How to Practice Programming Fundamentals Daily: A Comprehensive Guide
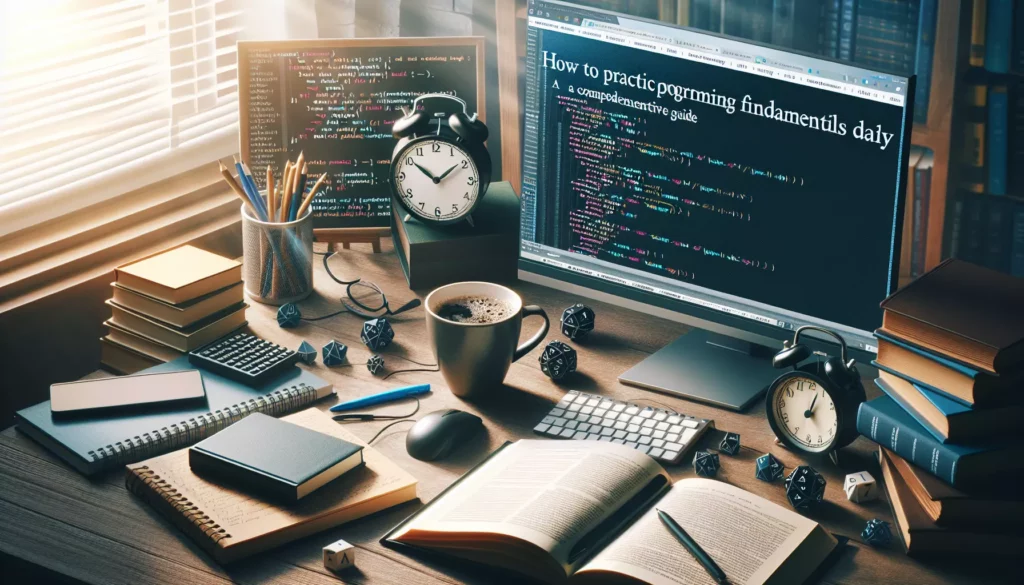
In the ever-evolving world of technology, mastering programming fundamentals is crucial for anyone aspiring to build a successful career in software development. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills, daily practice is the key to improvement. This comprehensive guide will explore various strategies and techniques to help you practice programming fundamentals effectively every day.
Why Daily Practice Matters
Before diving into the specifics of how to practice, it’s important to understand why daily practice is so vital in programming. Here are a few key reasons:
- Muscle Memory: Consistent practice helps develop muscle memory for coding syntax and common patterns.
- Problem-Solving Skills: Regular exposure to different problems enhances your ability to approach and solve complex issues.
- Keeping Up with Technology: The tech world evolves rapidly, and daily practice helps you stay current.
- Building Confidence: As you solve more problems, you’ll gain confidence in your abilities.
- Career Advancement: Improved skills can lead to better job opportunities and career growth.
Setting Up Your Daily Practice Routine
Establishing a consistent routine is crucial for effective daily practice. Here’s how you can set up a productive practice schedule:
1. Choose a Dedicated Time
Select a specific time each day for your practice. This could be early morning, during lunch breaks, or in the evening. Consistency is key, so pick a time that works best with your schedule.
2. Start Small
Begin with short practice sessions, perhaps 15-30 minutes a day. As you build the habit, you can gradually increase the duration.
3. Create a Distraction-Free Environment
Find a quiet space where you can focus without interruptions. Turn off notifications on your devices to maintain concentration.
4. Set Clear Goals
Define what you want to achieve in each practice session. This could be solving a specific number of problems, learning a new concept, or working on a personal project.
Effective Strategies for Daily Programming Practice
Now that you’ve set up your routine, let’s explore various strategies to make your daily practice sessions more effective and engaging.
1. Coding Challenges and Puzzles
Platforms like LeetCode, HackerRank, and CodeWars offer a wide range of coding challenges that test your problem-solving skills and knowledge of algorithms and data structures. Start with easier problems and gradually move to more complex ones.
Example of a simple coding challenge:
// Write a function that reverses a string
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("hello")); // Output: "olleh"
2. Build Small Projects
Working on small, personal projects is an excellent way to apply your knowledge and learn new concepts. Here are some ideas:
- A simple calculator
- A to-do list application
- A weather app that fetches data from an API
- A basic blog platform
These projects help you understand how different components work together in a real-world application.
3. Read and Analyze Code
Spend time reading and understanding code written by others. This could be open-source projects on GitHub or code snippets from programming blogs. Try to understand the logic, coding style, and best practices used.
4. Practice Typing Code
Improve your coding speed and accuracy by practicing typing code. Websites like TypeRacer for Code offer typing exercises specifically for programmers.
5. Implement Data Structures and Algorithms
Regularly implement common data structures and algorithms from scratch. This deepens your understanding of how they work and improves your problem-solving skills.
Example of implementing a simple linked list:
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
}
append(data) {
const newNode = new Node(data);
if (!this.head) {
this.head = newNode;
return;
}
let current = this.head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
print() {
let current = this.head;
while (current) {
console.log(current.data);
current = current.next;
}
}
}
const list = new LinkedList();
list.append(1);
list.append(2);
list.append(3);
list.print(); // Output: 1 2 3
6. Contribute to Open Source Projects
Contributing to open source projects is an excellent way to practice coding, learn from others, and give back to the community. Start with small contributions like fixing bugs or improving documentation.
7. Pair Programming
Find a coding buddy or join online communities where you can practice pair programming. This helps you learn different approaches to problem-solving and improves your ability to explain your code.
8. Code Review Practice
Regularly review your own code or participate in code reviews with peers. This helps you identify areas for improvement and learn best practices.
Tools and Resources for Daily Practice
To support your daily practice routine, consider using these tools and resources:
1. Online Learning Platforms
- AlgoCademy: Offers interactive coding tutorials and resources for learners, with a focus on algorithmic thinking and problem-solving.
- Codecademy: Provides interactive coding lessons in various programming languages.
- freeCodeCamp: Offers free coding challenges and projects with certifications.
2. Coding Challenge Websites
- LeetCode: Features a large collection of coding problems, often used for technical interview preparation.
- HackerRank: Offers coding challenges and competitions in various domains.
- CodeWars: Provides gamified coding challenges called “kata”.
3. Version Control Systems
- Git: Essential for tracking changes in your code and collaborating with others.
- GitHub: A platform for hosting and sharing your code repositories.
4. Integrated Development Environments (IDEs)
- Visual Studio Code: A popular, lightweight code editor with extensive plugin support.
- JetBrains IDEs: Specialized IDEs for different programming languages (e.g., IntelliJ IDEA for Java, PyCharm for Python).
5. Documentation and Learning Resources
- MDN Web Docs: Comprehensive documentation for web technologies.
- Stack Overflow: A community-driven Q&A platform for programming-related questions.
- Programming Language Official Docs: Always refer to the official documentation of the language you’re practicing.
Tracking Your Progress
Monitoring your progress is crucial for staying motivated and identifying areas for improvement. Here are some ways to track your programming practice:
1. Keep a Coding Journal
Maintain a daily log of what you’ve learned, challenges you’ve faced, and solutions you’ve discovered. This helps reinforce your learning and provides a record of your progress.
2. Use GitHub Contributions
Make it a habit to commit your daily practice code to GitHub. The contributions graph provides a visual representation of your consistency.
3. Set Milestones
Create short-term and long-term goals for your programming skills. Celebrate when you achieve these milestones to stay motivated.
4. Take Regular Assessments
Periodically test your skills through coding assessments or by revisiting problems you struggled with in the past. This helps you gauge your improvement over time.
Overcoming Challenges in Daily Practice
Maintaining a daily practice routine can be challenging. Here are some common obstacles and how to overcome them:
1. Lack of Motivation
Solution: Set clear, achievable goals and remind yourself why you started this journey. Celebrate small wins to stay motivated.
2. Time Constraints
Solution: Start with short, focused practice sessions. Even 15 minutes of quality practice is better than no practice at all.
3. Feeling Overwhelmed
Solution: Break down complex topics into smaller, manageable parts. Focus on one concept at a time.
4. Plateau in Learning
Solution: Vary your practice routine. Try new challenges, switch programming languages, or work on different types of projects to keep things interesting.
5. Imposter Syndrome
Solution: Remember that everyone starts somewhere. Focus on your progress rather than comparing yourself to others.
Advanced Techniques for Seasoned Programmers
If you’re an experienced programmer looking to take your skills to the next level, consider these advanced techniques:
1. Implement Complex Algorithms
Challenge yourself by implementing advanced algorithms like graph algorithms, dynamic programming solutions, or machine learning algorithms from scratch.
2. Explore New Programming Paradigms
If you’re comfortable with one programming paradigm (e.g., object-oriented), try learning a new one like functional programming or logic programming.
3. Deep Dive into System Design
Practice designing scalable systems by working on system design problems. This is crucial for senior-level positions and technical interviews at major tech companies.
4. Contribute to Large Open Source Projects
Move beyond small contributions and take on more significant roles in open source projects. This exposes you to large-scale software development practices.
5. Teach Others
Teaching is an excellent way to solidify your own understanding. Consider mentoring junior developers, writing technical blog posts, or creating coding tutorials.
Conclusion
Practicing programming fundamentals daily is a powerful way to improve your coding skills, problem-solving abilities, and overall understanding of software development. By establishing a consistent routine, utilizing various practice strategies, and leveraging the right tools and resources, you can make significant progress in your programming journey.
Remember, the key to success is consistency and perseverance. Start small, stay committed, and gradually increase the complexity of your practice as you grow. Whether you’re a beginner aiming to land your first programming job or an experienced developer looking to stay sharp, daily practice will help you achieve your goals and thrive in the dynamic world of technology.
Happy coding, and may your daily practice lead you to new heights in your programming career!