Deep Dive into Stacks and Queues: Simplified
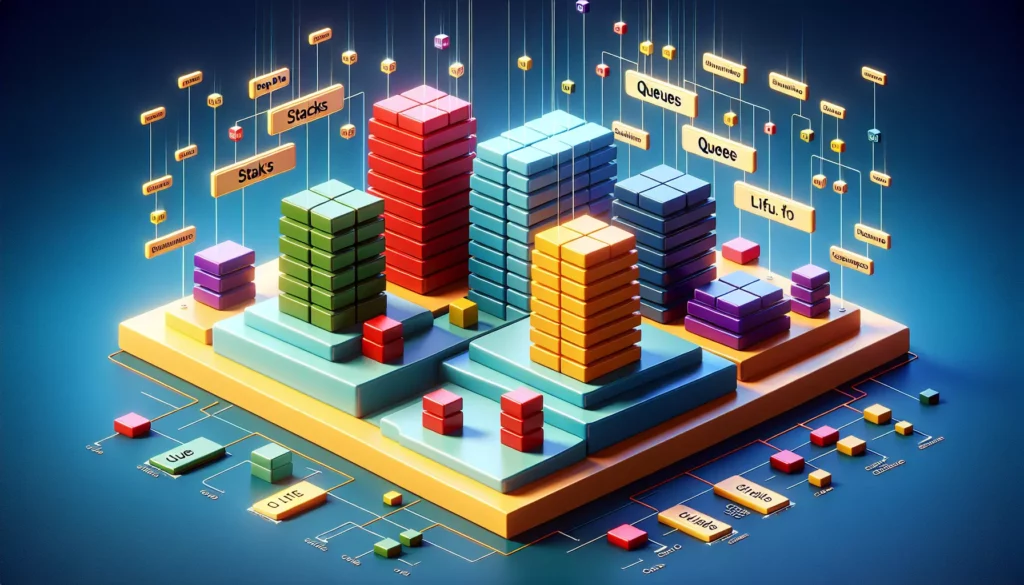
In the world of computer science and programming, data structures play a crucial role in organizing and managing information efficiently. Two fundamental data structures that every programmer should be familiar with are stacks and queues. These structures are not only essential for solving various programming problems but also form the basis for more complex data structures and algorithms. In this comprehensive guide, we’ll take a deep dive into stacks and queues, exploring their concepts, implementations, and real-world applications.
Understanding Stacks
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of a stack of plates in a cafeteria – you add plates to the top of the stack and remove them from the top as well.
Key Operations of a Stack
- Push: Adds an element to the top of the stack
- Pop: Removes the top element from the stack
- Peek or Top: Returns the top element without removing it
- IsEmpty: Checks if the stack is empty
- Size: Returns the number of elements in the stack
Implementing a Stack in Python
Let’s implement a simple stack using Python’s built-in list data structure:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Example usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
Applications of Stacks
- Function Call Management: Programming languages use stacks to manage function calls and their local variables.
- Expression Evaluation: Stacks are used to evaluate arithmetic expressions, especially in postfix notation.
- Undo Mechanism: Many applications use stacks to implement undo functionality.
- Backtracking Algorithms: Stacks are essential in backtracking algorithms, such as maze solving or tree traversals.
- Parentheses Matching: Stacks can be used to check if parentheses in an expression are balanced.
Understanding Queues
A queue is another linear data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. Think of a queue of people waiting in line – the first person to join the line is the first to be served.
Key Operations of a Queue
- Enqueue: Adds an element to the rear of the queue
- Dequeue: Removes the front element from the queue
- Front: Returns the front element without removing it
- IsEmpty: Checks if the queue is empty
- Size: Returns the number of elements in the queue
Implementing a Queue in Python
Let’s implement a simple queue using Python’s collections.deque, which provides an efficient implementation for queue operations:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Example usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
print(queue.size()) # Output: 2
Applications of Queues
- Task Scheduling: Operating systems use queues to manage processes and tasks.
- Breadth-First Search: Queues are used in graph algorithms like BFS for traversing nodes level by level.
- Buffering: Queues are used in various scenarios for buffering data, such as in print spooling or data streams.
- Message Queues: In distributed systems, queues are used for inter-process communication and task distribution.
- Handling of Requests: Web servers use queues to handle incoming client requests in a first-come, first-served manner.
Advanced Concepts: Variations of Stacks and Queues
While the basic implementations of stacks and queues are straightforward, there are several variations and advanced concepts that build upon these fundamental structures. Let’s explore some of these:
1. Double-Ended Queue (Deque)
A deque (pronounced “deck”) is a double-ended queue that allows insertion and deletion at both ends. It combines the features of both stacks and queues, providing a versatile data structure for various applications.
from collections import deque
d = deque()
d.append(1) # Add to right
d.appendleft(2) # Add to left
d.pop() # Remove from right
d.popleft() # Remove from left
2. Priority Queue
A priority queue is a type of queue where elements have associated priorities. Elements with higher priority are dequeued before elements with lower priority. This is often implemented using a heap data structure.
import heapq
pq = []
heapq.heappush(pq, (2, "task 2"))
heapq.heappush(pq, (1, "task 1"))
heapq.heappush(pq, (3, "task 3"))
while pq:
print(heapq.heappop(pq)) # Will print in order of priority
3. Circular Queue
A circular queue is a variation of a queue where the last position is connected back to the first position to form a circle. This is useful for applications where we want to reuse queue space efficiently.
class CircularQueue:
def __init__(self, size):
self.size = size
self.queue = [None] * size
self.front = self.rear = -1
def enqueue(self, data):
if ((self.rear + 1) % self.size == self.front):
print("Queue is full")
elif (self.front == -1):
self.front = 0
self.rear = 0
self.queue[self.rear] = data
else:
self.rear = (self.rear + 1) % self.size
self.queue[self.rear] = data
def dequeue(self):
if (self.front == -1):
print("Queue is empty")
elif (self.front == self.rear):
temp = self.queue[self.front]
self.front = -1
self.rear = -1
return temp
else:
temp = self.queue[self.front]
self.front = (self.front + 1) % self.size
return temp
4. Stack with Min/Max Operation
This is a stack that supports push, pop, and retrieving the minimum (or maximum) element in constant time. This can be achieved by maintaining an auxiliary stack that keeps track of the minimum (or maximum) elements.
class MinStack:
def __init__(self):
self.stack = []
self.min_stack = []
def push(self, x):
self.stack.append(x)
if not self.min_stack or x <= self.min_stack[-1]:
self.min_stack.append(x)
def pop(self):
if self.stack:
if self.stack[-1] == self.min_stack[-1]:
self.min_stack.pop()
return self.stack.pop()
def top(self):
if self.stack:
return self.stack[-1]
def getMin(self):
if self.min_stack:
return self.min_stack[-1]
Real-World Applications and Interview Questions
Understanding stacks and queues is crucial for solving various programming problems and is often tested in technical interviews. Let’s look at some common applications and interview questions related to these data structures:
1. Balanced Parentheses
Problem: Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
def is_valid(s):
stack = []
mapping = {")": "(", "}": "{", "]": "["}
for char in s:
if char in mapping:
top_element = stack.pop() if stack else '#'
if mapping[char] != top_element:
return False
else:
stack.append(char)
return not stack
# Test
print(is_valid("()[]{}")) # True
print(is_valid("([)]")) # False
2. Implement Queue using Stacks
Problem: Implement a first in first out (FIFO) queue using only two stacks. The implemented queue should support all the functions of a normal queue (enqueue, dequeue, front, empty).
class QueueUsingStacks:
def __init__(self):
self.stack1 = []
self.stack2 = []
def enqueue(self, x):
self.stack1.append(x)
def dequeue(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if self.stack2:
return self.stack2.pop()
return None
def front(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if self.stack2:
return self.stack2[-1]
return None
def empty(self):
return len(self.stack1) == 0 and len(self.stack2) == 0
# Test
q = QueueUsingStacks()
q.enqueue(1)
q.enqueue(2)
print(q.dequeue()) # 1
q.enqueue(3)
print(q.dequeue()) # 2
print(q.dequeue()) # 3
3. Sliding Window Maximum
Problem: Given an array nums and a sliding window of size k which is moving from the very left of the array to the very right. You can only see the k numbers in the window. Each time the sliding window moves right by one position, return the max sliding window.
from collections import deque
def max_sliding_window(nums, k):
result = []
window = deque()
for i, num in enumerate(nums):
while window and window[0] <= i - k:
window.popleft()
while window and nums[window[-1]] < num:
window.pop()
window.append(i)
if i >= k - 1:
result.append(nums[window[0]])
return result
# Test
print(max_sliding_window([1,3,-1,-3,5,3,6,7], 3))
# Output: [3,3,5,5,6,7]
Advanced Topics and Optimizations
As we delve deeper into the world of stacks and queues, it’s important to consider some advanced topics and optimizations that can enhance their performance and applicability:
1. Time and Space Complexity Analysis
Understanding the time and space complexity of stack and queue operations is crucial for efficient algorithm design. Here’s a quick overview:
- Stack operations (push, pop, peek): O(1) time complexity
- Queue operations (enqueue, dequeue, front): O(1) time complexity when implemented with a linked list or a circular array
- Space complexity: O(n) for both stacks and queues, where n is the number of elements
2. Amortized Analysis of Dynamic Arrays
When implementing stacks or queues using dynamic arrays (like Python’s list), the time complexity of push/enqueue operations can be amortized O(1). This concept is important to understand for a deeper grasp of data structure efficiency.
3. Thread-Safe Implementations
In multi-threaded environments, it’s crucial to have thread-safe implementations of stacks and queues. Python’s queue module provides thread-safe queue implementations:
import queue
# Thread-safe FIFO queue
q = queue.Queue()
q.put(1)
q.put(2)
print(q.get()) # 1
# Thread-safe LIFO queue (stack)
s = queue.LifoQueue()
s.put(1)
s.put(2)
print(s.get()) # 2
# Thread-safe priority queue
pq = queue.PriorityQueue()
pq.put((2, 'task 2'))
pq.put((1, 'task 1'))
print(pq.get()) # (1, 'task 1')
4. Memory-Efficient Implementations
For scenarios where memory efficiency is crucial, consider using fixed-size arrays or linked lists instead of dynamic arrays. This can help in reducing memory overhead and improving cache performance in certain cases.
5. Custom Comparators in Priority Queues
When working with priority queues, it’s often useful to define custom comparison logic for complex objects:
import heapq
class Task:
def __init__(self, priority, description):
self.priority = priority
self.description = description
def __lt__(self, other):
return self.priority < other.priority
pq = []
heapq.heappush(pq, Task(3, "Low priority task"))
heapq.heappush(pq, Task(1, "High priority task"))
heapq.heappush(pq, Task(2, "Medium priority task"))
while pq:
task = heapq.heappop(pq)
print(f"Processing: {task.description}")
Conclusion: Mastering Stacks and Queues
Stacks and queues are fundamental data structures that form the building blocks of many complex algorithms and systems. By understanding their principles, implementations, and applications, you’ll be well-equipped to tackle a wide range of programming challenges and technical interviews.
Remember these key takeaways:
- Stacks follow the LIFO principle, while queues follow the FIFO principle.
- Both structures have constant-time O(1) operations for their main functionalities.
- Variations like deques, priority queues, and circular queues extend their capabilities for specific use cases.
- Real-world applications include function call management, task scheduling, and graph algorithms.
- Proficiency in implementing and using these structures is crucial for technical interviews and practical programming scenarios.
As you continue your journey in programming and computer science, keep exploring more advanced topics related to stacks and queues. Practice implementing them in different programming languages, solve related algorithmic problems, and consider how they can be applied in various software engineering contexts. With dedication and practice, you’ll master these essential data structures and enhance your problem-solving skills significantly.