Introduction to Algorithms: What You Need to Know
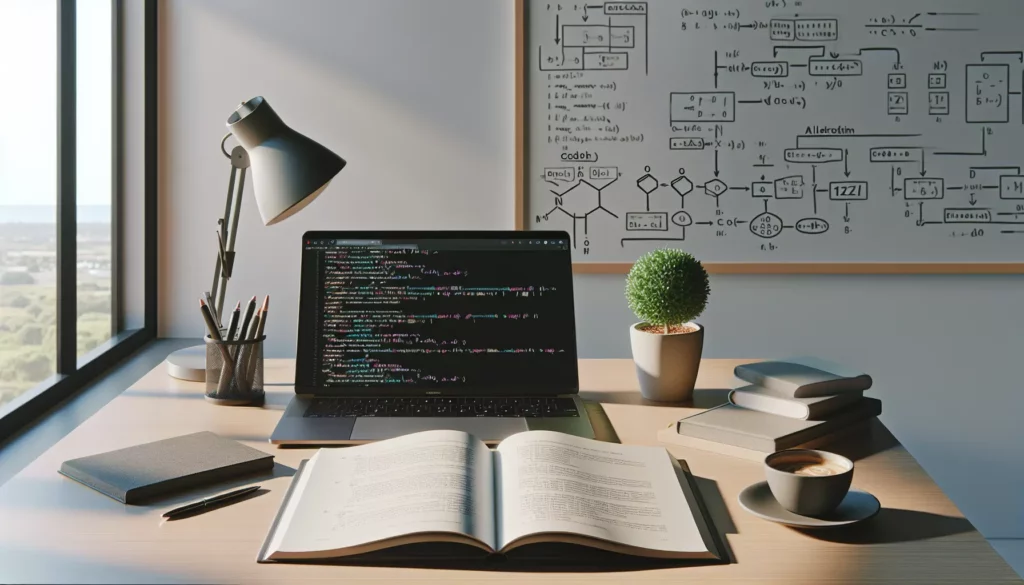
In the world of computer science and programming, algorithms are the backbone of efficient problem-solving. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding algorithms is crucial. This comprehensive guide will introduce you to the fundamental concepts of algorithms, their importance, and how they relate to coding education and skill development.
What Are Algorithms?
At its core, an algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In computer science, algorithms are precise sequences of instructions that tell a computer how to perform a specific operation or solve a particular problem.
Think of an algorithm as a recipe. Just as a recipe provides detailed instructions on how to prepare a dish, an algorithm provides a set of instructions for a computer to follow to achieve a desired outcome. These instructions can range from simple tasks like sorting a list of numbers to complex operations like analyzing large datasets or optimizing network traffic.
Why Are Algorithms Important?
Understanding algorithms is crucial for several reasons:
- Efficiency: Well-designed algorithms can significantly improve the speed and resource usage of your programs.
- Problem-solving: Algorithms provide structured approaches to tackle complex problems.
- Scalability: Efficient algorithms allow your programs to handle larger inputs and datasets without significant performance degradation.
- Interview preparation: Many technical interviews, especially at major tech companies, focus heavily on algorithmic problem-solving.
- Foundation for advanced concepts: A solid understanding of algorithms is essential for grasping more complex computer science topics.
Types of Algorithms
There are many types of algorithms, each designed to solve specific kinds of problems. Here are some of the most common categories:
1. Sorting Algorithms
Sorting algorithms arrange elements in a specific order, typically ascending or descending. Some popular sorting algorithms include:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
Here’s a simple example of the Bubble Sort algorithm implemented in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print(sorted_list) # Output: [11, 12, 22, 25, 34, 64, 90]
2. Searching Algorithms
Searching algorithms are used to find a specific element within a data structure. Common searching algorithms include:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Here’s an example of a Binary Search algorithm in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
}
if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Element not found
}
public static void main(String[] args) {
int[] arr = {2, 3, 4, 10, 40};
int target = 10;
int result = binarySearch(arr, target);
if (result == -1) {
System.out.println("Element not present");
} else {
System.out.println("Element found at index " + result);
}
}
}
3. Graph Algorithms
Graph algorithms are used to solve problems related to graph structures. Some important graph algorithms include:
- Dijkstra’s Algorithm (shortest path)
- Bellman-Ford Algorithm
- Kruskal’s Algorithm (minimum spanning tree)
- Prim’s Algorithm
- Topological Sorting
4. Dynamic Programming Algorithms
Dynamic programming is a technique for solving complex problems by breaking them down into simpler subproblems. It’s often used for optimization problems. Examples include:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack Problem
- Matrix Chain Multiplication
Here’s an example of a dynamic programming solution to the Fibonacci sequence in C++:
#include <iostream>
#include <vector>
using namespace std;
int fibonacci(int n) {
if (n <= 1) return n;
vector<int> fib(n + 1);
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i <= n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
return fib[n];
}
int main() {
int n = 10;
cout << "The " << n << "th Fibonacci number is: " << fibonacci(n) << endl;
return 0;
}
Algorithm Analysis
When studying algorithms, it’s crucial to understand how to analyze their performance. This analysis typically focuses on two main aspects:
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s usually expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time.
Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s often expressed using Big O notation.
Understanding both time and space complexity is crucial for writing efficient algorithms and choosing the right algorithm for a specific problem.
Algorithm Design Techniques
Several techniques can be employed when designing algorithms to solve complex problems efficiently:
1. Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them independently, and then combining the solutions. Examples include Merge Sort and Quick Sort.
2. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. They’re often used for optimization problems. Examples include Dijkstra’s algorithm and the Huffman coding algorithm.
3. Dynamic Programming
As mentioned earlier, dynamic programming solves complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems with overlapping subproblems.
4. Backtracking
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It’s often used for constraint satisfaction problems, such as the N-Queens problem or Sudoku solving.
Algorithms in Coding Education and Skill Development
Understanding algorithms is a crucial part of coding education and skill development. Here’s how algorithms fit into the broader context of learning to code:
1. Foundation for Problem-Solving
Algorithms provide a structured approach to problem-solving. Learning about algorithms helps developers think systematically about how to break down complex problems into manageable steps.
2. Efficiency and Optimization
Knowledge of algorithms allows developers to write more efficient code. Understanding the time and space complexity of different algorithms helps in choosing the most appropriate solution for a given problem.
3. Preparation for Technical Interviews
Many technical interviews, especially at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), focus heavily on algorithmic problem-solving. Being well-versed in algorithms is crucial for success in these interviews.
4. Language-Agnostic Skills
Algorithmic thinking is a skill that transcends specific programming languages. Once you understand the principles of algorithms, you can apply them in any programming language you work with.
5. Advanced Topic Understanding
A solid grasp of algorithms forms the foundation for understanding more advanced computer science topics like machine learning, artificial intelligence, and data science.
Learning Algorithms: Best Practices
If you’re looking to improve your algorithmic skills, here are some best practices to follow:
1. Start with the Basics
Begin with fundamental algorithms and data structures. Understand how they work and implement them from scratch in your preferred programming language.
2. Practice Regularly
Consistent practice is key to mastering algorithms. Solve problems on platforms like LeetCode, HackerRank, or CodeForces regularly.
3. Analyze Different Approaches
For each problem you solve, try to come up with multiple solutions. Analyze the time and space complexity of each approach to understand their trade-offs.
4. Study Algorithm Design Paradigms
Familiarize yourself with common algorithm design techniques like divide and conquer, dynamic programming, and greedy algorithms. Understanding these paradigms will help you approach a wide variety of problems.
5. Implement from Scratch
While many programming languages provide built-in implementations of common algorithms, it’s valuable to implement them yourself to truly understand how they work.
6. Use Visualization Tools
Algorithm visualizers can help you understand how algorithms work step-by-step. Tools like VisuAlgo or Algorithm Visualizer can be incredibly helpful.
7. Read and Analyze Code
Study implementations of algorithms written by others. This can expose you to different coding styles and potentially more efficient implementations.
8. Participate in Coding Competitions
Participating in coding competitions can help you apply your algorithmic knowledge under time pressure, simulating interview-like conditions.
Resources for Learning Algorithms
There are numerous resources available for learning about algorithms. Here are some recommendations:
Books:
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Grokking Algorithms” by Aditya Bhargava
Online Courses:
- Coursera: “Algorithms Specialization” by Stanford University
- edX: “Algorithms and Data Structures” by MIT
- Udacity: “Intro to Algorithms”
Websites and Platforms:
- LeetCode
- HackerRank
- CodeForces
- GeeksforGeeks
YouTube Channels:
- MIT OpenCourseWare
- mycodeschool
- Abdul Bari
Conclusion
Algorithms are a fundamental aspect of computer science and programming. They provide the tools and techniques necessary to solve complex problems efficiently. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews, a strong understanding of algorithms is crucial.
Remember that mastering algorithms is a gradual process that requires consistent practice and patience. Start with the basics, practice regularly, and gradually work your way up to more complex algorithms and problem-solving techniques. With dedication and the right resources, you can develop strong algorithmic thinking skills that will serve you well throughout your programming career.
As you continue your journey in coding education and skill development, keep in mind that understanding algorithms is just one piece of the puzzle. Combine your algorithmic knowledge with practical coding skills, software design principles, and an understanding of various programming paradigms to become a well-rounded and effective developer.
Happy coding, and may your algorithms always be efficient!