Understanding Edge Cases: Thinking Beyond the Basics
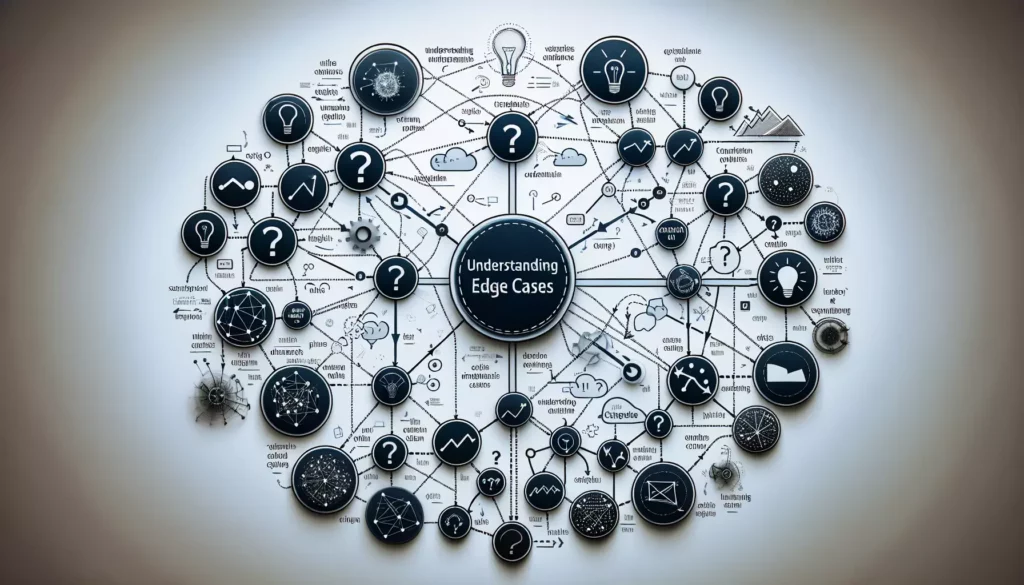
In the world of programming and software development, edge cases are those pesky scenarios that often slip through the cracks during initial planning and coding. They’re the unexpected inputs, unusual conditions, or rare situations that can cause our carefully crafted algorithms to stumble or even fail completely. As aspiring developers and coding enthusiasts, understanding and handling edge cases is crucial for creating robust, reliable software. In this comprehensive guide, we’ll dive deep into the concept of edge cases, explore their importance, and learn strategies for identifying and addressing them effectively.
What Are Edge Cases?
Edge cases, also known as corner cases or boundary cases, are situations that occur at the extreme ends of possible inputs or conditions in a program. These are scenarios that may be rare or unexpected but can still occur in real-world usage. Edge cases often test the limits of your code and can reveal hidden bugs or vulnerabilities that might not be apparent during typical use.
For example, consider a simple function that calculates the average of a list of numbers:
def calculate_average(numbers):
return sum(numbers) / len(numbers)
While this function works fine for most inputs, it fails to account for several edge cases:
- What happens if the list is empty?
- How does it handle negative numbers?
- What if the sum of the numbers exceeds the maximum value that can be represented by the programming language?
These scenarios represent edge cases that need to be considered to make the function more robust and reliable.
Why Are Edge Cases Important?
Understanding and addressing edge cases is crucial for several reasons:
- Improved Reliability: By accounting for edge cases, you create software that can handle a wider range of inputs and scenarios, making it more reliable in real-world use.
- Enhanced User Experience: Properly handled edge cases prevent unexpected errors or crashes, leading to a smoother and more enjoyable user experience.
- Reduced Bugs: Many bugs in software are a result of unhandled edge cases. By identifying and addressing these scenarios early, you can significantly reduce the number of bugs in your code.
- Better Code Quality: Thinking about edge cases forces you to write more comprehensive and well-thought-out code, improving overall code quality.
- Increased Security: Some edge cases can lead to security vulnerabilities if not properly handled. Addressing these scenarios can help protect your software from potential exploits.
Common Types of Edge Cases
While edge cases can vary depending on the specific problem or application, there are several common types that you should be aware of:
1. Empty or Null Inputs
Always consider what should happen when your function or method receives an empty input or null value. For example:
def process_string(text):
if not text:
return "Empty input"
# Process the string
return processed_result
2. Boundary Values
Test your code with the minimum and maximum allowed values, as well as values just outside these boundaries. For instance, if your function expects numbers between 1 and 100:
def validate_number(num):
if num < 1 or num > 100:
raise ValueError("Number must be between 1 and 100")
# Process the number
return processed_result
3. Type Mismatches
In dynamically typed languages, consider what happens if an unexpected type is passed to your function:
def add_numbers(a, b):
if not (isinstance(a, (int, float)) and isinstance(b, (int, float))):
raise TypeError("Both arguments must be numbers")
return a + b
4. Duplicate or Conflicting Inputs
Think about how your code should handle duplicate values or conflicting inputs:
def merge_dictionaries(dict1, dict2):
result = dict1.copy()
for key, value in dict2.items():
if key in result:
# Handle conflicting keys
result[key] = [result[key], value]
else:
result[key] = value
return result
5. Resource Limitations
Consider scenarios where system resources (memory, disk space, network connectivity) are limited or unavailable:
def download_file(url, max_size_mb=100):
# Check available disk space
if get_available_disk_space() < max_size_mb * 1024 * 1024:
raise IOError("Insufficient disk space")
# Proceed with download
# ...
Strategies for Identifying Edge Cases
Identifying edge cases requires a combination of analytical thinking, experience, and creativity. Here are some strategies to help you uncover potential edge cases in your code:
1. Boundary Analysis
Examine the boundaries of your input domains. Consider the minimum and maximum values, as well as values just outside these boundaries. For example, if your function processes ages:
- What happens with age 0?
- How about negative ages?
- What’s the maximum age you’ll allow?
- How do you handle ages above that maximum?
2. Equivalence Partitioning
Divide your input domain into classes of equivalent inputs. Test representatives from each class, including the boundaries between classes. For a function that categorizes BMI:
- Underweight: BMI < 18.5
- Normal weight: 18.5 ≤ BMI < 25
- Overweight: 25 ≤ BMI < 30
- Obese: BMI ≥ 30
Test values at and around these boundaries (e.g., 18.4, 18.5, 18.6, 24.9, 25.0, 25.1, etc.)
3. Error Guessing
Based on your experience and intuition, try to guess potential error scenarios. Think about what could go wrong or what unusual inputs might be provided. For example, for a user registration form:
- What if the user enters HTML tags in their name?
- How do you handle extremely long email addresses?
- What about passwords with only spaces?
4. Use Case Analysis
Consider all possible ways a user might interact with your software, including unintended or unexpected uses. For a file upload feature:
- What if the user tries to upload an empty file?
- How do you handle extremely large files?
- What about files with unusual or no file extensions?
5. Code Review and Pair Programming
Collaborate with other developers to review your code and brainstorm potential edge cases. Fresh perspectives can often uncover scenarios you might have overlooked.
Techniques for Handling Edge Cases
Once you’ve identified potential edge cases, the next step is to handle them effectively in your code. Here are some techniques to consider:
1. Input Validation
Validate inputs early to ensure they meet your expected criteria. This can prevent many edge cases from occurring in the first place:
def process_age(age):
if not isinstance(age, int) or age < 0 or age > 150:
raise ValueError("Invalid age. Must be an integer between 0 and 150.")
# Process the age
return processed_result
2. Defensive Programming
Assume that things will go wrong and write code to handle potential errors or unexpected situations:
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
return "Error: Cannot divide by zero"
except TypeError:
return "Error: Both inputs must be numbers"
return result
3. Graceful Degradation
Design your system to handle reduced functionality when resources are limited or unavailable:
def fetch_data(url):
try:
response = requests.get(url, timeout=5)
return response.json()
except requests.Timeout:
# Fall back to cached data if available
return get_cached_data(url)
except requests.RequestException:
# Return minimal data set if all else fails
return get_minimal_data()
4. Logging and Monitoring
Implement robust logging and monitoring to help identify and diagnose edge cases in production:
import logging
def process_order(order_data):
try:
# Process the order
result = do_process_order(order_data)
logging.info(f"Order processed successfully: {order_data['id']}")
return result
except Exception as e:
logging.error(f"Error processing order {order_data['id']}: {str(e)}")
raise
5. Unit Testing
Write comprehensive unit tests that cover both typical scenarios and edge cases:
import unittest
class TestCalculator(unittest.TestCase):
def test_divide_normal(self):
self.assertEqual(calculate.divide(10, 2), 5)
def test_divide_by_zero(self):
with self.assertRaises(ValueError):
calculate.divide(10, 0)
def test_divide_float_result(self):
self.assertAlmostEqual(calculate.divide(10, 3), 3.333333, places=6)
if __name__ == '__main__':
unittest.main()
Real-World Examples of Edge Cases
To better understand the importance of handling edge cases, let’s look at some real-world examples where overlooked edge cases led to significant issues:
1. The Y2K Bug
The Y2K bug is a classic example of an edge case that had widespread implications. Many older computer systems stored years using two digits (e.g., “99” for 1999) to save memory. As the year 2000 approached, there were concerns that these systems would interpret “00” as 1900 instead of 2000, potentially causing widespread failures.
This edge case required extensive efforts to update and patch systems worldwide to prevent potential disasters.
2. Ariane 5 Rocket Explosion
In 1996, the Ariane 5 rocket exploded just 40 seconds after launch due to a software error. The error occurred when a 64-bit floating-point number was converted to a 16-bit signed integer, causing an overflow. This edge case wasn’t properly handled, leading to the rocket’s destruction and the loss of its payload.
3. Amazon’s “$23,698,655.93 Textbook”
In 2011, a biology textbook on Amazon was priced at over $23 million due to an algorithmic pricing error. Two sellers were using automated pricing algorithms that kept raising the price in response to each other, creating an unintended feedback loop. This edge case of algorithmic interaction led to an absurdly high price that went unnoticed for days.
4. Therac-25 Radiation Therapy Machine
The Therac-25 was a radiation therapy machine that caused several accidents in the 1980s, resulting in severe injuries and deaths. One of the issues was a race condition that could occur if the operator changed the machine’s settings too quickly. This edge case in the user interface design and software implementation led to the machine delivering massive overdoses of radiation.
5. Zune Leap Year Bug
On December 31, 2008, many Microsoft Zune 30GB devices froze and became unusable due to a leap year bug. The issue was caused by an infinite loop in the code that didn’t properly handle the last day of a leap year. This edge case affected a specific model on a specific date, highlighting the importance of thorough date and time handling in software.
Best Practices for Handling Edge Cases
To effectively manage edge cases in your software development process, consider adopting these best practices:
1. Start Early
Begin thinking about potential edge cases during the design phase of your project. Incorporating edge case handling from the start is often easier and more effective than trying to add it later.
2. Document Assumptions
Clearly document the assumptions your code makes about inputs, system state, and external conditions. This helps identify potential edge cases and makes it easier for other developers to understand and maintain your code.
3. Use Type Checking and Contracts
Implement type checking or use languages with strong type systems to catch type-related edge cases early. Consider using design by contract principles to define preconditions, postconditions, and invariants for your functions and methods.
4. Implement Comprehensive Error Handling
Develop a consistent error handling strategy that includes appropriate use of exceptions, error codes, and logging. Ensure that your error messages are informative and actionable.
5. Conduct Thorough Testing
Implement a comprehensive testing strategy that includes unit tests, integration tests, and system tests. Use techniques like property-based testing and fuzzing to generate a wide range of inputs and uncover potential edge cases.
6. Perform Code Reviews
Conduct regular code reviews with a focus on identifying potential edge cases. Encourage team members to think critically about possible failure modes and unexpected inputs.
7. Monitor and Learn from Production
Implement robust logging and monitoring in your production systems. Regularly analyze logs and error reports to identify edge cases that weren’t caught during development and testing.
8. Stay Updated on Common Pitfalls
Keep yourself informed about common programming pitfalls and edge cases in your technology stack. Stay updated on security vulnerabilities and best practices in your field.
Conclusion
Understanding and effectively handling edge cases is a crucial skill for any software developer. It’s the difference between code that works most of the time and code that’s truly robust and reliable. By thinking beyond the basics and considering the full range of possible inputs and scenarios, you can create software that stands up to the unpredictable nature of real-world usage.
Remember, addressing edge cases isn’t just about preventing errors—it’s about creating a better user experience, improving the maintainability of your code, and building systems that can be trusted to perform correctly under a wide range of conditions. As you continue to develop your programming skills, make edge case analysis a fundamental part of your problem-solving approach.
By consistently applying the strategies and best practices outlined in this guide, you’ll be well-equipped to tackle edge cases in your own projects. Whether you’re preparing for technical interviews at top tech companies or building your own applications, your ability to anticipate and handle edge cases will set you apart as a thoughtful and thorough developer.
So the next time you’re working on a coding problem or designing a new feature, take a moment to ask yourself: “What are the edge cases here? What could go wrong?” Your future self (and your users) will thank you for it.