Mastering Hash Tables: Keys, Values, and Fast Lookups
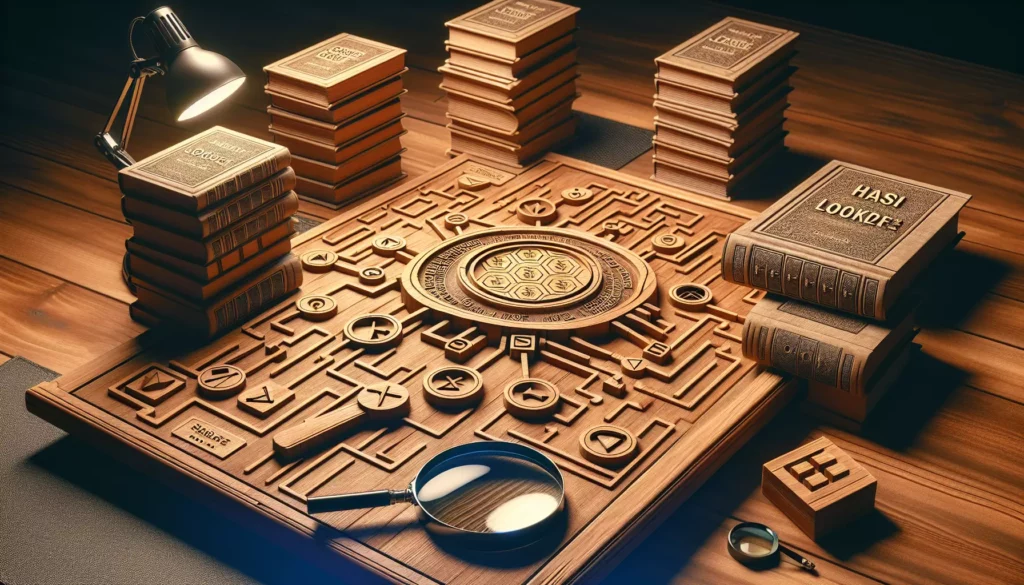
In the world of computer science and programming, efficient data structures are crucial for developing high-performance applications. Among these structures, hash tables stand out as a powerful tool for organizing and retrieving data quickly. In this comprehensive guide, we’ll dive deep into the world of hash tables, exploring their inner workings, implementation techniques, and practical applications.
What are Hash Tables?
Hash tables, also known as hash maps, are data structures that store key-value pairs and provide fast lookups, insertions, and deletions. They achieve this efficiency by using a hash function to map keys to array indices, allowing for constant-time average-case complexity for basic operations.
The main components of a hash table are:
- An array to store the data
- A hash function to map keys to array indices
- A collision resolution strategy to handle multiple keys mapping to the same index
How Hash Tables Work
The basic idea behind hash tables is to use a hash function to compute an index for each key, and then store the corresponding value at that index in an array. When you need to retrieve a value, you simply hash the key again and look up the value at the computed index.
Here’s a simple example of how a hash table might work:
// Hash function
function hash(key) {
return key.length % 10;
}
// Hash table
const table = new Array(10);
// Insert a key-value pair
function insert(key, value) {
const index = hash(key);
table[index] = value;
}
// Retrieve a value
function get(key) {
const index = hash(key);
return table[index];
}
// Usage
insert("apple", 5);
insert("banana", 7);
console.log(get("apple")); // Output: 5
console.log(get("banana")); // Output: 7
This is a simplified example, and real-world hash tables are more complex, but it illustrates the basic concept.
Hash Functions
The hash function is a critical component of a hash table. It should have the following properties:
- Deterministic: The same key should always produce the same hash value.
- Efficient: The hash function should be fast to compute.
- Uniform distribution: It should distribute keys evenly across the array to minimize collisions.
Common hash functions include:
- Division method: h(k) = k mod m, where m is the size of the hash table
- Multiplication method: h(k) = ⌊m(kA mod 1)⌋, where A is a constant between 0 and 1
- Universal hashing: A family of hash functions chosen at random to avoid worst-case scenarios
Collision Resolution
Collisions occur when two different keys hash to the same index. There are two main strategies for handling collisions:
1. Chaining
In chaining, each array element is a linked list (or another data structure) of key-value pairs. When a collision occurs, the new key-value pair is simply added to the list at that index.
class HashTable {
constructor(size = 10) {
this.buckets = new Array(size).fill(null).map(() => []);
}
hash(key) {
let total = 0;
for (let i = 0; i < key.length; i++) {
total += key.charCodeAt(i);
}
return total % this.buckets.length;
}
set(key, value) {
const index = this.hash(key);
const bucket = this.buckets[index];
const found = bucket.find(item => item[0] === key);
if (found) {
found[1] = value;
} else {
bucket.push([key, value]);
}
}
get(key) {
const index = this.hash(key);
const found = this.buckets[index].find(item => item[0] === key);
return found ? found[1] : undefined;
}
}
2. Open Addressing
In open addressing, when a collision occurs, we search for the next available slot in the array. Common probing techniques include:
- Linear probing: Check the next slot sequentially
- Quadratic probing: Check slots at quadratic intervals
- Double hashing: Use a second hash function to determine the interval
class HashTable {
constructor(size = 10) {
this.size = size;
this.keys = new Array(size);
this.values = new Array(size);
}
hash(key) {
let total = 0;
for (let i = 0; i < key.length; i++) {
total += key.charCodeAt(i);
}
return total % this.size;
}
set(key, value) {
let index = this.hash(key);
while (this.keys[index] !== undefined) {
if (this.keys[index] === key) {
this.values[index] = value;
return;
}
index = (index + 1) % this.size;
}
this.keys[index] = key;
this.values[index] = value;
}
get(key) {
let index = this.hash(key);
while (this.keys[index] !== undefined) {
if (this.keys[index] === key) {
return this.values[index];
}
index = (index + 1) % this.size;
}
return undefined;
}
}
Time Complexity
The time complexity of hash table operations is as follows:
- Average case:
- Insertion: O(1)
- Deletion: O(1)
- Search: O(1)
- Worst case (rare, with a poor hash function):
- Insertion: O(n)
- Deletion: O(n)
- Search: O(n)
The constant-time average case is what makes hash tables so powerful and widely used.
Load Factor and Resizing
The load factor of a hash table is the ratio of the number of stored elements to the size of the hash table. As the load factor increases, the likelihood of collisions also increases, which can degrade performance.
To maintain good performance, hash tables typically resize themselves when the load factor exceeds a certain threshold (often 0.75). Resizing involves creating a new, larger array and rehashing all existing elements into the new array.
class HashTable {
constructor(initialSize = 16) {
this.size = initialSize;
this.count = 0;
this.buckets = new Array(this.size).fill(null).map(() => []);
}
hash(key) {
let total = 0;
for (let i = 0; i < key.length; i++) {
total += key.charCodeAt(i);
}
return total % this.size;
}
set(key, value) {
const index = this.hash(key);
const bucket = this.buckets[index];
const found = bucket.find(item => item[0] === key);
if (found) {
found[1] = value;
} else {
bucket.push([key, value]);
this.count++;
if (this.count / this.size > 0.75) {
this.resize();
}
}
}
get(key) {
const index = this.hash(key);
const found = this.buckets[index].find(item => item[0] === key);
return found ? found[1] : undefined;
}
resize() {
const newSize = this.size * 2;
const newBuckets = new Array(newSize).fill(null).map(() => []);
for (let i = 0; i < this.buckets.length; i++) {
for (let j = 0; j < this.buckets[i].length; j++) {
const [key, value] = this.buckets[i][j];
const newIndex = this.hash(key) % newSize;
newBuckets[newIndex].push([key, value]);
}
}
this.size = newSize;
this.buckets = newBuckets;
}
}
Applications of Hash Tables
Hash tables are used in a wide variety of applications, including:
- Database indexing: Hash tables can be used to create indexes for fast data retrieval in databases.
- Caching: Web browsers and other applications use hash tables to cache frequently accessed data.
- Symbol tables in compilers: Compilers use hash tables to store and quickly look up information about variables and functions.
- Associative arrays: Many programming languages implement associative arrays (dictionaries) using hash tables.
- Password storage: Secure systems store hashed passwords rather than plaintext passwords.
- Spell checkers: Hash tables can be used to efficiently store and look up words in a dictionary.
- File systems: Some file systems use hash tables to map file names to their locations on disk.
Implementing a Hash Table in Different Languages
Let’s look at how to implement a basic hash table in a few popular programming languages:
Python
In Python, dictionaries are built-in hash tables:
# Creating a dictionary
my_dict = {}
# Adding key-value pairs
my_dict["apple"] = 5
my_dict["banana"] = 7
# Retrieving values
print(my_dict["apple"]) # Output: 5
# Checking if a key exists
if "cherry" in my_dict:
print("Cherry is in the dictionary")
else:
print("Cherry is not in the dictionary")
# Iterating over keys and values
for key, value in my_dict.items():
print(f"{key}: {value}")
Java
Java provides the HashMap class for hash table functionality:
import java.util.HashMap;
public class HashTableExample {
public static void main(String[] args) {
// Creating a HashMap
HashMap<String, Integer> map = new HashMap<>();
// Adding key-value pairs
map.put("apple", 5);
map.put("banana", 7);
// Retrieving values
System.out.println(map.get("apple")); // Output: 5
// Checking if a key exists
if (map.containsKey("cherry")) {
System.out.println("Cherry is in the map");
} else {
System.out.println("Cherry is not in the map");
}
// Iterating over keys and values
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
JavaScript
In JavaScript, you can use objects or the Map class for hash table functionality:
// Using an object
const objHashTable = {};
objHashTable["apple"] = 5;
objHashTable["banana"] = 7;
console.log(objHashTable["apple"]); // Output: 5
// Using Map
const mapHashTable = new Map();
mapHashTable.set("apple", 5);
mapHashTable.set("banana", 7);
console.log(mapHashTable.get("apple")); // Output: 5
// Checking if a key exists
if (mapHashTable.has("cherry")) {
console.log("Cherry is in the map");
} else {
console.log("Cherry is not in the map");
}
// Iterating over keys and values
for (const [key, value] of mapHashTable) {
console.log(`${key}: ${value}`);
}
Advanced Topics in Hash Tables
Perfect Hashing
Perfect hashing is a technique used when the set of keys is known in advance and does not change. It guarantees O(1) worst-case lookup time by creating a collision-free hash table. This is achieved by using two levels of hashing:
- The first level uses a universal hash function to distribute keys into buckets.
- The second level uses a perfect hash function for each bucket to achieve collision-free storage.
Perfect hashing is memory-efficient and useful in scenarios like compiler design and static dictionaries.
Cuckoo Hashing
Cuckoo hashing is an open addressing scheme that uses two hash functions instead of one. When inserting a new key, if both possible positions are occupied, the algorithm moves existing keys to their alternate positions to make room for the new key. This process continues until all keys find a place or a cycle is detected (rare case, requiring a rebuild).
Cuckoo hashing provides worst-case O(1) lookup time and amortized O(1) insertion time, making it attractive for high-performance applications.
Bloom Filters
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set. It can have false positives but no false negatives. Bloom filters are used in applications where space is at a premium and occasional false positives are acceptable, such as:
- Caching systems to avoid expensive lookups for non-existent items
- Spell checkers to quickly identify words that are definitely not in the dictionary
- Network routers to avoid routing loops
Consistent Hashing
Consistent hashing is a technique used in distributed systems to minimize the number of keys that need to be remapped when the number of slots (e.g., servers) changes. It’s particularly useful in distributed caching systems and content delivery networks (CDNs).
The basic idea is to hash both the keys and the slots onto a circular space, and assign each key to the nearest slot in the clockwise direction. When a slot is added or removed, only the keys in its immediate vicinity need to be remapped.
Best Practices and Common Pitfalls
Best Practices
- Choose an appropriate initial size: Start with a reasonable size to avoid frequent resizing in the early stages.
- Use a good hash function: Ensure your hash function distributes keys uniformly to minimize collisions.
- Monitor load factor: Keep track of the load factor and resize when necessary to maintain performance.
- Handle collisions efficiently: Choose an appropriate collision resolution strategy for your use case.
- Use immutable keys: If possible, use immutable objects as keys to ensure consistent behavior.
Common Pitfalls
- Poor hash function: A weak hash function can lead to many collisions and degrade performance.
- Ignoring load factor: Failing to resize the hash table can result in increased collisions and slower operations.
- Mutable keys: Using mutable objects as keys can lead to unexpected behavior if the key’s hash value changes after insertion.
- Incorrect equality comparison: Ensure that your key objects correctly implement both hashCode() and equals() methods (in languages like Java).
- Not handling collisions: Failing to implement a collision resolution strategy can result in data loss or incorrect lookups.
Conclusion
Hash tables are a fundamental data structure in computer science, offering fast lookups, insertions, and deletions. Their ability to provide constant-time average-case complexity for these operations makes them invaluable in a wide range of applications, from database systems to caching mechanisms.
By understanding the principles behind hash tables, including hash functions, collision resolution strategies, and performance considerations, you can effectively use and implement them in your own projects. As you continue to explore advanced topics like perfect hashing, cuckoo hashing, and consistent hashing, you’ll gain a deeper appreciation for the versatility and power of this essential data structure.
Mastering hash tables is a crucial step in becoming a proficient programmer and computer scientist. They form the backbone of many high-performance systems and are a common topic in technical interviews at major tech companies. By thoroughly understanding hash tables, you’ll be well-equipped to tackle complex problems and design efficient solutions in your programming career.