loop overhead by doing more work in each iteration.
- Minimizing Loop Contents: Move operations that don’t change within the loop outside of it.
- Using appropriate loop constructs: For example, using a while loop instead of a for loop when the number of iterations is unknown.
// Before optimization
for (int i = 0; i < n; i++) {
result += expensive_function(i);
}
// After optimization
int temp = expensive_function(0);
for (int i = 0; i < n; i++) {
result += temp;
temp = expensive_function(i + 1);
}
4. Data Structure Selection
Choosing the right data structure can dramatically affect performance. For example:
- Use a hash table (e.g., HashMap in Java, dict in Python) for fast lookups instead of a list when you need frequent access by key.
- Use a linked list for frequent insertions/deletions at the beginning or end of the list.
- Use a balanced binary search tree (e.g., TreeMap in Java) for maintaining a sorted collection with fast insertions, deletions, and lookups.
5. Caching
Caching involves storing computed results to avoid redundant calculations. This can be particularly effective in web applications:
import functools
@functools.lru_cache(maxsize=None)
def expensive_function(n):
# Some expensive computation
return result
This Python decorator will cache the results of the expensive_function, dramatically speeding up repeated calls with the same arguments.
6. Lazy Evaluation
Lazy evaluation delays the evaluation of an expression until its value is needed. This can save computation time and memory:
// Eager evaluation (computes all values immediately)
const allNumbers = Array.from({length: 1000000}, (_, i) => i);
const evenNumbers = allNumbers.filter(n => n % 2 === 0);
// Lazy evaluation (computes values on-demand)
function* lazyRange(start, end) {
for (let i = start; i < end; i++) {
yield i;
}
}
const lazyEvenNumbers = function* () {
for (const num of lazyRange(0, 1000000)) {
if (num % 2 === 0) yield num;
}
};
7. Compiler Optimization Flags
Most compilers offer optimization flags that can significantly improve performance. For example, in GCC:
gcc -O3 myprogram.c -o myprogram
The -O3 flag enables aggressive optimizations. Be sure to test thoroughly, as aggressive optimizations can sometimes introduce subtle bugs.
Advanced Optimization Techniques
As you become more proficient, you might explore more advanced optimization techniques:
1. Parallel Processing
Utilize multiple cores or processors to perform computations simultaneously:
import multiprocessing
def process_chunk(chunk):
# Process the chunk of data
return result
if __name__ == '__main__':
with multiprocessing.Pool() as pool:
results = pool.map(process_chunk, data_chunks)
2. Vectorization
Leverage Single Instruction, Multiple Data (SIMD) operations for parallel processing of data:
import numpy as np
# Scalar operations (slow)
result = [x + y for x, y in zip(array1, array2)]
# Vectorized operations (fast)
result = np.array(array1) + np.array(array2)
3. Memory Management
In languages with manual memory management, proper allocation and deallocation can significantly impact performance:
// Inefficient
for (int i = 0; i < n; i++) {
char* buffer = (char*)malloc(size);
// Use buffer
free(buffer);
}
// More efficient
char* buffer = (char*)malloc(size);
for (int i = 0; i < n; i++) {
// Use buffer
}
free(buffer);
4. Database Query Optimization
For database-driven applications, optimizing queries can lead to dramatic performance improvements:
- Use appropriate indexes
- Avoid SELECT * and fetch only needed columns
- Use EXPLAIN to understand query execution plans
-- Inefficient
SELECT * FROM users WHERE last_login > '2023-01-01';
-- More efficient (assuming an index on last_login)
SELECT id, username, email FROM users WHERE last_login > '2023-01-01';
Tools for Code Optimization
Several tools can assist you in the optimization process:
- Profilers: Tools like cProfile (Python), JProfiler (Java), or Chrome DevTools (JavaScript) can help identify performance bottlenecks.
- Static Code Analyzers: Tools like SonarQube or ESLint can identify potential performance issues in your code.
- Benchmark Suites: Frameworks like JMH (Java) or Benchmark.js (JavaScript) allow you to accurately measure the performance of your code.
- Memory Analyzers: Tools like Valgrind or Java VisualVM can help identify memory leaks and inefficient memory usage.
Common Pitfalls in Code Optimization
While optimizing code is important, it’s easy to fall into some common traps:
- Premature Optimization: Don’t optimize before you have a working solution and have identified actual bottlenecks.
- Over-optimization: Sometimes, the performance gain doesn’t justify the increased complexity or reduced readability.
- Micro-optimizations: Focusing on tiny optimizations while ignoring larger algorithmic improvements.
- Not Measuring: Always measure the impact of your optimizations. Sometimes, what seems like an optimization can actually degrade performance.
- Ignoring Maintainability: Extremely optimized code can be hard to understand and maintain. Strike a balance between performance and readability.
Case Study: Optimizing a Web Application
Let’s consider a hypothetical e-commerce web application that’s experiencing performance issues. Here’s how we might approach optimizing it:
- Profiling: We use browser developer tools to identify that the product listing page is slow to load.
- Database Optimization: We optimize the database query fetching products:
-- Before SELECT * FROM products ORDER BY created_at DESC; -- After SELECT id, name, price, image_url FROM products WHERE status = 'active' ORDER BY created_at DESC LIMIT 50;
- Caching: We implement Redis caching for frequently accessed data:
def get_product(product_id): # Try to get the product from cache product = redis_client.get(f'product:{product_id}') if product: return json.loads(product) # If not in cache, fetch from database product = db.query(Product).get(product_id) # Store in cache for future requests redis_client.setex(f'product:{product_id}', 3600, json.dumps(product.to_dict())) return product
- Front-end Optimization: We implement lazy loading for images and pagination for product listings.
- CDN Usage: We serve static assets (images, CSS, JavaScript) through a Content Delivery Network to reduce server load and improve load times for users across different geographical locations.
After implementing these optimizations, we measure the performance again and find that page load times have decreased by 60%, and the server can handle 3x more concurrent users.
Conclusion
Code optimization is a crucial skill for any developer looking to create efficient, scalable, and responsive applications. By understanding and applying these optimization techniques, you can significantly improve the performance of your code.
Remember, optimization is an ongoing process. As your application evolves and grows, new performance challenges will arise. Always be prepared to measure, analyze, and improve your code.
The journey to mastering code optimization is long and filled with continuous learning. But with each optimization you make, you’re not just improving your code – you’re becoming a better, more thoughtful programmer. So keep exploring, keep measuring, and keep optimizing. Your future self (and your users) will thank you for it!