Why Programming Fundamentals Matter More Than Syntax
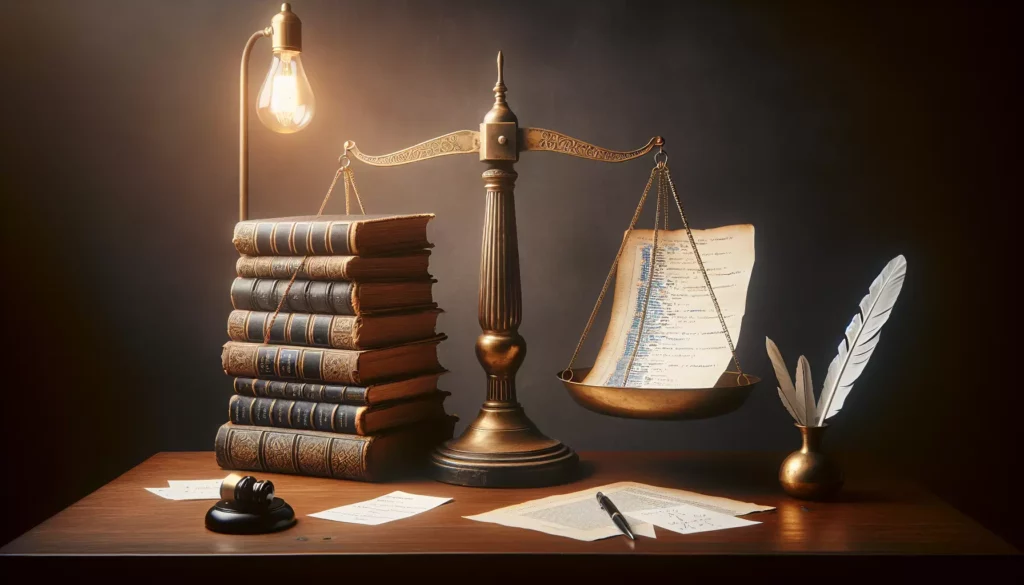
In the ever-evolving world of technology, aspiring programmers often find themselves caught up in the details of specific programming languages and their unique syntaxes. While mastering syntax is undoubtedly important, it’s crucial to understand that the true essence of programming lies in its fundamental concepts and problem-solving approaches. This article will explore why focusing on programming fundamentals is more valuable than getting bogged down in the intricacies of syntax, especially for those looking to build a strong foundation in coding and prepare for technical interviews at top tech companies.
The Essence of Programming: Beyond the Code
At its core, programming is about solving problems. It’s about taking complex challenges and breaking them down into manageable pieces that can be addressed systematically. This process requires critical thinking, logical reasoning, and creativity – skills that transcend any specific programming language.
When we focus too heavily on syntax, we risk losing sight of these essential problem-solving skills. It’s like learning to write by memorizing the spelling of words without understanding how to construct sentences or convey ideas effectively. Sure, you might be able to write individual words correctly, but you’d struggle to communicate complex thoughts or tell a compelling story.
The Transferability of Fundamental Concepts
One of the most significant advantages of mastering programming fundamentals is their transferability across different languages and platforms. Core concepts like variables, data structures, algorithms, and control flow remain consistent, even as the specific syntax for implementing them may vary.
For example, consider the concept of a loop. Whether you’re using a for loop in C++, a while loop in Python, or a forEach method in JavaScript, the underlying principle remains the same: repeating a set of instructions until a condition is met. Once you understand this fundamental concept, adapting to the specific syntax of any language becomes a much simpler task.
Example: Looping in Different Languages
// C++
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
# Python
for i in range(5):
print(i)
// JavaScript
for (let i = 0; i < 5; i++) {
console.log(i);
}
As you can see, while the syntax differs, the core concept of looping remains consistent across languages.
Problem-Solving: The Heart of Programming
When it comes to real-world programming challenges and technical interviews, particularly at top tech companies, the focus is rarely on perfect syntax. Instead, these assessments are designed to evaluate your problem-solving abilities, algorithmic thinking, and understanding of fundamental concepts.
Consider a common interview question: reversing a linked list. The interviewer is less interested in whether you can write syntactically perfect code and more concerned with:
- Do you understand what a linked list is?
- Can you visualize the problem and break it down into steps?
- Are you able to articulate your thought process clearly?
- Can you optimize your solution and discuss its time and space complexity?
These aspects all stem from a strong grasp of programming fundamentals rather than memorization of syntax.
The Role of Algorithmic Thinking
Algorithmic thinking is a critical skill that forms the backbone of effective programming. It involves the ability to approach problems methodically, devise step-by-step solutions, and optimize these solutions for efficiency. This skill is language-agnostic and is far more valuable than knowing the exact syntax for a particular operation in a specific language.
For instance, understanding the concept of binary search and its logarithmic time complexity is far more crucial than memorizing the exact syntax for implementing it in Java or Python. Once you grasp the algorithm, implementing it in any language becomes a matter of translating your logical steps into code, which can be easily looked up or auto-completed by modern IDEs.
Example: Binary Search Concept
function binarySearch(arr, target):
left = 0
right = length(arr) - 1
while left <= right:
mid = (left + right) / 2
if arr[mid] == target:
return mid
else if arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Target not found
This pseudocode demonstrates the binary search algorithm without being tied to any specific programming language syntax. The focus is on the logic and steps of the algorithm, which can be adapted to any language once understood.
Adapting to New Technologies
The tech industry is known for its rapid evolution. New programming languages, frameworks, and tools emerge regularly. For a programmer with a strong foundation in the fundamentals, adapting to these changes is significantly easier.
When you understand core concepts like object-oriented programming, functional programming, or asynchronous operations, learning a new language or framework becomes a matter of understanding its unique features and syntax, rather than learning everything from scratch. This adaptability is crucial in a field where staying current is essential for career growth.
The Importance of Data Structures
A solid understanding of data structures is another fundamental aspect of programming that far outweighs the importance of syntax. Knowing when and how to use arrays, linked lists, trees, graphs, hash tables, and other data structures is crucial for writing efficient and scalable code.
For example, understanding the characteristics and use cases of a hash table (fast lookups, insertions, and deletions) is more important than memorizing the exact syntax for creating one in C++ or Java. With this knowledge, you can choose the right data structure for your problem, regardless of the language you’re using.
Example: Choosing the Right Data Structure
// Problem: Implement a spell-checker
// Inefficient approach using an array
function checkSpelling(word, dictionary):
for each entry in dictionary:
if entry == word:
return true
return false
// Efficient approach using a hash set
function checkSpelling(word, dictionarySet):
return word in dictionarySet
In this example, understanding that a hash set provides O(1) average-case lookup time, compared to O(n) for an array, is more valuable than knowing the exact syntax for implementing either solution.
The Power of Abstraction
Abstraction is a fundamental concept in programming that allows us to manage complexity by hiding unnecessary details and exposing only the essential features of an object or system. This concept is crucial in designing large-scale systems and writing maintainable code.
Understanding abstraction helps programmers think at a higher level, focusing on the overall architecture and design patterns rather than getting lost in the minutiae of implementation details. This skill is particularly valuable when working on complex projects or in team environments where clear communication of ideas is essential.
Example: Abstraction in Object-Oriented Programming
// Abstract concept of a Shape
abstract class Shape {
abstract double area();
abstract double perimeter();
}
// Concrete implementation for a Circle
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double area() {
return Math.PI * radius * radius;
}
@Override
double perimeter() {
return 2 * Math.PI * radius;
}
}
This example demonstrates the concept of abstraction in object-oriented programming. The focus is on understanding the principle rather than the specific syntax of Java or any other language.
Debugging and Problem-Solving Skills
Another critical aspect of programming that relies more on fundamental understanding than syntax is debugging. Effective debugging requires a deep understanding of how programs work, how data flows through a system, and how to isolate and identify issues.
Debugging skills are built on a foundation of logical thinking, systematic problem-solving, and a thorough understanding of programming concepts. These skills are transferable across languages and are often more valuable than the ability to write syntactically perfect code on the first try.
The Role of Design Patterns
Design patterns are reusable solutions to common problems in software design. Understanding these patterns and when to apply them is a fundamental skill that goes beyond syntax. Design patterns help in creating more maintainable, scalable, and robust code.
For example, knowing when to use the Singleton pattern, the Observer pattern, or the Factory pattern is more important than memorizing their exact implementation in a specific language. These patterns represent best practices developed by experienced programmers over time and are applicable across different programming paradigms and languages.
Example: Factory Pattern Concept
// Factory interface
interface AnimalFactory {
Animal createAnimal();
}
// Concrete factories
class DogFactory implements AnimalFactory {
@Override
public Animal createAnimal() {
return new Dog();
}
}
class CatFactory implements AnimalFactory {
@Override
public Animal createAnimal() {
return new Cat();
}
}
// Usage
AnimalFactory factory = new DogFactory();
Animal animal = factory.createAnimal();
This example illustrates the concept of the Factory pattern without being tied to a specific language’s syntax. The focus is on understanding the pattern and its benefits, such as encapsulating object creation and promoting loose coupling.
The Importance of Clean Code and Best Practices
Writing clean, readable, and maintainable code is a fundamental skill that goes beyond syntax. It involves understanding principles like DRY (Don’t Repeat Yourself), SOLID principles, and the importance of meaningful naming conventions. These concepts are universal and apply regardless of the programming language being used.
For instance, understanding the Single Responsibility Principle (the ‘S’ in SOLID) helps in designing more modular and maintainable code, regardless of whether you’re working in Python, Java, or any other language.
Preparing for Technical Interviews
When preparing for technical interviews, especially at top tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), focusing on fundamentals is crucial. These companies are known for asking questions that test a candidate’s problem-solving abilities and understanding of core concepts rather than their knowledge of specific language syntax.
Interviewers are often more interested in your approach to solving problems, your ability to analyze time and space complexity, and your understanding of data structures and algorithms. They may even allow you to write pseudocode or use a language of your choice, emphasizing the importance of logic over syntax.
The Role of Continuous Learning
In the fast-paced world of technology, continuous learning is essential. By focusing on fundamental concepts, programmers build a strong foundation that makes it easier to adapt to new technologies and paradigms. This adaptability is crucial for long-term success in the field.
While new languages and frameworks may come and go, the underlying principles of computer science and software engineering remain relatively constant. Investing time in understanding these fundamentals pays dividends throughout a programmer’s career.
Balancing Fundamentals and Practical Skills
While this article emphasizes the importance of fundamentals over syntax, it’s important to note that practical coding skills are also crucial. The key is to find a balance. As you learn and practice coding, focus on understanding the underlying concepts while also gaining hands-on experience with real-world programming tasks.
Platforms like AlgoCademy recognize this need for balance. They provide interactive coding tutorials and resources that not only teach syntax but also emphasize algorithmic thinking and problem-solving skills. Features like AI-powered assistance and step-by-step guidance help learners connect theoretical concepts with practical application.
Conclusion
In the journey of becoming a proficient programmer, understanding and mastering the fundamental concepts of programming is far more valuable than focusing solely on the syntax of specific languages. These fundamentals – problem-solving skills, algorithmic thinking, data structures, and design patterns – form the bedrock of programming expertise.
By prioritizing these core concepts, aspiring programmers can:
- Develop transferable skills that apply across multiple languages and platforms
- Improve their problem-solving abilities, crucial for technical interviews and real-world challenges
- Adapt more easily to new technologies and programming paradigms
- Write more efficient, maintainable, and scalable code
- Build a strong foundation for continuous learning and growth in the field
While syntax is important and necessary for writing functional code, it should be seen as a tool for implementing your ideas rather than the focus of your learning. Remember, syntax can be easily referenced or auto-completed, but the ability to think like a programmer and solve complex problems is what truly sets apart great developers.
As you continue your programming journey, whether you’re preparing for technical interviews, working on personal projects, or advancing your career, keep in mind that the fundamental concepts you learn will serve you well across all aspects of software development. Embrace the challenge of mastering these fundamentals, and you’ll be well-equipped to tackle any programming task that comes your way.