Why Algorithms Are Key to Solving Coding Problems
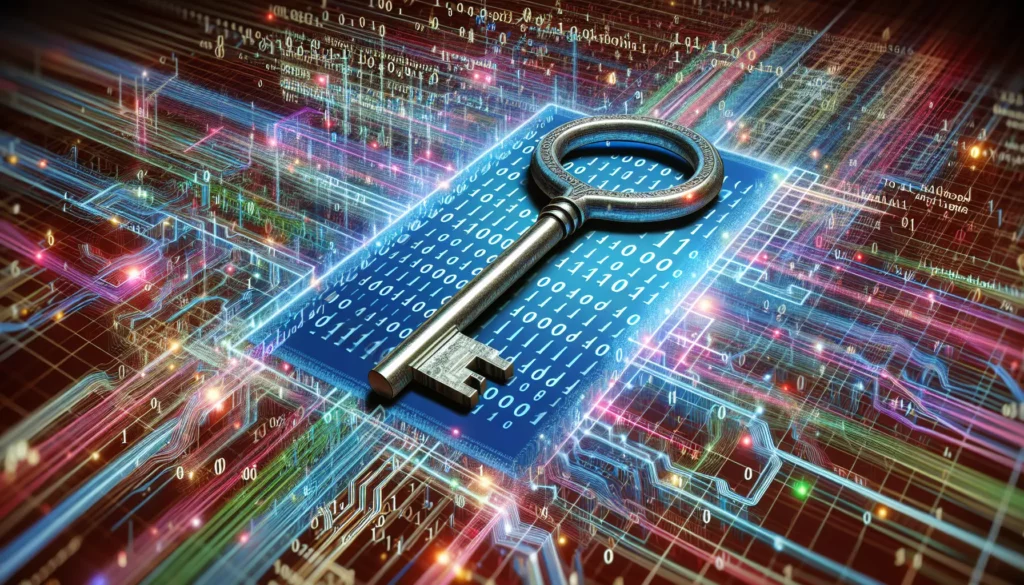
In the vast realm of computer science and programming, algorithms stand as the cornerstone of problem-solving. They are the essential building blocks that enable developers to create efficient, scalable, and robust solutions to complex computational challenges. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding and mastering algorithms is crucial for your success.
In this comprehensive guide, we’ll explore why algorithms are indispensable in solving coding problems, how they form the foundation of efficient programming, and how you can leverage them to enhance your problem-solving skills. We’ll also delve into practical examples, discuss common algorithmic paradigms, and provide insights on how to approach algorithm-based challenges in coding interviews.
What Are Algorithms?
Before we dive deeper, let’s establish a clear understanding of what algorithms are. In simple terms, an algorithm is a step-by-step procedure or a set of rules designed to solve a specific problem or perform a particular task. It’s like a recipe that outlines the exact steps needed to achieve a desired outcome.
In the context of computer science, algorithms are typically expressed as a series of instructions that a computer can execute to process input data and produce the desired output. These instructions can be implemented in various programming languages, but the underlying logic remains consistent regardless of the chosen language.
The Importance of Algorithms in Problem-Solving
Algorithms play a crucial role in problem-solving for several reasons:
- Efficiency: Well-designed algorithms can significantly improve the performance of your code, reducing execution time and resource consumption.
- Scalability: Algorithms help in creating solutions that can handle large amounts of data or complex operations without breaking down.
- Consistency: By following a well-defined algorithm, you can ensure consistent results across different inputs and scenarios.
- Reusability: Many algorithms can be applied to a wide range of problems, allowing you to leverage existing solutions for new challenges.
- Problem Decomposition: Algorithms help break down complex problems into smaller, more manageable steps.
Common Algorithmic Paradigms
To effectively solve coding problems, it’s essential to familiarize yourself with various algorithmic paradigms. These paradigms represent different approaches to problem-solving and can guide you in developing efficient solutions. Here are some of the most common paradigms:
1. Divide and Conquer
The divide and conquer approach involves breaking down a problem into smaller subproblems, solving them independently, and then combining the results to solve the original problem. This paradigm is particularly useful for tackling complex problems that can be recursively divided into simpler cases.
Example: Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
2. Dynamic Programming
Dynamic programming is a technique used to solve problems by breaking them down into smaller subproblems and storing the results for future use. This approach is particularly effective for optimization problems and can significantly reduce computational complexity.
Example: Fibonacci sequence using dynamic programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
3. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best overall solution, greedy algorithms are often simple to implement and can provide good approximations for certain problems.
Example: Coin change problem using a greedy approach
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
4. Backtracking
Backtracking is an algorithmic technique that involves building a solution incrementally and abandoning a path as soon as it determines that the path cannot lead to a valid solution. This approach is particularly useful for solving constraint satisfaction problems and combinatorial optimization challenges.
Example: N-Queens problem using backtracking
def solve_n_queens(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0) == False:
print("Solution does not exist")
return False
return board
# Example usage
solution = solve_n_queens(8)
if solution:
for row in solution:
print(row)
Why Algorithms Matter in Coding Interviews
Understanding and implementing algorithms is particularly crucial when preparing for coding interviews, especially for positions at top tech companies. Here’s why:
- Problem-Solving Skills: Algorithms demonstrate your ability to analyze problems, break them down into manageable components, and develop efficient solutions.
- Code Optimization: Knowledge of algorithms allows you to write more efficient and optimized code, which is highly valued in professional settings.
- Scalability Considerations: Interviewers often assess your ability to consider scalability when solving problems, which is directly tied to algorithmic efficiency.
- Foundation for Complex Systems: Understanding fundamental algorithms provides a strong foundation for working with and developing complex software systems.
- Industry Standard: Algorithmic problem-solving is a standard assessment method in the tech industry, particularly for software engineering roles.
How to Approach Algorithmic Problems
When faced with an algorithmic problem, whether in an interview or real-world scenario, follow these steps to develop an effective solution:
- Understand the Problem: Carefully read and analyze the problem statement. Identify the input, expected output, and any constraints or edge cases.
- Break It Down: Divide the problem into smaller, more manageable subproblems or steps.
- Consider Multiple Approaches: Think about different algorithmic paradigms that might be applicable to the problem.
- Start with a Brute Force Solution: Begin with a simple, straightforward solution to ensure you understand the problem correctly.
- Optimize: Look for ways to improve the efficiency of your initial solution, considering time and space complexity.
- Implement: Write clean, well-structured code to implement your chosen algorithm.
- Test and Debug: Verify your solution with various test cases, including edge cases, and debug any issues.
- Analyze Complexity: Determine the time and space complexity of your solution and be prepared to discuss trade-offs.
Common Algorithmic Concepts to Master
To excel in solving coding problems and performing well in technical interviews, focus on mastering these fundamental algorithmic concepts:
1. Array Manipulation
Arrays are fundamental data structures, and many problems involve manipulating or searching through arrays efficiently. Key concepts include:
- Two-pointer technique
- Sliding window
- Kadane’s algorithm for maximum subarray sum
Example: Two-pointer technique for finding a pair with a given sum
def find_pair(arr, target_sum):
left = 0
right = len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target_sum:
return (arr[left], arr[right])
elif current_sum < target_sum:
left += 1
else:
right -= 1
return None # No pair found
2. String Manipulation
String problems are common in coding interviews. Important concepts include:
- String matching algorithms (e.g., KMP, Rabin-Karp)
- Palindrome checking
- String parsing and tokenization
Example: Checking if a string is a palindrome
def is_palindrome(s):
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
3. Linked Lists
Linked list problems often test your ability to manipulate pointers and handle edge cases. Key operations include:
- Reversing a linked list
- Detecting cycles
- Merging sorted lists
Example: Reversing a linked list
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
4. Trees and Graphs
Tree and graph problems are crucial for assessing your understanding of more complex data structures. Important concepts include:
- Tree traversals (in-order, pre-order, post-order)
- Binary search trees
- Graph traversals (BFS, DFS)
- Shortest path algorithms (e.g., Dijkstra’s, Bellman-Ford)
Example: Depth-First Search (DFS) for a binary tree
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs_inorder(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
5. Sorting and Searching
Understanding various sorting and searching algorithms is essential. Key algorithms include:
- Quick Sort
- Merge Sort
- Binary Search
- Heap Sort
Example: Binary search implementation
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Practicing Algorithmic Problem-Solving
To improve your algorithmic problem-solving skills, consider the following strategies:
- Solve Problems Regularly: Dedicate time each day to solving coding problems on platforms like LeetCode, HackerRank, or CodeSignal.
- Study Classic Algorithms: Familiarize yourself with well-known algorithms and their implementations.
- Analyze Time and Space Complexity: Practice evaluating the efficiency of your solutions in terms of big O notation.
- Participate in Coding Contests: Join online coding competitions to challenge yourself and learn from others.
- Review and Optimize: After solving a problem, look for ways to improve your solution or explore alternative approaches.
- Collaborate and Discuss: Join coding communities or study groups to share ideas and learn from peers.
- Mock Interviews: Practice explaining your thought process and coding in a simulated interview environment.
Conclusion
Algorithms are the backbone of efficient problem-solving in computer science and software development. By mastering algorithmic thinking and common paradigms, you’ll be better equipped to tackle complex coding challenges, optimize your solutions, and excel in technical interviews.
Remember that becoming proficient in algorithms is a journey that requires consistent practice and a willingness to learn from both successes and failures. As you continue to hone your skills, you’ll find that algorithmic problem-solving becomes second nature, enabling you to approach new challenges with confidence and creativity.
Whether you’re preparing for interviews at top tech companies or simply aiming to become a more skilled developer, investing time in understanding and implementing algorithms will pay dividends throughout your programming career. Embrace the challenge, stay curious, and keep coding!