The Importance of Recursion in Problem Solving
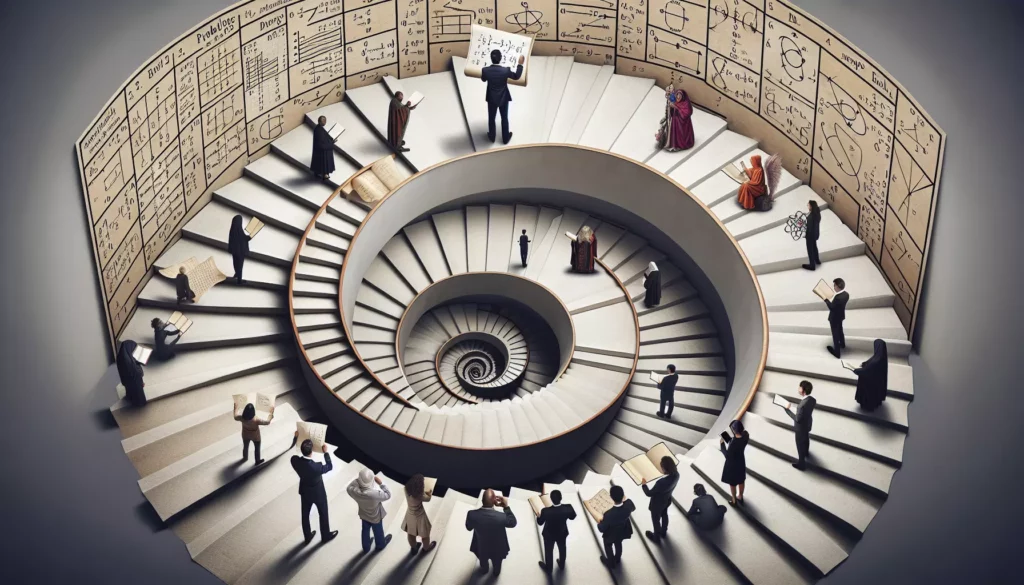
In the world of programming and computer science, recursion stands as a fundamental concept that plays a crucial role in problem-solving. As we delve into the realm of coding education and skills development, understanding recursion becomes increasingly important, especially when preparing for technical interviews at major tech companies. This article will explore the significance of recursion, its applications, and how mastering this concept can elevate your problem-solving abilities.
What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable subproblems. It’s a powerful approach that allows for elegant and concise solutions to complex problems. At its core, recursion involves two key components:
- Base case: The condition that stops the recursion
- Recursive case: The part where the function calls itself with a modified input
A classic example of recursion is the factorial function. Here’s a simple implementation in Python:
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else: # Recursive case
return n * factorial(n - 1)
In this example, the base case is when n is 0 or 1, and the recursive case multiplies n by the factorial of (n-1).
Why is Recursion Important in Problem Solving?
Recursion is not just a programming technique; it’s a powerful problem-solving tool that offers several advantages:
1. Simplifies Complex Problems
Recursion allows us to break down complex problems into smaller, more manageable subproblems. This divide-and-conquer approach can make seemingly insurmountable tasks much more approachable. For instance, traversing a complex data structure like a tree or graph becomes straightforward with recursion.
2. Leads to Elegant Solutions
Recursive solutions are often more concise and elegant than their iterative counterparts. They can express the nature of the problem more clearly, making the code easier to understand and maintain. Consider the Fibonacci sequence implementation:
def fibonacci(n):
if n <= 1: # Base case
return n
else: # Recursive case
return fibonacci(n - 1) + fibonacci(n - 2)
This recursive implementation captures the essence of the Fibonacci sequence in just a few lines of code.
3. Mirrors Natural Problem-Solving Processes
Many real-world problems have a recursive nature. For example, exploring a maze, organizing hierarchical data, or solving mathematical sequences often have inherent recursive structures. By using recursion, we can model these problems more naturally in our code.
4. Essential for Certain Algorithms and Data Structures
Some algorithms and data structures are inherently recursive and are best implemented using recursion. Examples include:
- Tree traversals (inorder, preorder, postorder)
- Depth-First Search (DFS) in graphs
- Divide and conquer algorithms (e.g., Quicksort, Merge Sort)
- Backtracking algorithms
5. Improves Problem-Solving Skills
Learning to think recursively enhances overall problem-solving abilities. It encourages breaking down problems, identifying patterns, and thinking about base cases and recursive steps. These skills are valuable not just in programming but in general problem-solving scenarios.
Common Applications of Recursion
Recursion finds applications in various areas of computer science and programming. Let’s explore some common use cases:
1. Tree and Graph Traversals
Recursion is particularly useful when working with hierarchical data structures like trees and graphs. For example, here’s a recursive implementation of an inorder traversal of a binary tree:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.value)
inorder_traversal(root.right)
2. Divide and Conquer Algorithms
Many efficient sorting and searching algorithms use a divide-and-conquer approach that is naturally recursive. The Quicksort algorithm is a prime example:
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
3. Dynamic Programming
While dynamic programming often uses iteration for efficiency, many problems are initially conceptualized recursively. The Fibonacci sequence, mentioned earlier, is a classic example that can be optimized using dynamic programming techniques.
4. Backtracking Algorithms
Backtracking algorithms, used in solving puzzles, path finding, and combinatorial optimization, often rely on recursion. Here’s a simple example of generating all possible subsets of a set:
def generate_subsets(nums):
def backtrack(start, current):
result.append(current[:])
for i in range(start, len(nums)):
current.append(nums[i])
backtrack(i + 1, current)
current.pop()
result = []
backtrack(0, [])
return result
5. Fractals and Computer Graphics
Recursion is often used in generating fractals and other complex geometric patterns. The Sierpinski Triangle is a classic example:
import turtle
def sierpinski(length, depth):
if depth == 0:
for _ in range(3):
turtle.forward(length)
turtle.left(120)
else:
sierpinski(length/2, depth-1)
turtle.forward(length/2)
sierpinski(length/2, depth-1)
turtle.backward(length/2)
turtle.left(60)
turtle.forward(length/2)
turtle.right(60)
sierpinski(length/2, depth-1)
turtle.left(60)
turtle.backward(length/2)
turtle.right(60)
Challenges and Considerations in Using Recursion
While recursion is a powerful tool, it comes with its own set of challenges and considerations:
1. Stack Overflow
Each recursive call adds a new layer to the call stack. If the recursion is too deep, it can lead to a stack overflow error. This is particularly problematic with languages that don’t optimize tail recursion.
2. Performance Overhead
Recursive functions can be less efficient than their iterative counterparts due to the overhead of multiple function calls. This is especially true for problems with simple recursive structures, like calculating factorials.
3. Difficulty in Debugging
Recursive functions can be more challenging to debug, as the state of the program at any given point depends on multiple nested function calls.
4. Risk of Infinite Recursion
If the base case is not properly defined or never reached, the function will recurse indefinitely, causing a stack overflow or program crash.
Best Practices for Using Recursion
To effectively use recursion in problem-solving, consider the following best practices:
1. Identify the Base Case
Always start by clearly defining the base case. This is crucial for preventing infinite recursion and ensuring your function terminates correctly.
2. Ensure Progress Towards the Base Case
Make sure that each recursive call brings you closer to the base case. This often involves reducing the size of the input or modifying a counter.
3. Think Recursively
When approaching a problem, try to identify its recursive nature. Ask yourself: “How can I break this problem into smaller, similar subproblems?”
4. Use Recursion Wisely
While recursion can lead to elegant solutions, it’s not always the best approach. Consider the trade-offs between recursion and iteration, especially for performance-critical code.
5. Optimize with Memoization
For recursive problems with overlapping subproblems, use memoization to store and reuse previously computed results. This can significantly improve performance.
6. Consider Tail Recursion
Where possible, use tail recursion (where the recursive call is the last operation in the function). Some languages and compilers can optimize tail-recursive functions to be as efficient as loops.
Recursion in Technical Interviews
Recursion is a favorite topic in technical interviews, especially at major tech companies. Here’s why it’s important and how to approach recursive problems in interviews:
Why Recursion is Important in Interviews
- Problem-solving skills: Recursion tests a candidate’s ability to break down complex problems into smaller, manageable parts.
- Algorithmic thinking: Many efficient algorithms for searching, sorting, and traversing data structures are recursive in nature.
- Code organization: Recursive solutions often lead to cleaner, more readable code, which is valued in professional settings.
- Understanding of stack and memory: Discussing recursion allows interviewers to assess a candidate’s understanding of function call stacks and memory management.
Common Recursive Problems in Interviews
Some typical recursive problems you might encounter in technical interviews include:
- Tree traversals and manipulations
- Graph traversals (DFS)
- Divide and conquer algorithms (e.g., binary search, merge sort)
- Backtracking problems (e.g., generating permutations, solving Sudoku)
- Dynamic programming problems (often introduced as recursive solutions before optimization)
Approaching Recursive Problems in Interviews
- Identify the base case: Start by clearly defining when the recursion should stop.
- Define the recursive case: Determine how the problem can be broken down and how the function should call itself.
- Trace the recursion: Walk through a small example to ensure your logic is correct.
- Consider edge cases: Think about potential issues like empty inputs or boundary conditions.
- Optimize if necessary: Discuss potential optimizations like memoization or converting to an iterative solution if appropriate.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your recursive solution.
Example Interview Question: Palindrome Checker
Let’s walk through a common recursive interview question: checking if a string is a palindrome.
def is_palindrome(s):
# Base cases
if len(s) <= 1:
return True
# Recursive case
if s[0] == s[-1]:
return is_palindrome(s[1:-1])
else:
return False
# Test cases
print(is_palindrome("racecar")) # True
print(is_palindrome("hello")) # False
print(is_palindrome("A man a plan a canal Panama")) # True (ignoring spaces and case)
In this solution:
- The base case is when the string has 0 or 1 character, which is always a palindrome.
- The recursive case checks if the first and last characters match, then recurs on the substring between them.
- If at any point the characters don’t match, it returns False.
When discussing this solution in an interview, you might talk about:
- How it handles different string lengths
- The time and space complexity (O(n) time, O(n) space due to the call stack)
- Potential optimizations (e.g., handling spaces and case sensitivity)
- How it compares to an iterative solution
Conclusion
Recursion is a powerful problem-solving technique that plays a crucial role in computer science and programming. Its ability to simplify complex problems, create elegant solutions, and mirror natural problem-solving processes makes it an essential skill for any programmer. While it comes with challenges like potential stack overflow issues and performance considerations, the benefits of mastering recursion far outweigh these drawbacks.
As you continue your journey in coding education and skills development, particularly in preparation for technical interviews at major tech companies, make sure to dedicate time to understanding and practicing recursive problem-solving. It will not only enhance your coding abilities but also strengthen your overall approach to tackling complex problems in any domain.
Remember, the key to mastering recursion is practice. Start with simple problems and gradually move to more complex ones. Analyze recursive solutions, understand their inner workings, and don’t hesitate to trace through the recursion manually to build your intuition. With time and effort, you’ll find that thinking recursively becomes second nature, opening up new avenues for elegant and efficient problem-solving in your programming career.